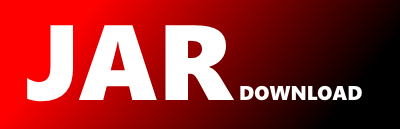
csharp.Multimap.mustache Maven / Gradle / Ivy
{{>partial_header}}
using System;
using System.Collections;
using System.Collections.Generic;
namespace {{packageName}}.Client
{
///
/// A dictionary in which one key has many associated values.
///
/// The type of the key
/// The type of the value associated with the key.
public class Multimap : IDictionary>
{
#region Private Fields
private readonly Dictionary> _dictionary;
#endregion Private Fields
#region Constructors
///
/// Empty Constructor.
///
public Multimap()
{
_dictionary = new Dictionary>();
}
///
/// Constructor with comparer.
///
///
public Multimap(IEqualityComparer comparer)
{
_dictionary = new Dictionary>(comparer);
}
#endregion Constructors
#region Enumerators
///
/// To get the enumerator.
///
/// Enumerator
public IEnumerator>> GetEnumerator()
{
return _dictionary.GetEnumerator();
}
///
/// To get the enumerator.
///
/// Enumerator
IEnumerator IEnumerable.GetEnumerator()
{
return _dictionary.GetEnumerator();
}
#endregion Enumerators
#region Public Members
///
/// Add values to Multimap
///
/// Key value pair
public void Add(KeyValuePair> item)
{
if (!TryAdd(item.Key, item.Value))
throw new InvalidOperationException("Could not add values to Multimap.");
}
///
/// Add Multimap to Multimap
///
/// Multimap
public void Add(Multimap multimap)
{
foreach (var item in multimap)
{
if (!TryAdd(item.Key, item.Value))
throw new InvalidOperationException("Could not add values to Multimap.");
}
}
///
/// Clear Multimap
///
public void Clear()
{
_dictionary.Clear();
}
///
/// Determines whether Multimap contains the specified item.
///
/// Key value pair
/// Method needs to be implemented
/// true if the Multimap contains the item; otherwise, false.
public bool Contains(KeyValuePair> item)
{
throw new NotImplementedException();
}
///
/// Copy items of the Multimap to an array,
/// starting at a particular array index.
///
/// The array that is the destination of the items copied
/// from Multimap. The array must have zero-based indexing.
/// The zero-based index in array at which copying begins.
/// Method needs to be implemented
public void CopyTo(KeyValuePair>[] array, int arrayIndex)
{
throw new NotImplementedException();
}
///
/// Removes the specified item from the Multimap.
///
/// Key value pair
/// true if the item is successfully removed; otherwise, false.
/// Method needs to be implemented
public bool Remove(KeyValuePair> item)
{
throw new NotImplementedException();
}
///
/// Gets the number of items contained in the Multimap.
///
public int Count => _dictionary.Count;
///
/// Gets a value indicating whether the Multimap is read-only.
///
public bool IsReadOnly => false;
///
/// Adds an item with the provided key and value to the Multimap.
///
/// The object to use as the key of the item to add.
/// The object to use as the value of the item to add.
/// Thrown when couldn't add the value to Multimap.
public void Add(TKey key, IList value)
{
if (value != null && value.Count > 0)
{
if (_dictionary.TryGetValue(key, out var list))
{
foreach (var k in value) list.Add(k);
}
else
{
list = new List(value);
if (!TryAdd(key, list))
throw new InvalidOperationException("Could not add values to Multimap.");
}
}
}
///
/// Determines whether the Multimap contains an item with the specified key.
///
/// The key to locate in the Multimap.
/// true if the Multimap contains an item with
/// the key; otherwise, false.
public bool ContainsKey(TKey key)
{
return _dictionary.ContainsKey(key);
}
///
/// Removes item with the specified key from the Multimap.
///
/// The key to locate in the Multimap.
/// true if the item is successfully removed; otherwise, false.
public bool Remove(TKey key)
{
return TryRemove(key, out var _);
}
///
/// Gets the value associated with the specified key.
///
/// The key whose value to get.
/// When this method returns, the value associated with the specified key, if the
/// key is found; otherwise, the default value for the type of the value parameter.
/// This parameter is passed uninitialized.
/// true if the object that implements Multimap contains
/// an item with the specified key; otherwise, false.
public bool TryGetValue(TKey key, out IList value)
{
return _dictionary.TryGetValue(key, out value);
}
///
/// Gets or sets the item with the specified key.
///
/// The key of the item to get or set.
/// The value of the specified key.
public IList this[TKey key]
{
get => _dictionary[key];
set => _dictionary[key] = value;
}
///
/// Gets a System.Collections.Generic.ICollection containing the keys of the Multimap.
///
public ICollection Keys => _dictionary.Keys;
///
/// Gets a System.Collections.Generic.ICollection containing the values of the Multimap.
///
public ICollection> Values => _dictionary.Values;
///
/// Copy the items of the Multimap to an System.Array,
/// starting at a particular System.Array index.
///
/// The one-dimensional System.Array that is the destination of the items copied
/// from Multimap. The System.Array must have zero-based indexing.
/// The zero-based index in array at which copying begins.
public void CopyTo(Array array, int index)
{
((ICollection)_dictionary).CopyTo(array, index);
}
///
/// Adds an item with the provided key and value to the Multimap.
///
/// The object to use as the key of the item to add.
/// The object to use as the value of the item to add.
/// Thrown when couldn't add value to Multimap.
public void Add(TKey key, TValue value)
{
if (value != null)
{
if (_dictionary.TryGetValue(key, out var list))
{
list.Add(value);
}
else
{
list = new List { value };
if (!TryAdd(key, list))
throw new InvalidOperationException("Could not add value to Multimap.");
}
}
}
#endregion Public Members
#region Private Members
/**
* Helper method to encapsulate generator differences between dictionary types.
*/
private bool TryRemove(TKey key, out IList value)
{
_dictionary.TryGetValue(key, out value);
return _dictionary.Remove(key);
}
/**
* Helper method to encapsulate generator differences between dictionary types.
*/
private bool TryAdd(TKey key, IList value)
{
try
{
_dictionary.Add(key, value);
}
catch (ArgumentException)
{
return false;
}
return true;
}
#endregion Private Members
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy