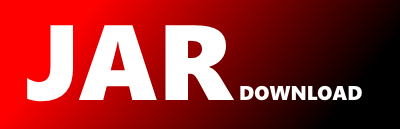
csharp.modelGeneric.mustache Maven / Gradle / Ivy
///
/// {{#description}}{{.}}{{/description}}{{^description}}{{classname}}{{/description}}
///
[DataContract]
{{#discriminator}}
[JsonConverter(typeof(JsonSubtypes), "{{{discriminatorName}}}")]{{#children}}
[JsonSubtypes.KnownSubType(typeof({{classname}}), "{{^vendorExtensions.x-discriminator-value}}{{name}}{{/vendorExtensions.x-discriminator-value}}{{#vendorExtensions.x-discriminator-value}}{{{vendorExtensions.x-discriminator-value}}}{{/vendorExtensions.x-discriminator-value}}")]{{/children}}
{{/discriminator}}
{{#generatePropertyChanged}}
[ImplementPropertyChanged]
{{/generatePropertyChanged}}
{{>visibility}} partial class {{classname}} : {{#parent}}{{{parent}}}, {{/parent}} IEquatable<{{classname}}>{{^netStandard}}{{#validatable}}, IValidatableObject{{/validatable}}{{/netStandard}}
{
{{#vars}}
{{#items.isEnum}}
{{#items}}
{{^complexType}}
{{>modelInnerEnum}}
{{/complexType}}
{{/items}}
{{/items.isEnum}}
{{#isEnum}}
{{^complexType}}
{{>modelInnerEnum}}
{{/complexType}}
{{/isEnum}}
{{#isEnum}}
///
/// {{^description}}Gets or Sets {{{name}}}{{/description}}{{#description}}{{description}}{{/description}}
///
{{#description}}
/// {{description}}
{{/description}}
[DataMember(Name="{{baseName}}", EmitDefaultValue={{#vendorExtensions.x-emit-default-value}}true{{/vendorExtensions.x-emit-default-value}}{{^vendorExtensions.x-emit-default-value}}{{#isNullable}}true{{/isNullable}}{{^isNullable}}false{{/isNullable}}{{/vendorExtensions.x-emit-default-value}})]
public {{#complexType}}{{{complexType}}}{{/complexType}}{{^complexType}}{{{datatypeWithEnum}}}{{/complexType}}{{^isContainer}}{{^required}}?{{/required}}{{/isContainer}} {{name}} { get; set; }
{{/isEnum}}
{{/vars}}
{{#hasRequired}}
{{^hasOnlyReadOnly}}
///
/// Initializes a new instance of the class.
///
[JsonConstructorAttribute]
protected {{classname}}() { }
{{/hasOnlyReadOnly}}
{{/hasRequired}}
///
/// Initializes a new instance of the class.
///
{{#vars}}
{{^isReadOnly}}
/// {{#description}}{{description}}{{/description}}{{^description}}{{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}}{{/description}}{{#required}} (required){{/required}}{{#defaultValue}} (default to {{defaultValue}}){{/defaultValue}}.
{{/isReadOnly}}
{{/vars}}
{{#hasOnlyReadOnly}}
[JsonConstructorAttribute]
{{/hasOnlyReadOnly}}
public {{classname}}({{#readWriteVars}}{{{datatypeWithEnum}}}{{#isEnum}}{{^isContainer}}{{^required}}?{{/required}}{{/isContainer}}{{/isEnum}} {{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}} = {{#defaultValue}}{{{defaultValue}}}{{/defaultValue}}{{^defaultValue}}default({{{datatypeWithEnum}}}{{#isEnum}}{{^isContainer}}{{^required}}?{{/required}}{{/isContainer}}{{/isEnum}}){{/defaultValue}}{{^-last}}, {{/-last}}{{/readWriteVars}}){{#parent}} : base({{#parentVars}}{{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}}{{#hasMore}}, {{/hasMore}}{{/parentVars}}){{/parent}}
{
{{#vars}}
{{^isInherited}}
{{^isReadOnly}}
{{#required}}
// to ensure "{{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}}" is required (not null)
if ({{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}} == null)
{
throw new InvalidDataException("{{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}} is a required property for {{classname}} and cannot be null");
}
else
{
this.{{name}} = {{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}};
}
{{/required}}
{{#isNullable}}
this.{{name}} = {{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}};
{{/isNullable}}
{{/isReadOnly}}
{{/isInherited}}
{{/vars}}
{{#vars}}
{{^isInherited}}
{{^isReadOnly}}
{{^required}}
{{#defaultValue}}// use default value if no "{{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}}" provided
if ({{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}} == null)
{
this.{{name}} = {{{defaultValue}}};
}
else
{
this.{{name}} = {{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}};
}
{{/defaultValue}}
{{^defaultValue}}
this.{{name}} = {{#lambda.camelcase_param}}{{name}}{{/lambda.camelcase_param}};
{{/defaultValue}}
{{/required}}
{{/isReadOnly}}
{{/isInherited}}
{{/vars}}
}
{{#vars}}
{{^isInherited}}
{{^isEnum}}
///
/// {{^description}}Gets or Sets {{{name}}}{{/description}}{{#description}}{{description}}{{/description}}
/// {{#description}}
/// {{description}} {{/description}}
[DataMember(Name="{{baseName}}", EmitDefaultValue={{#vendorExtensions.x-emit-default-value}}true{{/vendorExtensions.x-emit-default-value}}{{^vendorExtensions.x-emit-default-value}}{{#isNullable}}true{{/isNullable}}{{^isNullable}}false{{/isNullable}}{{/vendorExtensions.x-emit-default-value}})]{{#isDate}}
[JsonConverter(typeof(OpenAPIDateConverter))]{{/isDate}}
public {{{dataType}}} {{name}} { get; {{#isReadOnly}}private {{/isReadOnly}}set; }
{{/isEnum}}
{{/isInherited}}
{{/vars}}
///
/// Returns the string presentation of the object
///
/// String presentation of the object
public override string ToString()
{
var sb = new StringBuilder();
sb.Append("class {{classname}} {\n");
{{#parent}}
sb.Append(" ").Append(base.ToString().Replace("\n", "\n ")).Append("\n");
{{/parent}}
{{#vars}}
sb.Append(" {{name}}: ").Append({{name}}).Append("\n");
{{/vars}}
sb.Append("}\n");
return sb.ToString();
}
///
/// Returns the JSON string presentation of the object
///
/// JSON string presentation of the object
public {{#parent}}{{^isArrayModel}}{{^isMapModel}}override {{/isMapModel}}{{/isArrayModel}}{{/parent}}{{^parent}}virtual {{/parent}}string ToJson()
{
return JsonConvert.SerializeObject(this, Formatting.Indented);
}
///
/// Returns true if objects are equal
///
/// Object to be compared
/// Boolean
public override bool Equals(object input)
{
return this.Equals(input as {{classname}});
}
///
/// Returns true if {{classname}} instances are equal
///
/// Instance of {{classname}} to be compared
/// Boolean
public bool Equals({{classname}} input)
{
if (input == null)
return false;
return {{#vars}}{{#parent}}base.Equals(input) && {{/parent}}{{^isContainer}}
(
this.{{name}} == input.{{name}} ||
(this.{{name}} != null &&
this.{{name}}.Equals(input.{{name}}))
){{#hasMore}} && {{/hasMore}}{{/isContainer}}{{#isContainer}}
(
this.{{name}} == input.{{name}} ||
this.{{name}} != null &&
input.{{name}} != null &&
this.{{name}}.SequenceEqual(input.{{name}})
){{#hasMore}} && {{/hasMore}}{{/isContainer}}{{/vars}}{{^vars}}{{#parent}}base.Equals(input){{/parent}}{{^parent}}false{{/parent}}{{/vars}};
}
///
/// Gets the hash code
///
/// Hash code
public override int GetHashCode()
{
unchecked // Overflow is fine, just wrap
{
{{#parent}}
int hashCode = base.GetHashCode();
{{/parent}}
{{^parent}}
int hashCode = 41;
{{/parent}}
{{#vars}}
if (this.{{name}} != null)
hashCode = hashCode * 59 + this.{{name}}.GetHashCode();
{{/vars}}
return hashCode;
}
}
{{^netStandard}}
{{#generatePropertyChanged}}
///
/// Property changed event handler
///
public event PropertyChangedEventHandler PropertyChanged;
///
/// Trigger when a property changed
///
/// Property Name
public virtual void OnPropertyChanged(string propertyName)
{
// NOTE: property changed is handled via "code weaving" using Fody.
// Properties with setters are modified at compile time to notify of changes.
var propertyChanged = PropertyChanged;
if (propertyChanged != null)
{
propertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
{{/generatePropertyChanged}}
{{#validatable}}
{{#hasChildren}}
///
/// To validate all properties of the instance
///
/// Validation context
/// Validation Result
IEnumerable IValidatableObject.Validate(ValidationContext validationContext)
{
return this.BaseValidate(validationContext);
}
///
/// To validate all properties of the instance
///
/// Validation context
/// Validation Result
protected IEnumerable BaseValidate(ValidationContext validationContext)
{
{{/hasChildren}}
{{^hasChildren}}
///
/// To validate all properties of the instance
///
/// Validation context
/// Validation Result
IEnumerable IValidatableObject.Validate(ValidationContext validationContext)
{
{{/hasChildren}}
{{#parent}}
{{^isArrayModel}}
{{^isMapModel}}
foreach(var x in base.BaseValidate(validationContext)) yield return x;
{{/isMapModel}}
{{/isArrayModel}}
{{/parent}}
{{#vars}}
{{#hasValidation}}
{{#maxLength}}
// {{{name}}} ({{{dataType}}}) maxLength
if(this.{{{name}}} != null && this.{{{name}}}.Length > {{maxLength}})
{
yield return new System.ComponentModel.DataAnnotations.ValidationResult("Invalid value for {{{name}}}, length must be less than {{maxLength}}.", new [] { "{{{name}}}" });
}
{{/maxLength}}
{{#minLength}}
// {{{name}}} ({{{dataType}}}) minLength
if(this.{{{name}}} != null && this.{{{name}}}.Length < {{minLength}})
{
yield return new System.ComponentModel.DataAnnotations.ValidationResult("Invalid value for {{{name}}}, length must be greater than {{minLength}}.", new [] { "{{{name}}}" });
}
{{/minLength}}
{{#maximum}}
// {{{name}}} ({{{dataType}}}) maximum
if(this.{{{name}}} > ({{{dataType}}}){{maximum}})
{
yield return new System.ComponentModel.DataAnnotations.ValidationResult("Invalid value for {{{name}}}, must be a value less than or equal to {{maximum}}.", new [] { "{{{name}}}" });
}
{{/maximum}}
{{#minimum}}
// {{{name}}} ({{{dataType}}}) minimum
if(this.{{{name}}} < ({{{dataType}}}){{minimum}})
{
yield return new System.ComponentModel.DataAnnotations.ValidationResult("Invalid value for {{{name}}}, must be a value greater than or equal to {{minimum}}.", new [] { "{{{name}}}" });
}
{{/minimum}}
{{#pattern}}
{{^isByteArray}}
// {{{name}}} ({{{dataType}}}) pattern
Regex regex{{{name}}} = new Regex(@"{{{vendorExtensions.x-regex}}}"{{#vendorExtensions.x-modifiers}}{{#-first}}, {{/-first}}RegexOptions.{{{.}}}{{^-last}} | {{/-last}}{{/vendorExtensions.x-modifiers}});
if (false == regex{{{name}}}.Match(this.{{{name}}}).Success)
{
yield return new System.ComponentModel.DataAnnotations.ValidationResult("Invalid value for {{{name}}}, must match a pattern of " + regex{{{name}}}, new [] { "{{{name}}}" });
}
{{/isByteArray}}
{{/pattern}}
{{/hasValidation}}
{{/vars}}
yield break;
}
{{/validatable}}
{{/netStandard}}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy