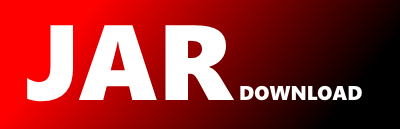
php-slim-server.SlimRouter.mustache Maven / Gradle / Ivy
'{{httpMethod}}',
'basePathWithoutHost' => '{{{basePathWithoutHost}}}',
'path' => '{{{path}}}',
'apiPackage' => '{{apiPackage}}',
'classname' => '{{classname}}',
'userClassname' => '{{userClassname}}',
'operationId' => '{{operationId}}',
'authMethods' => [
{{#hasAuthMethods}}
{{#authMethods}}
// {{type}} security schema named '{{name}}'
{{#isBasic}}
[
'type' => '{{type}}',
'isBasic' => true,
'isApiKey' => false,
'isOAuth' => false,
],
{{/isBasic}}
{{#isApiKey}}
[
'type' => '{{type}}',
'isBasic' => false,
'isApiKey' => true,
'isOAuth' => false,
'keyParamName' => '{{keyParamName}}',
'isKeyInHeader' => {{#isKeyInHeader}}true{{/isKeyInHeader}}{{^isKeyInHeader}}false{{/isKeyInHeader}},
'isKeyInQuery' => {{#isKeyInQuery}}true{{/isKeyInQuery}}{{^isKeyInQuery}}false{{/isKeyInQuery}},
'isKeyInCookie' => {{#isKeyInCookie}}true{{/isKeyInCookie}}{{^isKeyInCookie}}false{{/isKeyInCookie}},
],
{{/isApiKey}}
{{#isOAuth}}
[
'type' => '{{type}}',
'isBasic' => false,
'isApiKey' => false,
'isOAuth' => true,
'scopes' => [
{{#scopes}}
'{{scope}}',{{#description}} // {{description}}{{/description}}
{{/scopes}}
],
],
{{/isOAuth}}
{{/authMethods}}
{{/hasAuthMethods}}
],
],
{{/operation}}
{{/operations}}
{{/apis}}
];
/**
* Class constructor
*
* @param ContainerInterface|array $container Either a ContainerInterface or an associative array of app settings
*
* @throws InvalidArgumentException When no container is provided that implements ContainerInterface
* @throws Exception When implementation class doesn't exists
*/
public function __construct($container = [])
{
$this->slimApp = new App($container);
{{#hasAuthMethods}}
$authPackage = '{{authPackage}}';
$basicAuthenticator = function (ServerRequestInterface &$request, TokenSearch $tokenSearch) use ($authPackage) {
$message = "How about extending {{abstractNamePrefix}}Authenticator{{abstractNameSuffix}} class by {$authPackage}\BasicAuthenticator?";
throw new Exception($message);
};
$apiKeyAuthenticator = function (ServerRequestInterface &$request, TokenSearch $tokenSearch) use ($authPackage) {
$message = "How about extending {{abstractNamePrefix}}Authenticator{{abstractNameSuffix}} class by {$authPackage}\ApiKeyAuthenticator?";
throw new Exception($message);
};
$oAuthAuthenticator = function (ServerRequestInterface &$request, TokenSearch $tokenSearch) use ($authPackage) {
$message = "How about extending {{abstractNamePrefix}}Authenticator{{abstractNameSuffix}} class by {$authPackage}\OAuthAuthenticator?";
throw new Exception($message);
};
{{/hasAuthMethods}}
foreach ($this->operations as $operation) {
$callback = function ($request, $response, $arguments) use ($operation) {
$message = "How about extending {$operation['classname']} by {$operation['apiPackage']}\\{$operation['userClassname']} class implementing {$operation['operationId']} as a {$operation['httpMethod']} method?";
throw new Exception($message);
return $response->withStatus(501)->write($message);
};
$middlewares = [];
if (class_exists("\\{$operation['apiPackage']}\\{$operation['userClassname']}")) {
$callback = "\\{$operation['apiPackage']}\\{$operation['userClassname']}:{$operation['operationId']}";
}
{{#hasAuthMethods}}
foreach ($operation['authMethods'] as $authMethod) {
switch ($authMethod['type']) {
case 'http':
$authenticatorClassname = "\\{$authPackage}\\BasicAuthenticator";
if (class_exists($authenticatorClassname)) {
$basicAuthenticator = new $authenticatorClassname($container);
}
$middlewares[] = new TokenAuthentication($this->getTokenAuthenticationOptions([
'authenticator' => $basicAuthenticator,
'regex' => '/Basic\s+(.*)$/i',
'header' => 'Authorization',
'parameter' => null,
'cookie' => null,
'argument' => null,
]));
break;
case 'apiKey':
$authenticatorClassname = "\\{$authPackage}\\ApiKeyAuthenticator";
if (class_exists($authenticatorClassname)) {
$apiKeyAuthenticator = new $authenticatorClassname($container);
}
$middlewares[] = new TokenAuthentication($this->getTokenAuthenticationOptions([
'authenticator' => $apiKeyAuthenticator,
'regex' => '/^(.*)$/i',
'header' => $authMethod['isKeyInHeader'] ? $authMethod['keyParamName'] : null,
'parameter' => $authMethod['isKeyInQuery'] ? $authMethod['keyParamName'] : null,
'cookie' => $authMethod['isKeyInCookie'] ? $authMethod['keyParamName'] : null,
'argument' => null,
]));
break;
case 'oauth2':
$authenticatorClassname = "\\{$authPackage}\\OAuthAuthenticator";
if (class_exists($authenticatorClassname)) {
$oAuthAuthenticator = new $authenticatorClassname($container, $authMethod['scopes']);
}
$middlewares[] = new TokenAuthentication($this->getTokenAuthenticationOptions([
'authenticator' => $oAuthAuthenticator,
'regex' => '/Bearer\s+(.*)$/i',
'header' => 'Authorization',
'parameter' => null,
'cookie' => null,
'argument' => null,
]));
break;
default:
throw new Exception('Unknown authorization schema type');
}
}
{{/hasAuthMethods}}
$this->addRoute(
[$operation['httpMethod']],
"{$operation['basePathWithoutHost']}{$operation['path']}",
$callback,
$middlewares
)->setName($operation['operationId']);
}
}
/**
* Merges user defined options with dynamic params
*
* @param array $options Params which need to merge into user options
*
* @return array Merged array
*/
private function getTokenAuthenticationOptions(array $options)
{
if (is_array($this->slimApp->getContainer()['tokenAuthenticationOptions']) === false) {
return $options;
}
return array_merge($this->slimApp->getContainer()['tokenAuthenticationOptions'], $options);
}
/**
* Add route with multiple methods
*
* @param string[] $methods Numeric array of HTTP method names
* @param string $pattern The route URI pattern
* @param callable|string $callable The route callback routine
* @param array|null $middlewares List of middlewares
*
* @return RouteInterface
*
* @throws InvalidArgumentException If the route pattern isn't a string
*/
public function addRoute(array $methods, string $pattern, $callable, $middlewares = [])
{
$route = $this->slimApp->map($methods, $pattern, $callable);
foreach ($middlewares as $middleware) {
$route->add($middleware);
}
return $route;
}
/**
* Returns Slim Framework instance
*
* @return App
*/
public function getSlimApp()
{
return $this->slimApp;
}
}
{{/apiInfo}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy