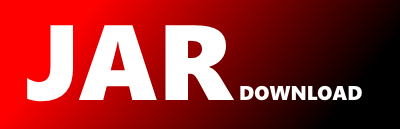
csharp-netcore.ISynchronousClient.mustache Maven / Gradle / Ivy
{{>partial_header}}
using System;
using System.IO;
namespace {{packageName}}.Client
{
///
/// Contract for Synchronous RESTful API interactions.
///
/// This interface allows consumers to provide a custom API accessor client.
///
public interface ISynchronousClient
{
///
/// Executes a blocking call to some using the GET http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Get(String path, RequestOptions options, IReadableConfiguration configuration = null);
///
/// Executes a blocking call to some using the POST http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Post(String path, RequestOptions options, IReadableConfiguration configuration = null);
///
/// Executes a blocking call to some using the PUT http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Put(String path, RequestOptions options, IReadableConfiguration configuration = null);
///
/// Executes a blocking call to some using the DELETE http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Delete(String path, RequestOptions options, IReadableConfiguration configuration = null);
///
/// Executes a blocking call to some using the HEAD http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Head(String path, RequestOptions options, IReadableConfiguration configuration = null);
///
/// Executes a blocking call to some using the OPTIONS http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Options(String path, RequestOptions options, IReadableConfiguration configuration = null);
///
/// Executes a blocking call to some using the PATCH http verb.
///
/// The relative path to invoke.
/// The request parameters to pass along to the client.
/// Per-request configurable settings.
/// The return type.
/// The response data, decorated with
ApiResponse Patch(String path, RequestOptions options, IReadableConfiguration configuration = null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy