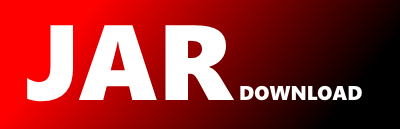
org.openmetadata.schema.entity.events.FailedEventResponse Maven / Gradle / Ivy
package org.openmetadata.schema.entity.events;
import java.util.UUID;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.openmetadata.schema.type.ChangeEvent;
/**
* FailedEvents
*
* Failed Events Schema
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"failingSubscriptionId",
"changeEvent",
"reason",
"source",
"timestamp"
})
@Generated("jsonschema2pojo")
public class FailedEventResponse {
/**
* Unique id used to identify an entity.
*
*/
@JsonProperty("failingSubscriptionId")
@JsonPropertyDescription("Unique id used to identify an entity.")
private UUID failingSubscriptionId;
/**
* ChangeEvent
*
* This schema defines the change event type to capture the changes to entities. Entities change due to user activity, such as updating description of a dataset, changing ownership, or adding new tags. Entity also changes due to activities at the metadata sources, such as a new dataset was created, a datasets was deleted, or schema of a dataset is modified. When state of entity changes, an event is produced. These events can be used to build apps and bots that respond to the change from activities.
*
*/
@JsonProperty("changeEvent")
@JsonPropertyDescription("This schema defines the change event type to capture the changes to entities. Entities change due to user activity, such as updating description of a dataset, changing ownership, or adding new tags. Entity also changes due to activities at the metadata sources, such as a new dataset was created, a datasets was deleted, or schema of a dataset is modified. When state of entity changes, an event is produced. These events can be used to build apps and bots that respond to the change from activities.")
@Valid
private ChangeEvent changeEvent;
/**
* Reason for failure
*
*/
@JsonProperty("reason")
@JsonPropertyDescription("Reason for failure")
private String reason;
/**
* Source of the failed event
*
*/
@JsonProperty("source")
@JsonPropertyDescription("Source of the failed event")
private String source;
/**
* Timestamp in Unix epoch time milliseconds.@om-field-type
*
*/
@JsonProperty("timestamp")
@JsonPropertyDescription("Timestamp in Unix epoch time milliseconds.")
private Long timestamp;
/**
* Unique id used to identify an entity.
*
*/
@JsonProperty("failingSubscriptionId")
public UUID getFailingSubscriptionId() {
return failingSubscriptionId;
}
/**
* Unique id used to identify an entity.
*
*/
@JsonProperty("failingSubscriptionId")
public void setFailingSubscriptionId(UUID failingSubscriptionId) {
this.failingSubscriptionId = failingSubscriptionId;
}
public FailedEventResponse withFailingSubscriptionId(UUID failingSubscriptionId) {
this.failingSubscriptionId = failingSubscriptionId;
return this;
}
/**
* ChangeEvent
*
* This schema defines the change event type to capture the changes to entities. Entities change due to user activity, such as updating description of a dataset, changing ownership, or adding new tags. Entity also changes due to activities at the metadata sources, such as a new dataset was created, a datasets was deleted, or schema of a dataset is modified. When state of entity changes, an event is produced. These events can be used to build apps and bots that respond to the change from activities.
*
*/
@JsonProperty("changeEvent")
public ChangeEvent getChangeEvent() {
return changeEvent;
}
/**
* ChangeEvent
*
* This schema defines the change event type to capture the changes to entities. Entities change due to user activity, such as updating description of a dataset, changing ownership, or adding new tags. Entity also changes due to activities at the metadata sources, such as a new dataset was created, a datasets was deleted, or schema of a dataset is modified. When state of entity changes, an event is produced. These events can be used to build apps and bots that respond to the change from activities.
*
*/
@JsonProperty("changeEvent")
public void setChangeEvent(ChangeEvent changeEvent) {
this.changeEvent = changeEvent;
}
public FailedEventResponse withChangeEvent(ChangeEvent changeEvent) {
this.changeEvent = changeEvent;
return this;
}
/**
* Reason for failure
*
*/
@JsonProperty("reason")
public String getReason() {
return reason;
}
/**
* Reason for failure
*
*/
@JsonProperty("reason")
public void setReason(String reason) {
this.reason = reason;
}
public FailedEventResponse withReason(String reason) {
this.reason = reason;
return this;
}
/**
* Source of the failed event
*
*/
@JsonProperty("source")
public String getSource() {
return source;
}
/**
* Source of the failed event
*
*/
@JsonProperty("source")
public void setSource(String source) {
this.source = source;
}
public FailedEventResponse withSource(String source) {
this.source = source;
return this;
}
/**
* Timestamp in Unix epoch time milliseconds.@om-field-type
*
*/
@JsonProperty("timestamp")
public Long getTimestamp() {
return timestamp;
}
/**
* Timestamp in Unix epoch time milliseconds.@om-field-type
*
*/
@JsonProperty("timestamp")
public void setTimestamp(Long timestamp) {
this.timestamp = timestamp;
}
public FailedEventResponse withTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(FailedEventResponse.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("failingSubscriptionId");
sb.append('=');
sb.append(((this.failingSubscriptionId == null)?"":this.failingSubscriptionId));
sb.append(',');
sb.append("changeEvent");
sb.append('=');
sb.append(((this.changeEvent == null)?"":this.changeEvent));
sb.append(',');
sb.append("reason");
sb.append('=');
sb.append(((this.reason == null)?"":this.reason));
sb.append(',');
sb.append("source");
sb.append('=');
sb.append(((this.source == null)?"":this.source));
sb.append(',');
sb.append("timestamp");
sb.append('=');
sb.append(((this.timestamp == null)?"":this.timestamp));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.reason == null)? 0 :this.reason.hashCode()));
result = ((result* 31)+((this.failingSubscriptionId == null)? 0 :this.failingSubscriptionId.hashCode()));
result = ((result* 31)+((this.source == null)? 0 :this.source.hashCode()));
result = ((result* 31)+((this.changeEvent == null)? 0 :this.changeEvent.hashCode()));
result = ((result* 31)+((this.timestamp == null)? 0 :this.timestamp.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof FailedEventResponse) == false) {
return false;
}
FailedEventResponse rhs = ((FailedEventResponse) other);
return ((((((this.reason == rhs.reason)||((this.reason!= null)&&this.reason.equals(rhs.reason)))&&((this.failingSubscriptionId == rhs.failingSubscriptionId)||((this.failingSubscriptionId!= null)&&this.failingSubscriptionId.equals(rhs.failingSubscriptionId))))&&((this.source == rhs.source)||((this.source!= null)&&this.source.equals(rhs.source))))&&((this.changeEvent == rhs.changeEvent)||((this.changeEvent!= null)&&this.changeEvent.equals(rhs.changeEvent))))&&((this.timestamp == rhs.timestamp)||((this.timestamp!= null)&&this.timestamp.equals(rhs.timestamp))));
}
}