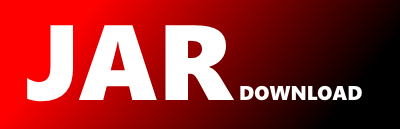
org.openmetadata.schema.system.EventPublisherJob Maven / Gradle / Ivy
package org.openmetadata.schema.system;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import org.openmetadata.schema.type.IndexMappingLanguage;
/**
* EventPublisherResult
*
* This schema defines Event Publisher Job.
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"name",
"timestamp",
"status",
"failure",
"stats",
"entities",
"recreateIndex",
"batchSize",
"payLoadSize",
"producerThreads",
"consumerThreads",
"queueSize",
"maxConcurrentRequests",
"maxRetries",
"initialBackoff",
"maxBackoff",
"searchIndexMappingLanguage",
"afterCursor"
})
@Generated("jsonschema2pojo")
public class EventPublisherJob {
/**
* Name of the result
*
*/
@JsonProperty("name")
@JsonPropertyDescription("Name of the result")
private String name;
/**
* Timestamp in Unix epoch time milliseconds.@om-field-type
* (Required)
*
*/
@JsonProperty("timestamp")
@JsonPropertyDescription("Timestamp in Unix epoch time milliseconds.")
@NotNull
private Long timestamp;
/**
* This schema publisher run job status.
* (Required)
*
*/
@JsonProperty("status")
@JsonPropertyDescription("This schema publisher run job status.")
@NotNull
private EventPublisherJob.Status status;
/**
* IndexingAppError
*
* This schema defines Event Publisher Job Error Schema.
*
*/
@JsonProperty("failure")
@JsonPropertyDescription("This schema defines Event Publisher Job Error Schema.")
@Valid
private IndexingError failure;
@JsonProperty("stats")
@Valid
private Stats stats;
/**
* List of Entities to Reindex
*
*/
@JsonProperty("entities")
@JsonDeserialize(as = java.util.LinkedHashSet.class)
@JsonPropertyDescription("List of Entities to Reindex")
@Valid
private Set entities = new LinkedHashSet();
/**
* This schema publisher run modes.
*
*/
@JsonProperty("recreateIndex")
@JsonPropertyDescription("This schema publisher run modes.")
private Boolean recreateIndex;
/**
* Maximum number of events sent in a batch (Default 10).
*
*/
@JsonProperty("batchSize")
@JsonPropertyDescription("Maximum number of events sent in a batch (Default 10).")
private Integer batchSize = 100;
/**
* Payload size in bytes depending on config.
*
*/
@JsonProperty("payLoadSize")
@JsonPropertyDescription("Payload size in bytes depending on config.")
private Long payLoadSize = 104857600L;
/**
* Number of Producer Threads to use
*
* Number of producer threads to use for reindexing
*
*/
@JsonProperty("producerThreads")
@JsonPropertyDescription("Number of producer threads to use for reindexing")
private Integer producerThreads = 1;
/**
* Number of Consumer Threads to use
*
* Number of consumer threads to use for reindexing
*
*/
@JsonProperty("consumerThreads")
@JsonPropertyDescription("Number of consumer threads to use for reindexing")
private Integer consumerThreads = 1;
/**
* Queue Size to use.
*
* Queue Size to use internally for reindexing.
*
*/
@JsonProperty("queueSize")
@JsonPropertyDescription("Queue Size to use internally for reindexing.")
private Integer queueSize = 100;
/**
* Max Concurrent Requests
*
* Maximum number of concurrent requests to the search index
*
*/
@JsonProperty("maxConcurrentRequests")
@JsonPropertyDescription("Maximum number of concurrent requests to the search index")
private Integer maxConcurrentRequests = 100;
/**
* Max Retries
*
* Maximum number of retries for a failed request
*
*/
@JsonProperty("maxRetries")
@JsonPropertyDescription("Maximum number of retries for a failed request")
private Integer maxRetries = 5;
/**
* Initial Backoff Millis
*
* Initial backoff time in milliseconds
*
*/
@JsonProperty("initialBackoff")
@JsonPropertyDescription("Initial backoff time in milliseconds")
private Integer initialBackoff = 1000;
/**
* Max Backoff Millis
*
* Maximum backoff time in milliseconds
*
*/
@JsonProperty("maxBackoff")
@JsonPropertyDescription("Maximum backoff time in milliseconds")
private Integer maxBackoff = 10000;
/**
* This schema defines the language options available for search index mappings.
