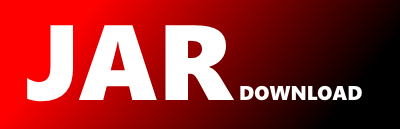
dart.api_client.mustache Maven / Gradle / Ivy
part of {{pubName}}.api;
class QueryParam {
String name;
String value;
QueryParam(this.name, this.value);
}
class ApiClient {
String basePath;
var client = new {{#browserClient}}Browser{{/browserClient}}Client();
Map _defaultHeaderMap = {};
Map _authentications = {};
final _RegList = new RegExp(r'^List<(.*)>$');
final _RegMap = new RegExp(r'^Map$');
ApiClient({this.basePath: "{{{basePath}}}"}) {
// Setup authentications (key: authentication name, value: authentication).{{#authMethods}}{{#isBasic}}
_authentications['{{name}}'] = new HttpBasicAuth();{{/isBasic}}{{#isApiKey}}
_authentications['{{name}}'] = new ApiKeyAuth({{#isKeyInHeader}}"header"{{/isKeyInHeader}}{{^isKeyInHeader}}"query"{{/isKeyInHeader}}, "{{keyParamName}}");{{/isApiKey}}{{#isOAuth}}
_authentications['{{name}}'] = new OAuth();{{/isOAuth}}{{/authMethods}}
}
void addDefaultHeader(String key, String value) {
_defaultHeaderMap[key] = value;
}
dynamic _deserialize(dynamic value, String targetType) {
try {
switch (targetType) {
case 'String':
return '$value';
case 'int':
return value is int ? value : int.parse('$value');
case 'bool':
return value is bool ? value : '$value'.toLowerCase() == 'true';
case 'double':
return value is double ? value : double.parse('$value');
{{#models}}
{{#model}}
case '{{classname}}':
{{#isEnum}}
// Enclose the value in a list so that Dartson can use a transformer
// to decode it.
final listValue = [value];
final List listResult = dson.map(listValue, []);
return listResult[0];
{{/isEnum}}
{{^isEnum}}
return new {{classname}}.fromJson(value);
{{/isEnum}}
{{/model}}
{{/models}}
default:
{
Match match;
if (value is List &&
(match = _RegList.firstMatch(targetType)) != null) {
var newTargetType = match[1];
return value.map((v) => _deserialize(v, newTargetType)).toList();
} else if (value is Map &&
(match = _RegMap.firstMatch(targetType)) != null) {
var newTargetType = match[1];
return new Map.fromIterables(value.keys,
value.values.map((v) => _deserialize(v, newTargetType)));
}
}
}
} catch (e, stack) {
throw new ApiException.withInner(500, 'Exception during deserialization.', e, stack);
}
throw new ApiException(500, 'Could not find a suitable class for deserialization');
}
dynamic deserialize(String json, String targetType) {
// Remove all spaces. Necessary for reg expressions as well.
targetType = targetType.replaceAll(' ', '');
if (targetType == 'String') return json;
var decodedJson = JSON.decode(json);
return _deserialize(decodedJson, targetType);
}
String serialize(Object obj) {
String serialized = '';
if (obj == null) {
serialized = '';
} else {
serialized = JSON.encode(obj);
}
return serialized;
}
// We don't use a Map for queryParams.
// If collectionFormat is 'multi' a key might appear multiple times.
Future invokeAPI(String path,
String method,
Iterable queryParams,
Object body,
Map headerParams,
Map formParams,
String contentType,
List authNames) async {
_updateParamsForAuth(authNames, queryParams, headerParams);
var ps = queryParams.where((p) => p.value != null).map((p) => '${p.name}=${p.value}');
String queryString = ps.isNotEmpty ?
'?' + ps.join('&') :
'';
String url = basePath + path + queryString;
headerParams.addAll(_defaultHeaderMap);
headerParams['Content-Type'] = contentType;
if(body is MultipartRequest) {
var request = new MultipartRequest(method, Uri.parse(url));
request.fields.addAll(body.fields);
request.files.addAll(body.files);
request.headers.addAll(body.headers);
request.headers.addAll(headerParams);
var response = await client.send(request);
return Response.fromStream(response);
} else {
var msgBody = contentType == "application/x-www-form-urlencoded" ? formParams : serialize(body);
switch(method) {
case "POST":
return client.post(url, headers: headerParams, body: msgBody);
case "PUT":
return client.put(url, headers: headerParams, body: msgBody);
case "DELETE":
return client.delete(url, headers: headerParams);
case "PATCH":
return client.patch(url, headers: headerParams, body: msgBody);
default:
return client.get(url, headers: headerParams);
}
}
}
/// Update query and header parameters based on authentication settings.
/// @param authNames The authentications to apply
void _updateParamsForAuth(List authNames, List queryParams, Map headerParams) {
authNames.forEach((authName) {
Authentication auth = _authentications[authName];
if (auth == null) throw new ArgumentError("Authentication undefined: " + authName);
auth.applyToParams(queryParams, headerParams);
});
}
void setAccessToken(String accessToken) {
_authentications.forEach((key, auth) {
if (auth is OAuth) {
auth.setAccessToken(accessToken);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy