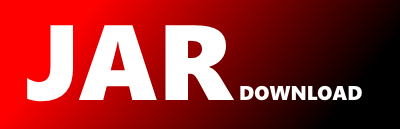
org.openmetadata.schema.metadataIngestion.DatabaseServiceProfilerPipeline Maven / Gradle / Ivy
package org.openmetadata.schema.metadataIngestion;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
/**
* DatabaseServiceProfilerPipeline
*
* DatabaseService Profiler Pipeline Configuration.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"type",
"classificationFilterPattern",
"schemaFilterPattern",
"tableFilterPattern",
"databaseFilterPattern",
"includeViews",
"useFqnForFiltering",
"computeMetrics",
"computeTableMetrics",
"computeColumnMetrics",
"useStatistics",
"profileSampleType",
"profileSample",
"samplingMethodType",
"threadCount",
"timeoutSeconds"
})
@Generated("jsonschema2pojo")
public class DatabaseServiceProfilerPipeline {
/**
* Profiler Source Config Pipeline type
*
*/
@JsonProperty("type")
@JsonPropertyDescription("Profiler Source Config Pipeline type")
private DatabaseServiceProfilerPipeline.ProfilerConfigType type = DatabaseServiceProfilerPipeline.ProfilerConfigType.fromValue("Profiler");
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("classificationFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern classificationFilterPattern;
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("schemaFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern schemaFilterPattern;
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("tableFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern tableFilterPattern;
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("databaseFilterPattern")
@JsonPropertyDescription("Regex to only fetch dashboards or charts that matches the pattern.")
@Valid
private FilterPattern databaseFilterPattern;
/**
* Include Views
*
* Optional configuration to turn off fetching metadata for views.
*
*/
@JsonProperty("includeViews")
@JsonPropertyDescription("Optional configuration to turn off fetching metadata for views.")
private Boolean includeViews = false;
/**
* Use FQN For Filtering
*
* Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)
*
*/
@JsonProperty("useFqnForFiltering")
@JsonPropertyDescription("Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)")
private Boolean useFqnForFiltering = false;
/**
* Compute Metrics
*
* Option to turn on/off computing profiler metrics.
*
*/
@JsonProperty("computeMetrics")
@JsonPropertyDescription("Option to turn on/off computing profiler metrics.")
private Boolean computeMetrics = true;
/**
* Compute Table Metrics
*
* Option to turn on/off table metric computation. If enabled, profiler will compute table level metrics.
*
*/
@JsonProperty("computeTableMetrics")
@JsonPropertyDescription("Option to turn on/off table metric computation. If enabled, profiler will compute table level metrics.")
private Boolean computeTableMetrics = true;
/**
* Compute Column Metrics
*
* Option to turn on/off column metric computation. If enabled, profiler will compute column level metrics.
*
*/
@JsonProperty("computeColumnMetrics")
@JsonPropertyDescription("Option to turn on/off column metric computation. If enabled, profiler will compute column level metrics.")
private Boolean computeColumnMetrics = true;
/**
* Use Gathered Statistics
*
* Use system tables to extract metrics. Metrics that cannot be gathered from system tables will use the default methods. Using system tables can be faster but requires gathering statistics before running (for example using the ANALYZE procedure). More information can be found in the documentation: https://docs.openmetadata.org/latest/profler
*
*/
@JsonProperty("useStatistics")
@JsonPropertyDescription("Use system tables to extract metrics. Metrics that cannot be gathered from system tables will use the default methods. Using system tables can be faster but requires gathering statistics before running (for example using the ANALYZE procedure). More information can be found in the documentation: https://docs.openmetadata.org/latest/profler")
private Boolean useStatistics = false;
/**
* Type of Profile Sample (percentage or rows)
*
*/
@JsonProperty("profileSampleType")
@JsonPropertyDescription("Type of Profile Sample (percentage or rows)")
private org.openmetadata.schema.type.TableProfilerConfig.ProfileSampleType profileSampleType = org.openmetadata.schema.type.TableProfilerConfig.ProfileSampleType.fromValue("PERCENTAGE");
/**
* Profile Sample
*
* Percentage of data or no. of rows used to compute the profiler metrics and run data quality tests
*
*/
@JsonProperty("profileSample")
@JsonPropertyDescription("Percentage of data or no. of rows used to compute the profiler metrics and run data quality tests")
private Double profileSample = null;
/**
* Type of Sampling Method (BERNOULLI or SYSTEM)
*
*/
@JsonProperty("samplingMethodType")
@JsonPropertyDescription("Type of Sampling Method (BERNOULLI or SYSTEM)")
private org.openmetadata.schema.type.TableProfilerConfig.SamplingMethodType samplingMethodType;
/**
* Thread Count
*
* Number of threads to use during metric computations
*
*/
@JsonProperty("threadCount")
@JsonPropertyDescription("Number of threads to use during metric computations")
private Double threadCount = 5.0D;
/**
* Timeout (in sec.)
*
* Profiler Timeout in Seconds
*
*/
@JsonProperty("timeoutSeconds")
@JsonPropertyDescription("Profiler Timeout in Seconds")
private Integer timeoutSeconds = 43200;
/**
* Profiler Source Config Pipeline type
*
*/
@JsonProperty("type")
public DatabaseServiceProfilerPipeline.ProfilerConfigType getType() {
return type;
}
/**
* Profiler Source Config Pipeline type
*
*/
@JsonProperty("type")
public void setType(DatabaseServiceProfilerPipeline.ProfilerConfigType type) {
this.type = type;
}
public DatabaseServiceProfilerPipeline withType(DatabaseServiceProfilerPipeline.ProfilerConfigType type) {
this.type = type;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("classificationFilterPattern")
public FilterPattern getClassificationFilterPattern() {
return classificationFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("classificationFilterPattern")
public void setClassificationFilterPattern(FilterPattern classificationFilterPattern) {
this.classificationFilterPattern = classificationFilterPattern;
}
public DatabaseServiceProfilerPipeline withClassificationFilterPattern(FilterPattern classificationFilterPattern) {
this.classificationFilterPattern = classificationFilterPattern;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("schemaFilterPattern")
public FilterPattern getSchemaFilterPattern() {
return schemaFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("schemaFilterPattern")
public void setSchemaFilterPattern(FilterPattern schemaFilterPattern) {
this.schemaFilterPattern = schemaFilterPattern;
}
public DatabaseServiceProfilerPipeline withSchemaFilterPattern(FilterPattern schemaFilterPattern) {
this.schemaFilterPattern = schemaFilterPattern;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("tableFilterPattern")
public FilterPattern getTableFilterPattern() {
return tableFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("tableFilterPattern")
public void setTableFilterPattern(FilterPattern tableFilterPattern) {
this.tableFilterPattern = tableFilterPattern;
}
public DatabaseServiceProfilerPipeline withTableFilterPattern(FilterPattern tableFilterPattern) {
this.tableFilterPattern = tableFilterPattern;
return this;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("databaseFilterPattern")
public FilterPattern getDatabaseFilterPattern() {
return databaseFilterPattern;
}
/**
* Regex to only fetch dashboards or charts that matches the pattern.
*
*/
@JsonProperty("databaseFilterPattern")
public void setDatabaseFilterPattern(FilterPattern databaseFilterPattern) {
this.databaseFilterPattern = databaseFilterPattern;
}
public DatabaseServiceProfilerPipeline withDatabaseFilterPattern(FilterPattern databaseFilterPattern) {
this.databaseFilterPattern = databaseFilterPattern;
return this;
}
/**
* Include Views
*
* Optional configuration to turn off fetching metadata for views.
*
*/
@JsonProperty("includeViews")
public Boolean getIncludeViews() {
return includeViews;
}
/**
* Include Views
*
* Optional configuration to turn off fetching metadata for views.
*
*/
@JsonProperty("includeViews")
public void setIncludeViews(Boolean includeViews) {
this.includeViews = includeViews;
}
public DatabaseServiceProfilerPipeline withIncludeViews(Boolean includeViews) {
this.includeViews = includeViews;
return this;
}
/**
* Use FQN For Filtering
*
* Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)
*
*/
@JsonProperty("useFqnForFiltering")
public Boolean getUseFqnForFiltering() {
return useFqnForFiltering;
}
/**
* Use FQN For Filtering
*
* Regex will be applied on fully qualified name (e.g service_name.db_name.schema_name.table_name) instead of raw name (e.g. table_name)
*
*/
@JsonProperty("useFqnForFiltering")
public void setUseFqnForFiltering(Boolean useFqnForFiltering) {
this.useFqnForFiltering = useFqnForFiltering;
}
public DatabaseServiceProfilerPipeline withUseFqnForFiltering(Boolean useFqnForFiltering) {
this.useFqnForFiltering = useFqnForFiltering;
return this;
}
/**
* Compute Metrics
*
* Option to turn on/off computing profiler metrics.
*
*/
@JsonProperty("computeMetrics")
public Boolean getComputeMetrics() {
return computeMetrics;
}
/**
* Compute Metrics
*
* Option to turn on/off computing profiler metrics.
*
*/
@JsonProperty("computeMetrics")
public void setComputeMetrics(Boolean computeMetrics) {
this.computeMetrics = computeMetrics;
}
public DatabaseServiceProfilerPipeline withComputeMetrics(Boolean computeMetrics) {
this.computeMetrics = computeMetrics;
return this;
}
/**
* Compute Table Metrics
*
* Option to turn on/off table metric computation. If enabled, profiler will compute table level metrics.
*
*/
@JsonProperty("computeTableMetrics")
public Boolean getComputeTableMetrics() {
return computeTableMetrics;
}
/**
* Compute Table Metrics
*
* Option to turn on/off table metric computation. If enabled, profiler will compute table level metrics.
*
*/
@JsonProperty("computeTableMetrics")
public void setComputeTableMetrics(Boolean computeTableMetrics) {
this.computeTableMetrics = computeTableMetrics;
}
public DatabaseServiceProfilerPipeline withComputeTableMetrics(Boolean computeTableMetrics) {
this.computeTableMetrics = computeTableMetrics;
return this;
}
/**
* Compute Column Metrics
*
* Option to turn on/off column metric computation. If enabled, profiler will compute column level metrics.
*
*/
@JsonProperty("computeColumnMetrics")
public Boolean getComputeColumnMetrics() {
return computeColumnMetrics;
}
/**
* Compute Column Metrics
*
* Option to turn on/off column metric computation. If enabled, profiler will compute column level metrics.
*
*/
@JsonProperty("computeColumnMetrics")
public void setComputeColumnMetrics(Boolean computeColumnMetrics) {
this.computeColumnMetrics = computeColumnMetrics;
}
public DatabaseServiceProfilerPipeline withComputeColumnMetrics(Boolean computeColumnMetrics) {
this.computeColumnMetrics = computeColumnMetrics;
return this;
}
/**
* Use Gathered Statistics
*
* Use system tables to extract metrics. Metrics that cannot be gathered from system tables will use the default methods. Using system tables can be faster but requires gathering statistics before running (for example using the ANALYZE procedure). More information can be found in the documentation: https://docs.openmetadata.org/latest/profler
*
*/
@JsonProperty("useStatistics")
public Boolean getUseStatistics() {
return useStatistics;
}
/**
* Use Gathered Statistics
*
* Use system tables to extract metrics. Metrics that cannot be gathered from system tables will use the default methods. Using system tables can be faster but requires gathering statistics before running (for example using the ANALYZE procedure). More information can be found in the documentation: https://docs.openmetadata.org/latest/profler
*
*/
@JsonProperty("useStatistics")
public void setUseStatistics(Boolean useStatistics) {
this.useStatistics = useStatistics;
}
public DatabaseServiceProfilerPipeline withUseStatistics(Boolean useStatistics) {
this.useStatistics = useStatistics;
return this;
}
/**
* Type of Profile Sample (percentage or rows)
*
*/
@JsonProperty("profileSampleType")
public org.openmetadata.schema.type.TableProfilerConfig.ProfileSampleType getProfileSampleType() {
return profileSampleType;
}
/**
* Type of Profile Sample (percentage or rows)
*
*/
@JsonProperty("profileSampleType")
public void setProfileSampleType(org.openmetadata.schema.type.TableProfilerConfig.ProfileSampleType profileSampleType) {
this.profileSampleType = profileSampleType;
}
public DatabaseServiceProfilerPipeline withProfileSampleType(org.openmetadata.schema.type.TableProfilerConfig.ProfileSampleType profileSampleType) {
this.profileSampleType = profileSampleType;
return this;
}
/**
* Profile Sample
*
* Percentage of data or no. of rows used to compute the profiler metrics and run data quality tests
*
*/
@JsonProperty("profileSample")
public Double getProfileSample() {
return profileSample;
}
/**
* Profile Sample
*
* Percentage of data or no. of rows used to compute the profiler metrics and run data quality tests
*
*/
@JsonProperty("profileSample")
public void setProfileSample(Double profileSample) {
this.profileSample = profileSample;
}
public DatabaseServiceProfilerPipeline withProfileSample(Double profileSample) {
this.profileSample = profileSample;
return this;
}
/**
* Type of Sampling Method (BERNOULLI or SYSTEM)
*
*/
@JsonProperty("samplingMethodType")
public org.openmetadata.schema.type.TableProfilerConfig.SamplingMethodType getSamplingMethodType() {
return samplingMethodType;
}
/**
* Type of Sampling Method (BERNOULLI or SYSTEM)
*
*/
@JsonProperty("samplingMethodType")
public void setSamplingMethodType(org.openmetadata.schema.type.TableProfilerConfig.SamplingMethodType samplingMethodType) {
this.samplingMethodType = samplingMethodType;
}
public DatabaseServiceProfilerPipeline withSamplingMethodType(org.openmetadata.schema.type.TableProfilerConfig.SamplingMethodType samplingMethodType) {
this.samplingMethodType = samplingMethodType;
return this;
}
/**
* Thread Count
*
* Number of threads to use during metric computations
*
*/
@JsonProperty("threadCount")
public Double getThreadCount() {
return threadCount;
}
/**
* Thread Count
*
* Number of threads to use during metric computations
*
*/
@JsonProperty("threadCount")
public void setThreadCount(Double threadCount) {
this.threadCount = threadCount;
}
public DatabaseServiceProfilerPipeline withThreadCount(Double threadCount) {
this.threadCount = threadCount;
return this;
}
/**
* Timeout (in sec.)
*
* Profiler Timeout in Seconds
*
*/
@JsonProperty("timeoutSeconds")
public Integer getTimeoutSeconds() {
return timeoutSeconds;
}
/**
* Timeout (in sec.)
*
* Profiler Timeout in Seconds
*
*/
@JsonProperty("timeoutSeconds")
public void setTimeoutSeconds(Integer timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
}
public DatabaseServiceProfilerPipeline withTimeoutSeconds(Integer timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(DatabaseServiceProfilerPipeline.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("type");
sb.append('=');
sb.append(((this.type == null)?"":this.type));
sb.append(',');
sb.append("classificationFilterPattern");
sb.append('=');
sb.append(((this.classificationFilterPattern == null)?"":this.classificationFilterPattern));
sb.append(',');
sb.append("schemaFilterPattern");
sb.append('=');
sb.append(((this.schemaFilterPattern == null)?"":this.schemaFilterPattern));
sb.append(',');
sb.append("tableFilterPattern");
sb.append('=');
sb.append(((this.tableFilterPattern == null)?"":this.tableFilterPattern));
sb.append(',');
sb.append("databaseFilterPattern");
sb.append('=');
sb.append(((this.databaseFilterPattern == null)?"":this.databaseFilterPattern));
sb.append(',');
sb.append("includeViews");
sb.append('=');
sb.append(((this.includeViews == null)?"":this.includeViews));
sb.append(',');
sb.append("useFqnForFiltering");
sb.append('=');
sb.append(((this.useFqnForFiltering == null)?"":this.useFqnForFiltering));
sb.append(',');
sb.append("computeMetrics");
sb.append('=');
sb.append(((this.computeMetrics == null)?"":this.computeMetrics));
sb.append(',');
sb.append("computeTableMetrics");
sb.append('=');
sb.append(((this.computeTableMetrics == null)?"":this.computeTableMetrics));
sb.append(',');
sb.append("computeColumnMetrics");
sb.append('=');
sb.append(((this.computeColumnMetrics == null)?"":this.computeColumnMetrics));
sb.append(',');
sb.append("useStatistics");
sb.append('=');
sb.append(((this.useStatistics == null)?"":this.useStatistics));
sb.append(',');
sb.append("profileSampleType");
sb.append('=');
sb.append(((this.profileSampleType == null)?"":this.profileSampleType));
sb.append(',');
sb.append("profileSample");
sb.append('=');
sb.append(((this.profileSample == null)?"":this.profileSample));
sb.append(',');
sb.append("samplingMethodType");
sb.append('=');
sb.append(((this.samplingMethodType == null)?"":this.samplingMethodType));
sb.append(',');
sb.append("threadCount");
sb.append('=');
sb.append(((this.threadCount == null)?"":this.threadCount));
sb.append(',');
sb.append("timeoutSeconds");
sb.append('=');
sb.append(((this.timeoutSeconds == null)?"":this.timeoutSeconds));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.tableFilterPattern == null)? 0 :this.tableFilterPattern.hashCode()));
result = ((result* 31)+((this.includeViews == null)? 0 :this.includeViews.hashCode()));
result = ((result* 31)+((this.samplingMethodType == null)? 0 :this.samplingMethodType.hashCode()));
result = ((result* 31)+((this.profileSampleType == null)? 0 :this.profileSampleType.hashCode()));
result = ((result* 31)+((this.databaseFilterPattern == null)? 0 :this.databaseFilterPattern.hashCode()));
result = ((result* 31)+((this.useFqnForFiltering == null)? 0 :this.useFqnForFiltering.hashCode()));
result = ((result* 31)+((this.threadCount == null)? 0 :this.threadCount.hashCode()));
result = ((result* 31)+((this.type == null)? 0 :this.type.hashCode()));
result = ((result* 31)+((this.computeMetrics == null)? 0 :this.computeMetrics.hashCode()));
result = ((result* 31)+((this.useStatistics == null)? 0 :this.useStatistics.hashCode()));
result = ((result* 31)+((this.schemaFilterPattern == null)? 0 :this.schemaFilterPattern.hashCode()));
result = ((result* 31)+((this.computeTableMetrics == null)? 0 :this.computeTableMetrics.hashCode()));
result = ((result* 31)+((this.computeColumnMetrics == null)? 0 :this.computeColumnMetrics.hashCode()));
result = ((result* 31)+((this.timeoutSeconds == null)? 0 :this.timeoutSeconds.hashCode()));
result = ((result* 31)+((this.classificationFilterPattern == null)? 0 :this.classificationFilterPattern.hashCode()));
result = ((result* 31)+((this.profileSample == null)? 0 :this.profileSample.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof DatabaseServiceProfilerPipeline) == false) {
return false;
}
DatabaseServiceProfilerPipeline rhs = ((DatabaseServiceProfilerPipeline) other);
return (((((((((((((((((this.tableFilterPattern == rhs.tableFilterPattern)||((this.tableFilterPattern!= null)&&this.tableFilterPattern.equals(rhs.tableFilterPattern)))&&((this.includeViews == rhs.includeViews)||((this.includeViews!= null)&&this.includeViews.equals(rhs.includeViews))))&&((this.samplingMethodType == rhs.samplingMethodType)||((this.samplingMethodType!= null)&&this.samplingMethodType.equals(rhs.samplingMethodType))))&&((this.profileSampleType == rhs.profileSampleType)||((this.profileSampleType!= null)&&this.profileSampleType.equals(rhs.profileSampleType))))&&((this.databaseFilterPattern == rhs.databaseFilterPattern)||((this.databaseFilterPattern!= null)&&this.databaseFilterPattern.equals(rhs.databaseFilterPattern))))&&((this.useFqnForFiltering == rhs.useFqnForFiltering)||((this.useFqnForFiltering!= null)&&this.useFqnForFiltering.equals(rhs.useFqnForFiltering))))&&((this.threadCount == rhs.threadCount)||((this.threadCount!= null)&&this.threadCount.equals(rhs.threadCount))))&&((this.type == rhs.type)||((this.type!= null)&&this.type.equals(rhs.type))))&&((this.computeMetrics == rhs.computeMetrics)||((this.computeMetrics!= null)&&this.computeMetrics.equals(rhs.computeMetrics))))&&((this.useStatistics == rhs.useStatistics)||((this.useStatistics!= null)&&this.useStatistics.equals(rhs.useStatistics))))&&((this.schemaFilterPattern == rhs.schemaFilterPattern)||((this.schemaFilterPattern!= null)&&this.schemaFilterPattern.equals(rhs.schemaFilterPattern))))&&((this.computeTableMetrics == rhs.computeTableMetrics)||((this.computeTableMetrics!= null)&&this.computeTableMetrics.equals(rhs.computeTableMetrics))))&&((this.computeColumnMetrics == rhs.computeColumnMetrics)||((this.computeColumnMetrics!= null)&&this.computeColumnMetrics.equals(rhs.computeColumnMetrics))))&&((this.timeoutSeconds == rhs.timeoutSeconds)||((this.timeoutSeconds!= null)&&this.timeoutSeconds.equals(rhs.timeoutSeconds))))&&((this.classificationFilterPattern == rhs.classificationFilterPattern)||((this.classificationFilterPattern!= null)&&this.classificationFilterPattern.equals(rhs.classificationFilterPattern))))&&((this.profileSample == rhs.profileSample)||((this.profileSample!= null)&&this.profileSample.equals(rhs.profileSample))));
}
/**
* Profiler Source Config Pipeline type
*
*/
@Generated("jsonschema2pojo")
public enum ProfilerConfigType {
PROFILER("Profiler");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (DatabaseServiceProfilerPipeline.ProfilerConfigType c: values()) {
CONSTANTS.put(c.value, c);
}
}
ProfilerConfigType(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static DatabaseServiceProfilerPipeline.ProfilerConfigType fromValue(String value) {
DatabaseServiceProfilerPipeline.ProfilerConfigType constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}