*
*/
@JsonProperty("searchIndexMappingLanguage")
@JsonPropertyDescription("This schema defines the language options available for search index mappings.")
private IndexMappingLanguage searchIndexMappingLanguage = IndexMappingLanguage.fromValue("EN");
/**
* Provide After in case of failure to start reindexing after the issue is solved
*
*/
@JsonProperty("afterCursor")
@JsonPropertyDescription("Provide After in case of failure to start reindexing after the issue is solved")
private String afterCursor;
/**
* Name of the result
*
*/
@JsonProperty("name")
public String getName() {
return name;
}
/**
* Name of the result
*
*/
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
public EventPublisherJob withName(String name) {
this.name = name;
return this;
}
/**
* Timestamp in Unix epoch time milliseconds.@om-field-type
* (Required)
*
*/
@JsonProperty("timestamp")
public Long getTimestamp() {
return timestamp;
}
/**
* Timestamp in Unix epoch time milliseconds.@om-field-type
* (Required)
*
*/
@JsonProperty("timestamp")
public void setTimestamp(Long timestamp) {
this.timestamp = timestamp;
}
public EventPublisherJob withTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* This schema publisher run job status.
* (Required)
*
*/
@JsonProperty("status")
public EventPublisherJob.Status getStatus() {
return status;
}
/**
* This schema publisher run job status.
* (Required)
*
*/
@JsonProperty("status")
public void setStatus(EventPublisherJob.Status status) {
this.status = status;
}
public EventPublisherJob withStatus(EventPublisherJob.Status status) {
this.status = status;
return this;
}
/**
* IndexingAppError
*
* This schema defines Event Publisher Job Error Schema.
*
*/
@JsonProperty("failure")
public IndexingError getFailure() {
return failure;
}
/**
* IndexingAppError
*
* This schema defines Event Publisher Job Error Schema.
*
*/
@JsonProperty("failure")
public void setFailure(IndexingError failure) {
this.failure = failure;
}
public EventPublisherJob withFailure(IndexingError failure) {
this.failure = failure;
return this;
}
@JsonProperty("stats")
public Stats getStats() {
return stats;
}
@JsonProperty("stats")
public void setStats(Stats stats) {
this.stats = stats;
}
public EventPublisherJob withStats(Stats stats) {
this.stats = stats;
return this;
}
/**
* List of Entities to Reindex
*
*/
@JsonProperty("entities")
public Set getEntities() {
return entities;
}
/**
* List of Entities to Reindex
*
*/
@JsonProperty("entities")
public void setEntities(Set entities) {
this.entities = entities;
}
public EventPublisherJob withEntities(Set entities) {
this.entities = entities;
return this;
}
/**
* This schema publisher run modes.
*
*/
@JsonProperty("recreateIndex")
public Boolean getRecreateIndex() {
return recreateIndex;
}
/**
* This schema publisher run modes.
*
*/
@JsonProperty("recreateIndex")
public void setRecreateIndex(Boolean recreateIndex) {
this.recreateIndex = recreateIndex;
}
public EventPublisherJob withRecreateIndex(Boolean recreateIndex) {
this.recreateIndex = recreateIndex;
return this;
}
/**
* Maximum number of events sent in a batch (Default 10).
*
*/
@JsonProperty("batchSize")
public Integer getBatchSize() {
return batchSize;
}
/**
* Maximum number of events sent in a batch (Default 10).
*
*/
@JsonProperty("batchSize")
public void setBatchSize(Integer batchSize) {
this.batchSize = batchSize;
}
public EventPublisherJob withBatchSize(Integer batchSize) {
this.batchSize = batchSize;
return this;
}
/**
* Payload size in bytes depending on config.
*
*/
@JsonProperty("payLoadSize")
public Long getPayLoadSize() {
return payLoadSize;
}
/**
* Payload size in bytes depending on config.
