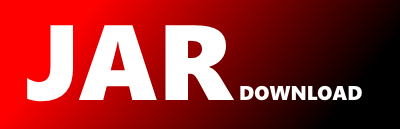
org.openmetadata.schema.dataInsight.custom.FormulaHolder Maven / Gradle / Ivy
package org.openmetadata.schema.dataInsight.custom;
import javax.annotation.processing.Generated;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* formulaHolder
*
* formulaHolder
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"formula",
"function",
"field",
"query"
})
@Generated("jsonschema2pojo")
public class FormulaHolder {
/**
* Formula
*
*/
@JsonProperty("formula")
@JsonPropertyDescription("Formula")
private String formula;
/**
* aggregation function for chart
*
*/
@JsonProperty("function")
@JsonPropertyDescription("aggregation function for chart")
private Function function;
/**
* Group of Result
*
*/
@JsonProperty("field")
@JsonPropertyDescription("Group of Result")
private String field;
/**
* Group of Result
*
*/
@JsonProperty("query")
@JsonPropertyDescription("Group of Result")
private String query;
/**
* Formula
*
*/
@JsonProperty("formula")
public String getFormula() {
return formula;
}
/**
* Formula
*
*/
@JsonProperty("formula")
public void setFormula(String formula) {
this.formula = formula;
}
public FormulaHolder withFormula(String formula) {
this.formula = formula;
return this;
}
/**
* aggregation function for chart
*
*/
@JsonProperty("function")
public Function getFunction() {
return function;
}
/**
* aggregation function for chart
*
*/
@JsonProperty("function")
public void setFunction(Function function) {
this.function = function;
}
public FormulaHolder withFunction(Function function) {
this.function = function;
return this;
}
/**
* Group of Result
*
*/
@JsonProperty("field")
public String getField() {
return field;
}
/**
* Group of Result
*
*/
@JsonProperty("field")
public void setField(String field) {
this.field = field;
}
public FormulaHolder withField(String field) {
this.field = field;
return this;
}
/**
* Group of Result
*
*/
@JsonProperty("query")
public String getQuery() {
return query;
}
/**
* Group of Result
*
*/
@JsonProperty("query")
public void setQuery(String query) {
this.query = query;
}
public FormulaHolder withQuery(String query) {
this.query = query;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(FormulaHolder.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("formula");
sb.append('=');
sb.append(((this.formula == null)?"":this.formula));
sb.append(',');
sb.append("function");
sb.append('=');
sb.append(((this.function == null)?"":this.function));
sb.append(',');
sb.append("field");
sb.append('=');
sb.append(((this.field == null)?"":this.field));
sb.append(',');
sb.append("query");
sb.append('=');
sb.append(((this.query == null)?"":this.query));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.formula == null)? 0 :this.formula.hashCode()));
result = ((result* 31)+((this.field == null)? 0 :this.field.hashCode()));
result = ((result* 31)+((this.function == null)? 0 :this.function.hashCode()));
result = ((result* 31)+((this.query == null)? 0 :this.query.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof FormulaHolder) == false) {
return false;
}
FormulaHolder rhs = ((FormulaHolder) other);
return (((((this.formula == rhs.formula)||((this.formula!= null)&&this.formula.equals(rhs.formula)))&&((this.field == rhs.field)||((this.field!= null)&&this.field.equals(rhs.field))))&&((this.function == rhs.function)||((this.function!= null)&&this.function.equals(rhs.function))))&&((this.query == rhs.query)||((this.query!= null)&&this.query.equals(rhs.query))));
}
}