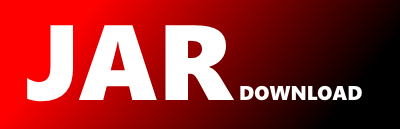
python.endpoint.handlebars Maven / Gradle / Ivy
# coding: utf-8
{{>partial_header}}
from dataclasses import dataclass
import typing_extensions
import urllib3
{{#with operation}}
{{#or headerParams bodyParam produces}}
from urllib3._collections import HTTPHeaderDict
{{/or}}
{{/with}}
from {{packageName}} import api_client, exceptions
{{> model_templates/imports_schema_types }}
{{> model_templates/imports_schemas }}
{{#unless isStub}}
from . import path
{{/unless}}
{{#with operation}}
{{#if queryParams}}
{{> endpoint_parameter_schema_and_def xParams=queryParams xParamsName="Query" }}
{{/if}}
{{#if headerParams}}
{{> endpoint_parameter_schema_and_def xParams=headerParams xParamsName="Header" }}
{{/if}}
{{#if pathParams}}
{{> endpoint_parameter_schema_and_def xParams=pathParams xParamsName="Path" }}
{{/if}}
{{#if cookieParams}}
{{> endpoint_parameter_schema_and_def xParams=cookieParams xParamsName="Cookie" }}
{{/if}}
{{#with bodyParam}}
# body param
{{#each content}}
{{#with this.schema}}
{{> model_templates/schema }}
{{/with}}
{{/each}}
request_body_{{paramName}} = api_client.RequestBody(
content={
{{#each content}}
'{{{@key}}}': api_client.MediaType({{#if this.schema}}
schema={{this.schema.baseName}}{{/if}}),
{{/each}}
},
{{#if required}}
required=True,
{{/if}}
)
{{/with}}
{{#unless isStub}}
{{#each authMethods}}
{{#if @first}}
_auth = [
{{/if}}
'{{name}}',
{{#if @last}}
]
{{/if}}
{{/each}}
{{#each servers}}
{{#if @first}}
_servers = (
{{/if}}
{
'url': "{{{url}}}",
'description': "{{#unless description}}No description provided{{else}}{{{description}}}{{/unless}}",
{{#each variables}}
{{#if @first}}
'variables': {
{{/if}}
'{{{name}}}': {
'description': "{{#unless description}}No description provided{{else}}{{{description}}}{{/unless}}",
'default_value': "{{{defaultValue}}}",
{{#each enumValues}}
{{#if @first}}
'enum_values': [
{{/if}}
"{{{.}}}"{{#unless @last}},{{/unless}}
{{#if @last}}
]
{{/if}}
{{/each}}
}{{#unless @last}},{{/unless}}
{{#if @last}}
}
{{/if}}
{{/each}}
},
{{#if @last}}
)
{{/if}}
{{/each}}
{{/unless}}
{{#each responses}}
{{#each responseHeaders}}
{{#with schema}}
{{> model_templates/schema }}
{{/with}}
{{#unless isStub}}
{{paramName}}_parameter = api_client.HeaderParameter(
name="{{baseName}}",
{{#if style}}
style=api_client.ParameterStyle.{{style}},
{{/if}}
{{#if schema}}
{{#with schema}}
schema={{baseName}},
{{/with}}
{{/if}}
{{#if required}}
required=True,
{{/if}}
{{#if isExplode}}
explode=True,
{{/if}}
)
{{/unless}}
{{/each}}
{{#each content}}
{{#with this.schema}}
{{> model_templates/schema }}
{{/with}}
{{/each}}
{{#if responseHeaders}}
ResponseHeadersFor{{code}} = typing_extensions.TypedDict(
'ResponseHeadersFor{{code}}',
{
{{#each responseHeaders}}
'{{baseName}}': {{#with schema}}{{baseName}},{{/with}}
{{/each}}
}
)
{{/if}}
@dataclass
{{#if isDefault}}
class ApiResponseForDefault(api_client.ApiResponse):
{{else}}
class ApiResponseFor{{code}}(api_client.ApiResponse):
{{/if}}
response: urllib3.HTTPResponse
{{#and responseHeaders content}}
body: typing.Union[
{{#each content}}
{{#if this.schema}}
{{this.schema.baseName}},
{{else}}
schemas.Unset,
{{/if}}
{{/each}}
]
headers: ResponseHeadersFor{{code}}
{{else}}
{{#or responseHeaders content}}
{{#if responseHeaders}}
headers: ResponseHeadersFor{{code}}
body: schemas.Unset = schemas.unset
{{else}}
body: typing.Union[
{{#each content}}
{{#if this.schema}}
{{this.schema.baseName}},
{{else}}
schemas.Unset,
{{/if}}
{{/each}}
]
headers: schemas.Unset = schemas.unset
{{/if}}
{{/or}}
{{/and}}
{{#unless responseHeaders}}
{{#unless content}}
body: schemas.Unset = schemas.unset
headers: schemas.Unset = schemas.unset
{{/unless}}
{{/unless}}
{{#if isDefault}}
_response_for_default = api_client.OpenApiResponse(
response_cls=ApiResponseForDefault,
{{else}}
_response_for_{{code}} = api_client.OpenApiResponse(
response_cls=ApiResponseFor{{code}},
{{/if}}
{{#each content}}
{{#if @first}}
content={
{{/if}}
'{{{@key}}}': api_client.MediaType({{#if this.schema}}
schema={{this.schema.baseName}}{{/if}}),
{{#if @last}}
},
{{/if}}
{{/each}}
{{#if responseHeaders}}
headers=[
{{#each responseHeaders}}
{{paramName}}_parameter,
{{/each}}
]
{{/if}}
)
{{/each}}
{{#unless isStub}}
_status_code_to_response = {
{{#each responses}}
{{#if isDefault}}
'default': _response_for_default,
{{else}}
'{{code}}': _response_for_{{code}},
{{/if}}
{{/each}}
}
{{/unless}}
{{#each produces}}
{{#if @first}}
_all_accept_content_types = (
{{/if}}
'{{{this.mediaType}}}',
{{#if @last}}
)
{{/if}}
{{/each}}
class BaseApi(api_client.Api):
{{#if bodyParam}}
{{#each contentTypeToOperation}}
{{> endpoint_args_baseapi_wrapper contentType=@key this=this}}
{{/each}}
{{> endpoint_args_baseapi_wrapper contentType="null" this=this}}
{{else}}
@typing.overload
def _{{operationId}}_oapg(
{{> endpoint_args isOverload=true skipDeserialization="False" contentType="null"}}
{{/if}}
@typing.overload
def _{{operationId}}_oapg(
{{> endpoint_args isOverload=true skipDeserialization="True" contentType="null"}}
@typing.overload
def _{{operationId}}_oapg(
{{> endpoint_args isOverload=true skipDeserialization="null" contentType="null"}}
def _{{operationId}}_oapg(
{{> endpoint_args isOverload=false skipDeserialization="null" contentType="null"}}
"""
{{#if summary}}
{{summary}}
{{/if}}
:param skip_deserialization: If true then api_response.response will be set but
api_response.body and api_response.headers will not be deserialized into schema
class instances
"""
{{#if queryParams}}
self._verify_typed_dict_inputs_oapg(RequestQueryParams, query_params)
{{/if}}
{{#if headerParams}}
self._verify_typed_dict_inputs_oapg(RequestHeaderParams, header_params)
{{/if}}
{{#if pathParams}}
self._verify_typed_dict_inputs_oapg(RequestPathParams, path_params)
{{/if}}
{{#if cookieParams}}
self._verify_typed_dict_inputs_oapg(RequestCookieParams, cookie_params)
{{/if}}
used_path = path.value
{{#if pathParams}}
_path_params = {}
for parameter in (
{{#each pathParams}}
request_path_{{paramName}},
{{/each}}
):
parameter_data = path_params.get(parameter.name, schemas.unset)
if parameter_data is schemas.unset:
continue
serialized_data = parameter.serialize(parameter_data)
_path_params.update(serialized_data)
for k, v in _path_params.items():
used_path = used_path.replace('{%s}' % k, v)
{{/if}}
{{#if queryParams}}
prefix_separator_iterator = None
for parameter in (
{{#each queryParams}}
request_query_{{paramName}},
{{/each}}
):
parameter_data = query_params.get(parameter.name, schemas.unset)
if parameter_data is schemas.unset:
continue
if prefix_separator_iterator is None:
prefix_separator_iterator = parameter.get_prefix_separator_iterator()
serialized_data = parameter.serialize(parameter_data, prefix_separator_iterator)
for serialized_value in serialized_data.values():
used_path += serialized_value
{{/if}}
{{#or headerParams bodyParam produces}}
_headers = HTTPHeaderDict()
{{else}}
{{/or}}
{{#if headerParams}}
for parameter in (
{{#each headerParams}}
request_header_{{paramName}},
{{/each}}
):
parameter_data = header_params.get(parameter.name, schemas.unset)
if parameter_data is schemas.unset:
continue
serialized_data = parameter.serialize(parameter_data)
_headers.extend(serialized_data)
{{/if}}
# TODO add cookie handling
{{#if produces}}
if accept_content_types:
for accept_content_type in accept_content_types:
_headers.add('Accept', accept_content_type)
{{/if}}
{{#with bodyParam}}
{{#if required}}
if body is schemas.unset:
raise exceptions.ApiValueError(
'The required body parameter has an invalid value of: unset. Set a valid value instead')
{{/if}}
_fields = None
_body = None
{{#if required}}
{{> endpoint_body_serialization }}
{{else}}
if body is not schemas.unset:
{{> endpoint_body_serialization }}
{{/if}}
{{/with}}
{{#if servers}}
host = self._get_host_oapg('{{operationId}}', _servers, host_index)
{{/if}}
response = self.api_client.call_api(
resource_path=used_path,
method='{{httpMethod}}'.upper(),
{{#or headerParams bodyParam produces}}
headers=_headers,
{{/or}}
{{#if bodyParam}}
fields=_fields,
body=_body,
{{/if}}
{{#if hasAuthMethods}}
auth_settings=_auth,
{{/if}}
{{#if servers}}
host=host,
{{/if}}
stream=stream,
timeout=timeout,
)
if skip_deserialization:
api_response = api_client.ApiResponseWithoutDeserialization(response=response)
else:
response_for_status = _status_code_to_response.get(str(response.status))
if response_for_status:
api_response = response_for_status.deserialize(response, self.api_client.configuration)
else:
{{#if hasDefaultResponse}}
default_response = _status_code_to_response.get('default')
if default_response:
api_response = default_response.deserialize(response, self.api_client.configuration)
else:
api_response = api_client.ApiResponseWithoutDeserialization(response=response)
{{else}}
api_response = api_client.ApiResponseWithoutDeserialization(response=response)
{{/if}}
if not 200 <= response.status <= 299:
raise exceptions.ApiException(
status=response.status,
reason=response.reason,
api_response=api_response
)
return api_response
class {{operationIdCamelCase}}(BaseApi):
# this class is used by api classes that refer to endpoints with operationId fn names
{{#if bodyParam}}
{{#each contentTypeToOperation}}
{{> endpoint_args_operationid_wrapper contentType=@key this=this}}
{{/each}}
{{> endpoint_args_operationid_wrapper contentType="null" this=this}}
{{else}}
@typing.overload
def {{operationId}}(
{{> endpoint_args isOverload=true skipDeserialization="False" contentType="null"}}
{{/if}}
@typing.overload
def {{operationId}}(
{{> endpoint_args isOverload=true skipDeserialization="True" contentType="null"}}
@typing.overload
def {{operationId}}(
{{> endpoint_args isOverload=true skipDeserialization="null" contentType="null"}}
def {{operationId}}(
{{> endpoint_args isOverload=false skipDeserialization="null" contentType="null"}}
return self._{{operationId}}_oapg(
{{> endpoint_args_passed }}
)
class ApiFor{{httpMethod}}(BaseApi):
# this class is used by api classes that refer to endpoints by path and http method names
{{#if bodyParam}}
{{#each contentTypeToOperation}}
{{> endpoint_args_httpmethod_wrapper contentType=@key this=this}}
{{/each}}
{{> endpoint_args_httpmethod_wrapper contentType="null" this=this}}
{{else}}
@typing.overload
def {{httpMethod}}(
{{> endpoint_args isOverload=true skipDeserialization="False" contentType="null"}}
{{/if}}
@typing.overload
def {{httpMethod}}(
{{> endpoint_args isOverload=true skipDeserialization="True" contentType="null"}}
@typing.overload
def {{httpMethod}}(
{{> endpoint_args isOverload=true skipDeserialization="null" contentType="null"}}
def {{httpMethod}}(
{{> endpoint_args isOverload=false skipDeserialization="null" contentType="null"}}
return self._{{operationId}}_oapg(
{{> endpoint_args_passed }}
)
{{/with}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy