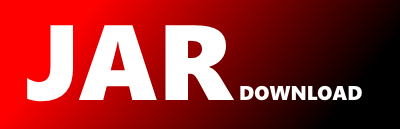
org.openmetadata.schema.service.configuration.slackApp.SlackAppConfiguration Maven / Gradle / Ivy
package org.openmetadata.schema.service.configuration.slackApp;
import javax.annotation.processing.Generated;
import javax.validation.constraints.NotNull;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* SlackAppConfiguration
*
* This schema defines the Slack App Information
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"clientId",
"clientSecret",
"signingSecret",
"scopes",
"callbackUrl",
"callbackRedirectURL"
})
@Generated("jsonschema2pojo")
public class SlackAppConfiguration {
/**
* Client Id of the Application
* (Required)
*
*/
@JsonProperty("clientId")
@JsonPropertyDescription("Client Id of the Application")
@NotNull
private String clientId;
/**
* Client Secret of the Application.
* (Required)
*
*/
@JsonProperty("clientSecret")
@JsonPropertyDescription("Client Secret of the Application.")
@NotNull
private String clientSecret;
/**
* Signing Secret of the Application. Confirm that each request comes from Slack by verifying its unique signature.
* (Required)
*
*/
@JsonProperty("signingSecret")
@JsonPropertyDescription("Signing Secret of the Application. Confirm that each request comes from Slack by verifying its unique signature.")
@NotNull
private String signingSecret;
/**
* Scopes to Request in OAuth
* (Required)
*
*/
@JsonProperty("scopes")
@JsonPropertyDescription("Scopes to Request in OAuth")
@NotNull
private String scopes;
/**
* The callback URL where temporary authorization code is exchanged for access tokens
* (Required)
*
*/
@JsonProperty("callbackUrl")
@JsonPropertyDescription("The callback URL where temporary authorization code is exchanged for access tokens")
@NotNull
private String callbackUrl;
/**
* The URL where the application redirects after handling the OAuth callback
* (Required)
*
*/
@JsonProperty("callbackRedirectURL")
@JsonPropertyDescription("The URL where the application redirects after handling the OAuth callback")
@NotNull
private String callbackRedirectURL;
/**
* Client Id of the Application
* (Required)
*
*/
@JsonProperty("clientId")
public String getClientId() {
return clientId;
}
/**
* Client Id of the Application
* (Required)
*
*/
@JsonProperty("clientId")
public void setClientId(String clientId) {
this.clientId = clientId;
}
public SlackAppConfiguration withClientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Client Secret of the Application.
* (Required)
*
*/
@JsonProperty("clientSecret")
public String getClientSecret() {
return clientSecret;
}
/**
* Client Secret of the Application.
* (Required)
*
*/
@JsonProperty("clientSecret")
public void setClientSecret(String clientSecret) {
this.clientSecret = clientSecret;
}
public SlackAppConfiguration withClientSecret(String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/**
* Signing Secret of the Application. Confirm that each request comes from Slack by verifying its unique signature.
* (Required)
*
*/
@JsonProperty("signingSecret")
public String getSigningSecret() {
return signingSecret;
}
/**
* Signing Secret of the Application. Confirm that each request comes from Slack by verifying its unique signature.
* (Required)
*
*/
@JsonProperty("signingSecret")
public void setSigningSecret(String signingSecret) {
this.signingSecret = signingSecret;
}
public SlackAppConfiguration withSigningSecret(String signingSecret) {
this.signingSecret = signingSecret;
return this;
}
/**
* Scopes to Request in OAuth
* (Required)
*
*/
@JsonProperty("scopes")
public String getScopes() {
return scopes;
}
/**
* Scopes to Request in OAuth
* (Required)
*
*/
@JsonProperty("scopes")
public void setScopes(String scopes) {
this.scopes = scopes;
}
public SlackAppConfiguration withScopes(String scopes) {
this.scopes = scopes;
return this;
}
/**
* The callback URL where temporary authorization code is exchanged for access tokens
* (Required)
*
*/
@JsonProperty("callbackUrl")
public String getCallbackUrl() {
return callbackUrl;
}
/**
* The callback URL where temporary authorization code is exchanged for access tokens
* (Required)
*
*/
@JsonProperty("callbackUrl")
public void setCallbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
}
public SlackAppConfiguration withCallbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
return this;
}
/**
* The URL where the application redirects after handling the OAuth callback
* (Required)
*
*/
@JsonProperty("callbackRedirectURL")
public String getCallbackRedirectURL() {
return callbackRedirectURL;
}
/**
* The URL where the application redirects after handling the OAuth callback
* (Required)
*
*/
@JsonProperty("callbackRedirectURL")
public void setCallbackRedirectURL(String callbackRedirectURL) {
this.callbackRedirectURL = callbackRedirectURL;
}
public SlackAppConfiguration withCallbackRedirectURL(String callbackRedirectURL) {
this.callbackRedirectURL = callbackRedirectURL;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(SlackAppConfiguration.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("clientId");
sb.append('=');
sb.append(((this.clientId == null)?"":this.clientId));
sb.append(',');
sb.append("clientSecret");
sb.append('=');
sb.append(((this.clientSecret == null)?"":this.clientSecret));
sb.append(',');
sb.append("signingSecret");
sb.append('=');
sb.append(((this.signingSecret == null)?"":this.signingSecret));
sb.append(',');
sb.append("scopes");
sb.append('=');
sb.append(((this.scopes == null)?"":this.scopes));
sb.append(',');
sb.append("callbackUrl");
sb.append('=');
sb.append(((this.callbackUrl == null)?"":this.callbackUrl));
sb.append(',');
sb.append("callbackRedirectURL");
sb.append('=');
sb.append(((this.callbackRedirectURL == null)?"":this.callbackRedirectURL));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.callbackRedirectURL == null)? 0 :this.callbackRedirectURL.hashCode()));
result = ((result* 31)+((this.clientId == null)? 0 :this.clientId.hashCode()));
result = ((result* 31)+((this.signingSecret == null)? 0 :this.signingSecret.hashCode()));
result = ((result* 31)+((this.clientSecret == null)? 0 :this.clientSecret.hashCode()));
result = ((result* 31)+((this.callbackUrl == null)? 0 :this.callbackUrl.hashCode()));
result = ((result* 31)+((this.scopes == null)? 0 :this.scopes.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof SlackAppConfiguration) == false) {
return false;
}
SlackAppConfiguration rhs = ((SlackAppConfiguration) other);
return (((((((this.callbackRedirectURL == rhs.callbackRedirectURL)||((this.callbackRedirectURL!= null)&&this.callbackRedirectURL.equals(rhs.callbackRedirectURL)))&&((this.clientId == rhs.clientId)||((this.clientId!= null)&&this.clientId.equals(rhs.clientId))))&&((this.signingSecret == rhs.signingSecret)||((this.signingSecret!= null)&&this.signingSecret.equals(rhs.signingSecret))))&&((this.clientSecret == rhs.clientSecret)||((this.clientSecret!= null)&&this.clientSecret.equals(rhs.clientSecret))))&&((this.callbackUrl == rhs.callbackUrl)||((this.callbackUrl!= null)&&this.callbackUrl.equals(rhs.callbackUrl))))&&((this.scopes == rhs.scopes)||((this.scopes!= null)&&this.scopes.equals(rhs.scopes))));
}
}