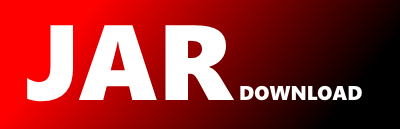
org.openmetadata.schema.dataInsight.custom.LineChart Maven / Gradle / Ivy
package org.openmetadata.schema.dataInsight.custom;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
/**
* LineChart
*
* Line Chart
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"type",
"metrics",
"groupBy",
"includeGroups",
"excludeGroups",
"xAxisLabel",
"yAxisLabel",
"kpiDetails",
"xAxisField"
})
@Generated("jsonschema2pojo")
public class LineChart {
/**
* Type of the data insight chart.
*
*/
@JsonProperty("type")
@JsonPropertyDescription("Type of the data insight chart.")
private LineChart.Type type = LineChart.Type.fromValue("LineChart");
/**
* Metrics for the data insight chart.
*
*/
@JsonProperty("metrics")
@JsonPropertyDescription("Metrics for the data insight chart.")
@Valid
private List metrics = new ArrayList();
/**
* Breakdown field for the data insight chart.
*
*/
@JsonProperty("groupBy")
@JsonPropertyDescription("Breakdown field for the data insight chart.")
private String groupBy;
/**
* List of groups to be included in the data insight chart when groupBy is specified.
*
*/
@JsonProperty("includeGroups")
@JsonPropertyDescription("List of groups to be included in the data insight chart when groupBy is specified.")
@Valid
private List includeGroups = new ArrayList();
/**
* List of groups to be excluded in the data insight chart when groupBy is specified.
*
*/
@JsonProperty("excludeGroups")
@JsonPropertyDescription("List of groups to be excluded in the data insight chart when groupBy is specified.")
@Valid
private List excludeGroups = new ArrayList();
/**
* X-axis label for the data insight chart.
*
*/
@JsonProperty("xAxisLabel")
@JsonPropertyDescription("X-axis label for the data insight chart.")
private String xAxisLabel;
/**
* Y-axis label for the data insight chart.
*
*/
@JsonProperty("yAxisLabel")
@JsonPropertyDescription("Y-axis label for the data insight chart.")
private String yAxisLabel;
/**
* KPI details for the data insight chart.
*
*/
@JsonProperty("kpiDetails")
@JsonPropertyDescription("KPI details for the data insight chart.")
@Valid
private KPIDetails kpiDetails;
/**
* X-axis field for the data insight chart.
*
*/
@JsonProperty("xAxisField")
@JsonPropertyDescription("X-axis field for the data insight chart.")
private String xAxisField = "@timestamp";
/**
* Type of the data insight chart.
*
*/
@JsonProperty("type")
public LineChart.Type getType() {
return type;
}
/**
* Type of the data insight chart.
*
*/
@JsonProperty("type")
public void setType(LineChart.Type type) {
this.type = type;
}
public LineChart withType(LineChart.Type type) {
this.type = type;
return this;
}
/**
* Metrics for the data insight chart.
*
*/
@JsonProperty("metrics")
public List getMetrics() {
return metrics;
}
/**
* Metrics for the data insight chart.
*
*/
@JsonProperty("metrics")
public void setMetrics(List metrics) {
this.metrics = metrics;
}
public LineChart withMetrics(List metrics) {
this.metrics = metrics;
return this;
}
/**
* Breakdown field for the data insight chart.
*
*/
@JsonProperty("groupBy")
public String getGroupBy() {
return groupBy;
}
/**
* Breakdown field for the data insight chart.
*
*/
@JsonProperty("groupBy")
public void setGroupBy(String groupBy) {
this.groupBy = groupBy;
}
public LineChart withGroupBy(String groupBy) {
this.groupBy = groupBy;
return this;
}
/**
* List of groups to be included in the data insight chart when groupBy is specified.
*
*/
@JsonProperty("includeGroups")
public List getIncludeGroups() {
return includeGroups;
}
/**
* List of groups to be included in the data insight chart when groupBy is specified.
*
*/
@JsonProperty("includeGroups")
public void setIncludeGroups(List includeGroups) {
this.includeGroups = includeGroups;
}
public LineChart withIncludeGroups(List includeGroups) {
this.includeGroups = includeGroups;
return this;
}
/**
* List of groups to be excluded in the data insight chart when groupBy is specified.
*
*/
@JsonProperty("excludeGroups")
public List getExcludeGroups() {
return excludeGroups;
}
/**
* List of groups to be excluded in the data insight chart when groupBy is specified.
*
*/
@JsonProperty("excludeGroups")
public void setExcludeGroups(List excludeGroups) {
this.excludeGroups = excludeGroups;
}
public LineChart withExcludeGroups(List excludeGroups) {
this.excludeGroups = excludeGroups;
return this;
}
/**
* X-axis label for the data insight chart.
*
*/
@JsonProperty("xAxisLabel")
public String getxAxisLabel() {
return xAxisLabel;
}
/**
* X-axis label for the data insight chart.
*
*/
@JsonProperty("xAxisLabel")
public void setxAxisLabel(String xAxisLabel) {
this.xAxisLabel = xAxisLabel;
}
public LineChart withxAxisLabel(String xAxisLabel) {
this.xAxisLabel = xAxisLabel;
return this;
}
/**
* Y-axis label for the data insight chart.
*
*/
@JsonProperty("yAxisLabel")
public String getyAxisLabel() {
return yAxisLabel;
}
/**
* Y-axis label for the data insight chart.
*
*/
@JsonProperty("yAxisLabel")
public void setyAxisLabel(String yAxisLabel) {
this.yAxisLabel = yAxisLabel;
}
public LineChart withyAxisLabel(String yAxisLabel) {
this.yAxisLabel = yAxisLabel;
return this;
}
/**
* KPI details for the data insight chart.
*
*/
@JsonProperty("kpiDetails")
public KPIDetails getKpiDetails() {
return kpiDetails;
}
/**
* KPI details for the data insight chart.
*
*/
@JsonProperty("kpiDetails")
public void setKpiDetails(KPIDetails kpiDetails) {
this.kpiDetails = kpiDetails;
}
public LineChart withKpiDetails(KPIDetails kpiDetails) {
this.kpiDetails = kpiDetails;
return this;
}
/**
* X-axis field for the data insight chart.
*
*/
@JsonProperty("xAxisField")
public String getxAxisField() {
return xAxisField;
}
/**
* X-axis field for the data insight chart.
*
*/
@JsonProperty("xAxisField")
public void setxAxisField(String xAxisField) {
this.xAxisField = xAxisField;
}
public LineChart withxAxisField(String xAxisField) {
this.xAxisField = xAxisField;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(LineChart.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("type");
sb.append('=');
sb.append(((this.type == null)?"":this.type));
sb.append(',');
sb.append("metrics");
sb.append('=');
sb.append(((this.metrics == null)?"":this.metrics));
sb.append(',');
sb.append("groupBy");
sb.append('=');
sb.append(((this.groupBy == null)?"":this.groupBy));
sb.append(',');
sb.append("includeGroups");
sb.append('=');
sb.append(((this.includeGroups == null)?"":this.includeGroups));
sb.append(',');
sb.append("excludeGroups");
sb.append('=');
sb.append(((this.excludeGroups == null)?"":this.excludeGroups));
sb.append(',');
sb.append("xAxisLabel");
sb.append('=');
sb.append(((this.xAxisLabel == null)?"":this.xAxisLabel));
sb.append(',');
sb.append("yAxisLabel");
sb.append('=');
sb.append(((this.yAxisLabel == null)?"":this.yAxisLabel));
sb.append(',');
sb.append("kpiDetails");
sb.append('=');
sb.append(((this.kpiDetails == null)?"":this.kpiDetails));
sb.append(',');
sb.append("xAxisField");
sb.append('=');
sb.append(((this.xAxisField == null)?"":this.xAxisField));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.xAxisField == null)? 0 :this.xAxisField.hashCode()));
result = ((result* 31)+((this.yAxisLabel == null)? 0 :this.yAxisLabel.hashCode()));
result = ((result* 31)+((this.kpiDetails == null)? 0 :this.kpiDetails.hashCode()));
result = ((result* 31)+((this.excludeGroups == null)? 0 :this.excludeGroups.hashCode()));
result = ((result* 31)+((this.xAxisLabel == null)? 0 :this.xAxisLabel.hashCode()));
result = ((result* 31)+((this.metrics == null)? 0 :this.metrics.hashCode()));
result = ((result* 31)+((this.groupBy == null)? 0 :this.groupBy.hashCode()));
result = ((result* 31)+((this.type == null)? 0 :this.type.hashCode()));
result = ((result* 31)+((this.includeGroups == null)? 0 :this.includeGroups.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof LineChart) == false) {
return false;
}
LineChart rhs = ((LineChart) other);
return ((((((((((this.xAxisField == rhs.xAxisField)||((this.xAxisField!= null)&&this.xAxisField.equals(rhs.xAxisField)))&&((this.yAxisLabel == rhs.yAxisLabel)||((this.yAxisLabel!= null)&&this.yAxisLabel.equals(rhs.yAxisLabel))))&&((this.kpiDetails == rhs.kpiDetails)||((this.kpiDetails!= null)&&this.kpiDetails.equals(rhs.kpiDetails))))&&((this.excludeGroups == rhs.excludeGroups)||((this.excludeGroups!= null)&&this.excludeGroups.equals(rhs.excludeGroups))))&&((this.xAxisLabel == rhs.xAxisLabel)||((this.xAxisLabel!= null)&&this.xAxisLabel.equals(rhs.xAxisLabel))))&&((this.metrics == rhs.metrics)||((this.metrics!= null)&&this.metrics.equals(rhs.metrics))))&&((this.groupBy == rhs.groupBy)||((this.groupBy!= null)&&this.groupBy.equals(rhs.groupBy))))&&((this.type == rhs.type)||((this.type!= null)&&this.type.equals(rhs.type))))&&((this.includeGroups == rhs.includeGroups)||((this.includeGroups!= null)&&this.includeGroups.equals(rhs.includeGroups))));
}
/**
* Type of the data insight chart.
*
*/
@Generated("jsonschema2pojo")
public enum Type {
LINE_CHART("LineChart");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (LineChart.Type c: values()) {
CONSTANTS.put(c.value, c);
}
}
Type(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static LineChart.Type fromValue(String value) {
LineChart.Type constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}