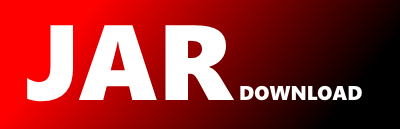
csharp-dotnet2.Configuration.mustache Maven / Gradle / Ivy
using System;
using System.Reflection;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
namespace {{clientPackage}}
{
///
/// Represents a set of configuration settings
///
public class Configuration
{
///
/// Version of the package.
///
/// Version of the package.
public const string Version = "{{packageVersion}}";
///
/// Gets or sets the default API client for making HTTP calls.
///
/// The API client.
public static ApiClient DefaultApiClient = new ApiClient();
///
/// Gets or sets the username (HTTP basic authentication).
///
/// The username.
public static String Username { get; set; }
///
/// Gets or sets the password (HTTP basic authentication).
///
/// The password.
public static String Password { get; set; }
///
/// Gets or sets the API key based on the authentication name.
///
/// The API key.
public static Dictionary ApiKey = new Dictionary();
///
/// Gets or sets the prefix (e.g. Token) of the API key based on the authentication name.
///
/// The prefix of the API key.
public static Dictionary ApiKeyPrefix = new Dictionary();
private static string _tempFolderPath = Path.GetTempPath();
///
/// Gets or sets the temporary folder path to store the files downloaded from the server.
///
/// Folder path.
public static String TempFolderPath
{
get { return _tempFolderPath; }
set
{
if (String.IsNullOrEmpty(value))
{
_tempFolderPath = value;
return;
}
// create the directory if it does not exist
if (!Directory.Exists(value))
Directory.CreateDirectory(value);
// check if the path contains directory separator at the end
if (value[value.Length - 1] == Path.DirectorySeparatorChar)
_tempFolderPath = value;
else
_tempFolderPath = value + Path.DirectorySeparatorChar;
}
}
private const string ISO8601_DATETIME_FORMAT = "o";
private static string _dateTimeFormat = ISO8601_DATETIME_FORMAT;
///
/// Gets or sets the the date time format used when serializing in the ApiClient
/// By default, it's set to ISO 8601 - "o", for others see:
/// https://msdn.microsoft.com/en-us/library/az4se3k1(v=vs.110).aspx
/// and https://msdn.microsoft.com/en-us/library/8kb3ddd4(v=vs.110).aspx
/// No validation is done to ensure that the string you're providing is valid
///
/// The DateTimeFormat string
public static String DateTimeFormat
{
get
{
return _dateTimeFormat;
}
set
{
if (string.IsNullOrEmpty(value))
{
// Never allow a blank or null string, go back to the default
_dateTimeFormat = ISO8601_DATETIME_FORMAT;
return;
}
// Caution, no validation when you choose date time format other than ISO 8601
// Take a look at the above links
_dateTimeFormat = value;
}
}
///
/// Returns a string with essential information for debugging.
///
public static String ToDebugReport()
{
String report = "C# SDK ({{packageName}}) Debug Report:\n";
report += " OS: " + Environment.OSVersion + "\n";
report += " .NET Framework Version: " + Assembly
.GetExecutingAssembly()
.GetReferencedAssemblies()
.Where(x => x.Name == "System.Core").First().Version.ToString() + "\n";
report += " Version of the API: {{version}}\n";
report += " SDK Package Version: {{packageVersion}}\n";
return report;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy