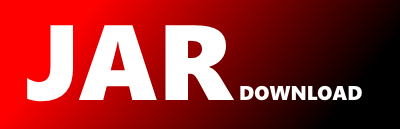
csharp-dotnet2.api.mustache Maven / Gradle / Ivy
using System;
using System.Collections.Generic;
using RestSharp;
using {{clientPackage}};
{{#hasImport}}using {{modelPackage}};
{{/hasImport}}
namespace {{apiPackage}}
{
{{#operations}}
///
/// Represents a collection of functions to interact with the API endpoints
///
public interface I{{classname}}
{
{{#operation}}
///
/// {{summary}} {{notes}}
///
{{#allParams}}/// {{description}}
{{/allParams}}/// {{#returnType}}{{returnType}}{{/returnType}}
{{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}void{{/returnType}} {{nickname}} ({{#allParams}}{{{dataType}}} {{paramName}}{{#hasMore}}, {{/hasMore}}{{/allParams}});
{{/operation}}
}
///
/// Represents a collection of functions to interact with the API endpoints
///
public class {{classname}} : I{{classname}}
{
///
/// Initializes a new instance of the class.
///
/// an instance of ApiClient (optional)
///
public {{classname}}(ApiClient apiClient = null)
{
if (apiClient == null) // use the default one in Configuration
this.ApiClient = Configuration.DefaultApiClient;
else
this.ApiClient = apiClient;
}
///
/// Initializes a new instance of the class.
///
///
public {{classname}}(String basePath)
{
this.ApiClient = new ApiClient(basePath);
}
///
/// Sets the base path of the API client.
///
/// The base path
/// The base path
public void SetBasePath(String basePath)
{
this.ApiClient.BasePath = basePath;
}
///
/// Gets the base path of the API client.
///
/// The base path
/// The base path
public String GetBasePath(String basePath)
{
return this.ApiClient.BasePath;
}
///
/// Gets or sets the API client.
///
/// An instance of the ApiClient
public ApiClient ApiClient {get; set;}
{{#operation}}
///
/// {{summary}} {{notes}}
///
{{#allParams}}/// {{description}}
{{/allParams}}/// {{#returnType}}{{returnType}}{{/returnType}}
public {{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}void{{/returnType}} {{nickname}} ({{#allParams}}{{{dataType}}} {{paramName}}{{#hasMore}}, {{/hasMore}}{{/allParams}})
{
{{#allParams}}{{#required}}
// verify the required parameter '{{paramName}}' is set
if ({{paramName}} == null) throw new ApiException(400, "Missing required parameter '{{paramName}}' when calling {{nickname}}");
{{/required}}{{/allParams}}
var path = "{{{path}}}";
path = path.Replace("{format}", "json");
{{#pathParams}}path = path.Replace("{" + "{{baseName}}" + "}", ApiClient.ParameterToString({{{paramName}}}));
{{/pathParams}}
var queryParams = new Dictionary();
var headerParams = new Dictionary();
var formParams = new Dictionary();
var fileParams = new Dictionary();
String postBody = null;
{{#queryParams}} if ({{paramName}} != null) queryParams.Add("{{baseName}}", ApiClient.ParameterToString({{paramName}})); // query parameter
{{/queryParams}}
{{#headerParams}} if ({{paramName}} != null) headerParams.Add("{{baseName}}", ApiClient.ParameterToString({{paramName}})); // header parameter
{{/headerParams}}
{{#formParams}}if ({{paramName}} != null) {{#isFile}}fileParams.Add("{{baseName}}", ApiClient.ParameterToFile("{{baseName}}", {{paramName}}));{{/isFile}}{{^isFile}}formParams.Add("{{baseName}}", ApiClient.ParameterToString({{paramName}})); // form parameter{{/isFile}}
{{/formParams}}
{{#bodyParam}}postBody = ApiClient.Serialize({{paramName}}); // http body (model) parameter
{{/bodyParam}}
// authentication setting, if any
String[] authSettings = new String[] { {{#authMethods}}"{{name}}"{{#hasMore}}, {{/hasMore}}{{/authMethods}} };
// make the HTTP request
IRestResponse response = (IRestResponse) ApiClient.CallApi(path, Method.{{httpMethod}}, queryParams, postBody, headerParams, formParams, fileParams, authSettings);
if (((int)response.StatusCode) >= 400)
throw new ApiException ((int)response.StatusCode, "Error calling {{nickname}}: " + response.Content, response.Content);
else if (((int)response.StatusCode) == 0)
throw new ApiException ((int)response.StatusCode, "Error calling {{nickname}}: " + response.ErrorMessage, response.ErrorMessage);
{{#returnType}}return ({{{returnType}}}) ApiClient.Deserialize(response.Content, typeof({{{returnType}}}), response.Headers);{{/returnType}}{{^returnType}}return;{{/returnType}}
}
{{/operation}}
}
{{/operations}}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy