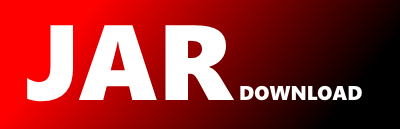
org.openmetadata.schema.metadataIngestion.DatabaseServiceAutoClassificationPipeline Maven / Gradle / Ivy
package org.openmetadata.schema.metadataIngestion;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
/**
* DatabaseServiceAutoClassificationPipeline
*
* DatabaseService AutoClassification & Auto Classification Pipeline Configuration.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"type",
"classificationFilterPattern",
"schemaFilterPattern",
"tableFilterPattern",
"databaseFilterPattern",
"includeViews",
"useFqnForFiltering",
"storeSampleData",
"enableAutoClassification",
"confidence",
"sampleDataCount"
})
@Generated("jsonschema2pojo")
public class DatabaseServiceAutoClassificationPipeline {
/**
* Profiler Source Config Pipeline type
*
*/
@JsonProperty("type")
@JsonPropertyDescription("Profiler Source Config Pipeline type")
private DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType type = DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType.fromValue("AutoClassification");
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("classificationFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern classificationFilterPattern;
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("schemaFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern schemaFilterPattern;
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("tableFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern tableFilterPattern;
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("databaseFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern databaseFilterPattern;
/**
* Include Views
*
* Optional configuration to turn off fetching metadata for views.
*
*/
@JsonProperty("includeViews")
@JsonPropertyDescription("Optional configuration to turn off fetching metadata for views.")
private Boolean includeViews = true;
/**
* Use FQN For Filtering
*
* Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)
*
*/
@JsonProperty("useFqnForFiltering")
@JsonPropertyDescription("Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)")
private Boolean useFqnForFiltering = false;
/**
* Store Sample Data
*
* Option to turn on/off storing sample data. If enabled, we will ingest sample data for each table.
*
*/
@JsonProperty("storeSampleData")
@JsonPropertyDescription("Option to turn on/off storing sample data. If enabled, we will ingest sample data for each table.")
private Boolean storeSampleData = true;
/**
* Enable Auto Classification
*
* Optional configuration to automatically tag columns that might contain sensitive information
*
*/
@JsonProperty("enableAutoClassification")
@JsonPropertyDescription("Optional configuration to automatically tag columns that might contain sensitive information")
private Boolean enableAutoClassification = false;
/**
* Auto Classification Inference Confidence Level
*
* Set the Confidence value for which you want the column to be tagged as PII. Confidence value ranges from 0 to 100. A higher number will yield less false positives but more false negatives. A lower number will yield more false positives but less false negatives.
*
*/
@JsonProperty("confidence")
@JsonPropertyDescription("Set the Confidence value for which you want the column to be tagged as PII. Confidence value ranges from 0 to 100. A higher number will yield less false positives but more false negatives. A lower number will yield more false positives but less false negatives.")
private Double confidence = 80.0D;
/**
* Sample Data Rows Count
*
* Number of sample rows to ingest when 'Generate Sample Data' is enabled
*
*/
@JsonProperty("sampleDataCount")
@JsonPropertyDescription("Number of sample rows to ingest when 'Generate Sample Data' is enabled")
private Integer sampleDataCount = 50;
/**
* Profiler Source Config Pipeline type
*
*/
@JsonProperty("type")
public DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType getType() {
return type;
}
/**
* Profiler Source Config Pipeline type
*
*/
@JsonProperty("type")
public void setType(DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType type) {
this.type = type;
}
public DatabaseServiceAutoClassificationPipeline withType(DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType type) {
this.type = type;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("classificationFilterPattern")
public FilterPattern getClassificationFilterPattern() {
return classificationFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("classificationFilterPattern")
public void setClassificationFilterPattern(FilterPattern classificationFilterPattern) {
this.classificationFilterPattern = classificationFilterPattern;
}
public DatabaseServiceAutoClassificationPipeline withClassificationFilterPattern(FilterPattern classificationFilterPattern) {
this.classificationFilterPattern = classificationFilterPattern;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("schemaFilterPattern")
public FilterPattern getSchemaFilterPattern() {
return schemaFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("schemaFilterPattern")
public void setSchemaFilterPattern(FilterPattern schemaFilterPattern) {
this.schemaFilterPattern = schemaFilterPattern;
}
public DatabaseServiceAutoClassificationPipeline withSchemaFilterPattern(FilterPattern schemaFilterPattern) {
this.schemaFilterPattern = schemaFilterPattern;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("tableFilterPattern")
public FilterPattern getTableFilterPattern() {
return tableFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("tableFilterPattern")
public void setTableFilterPattern(FilterPattern tableFilterPattern) {
this.tableFilterPattern = tableFilterPattern;
}
public DatabaseServiceAutoClassificationPipeline withTableFilterPattern(FilterPattern tableFilterPattern) {
this.tableFilterPattern = tableFilterPattern;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("databaseFilterPattern")
public FilterPattern getDatabaseFilterPattern() {
return databaseFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("databaseFilterPattern")
public void setDatabaseFilterPattern(FilterPattern databaseFilterPattern) {
this.databaseFilterPattern = databaseFilterPattern;
}
public DatabaseServiceAutoClassificationPipeline withDatabaseFilterPattern(FilterPattern databaseFilterPattern) {
this.databaseFilterPattern = databaseFilterPattern;
return this;
}
/**
* Include Views
*
* Optional configuration to turn off fetching metadata for views.
*
*/
@JsonProperty("includeViews")
public Boolean getIncludeViews() {
return includeViews;
}
/**
* Include Views
*
* Optional configuration to turn off fetching metadata for views.
*
*/
@JsonProperty("includeViews")
public void setIncludeViews(Boolean includeViews) {
this.includeViews = includeViews;
}
public DatabaseServiceAutoClassificationPipeline withIncludeViews(Boolean includeViews) {
this.includeViews = includeViews;
return this;
}
/**
* Use FQN For Filtering
*
* Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)
*
*/
@JsonProperty("useFqnForFiltering")
public Boolean getUseFqnForFiltering() {
return useFqnForFiltering;
}
/**
* Use FQN For Filtering
*
* Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)
*
*/
@JsonProperty("useFqnForFiltering")
public void setUseFqnForFiltering(Boolean useFqnForFiltering) {
this.useFqnForFiltering = useFqnForFiltering;
}
public DatabaseServiceAutoClassificationPipeline withUseFqnForFiltering(Boolean useFqnForFiltering) {
this.useFqnForFiltering = useFqnForFiltering;
return this;
}
/**
* Store Sample Data
*
* Option to turn on/off storing sample data. If enabled, we will ingest sample data for each table.
*
*/
@JsonProperty("storeSampleData")
public Boolean getStoreSampleData() {
return storeSampleData;
}
/**
* Store Sample Data
*
* Option to turn on/off storing sample data. If enabled, we will ingest sample data for each table.
*
*/
@JsonProperty("storeSampleData")
public void setStoreSampleData(Boolean storeSampleData) {
this.storeSampleData = storeSampleData;
}
public DatabaseServiceAutoClassificationPipeline withStoreSampleData(Boolean storeSampleData) {
this.storeSampleData = storeSampleData;
return this;
}
/**
* Enable Auto Classification
*
* Optional configuration to automatically tag columns that might contain sensitive information
*
*/
@JsonProperty("enableAutoClassification")
public Boolean getEnableAutoClassification() {
return enableAutoClassification;
}
/**
* Enable Auto Classification
*
* Optional configuration to automatically tag columns that might contain sensitive information
*
*/
@JsonProperty("enableAutoClassification")
public void setEnableAutoClassification(Boolean enableAutoClassification) {
this.enableAutoClassification = enableAutoClassification;
}
public DatabaseServiceAutoClassificationPipeline withEnableAutoClassification(Boolean enableAutoClassification) {
this.enableAutoClassification = enableAutoClassification;
return this;
}
/**
* Auto Classification Inference Confidence Level
*
* Set the Confidence value for which you want the column to be tagged as PII. Confidence value ranges from 0 to 100. A higher number will yield less false positives but more false negatives. A lower number will yield more false positives but less false negatives.
*
*/
@JsonProperty("confidence")
public Double getConfidence() {
return confidence;
}
/**
* Auto Classification Inference Confidence Level
*
* Set the Confidence value for which you want the column to be tagged as PII. Confidence value ranges from 0 to 100. A higher number will yield less false positives but more false negatives. A lower number will yield more false positives but less false negatives.
*
*/
@JsonProperty("confidence")
public void setConfidence(Double confidence) {
this.confidence = confidence;
}
public DatabaseServiceAutoClassificationPipeline withConfidence(Double confidence) {
this.confidence = confidence;
return this;
}
/**
* Sample Data Rows Count
*
* Number of sample rows to ingest when 'Generate Sample Data' is enabled
*
*/
@JsonProperty("sampleDataCount")
public Integer getSampleDataCount() {
return sampleDataCount;
}
/**
* Sample Data Rows Count
*
* Number of sample rows to ingest when 'Generate Sample Data' is enabled
*
*/
@JsonProperty("sampleDataCount")
public void setSampleDataCount(Integer sampleDataCount) {
this.sampleDataCount = sampleDataCount;
}
public DatabaseServiceAutoClassificationPipeline withSampleDataCount(Integer sampleDataCount) {
this.sampleDataCount = sampleDataCount;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(DatabaseServiceAutoClassificationPipeline.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("type");
sb.append('=');
sb.append(((this.type == null)?"":this.type));
sb.append(',');
sb.append("classificationFilterPattern");
sb.append('=');
sb.append(((this.classificationFilterPattern == null)?"":this.classificationFilterPattern));
sb.append(',');
sb.append("schemaFilterPattern");
sb.append('=');
sb.append(((this.schemaFilterPattern == null)?"":this.schemaFilterPattern));
sb.append(',');
sb.append("tableFilterPattern");
sb.append('=');
sb.append(((this.tableFilterPattern == null)?"":this.tableFilterPattern));
sb.append(',');
sb.append("databaseFilterPattern");
sb.append('=');
sb.append(((this.databaseFilterPattern == null)?"":this.databaseFilterPattern));
sb.append(',');
sb.append("includeViews");
sb.append('=');
sb.append(((this.includeViews == null)?"":this.includeViews));
sb.append(',');
sb.append("useFqnForFiltering");
sb.append('=');
sb.append(((this.useFqnForFiltering == null)?"":this.useFqnForFiltering));
sb.append(',');
sb.append("storeSampleData");
sb.append('=');
sb.append(((this.storeSampleData == null)?"":this.storeSampleData));
sb.append(',');
sb.append("enableAutoClassification");
sb.append('=');
sb.append(((this.enableAutoClassification == null)?"":this.enableAutoClassification));
sb.append(',');
sb.append("confidence");
sb.append('=');
sb.append(((this.confidence == null)?"":this.confidence));
sb.append(',');
sb.append("sampleDataCount");
sb.append('=');
sb.append(((this.sampleDataCount == null)?"":this.sampleDataCount));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.tableFilterPattern == null)? 0 :this.tableFilterPattern.hashCode()));
result = ((result* 31)+((this.includeViews == null)? 0 :this.includeViews.hashCode()));
result = ((result* 31)+((this.schemaFilterPattern == null)? 0 :this.schemaFilterPattern.hashCode()));
result = ((result* 31)+((this.sampleDataCount == null)? 0 :this.sampleDataCount.hashCode()));
result = ((result* 31)+((this.enableAutoClassification == null)? 0 :this.enableAutoClassification.hashCode()));
result = ((result* 31)+((this.confidence == null)? 0 :this.confidence.hashCode()));
result = ((result* 31)+((this.databaseFilterPattern == null)? 0 :this.databaseFilterPattern.hashCode()));
result = ((result* 31)+((this.useFqnForFiltering == null)? 0 :this.useFqnForFiltering.hashCode()));
result = ((result* 31)+((this.type == null)? 0 :this.type.hashCode()));
result = ((result* 31)+((this.classificationFilterPattern == null)? 0 :this.classificationFilterPattern.hashCode()));
result = ((result* 31)+((this.storeSampleData == null)? 0 :this.storeSampleData.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof DatabaseServiceAutoClassificationPipeline) == false) {
return false;
}
DatabaseServiceAutoClassificationPipeline rhs = ((DatabaseServiceAutoClassificationPipeline) other);
return ((((((((((((this.tableFilterPattern == rhs.tableFilterPattern)||((this.tableFilterPattern!= null)&&this.tableFilterPattern.equals(rhs.tableFilterPattern)))&&((this.includeViews == rhs.includeViews)||((this.includeViews!= null)&&this.includeViews.equals(rhs.includeViews))))&&((this.schemaFilterPattern == rhs.schemaFilterPattern)||((this.schemaFilterPattern!= null)&&this.schemaFilterPattern.equals(rhs.schemaFilterPattern))))&&((this.sampleDataCount == rhs.sampleDataCount)||((this.sampleDataCount!= null)&&this.sampleDataCount.equals(rhs.sampleDataCount))))&&((this.enableAutoClassification == rhs.enableAutoClassification)||((this.enableAutoClassification!= null)&&this.enableAutoClassification.equals(rhs.enableAutoClassification))))&&((this.confidence == rhs.confidence)||((this.confidence!= null)&&this.confidence.equals(rhs.confidence))))&&((this.databaseFilterPattern == rhs.databaseFilterPattern)||((this.databaseFilterPattern!= null)&&this.databaseFilterPattern.equals(rhs.databaseFilterPattern))))&&((this.useFqnForFiltering == rhs.useFqnForFiltering)||((this.useFqnForFiltering!= null)&&this.useFqnForFiltering.equals(rhs.useFqnForFiltering))))&&((this.type == rhs.type)||((this.type!= null)&&this.type.equals(rhs.type))))&&((this.classificationFilterPattern == rhs.classificationFilterPattern)||((this.classificationFilterPattern!= null)&&this.classificationFilterPattern.equals(rhs.classificationFilterPattern))))&&((this.storeSampleData == rhs.storeSampleData)||((this.storeSampleData!= null)&&this.storeSampleData.equals(rhs.storeSampleData))));
}
/**
* Profiler Source Config Pipeline type
*
*/
@Generated("jsonschema2pojo")
public enum AutoClassificationConfigType {
AUTO_CLASSIFICATION("AutoClassification");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType c: values()) {
CONSTANTS.put(c.value, c);
}
}
AutoClassificationConfigType(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType fromValue(String value) {
DatabaseServiceAutoClassificationPipeline.AutoClassificationConfigType constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}