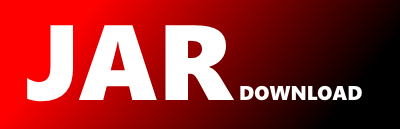
cpp-pistache-server.modelbase-header.mustache Maven / Gradle / Ivy
{{>licenseInfo}}
/*
* {{prefix}}ModelBase.h
*
* This is the base class for all model classes
*/
#ifndef {{prefix}}ModelBase_H_
#define {{prefix}}ModelBase_H_
{{{defaultInclude}}}
#include "json.hpp"
#include
#include
#include
#include
© 2015 - 2025 Weber Informatics LLC | Privacy Policy