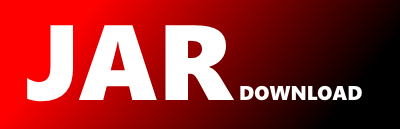
cpp-restbed-server.README.mustache Maven / Gradle / Ivy
# REST API Server for {{appName}}
## Overview
This API Server was generated by the [OpenAPI Generator](https://openapi-generator.tech) project.
It uses the [Restbed](https://github.com/Corvusoft/restbed) Framework.
## Installation
Put the package under your project folder and import the API stubs.
You need to complete the server stub, as it needs to be connected to a source.
## Libraries required
boost_system
ssl (if Restbed was built with SSL Support)
crypto
pthread
restbed
## Namespaces
{{{apiPackage}}}
{{{modelPackage}}}
## Example
The handler functionality can be implemented in two different ways.
Either inherit the given resource and override the handler methods.
Or set a handler lambda to a resource.
This example shows how this can be done with the pet store API.
```
#include "api/StoreApi.h"
#include "api/UserApi.h"
using namespace org::openapitools::server::api;
using namespace org::openapitools::server::api::StoreApiResources;
using namespace org::openapitools::server::api::UserApiResources;
/* 1. variant: inherit from the resource and override handler method */
class MyStoreApiStoreOrderResource : public StoreOrderResource {
public:
std::pair
handler_POST(Order &order) override {
auto ret = Order();
/* ... add your implementation here .... */
return std::make_pair(200, ret);
}
};
int main() {
const auto service = std::make_shared();
auto storeApi = StoreApi(service);
storeApi.setResource(std::make_shared());
auto userApi = UserApi(service);
/* 2. variant: implement handler as lambda */
userApi.getUserResource()->handler_POST_func = [](auto& user) {
/* ... add your implementation here .... */
return 200;};
const auto settings = std::make_shared();
settings->set_port(1236);
service->start(settings);
}
```
© 2015 - 2025 Weber Informatics LLC | Privacy Policy