*
*/
@JsonProperty("payLoadSize")
public void setPayLoadSize(Long payLoadSize) {
this.payLoadSize = payLoadSize;
}
public EventPublisherJob withPayLoadSize(Long payLoadSize) {
this.payLoadSize = payLoadSize;
return this;
}
/**
* Number of Producer Threads to use
*
* Number of producer threads to use for reindexing
*
*/
@JsonProperty("producerThreads")
public Integer getProducerThreads() {
return producerThreads;
}
/**
* Number of Producer Threads to use
*
* Number of producer threads to use for reindexing
*
*/
@JsonProperty("producerThreads")
public void setProducerThreads(Integer producerThreads) {
this.producerThreads = producerThreads;
}
public EventPublisherJob withProducerThreads(Integer producerThreads) {
this.producerThreads = producerThreads;
return this;
}
/**
* Number of Consumer Threads to use
*
* Number of consumer threads to use for reindexing
*
*/
@JsonProperty("consumerThreads")
public Integer getConsumerThreads() {
return consumerThreads;
}
/**
* Number of Consumer Threads to use
*
* Number of consumer threads to use for reindexing
*
*/
@JsonProperty("consumerThreads")
public void setConsumerThreads(Integer consumerThreads) {
this.consumerThreads = consumerThreads;
}
public EventPublisherJob withConsumerThreads(Integer consumerThreads) {
this.consumerThreads = consumerThreads;
return this;
}
/**
* Queue Size to use.
*
* Queue Size to use internally for reindexing.
*
*/
@JsonProperty("queueSize")
public Integer getQueueSize() {
return queueSize;
}
/**
* Queue Size to use.
*
* Queue Size to use internally for reindexing.
*
*/
@JsonProperty("queueSize")
public void setQueueSize(Integer queueSize) {
this.queueSize = queueSize;
}
public EventPublisherJob withQueueSize(Integer queueSize) {
this.queueSize = queueSize;
return this;
}
/**
* Max Concurrent Requests
*
* Maximum number of concurrent requests to the search index
*
*/
@JsonProperty("maxConcurrentRequests")
public Integer getMaxConcurrentRequests() {
return maxConcurrentRequests;
}
/**
* Max Concurrent Requests
*
* Maximum number of concurrent requests to the search index
*
*/
@JsonProperty("maxConcurrentRequests")
public void setMaxConcurrentRequests(Integer maxConcurrentRequests) {
this.maxConcurrentRequests = maxConcurrentRequests;
}
public EventPublisherJob withMaxConcurrentRequests(Integer maxConcurrentRequests) {
this.maxConcurrentRequests = maxConcurrentRequests;
return this;
}
/**
* Max Retries
*
* Maximum number of retries for a failed request
*
*/
@JsonProperty("maxRetries")
public Integer getMaxRetries() {
return maxRetries;
}
/**
* Max Retries
*
* Maximum number of retries for a failed request
*
*/
@JsonProperty("maxRetries")
public void setMaxRetries(Integer maxRetries) {
this.maxRetries = maxRetries;
}
public EventPublisherJob withMaxRetries(Integer maxRetries) {
this.maxRetries = maxRetries;
return this;
}
/**
* Initial Backoff Millis
*
* Initial backoff time in milliseconds
*
*/
@JsonProperty("initialBackoff")
public Integer getInitialBackoff() {
return initialBackoff;
}
/**
* Initial Backoff Millis
*
* Initial backoff time in milliseconds
*
*/
@JsonProperty("initialBackoff")
public void setInitialBackoff(Integer initialBackoff) {
this.initialBackoff = initialBackoff;
}
public EventPublisherJob withInitialBackoff(Integer initialBackoff) {
this.initialBackoff = initialBackoff;
return this;
}
/**
* Max Backoff Millis
*
* Maximum backoff time in milliseconds
*
*/
@JsonProperty("maxBackoff")
public Integer getMaxBackoff() {
return maxBackoff;
}
/**
* Max Backoff Millis
*
* Maximum backoff time in milliseconds
*
*/
@JsonProperty("maxBackoff")
public void setMaxBackoff(Integer maxBackoff) {
this.maxBackoff = maxBackoff;
}
public EventPublisherJob withMaxBackoff(Integer maxBackoff) {
this.maxBackoff = maxBackoff;
return this;
}
/**
* This schema defines the language options available for search index mappings.
*
*/
@JsonProperty("searchIndexMappingLanguage")
public IndexMappingLanguage getSearchIndexMappingLanguage() {
return searchIndexMappingLanguage;
}
/**
* This schema defines the language options available for search index mappings.
*
*/
@JsonProperty("searchIndexMappingLanguage")
public void setSearchIndexMappingLanguage(IndexMappingLanguage searchIndexMappingLanguage) {
this.searchIndexMappingLanguage = searchIndexMappingLanguage;
}
public EventPublisherJob withSearchIndexMappingLanguage(IndexMappingLanguage searchIndexMappingLanguage) {
this.searchIndexMappingLanguage = searchIndexMappingLanguage;
return this;
}
/**
* Provide After in case of failure to start reindexing after the issue is solved
*
*/
@JsonProperty("afterCursor")
public String getAfterCursor() {
return afterCursor;
}
/**
* Provide After in case of failure to start reindexing after the issue is solved
*
*/
@JsonProperty("afterCursor")
public void setAfterCursor(String afterCursor) {
this.afterCursor = afterCursor;
}
public EventPublisherJob withAfterCursor(String afterCursor) {
this.afterCursor = afterCursor;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(EventPublisherJob.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("name");
sb.append('=');
sb.append(((this.name == null)?"":this.name));
sb.append(',');
sb.append("timestamp");
sb.append('=');
sb.append(((this.timestamp == null)?"":this.timestamp));
sb.append(',');
sb.append("status");
sb.append('=');
sb.append(((this.status == null)?"":this.status));
sb.append(',');
sb.append("failure");
sb.append('=');
sb.append(((this.failure == null)?"":this.failure));
sb.append(',');
sb.append("stats");
sb.append('=');
sb.append(((this.stats == null)?"":this.stats));
sb.append(',');
sb.append("entities");
sb.append('=');
sb.append(((this.entities == null)?"":this.entities));
sb.append(',');
sb.append("recreateIndex");
sb.append('=');
sb.append(((this.recreateIndex == null)?"":this.recreateIndex));
sb.append(',');
sb.append("batchSize");
sb.append('=');
sb.append(((this.batchSize == null)?"":this.batchSize));
sb.append(',');
sb.append("payLoadSize");
sb.append('=');
sb.append(((this.payLoadSize == null)?"":this.payLoadSize));
sb.append(',');
sb.append("producerThreads");
sb.append('=');
sb.append(((this.producerThreads == null)?"":this.producerThreads));
sb.append(',');
sb.append("consumerThreads");
sb.append('=');
sb.append(((this.consumerThreads == null)?"":this.consumerThreads));
sb.append(',');
sb.append("queueSize");
sb.append('=');
sb.append(((this.queueSize == null)?"":this.queueSize));
sb.append(',');
sb.append("maxConcurrentRequests");
sb.append('=');
sb.append(((this.maxConcurrentRequests == null)?"":this.maxConcurrentRequests));
sb.append(',');
sb.append("maxRetries");
sb.append('=');
sb.append(((this.maxRetries == null)?"":this.maxRetries));
sb.append(',');
sb.append("initialBackoff");
sb.append('=');
sb.append(((this.initialBackoff == null)?"":this.initialBackoff));
sb.append(',');
sb.append("maxBackoff");
sb.append('=');
sb.append(((this.maxBackoff == null)?"":this.maxBackoff));
sb.append(',');
sb.append("searchIndexMappingLanguage");
sb.append('=');
sb.append(((this.searchIndexMappingLanguage == null)?"":this.searchIndexMappingLanguage));
sb.append(',');
sb.append("afterCursor");
sb.append('=');
sb.append(((this.afterCursor == null)?"":this.afterCursor));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.queueSize == null)? 0 :this.queueSize.hashCode()));
result = ((result* 31)+((this.maxBackoff == null)? 0 :this.maxBackoff.hashCode()));
result = ((result* 31)+((this.searchIndexMappingLanguage == null)? 0 :this.searchIndexMappingLanguage.hashCode()));
result = ((result* 31)+((this.recreateIndex == null)? 0 :this.recreateIndex.hashCode()));
result = ((result* 31)+((this.maxConcurrentRequests == null)? 0 :this.maxConcurrentRequests.hashCode()));
result = ((result* 31)+((this.initialBackoff == null)? 0 :this.initialBackoff.hashCode()));
result = ((result* 31)+((this.maxRetries == null)? 0 :this.maxRetries.hashCode()));
result = ((result* 31)+((this.stats == null)? 0 :this.stats.hashCode()));
result = ((result* 31)+((this.entities == null)? 0 :this.entities.hashCode()));
result = ((result* 31)+((this.producerThreads == null)? 0 :this.producerThreads.hashCode()));
result = ((result* 31)+((this.failure == null)? 0 :this.failure.hashCode()));
result = ((result* 31)+((this.afterCursor == null)? 0 :this.afterCursor.hashCode()));
result = ((result* 31)+((this.name == null)? 0 :this.name.hashCode()));
result = ((result* 31)+((this.payLoadSize == null)? 0 :this.payLoadSize.hashCode()));
result = ((result* 31)+((this.consumerThreads == null)? 0 :this.consumerThreads.hashCode()));
result = ((result* 31)+((this.batchSize == null)? 0 :this.batchSize.hashCode()));
result = ((result* 31)+((this.timestamp == null)? 0 :this.timestamp.hashCode()));
result = ((result* 31)+((this.status == null)? 0 :this.status.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof EventPublisherJob) == false) {
return false;
}
EventPublisherJob rhs = ((EventPublisherJob) other);
return (((((((((((((((((((this.queueSize == rhs.queueSize)||((this.queueSize!= null)&&this.queueSize.equals(rhs.queueSize)))&&((this.maxBackoff == rhs.maxBackoff)||((this.maxBackoff!= null)&&this.maxBackoff.equals(rhs.maxBackoff))))&&((this.searchIndexMappingLanguage == rhs.searchIndexMappingLanguage)||((this.searchIndexMappingLanguage!= null)&&this.searchIndexMappingLanguage.equals(rhs.searchIndexMappingLanguage))))&&((this.recreateIndex == rhs.recreateIndex)||((this.recreateIndex!= null)&&this.recreateIndex.equals(rhs.recreateIndex))))&&((this.maxConcurrentRequests == rhs.maxConcurrentRequests)||((this.maxConcurrentRequests!= null)&&this.maxConcurrentRequests.equals(rhs.maxConcurrentRequests))))&&((this.initialBackoff == rhs.initialBackoff)||((this.initialBackoff!= null)&&this.initialBackoff.equals(rhs.initialBackoff))))&&((this.maxRetries == rhs.maxRetries)||((this.maxRetries!= null)&&this.maxRetries.equals(rhs.maxRetries))))&&((this.stats == rhs.stats)||((this.stats!= null)&&this.stats.equals(rhs.stats))))&&((this.entities == rhs.entities)||((this.entities!= null)&&this.entities.equals(rhs.entities))))&&((this.producerThreads == rhs.producerThreads)||((this.producerThreads!= null)&&this.producerThreads.equals(rhs.producerThreads))))&&((this.failure == rhs.failure)||((this.failure!= null)&&this.failure.equals(rhs.failure))))&&((this.afterCursor == rhs.afterCursor)||((this.afterCursor!= null)&&this.afterCursor.equals(rhs.afterCursor))))&&((this.name == rhs.name)||((this.name!= null)&&this.name.equals(rhs.name))))&&((this.payLoadSize == rhs.payLoadSize)||((this.payLoadSize!= null)&&this.payLoadSize.equals(rhs.payLoadSize))))&&((this.consumerThreads == rhs.consumerThreads)||((this.consumerThreads!= null)&&this.consumerThreads.equals(rhs.consumerThreads))))&&((this.batchSize == rhs.batchSize)||((this.batchSize!= null)&&this.batchSize.equals(rhs.batchSize))))&&((this.timestamp == rhs.timestamp)||((this.timestamp!= null)&&this.timestamp.equals(rhs.timestamp))))&&((this.status == rhs.status)||((this.status!= null)&&this.status.equals(rhs.status))));
}
/**
* This schema publisher run job status.
*
*/
@Generated("jsonschema2pojo")
public enum Status {
STARTED("started"),
RUNNING("running"),
COMPLETED("completed"),
FAILED("failed"),
ACTIVE("active"),
ACTIVE_ERROR("activeError"),
STOPPED("stopped"),
SUCCESS("success"),
STOP_IN_PROGRESS("stopInProgress");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (EventPublisherJob.Status c: values()) {
CONSTANTS.put(c.value, c);
}
}
Status(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static EventPublisherJob.Status fromValue(String value) {
EventPublisherJob.Status constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}