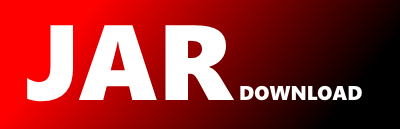
csharp-netcore.Multimap.mustache Maven / Gradle / Ivy
{{>partial_header}}
using System;
using System.Collections;
{{^net35}}using System.Collections.Concurrent;{{/net35}}
using System.Collections.Generic;
namespace {{packageName}}.Client
{
///
/// A dictionary in which one key has many associated values.
///
/// The type of the key
/// The type of the value associated with the key.
public class Multimap : IDictionary>
{
#region Private Fields
private readonly {{^net35}}Concurrent{{/net35}}Dictionary> _dictionary =
new {{^net35}}Concurrent{{/net35}}Dictionary>();
#endregion Private Fields
#region Enumerators
///
/// To get the enumerator.
///
/// Enumerator
public IEnumerator>> GetEnumerator()
{
return _dictionary.GetEnumerator();
}
///
/// To get the enumerator.
///
/// Enumerator
IEnumerator IEnumerable.GetEnumerator()
{
return _dictionary.GetEnumerator();
}
#endregion Enumerators
#region Public Members
///
/// Add values to Multimap
///
/// Key value pair
public void Add(KeyValuePair> item)
{
if (!TryAdd(item.Key, item.Value))
throw new InvalidOperationException("Could not add values to Multimap.");
}
///
/// Clear Multimap
///
public void Clear()
{
_dictionary.Clear();
}
public bool Contains(KeyValuePair> item)
{
throw new NotImplementedException();
}
public void CopyTo(KeyValuePair>[] array, int arrayIndex)
{
throw new NotImplementedException();
}
public bool Remove(KeyValuePair> item)
{
throw new NotImplementedException();
}
public int Count
{
get
{
return _dictionary.Count;
}
}
public bool IsReadOnly
{
get
{
return false;
}
}
public void Add(T key, IList value)
{
if (value != null && value.Count > 0)
{
IList list;
if (_dictionary.TryGetValue(key, out list))
{
foreach (var k in value) list.Add(k);
}
else
{
list = new List(value);
if (!TryAdd(key, list))
throw new InvalidOperationException("Could not add values to Multimap.");
}
}
}
public bool ContainsKey(T key)
{
return _dictionary.ContainsKey(key);
}
public bool Remove(T key)
{
IList list;
return TryRemove(key, out list);
}
public bool TryGetValue(T key, out IList value)
{
return _dictionary.TryGetValue(key, out value);
}
public IList this[T key]
{
get
{
return _dictionary[key];
}
set { _dictionary[key] = value; }
}
public ICollection Keys
{
get
{
return _dictionary.Keys;
}
}
public ICollection> Values
{
get
{
return _dictionary.Values;
}
}
public void CopyTo(Array array, int index)
{
((ICollection) _dictionary).CopyTo(array, index);
}
public void Add(T key, TValue value)
{
if (value != null)
{
IList list;
if (_dictionary.TryGetValue(key, out list))
{
list.Add(value);
}
else
{
list = new List();
list.Add(value);
if (!TryAdd(key, list))
throw new InvalidOperationException("Could not add value to Multimap.");
}
}
}
#endregion Public Members
#region Private Members
/**
* Helper method to encapsulate generator differences between dictioary types.
*/
private bool TryRemove(T key, out IList value)
{
{{^net35}}return _dictionary.TryRemove(key, out value);{{/net35}}
{{#net35}}try
{
_dictionary.TryGetValue(key, out value);
_dictionary.Remove(key);
}
#pragma warning disable 168
catch (ArgumentException e)
#pragma warning restore 168
{
value = null;
return false;
}
return true;{{/net35}}
}
/**
* Helper method to encapsulate generator differences between dictioary types.
*/
private bool TryAdd(T key, IList value)
{
{{^net35}}return _dictionary.TryAdd(key, value);{{/net35}}
{{#net35}}
try
{
_dictionary.Add(key, value);
}
#pragma warning disable 168
catch (ArgumentException e)
#pragma warning restore 168
{
return false;
}
return true;
{{/net35}}
}
#endregion Private Members
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy