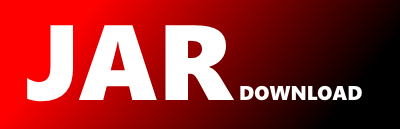
java-micronaut-client.query.QueryParamBinder.mustache Maven / Gradle / Ivy
{{>licenseInfo}}
package {{invokerPackage}}.query;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.beans.BeanProperty;
import io.micronaut.core.beans.BeanWrapper;
import io.micronaut.core.convert.ArgumentConversionContext;
import io.micronaut.core.convert.ConversionContext;
import io.micronaut.core.convert.ConversionService;
import io.micronaut.core.util.StringUtils;
import io.micronaut.http.MutableHttpRequest;
import io.micronaut.http.client.bind.AnnotatedClientArgumentRequestBinder;
import io.micronaut.http.client.bind.ClientRequestUriContext;
import java.util.Collection;
import java.util.Optional;
import jakarta.inject.Singleton;
import javax.annotation.Generated;
{{>generatedAnnotation}}
@Singleton
public class QueryParamBinder implements AnnotatedClientArgumentRequestBinder {
private static final Character COMMA_DELIMITER = ',';
private static final Character PIPE_DELIMITER = '|';
private static final Character SPACE_DELIMITER = ' ';
private final ConversionService> conversionService;
public QueryParamBinder(ConversionService> conversionService) {
this.conversionService = conversionService;
}
@NonNull
@Override
public Class getAnnotationType() {
return QueryParam.class;
}
@Override
public void bind(@NonNull ArgumentConversionContext
© 2015 - 2025 Weber Informatics LLC | Privacy Policy