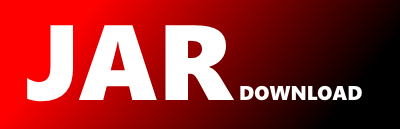
org.openmetadata.schema.api.events.EventSubscriptionDiagnosticInfo Maven / Gradle / Ivy
package org.openmetadata.schema.api.events;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.openmetadata.schema.type.ChangeEvent;
/**
* Event Subscription Diagnostic Info
*
* Schema defining the response for event subscription diagnostics, including details about processed and unprocessed events.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"latestOffset",
"currentOffset",
"startingOffset",
"hasProcessedAllEvents",
"successfulEventsCount",
"failedEventsCount",
"relevantUnprocessedEventsCount",
"totalUnprocessedEventsCount",
"relevantUnprocessedEventsList",
"totalUnprocessedEventsList"
})
@Generated("jsonschema2pojo")
public class EventSubscriptionDiagnosticInfo {
/**
* The latest offset of the event in the system.
*
*/
@JsonProperty("latestOffset")
@JsonPropertyDescription("The latest offset of the event in the system.")
private Long latestOffset;
/**
* The current offset of the event subscription.
*
*/
@JsonProperty("currentOffset")
@JsonPropertyDescription("The current offset of the event subscription.")
private Long currentOffset;
/**
* The initial offset of the event subscription when it started processing.
*
*/
@JsonProperty("startingOffset")
@JsonPropertyDescription("The initial offset of the event subscription when it started processing.")
private Long startingOffset;
/**
* Indicates whether all events have been processed.
*
*/
@JsonProperty("hasProcessedAllEvents")
@JsonPropertyDescription("Indicates whether all events have been processed.")
private Boolean hasProcessedAllEvents;
/**
* Count of successful events for specific alert.
*
*/
@JsonProperty("successfulEventsCount")
@JsonPropertyDescription("Count of successful events for specific alert.")
private Long successfulEventsCount;
/**
* Count of failed events for specific alert.
*
*/
@JsonProperty("failedEventsCount")
@JsonPropertyDescription("Count of failed events for specific alert.")
private Long failedEventsCount;
/**
* The number of relevant unprocessed events based on the alert's filtering rules for specific alert.
*
*/
@JsonProperty("relevantUnprocessedEventsCount")
@JsonPropertyDescription("The number of relevant unprocessed events based on the alert's filtering rules for specific alert.")
private Long relevantUnprocessedEventsCount;
/**
* The total number of unprocessed events.
*
*/
@JsonProperty("totalUnprocessedEventsCount")
@JsonPropertyDescription("The total number of unprocessed events.")
private Long totalUnprocessedEventsCount;
/**
* A list of relevant unprocessed events based on the alert's filtering criteria.
*
*/
@JsonProperty("relevantUnprocessedEventsList")
@JsonPropertyDescription("A list of relevant unprocessed events based on the alert's filtering criteria.")
@Valid
private List relevantUnprocessedEventsList = new ArrayList();
/**
* A list of all unprocessed events.
*
*/
@JsonProperty("totalUnprocessedEventsList")
@JsonPropertyDescription("A list of all unprocessed events.")
@Valid
private List totalUnprocessedEventsList = new ArrayList();
/**
* The latest offset of the event in the system.
*
*/
@JsonProperty("latestOffset")
public Long getLatestOffset() {
return latestOffset;
}
/**
* The latest offset of the event in the system.
*
*/
@JsonProperty("latestOffset")
public void setLatestOffset(Long latestOffset) {
this.latestOffset = latestOffset;
}
public EventSubscriptionDiagnosticInfo withLatestOffset(Long latestOffset) {
this.latestOffset = latestOffset;
return this;
}
/**
* The current offset of the event subscription.
*
*/
@JsonProperty("currentOffset")
public Long getCurrentOffset() {
return currentOffset;
}
/**
* The current offset of the event subscription.
*
*/
@JsonProperty("currentOffset")
public void setCurrentOffset(Long currentOffset) {
this.currentOffset = currentOffset;
}
public EventSubscriptionDiagnosticInfo withCurrentOffset(Long currentOffset) {
this.currentOffset = currentOffset;
return this;
}
/**
* The initial offset of the event subscription when it started processing.
*
*/
@JsonProperty("startingOffset")
public Long getStartingOffset() {
return startingOffset;
}
/**
* The initial offset of the event subscription when it started processing.
*
*/
@JsonProperty("startingOffset")
public void setStartingOffset(Long startingOffset) {
this.startingOffset = startingOffset;
}
public EventSubscriptionDiagnosticInfo withStartingOffset(Long startingOffset) {
this.startingOffset = startingOffset;
return this;
}
/**
* Indicates whether all events have been processed.
*
*/
@JsonProperty("hasProcessedAllEvents")
public Boolean getHasProcessedAllEvents() {
return hasProcessedAllEvents;
}
/**
* Indicates whether all events have been processed.
*
*/
@JsonProperty("hasProcessedAllEvents")
public void setHasProcessedAllEvents(Boolean hasProcessedAllEvents) {
this.hasProcessedAllEvents = hasProcessedAllEvents;
}
public EventSubscriptionDiagnosticInfo withHasProcessedAllEvents(Boolean hasProcessedAllEvents) {
this.hasProcessedAllEvents = hasProcessedAllEvents;
return this;
}
/**
* Count of successful events for specific alert.
*
*/
@JsonProperty("successfulEventsCount")
public Long getSuccessfulEventsCount() {
return successfulEventsCount;
}
/**
* Count of successful events for specific alert.
*
*/
@JsonProperty("successfulEventsCount")
public void setSuccessfulEventsCount(Long successfulEventsCount) {
this.successfulEventsCount = successfulEventsCount;
}
public EventSubscriptionDiagnosticInfo withSuccessfulEventsCount(Long successfulEventsCount) {
this.successfulEventsCount = successfulEventsCount;
return this;
}
/**
* Count of failed events for specific alert.
*
*/
@JsonProperty("failedEventsCount")
public Long getFailedEventsCount() {
return failedEventsCount;
}
/**
* Count of failed events for specific alert.
*
*/
@JsonProperty("failedEventsCount")
public void setFailedEventsCount(Long failedEventsCount) {
this.failedEventsCount = failedEventsCount;
}
public EventSubscriptionDiagnosticInfo withFailedEventsCount(Long failedEventsCount) {
this.failedEventsCount = failedEventsCount;
return this;
}
/**
* The number of relevant unprocessed events based on the alert's filtering rules for specific alert.
*
*/
@JsonProperty("relevantUnprocessedEventsCount")
public Long getRelevantUnprocessedEventsCount() {
return relevantUnprocessedEventsCount;
}
/**
* The number of relevant unprocessed events based on the alert's filtering rules for specific alert.
*
*/
@JsonProperty("relevantUnprocessedEventsCount")
public void setRelevantUnprocessedEventsCount(Long relevantUnprocessedEventsCount) {
this.relevantUnprocessedEventsCount = relevantUnprocessedEventsCount;
}
public EventSubscriptionDiagnosticInfo withRelevantUnprocessedEventsCount(Long relevantUnprocessedEventsCount) {
this.relevantUnprocessedEventsCount = relevantUnprocessedEventsCount;
return this;
}
/**
* The total number of unprocessed events.
*
*/
@JsonProperty("totalUnprocessedEventsCount")
public Long getTotalUnprocessedEventsCount() {
return totalUnprocessedEventsCount;
}
/**
* The total number of unprocessed events.
*
*/
@JsonProperty("totalUnprocessedEventsCount")
public void setTotalUnprocessedEventsCount(Long totalUnprocessedEventsCount) {
this.totalUnprocessedEventsCount = totalUnprocessedEventsCount;
}
public EventSubscriptionDiagnosticInfo withTotalUnprocessedEventsCount(Long totalUnprocessedEventsCount) {
this.totalUnprocessedEventsCount = totalUnprocessedEventsCount;
return this;
}
/**
* A list of relevant unprocessed events based on the alert's filtering criteria.
*
*/
@JsonProperty("relevantUnprocessedEventsList")
public List getRelevantUnprocessedEventsList() {
return relevantUnprocessedEventsList;
}
/**
* A list of relevant unprocessed events based on the alert's filtering criteria.
*
*/
@JsonProperty("relevantUnprocessedEventsList")
public void setRelevantUnprocessedEventsList(List relevantUnprocessedEventsList) {
this.relevantUnprocessedEventsList = relevantUnprocessedEventsList;
}
public EventSubscriptionDiagnosticInfo withRelevantUnprocessedEventsList(List relevantUnprocessedEventsList) {
this.relevantUnprocessedEventsList = relevantUnprocessedEventsList;
return this;
}
/**
* A list of all unprocessed events.
*
*/
@JsonProperty("totalUnprocessedEventsList")
public List getTotalUnprocessedEventsList() {
return totalUnprocessedEventsList;
}
/**
* A list of all unprocessed events.
*
*/
@JsonProperty("totalUnprocessedEventsList")
public void setTotalUnprocessedEventsList(List totalUnprocessedEventsList) {
this.totalUnprocessedEventsList = totalUnprocessedEventsList;
}
public EventSubscriptionDiagnosticInfo withTotalUnprocessedEventsList(List totalUnprocessedEventsList) {
this.totalUnprocessedEventsList = totalUnprocessedEventsList;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(EventSubscriptionDiagnosticInfo.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("latestOffset");
sb.append('=');
sb.append(((this.latestOffset == null)?"":this.latestOffset));
sb.append(',');
sb.append("currentOffset");
sb.append('=');
sb.append(((this.currentOffset == null)?"":this.currentOffset));
sb.append(',');
sb.append("startingOffset");
sb.append('=');
sb.append(((this.startingOffset == null)?"":this.startingOffset));
sb.append(',');
sb.append("hasProcessedAllEvents");
sb.append('=');
sb.append(((this.hasProcessedAllEvents == null)?"":this.hasProcessedAllEvents));
sb.append(',');
sb.append("successfulEventsCount");
sb.append('=');
sb.append(((this.successfulEventsCount == null)?"":this.successfulEventsCount));
sb.append(',');
sb.append("failedEventsCount");
sb.append('=');
sb.append(((this.failedEventsCount == null)?"":this.failedEventsCount));
sb.append(',');
sb.append("relevantUnprocessedEventsCount");
sb.append('=');
sb.append(((this.relevantUnprocessedEventsCount == null)?"":this.relevantUnprocessedEventsCount));
sb.append(',');
sb.append("totalUnprocessedEventsCount");
sb.append('=');
sb.append(((this.totalUnprocessedEventsCount == null)?"":this.totalUnprocessedEventsCount));
sb.append(',');
sb.append("relevantUnprocessedEventsList");
sb.append('=');
sb.append(((this.relevantUnprocessedEventsList == null)?"":this.relevantUnprocessedEventsList));
sb.append(',');
sb.append("totalUnprocessedEventsList");
sb.append('=');
sb.append(((this.totalUnprocessedEventsList == null)?"":this.totalUnprocessedEventsList));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.hasProcessedAllEvents == null)? 0 :this.hasProcessedAllEvents.hashCode()));
result = ((result* 31)+((this.totalUnprocessedEventsCount == null)? 0 :this.totalUnprocessedEventsCount.hashCode()));
result = ((result* 31)+((this.successfulEventsCount == null)? 0 :this.successfulEventsCount.hashCode()));
result = ((result* 31)+((this.totalUnprocessedEventsList == null)? 0 :this.totalUnprocessedEventsList.hashCode()));
result = ((result* 31)+((this.startingOffset == null)? 0 :this.startingOffset.hashCode()));
result = ((result* 31)+((this.currentOffset == null)? 0 :this.currentOffset.hashCode()));
result = ((result* 31)+((this.failedEventsCount == null)? 0 :this.failedEventsCount.hashCode()));
result = ((result* 31)+((this.relevantUnprocessedEventsCount == null)? 0 :this.relevantUnprocessedEventsCount.hashCode()));
result = ((result* 31)+((this.relevantUnprocessedEventsList == null)? 0 :this.relevantUnprocessedEventsList.hashCode()));
result = ((result* 31)+((this.latestOffset == null)? 0 :this.latestOffset.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof EventSubscriptionDiagnosticInfo) == false) {
return false;
}
EventSubscriptionDiagnosticInfo rhs = ((EventSubscriptionDiagnosticInfo) other);
return (((((((((((this.hasProcessedAllEvents == rhs.hasProcessedAllEvents)||((this.hasProcessedAllEvents!= null)&&this.hasProcessedAllEvents.equals(rhs.hasProcessedAllEvents)))&&((this.totalUnprocessedEventsCount == rhs.totalUnprocessedEventsCount)||((this.totalUnprocessedEventsCount!= null)&&this.totalUnprocessedEventsCount.equals(rhs.totalUnprocessedEventsCount))))&&((this.successfulEventsCount == rhs.successfulEventsCount)||((this.successfulEventsCount!= null)&&this.successfulEventsCount.equals(rhs.successfulEventsCount))))&&((this.totalUnprocessedEventsList == rhs.totalUnprocessedEventsList)||((this.totalUnprocessedEventsList!= null)&&this.totalUnprocessedEventsList.equals(rhs.totalUnprocessedEventsList))))&&((this.startingOffset == rhs.startingOffset)||((this.startingOffset!= null)&&this.startingOffset.equals(rhs.startingOffset))))&&((this.currentOffset == rhs.currentOffset)||((this.currentOffset!= null)&&this.currentOffset.equals(rhs.currentOffset))))&&((this.failedEventsCount == rhs.failedEventsCount)||((this.failedEventsCount!= null)&&this.failedEventsCount.equals(rhs.failedEventsCount))))&&((this.relevantUnprocessedEventsCount == rhs.relevantUnprocessedEventsCount)||((this.relevantUnprocessedEventsCount!= null)&&this.relevantUnprocessedEventsCount.equals(rhs.relevantUnprocessedEventsCount))))&&((this.relevantUnprocessedEventsList == rhs.relevantUnprocessedEventsList)||((this.relevantUnprocessedEventsList!= null)&&this.relevantUnprocessedEventsList.equals(rhs.relevantUnprocessedEventsList))))&&((this.latestOffset == rhs.latestOffset)||((this.latestOffset!= null)&&this.latestOffset.equals(rhs.latestOffset))));
}
}