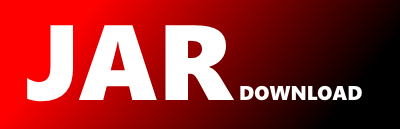
csharp.libraries.generichost.api.mustache Maven / Gradle / Ivy
The newest version!
{{#lambda.trimLineBreaks}}
//
{{>partial_header}}
{{#nrt}}
#nullable enable
{{/nrt}}
using System;
using System.Collections.Generic;
{{#net80OrLater}}
{{#lambda.uniqueLines}}
{{#operations}}
{{#operation}}
{{#vendorExtensions.x-set-cookie}}
using System.Linq;
{{/vendorExtensions.x-set-cookie}}
{{/operation}}
{{/operations}}
{{/lambda.uniqueLines}}
{{/net80OrLater}}
using System.Net;
using System.Threading.Tasks;
using Microsoft.Extensions.Logging;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text.Json;
using {{packageName}}.{{clientPackage}};
{{#hasImport}}
using {{packageName}}.{{modelPackage}};
{{/hasImport}}
{{^netStandard}}
using System.Diagnostics.CodeAnalysis;
{{/netStandard}}
namespace {{packageName}}.{{apiPackage}}
{
{{#operations}}
///
/// Represents a collection of functions to interact with the API endpoints
/// This class is registered as transient.
///
{{>visibility}} interface {{interfacePrefix}}{{classname}} : IApi
{
///
/// The class containing the events
///
{{classname}}Events Events { get; }
{{#operation}}
///
/// {{summary}}
///
///
/// {{notes}}
///
/// Thrown when fails to make API call
{{#allParams}}
/// {{description}}{{^required}} (optional{{#defaultValue}}, default to {{.}}{{/defaultValue}}){{/required}}{{#isDeprecated}} (deprecated){{/isDeprecated}}
{{/allParams}}
/// Cancellation Token to cancel the request.
/// < >
{{#isDeprecated}}
[Obsolete]
{{/isDeprecated}}
Task<{{interfacePrefix}}{{operationId}}ApiResponse> {{operationId}}Async({{>OperationSignature}});
///
/// {{summary}}
///
///
/// {{notes}}
///
{{#allParams}}
/// {{description}}{{^required}} (optional{{#defaultValue}}, default to {{.}}{{/defaultValue}}){{/required}}{{#isDeprecated}} (deprecated){{/isDeprecated}}
{{/allParams}}
/// Cancellation Token to cancel the request.
/// < {{nrt?}}>
{{#isDeprecated}}
[Obsolete]
{{/isDeprecated}}
Task<{{interfacePrefix}}{{operationId}}ApiResponse{{nrt?}}> {{operationId}}OrDefaultAsync({{>OperationSignature}});
{{^-last}}
{{/-last}}
{{/operation}}
}
{{#operation}}
{{^vendorExtensions.x-duplicates}}
{{#responses}}
{{#-first}}
///
/// The
///
{{>visibility}} interface {{interfacePrefix}}{{operationId}}ApiResponse : {{#lambda.joinWithComma}}{{packageName}}.{{clientPackage}}.{{interfacePrefix}}ApiResponse {{#responses}}{{#dataType}}{{interfacePrefix}}{{vendorExtensions.x-http-status}}<{{#isModel}}{{^containerType}}{{packageName}}.{{modelPackage}}.{{/containerType}}{{/isModel}}{{{dataType}}}{{#nrt}}?{{/nrt}}{{^nrt}}{{#vendorExtensions.x-is-value-type}}?{{/vendorExtensions.x-is-value-type}}{{/nrt}}> {{/dataType}}{{/responses}}{{/lambda.joinWithComma}}
{
{{#responses}}
{{#vendorExtensions.x-http-status-is-default}}
///
/// Returns true if the response is the default response type
///
///
bool Is{{vendorExtensions.x-http-status}} { get; }
{{/vendorExtensions.x-http-status-is-default}}
{{^vendorExtensions.x-http-status-is-default}}
///
/// Returns true if the response is {{code}} {{vendorExtensions.x-http-status}}
///
///
bool Is{{vendorExtensions.x-http-status}} { get; }
{{/vendorExtensions.x-http-status-is-default}}
{{^-last}}
{{/-last}}
{{/responses}}
}
{{/-first}}
{{/responses}}
{{/vendorExtensions.x-duplicates}}
{{/operation}}
///
/// Represents a collection of functions to interact with the API endpoints
///
{{>visibility}} class {{classname}}Events
{
{{#lambda.trimTrailingWithNewLine}}
{{#operation}}
///
/// The event raised after the server response
///
public event EventHandler{{nrt?}} On{{operationId}};
///
/// The event raised after an error querying the server
///
public event EventHandler{{nrt?}} OnError{{operationId}};
internal void ExecuteOn{{operationId}}({{#vendorExtensions.x-duplicates}}{{.}}{{/vendorExtensions.x-duplicates}}{{^vendorExtensions.x-duplicates}}{{classname}}{{/vendorExtensions.x-duplicates}}.{{operationId}}ApiResponse apiResponse)
{
On{{operationId}}?.Invoke(this, new ApiResponseEventArgs(apiResponse));
}
internal void ExecuteOnError{{operationId}}(Exception exception)
{
OnError{{operationId}}?.Invoke(this, new ExceptionEventArgs(exception));
}
{{/operation}}
{{/lambda.trimTrailingWithNewLine}}
}
///
/// Represents a collection of functions to interact with the API endpoints
///
{{>visibility}} sealed partial class {{classname}} : {{interfacePrefix}}{{classname}}
{
private JsonSerializerOptions _jsonSerializerOptions;
///
/// The logger factory
///
public ILoggerFactory LoggerFactory { get; }
///
/// The logger
///
public ILogger<{{classname}}> Logger { get; }
///
/// The HttpClient
///
public HttpClient HttpClient { get; }
///
/// The class containing the events
///
public {{classname}}Events Events { get; }{{#hasApiKeyMethods}}
///
/// A token provider of type
///
public TokenProvider ApiKeyProvider { get; }{{/hasApiKeyMethods}}{{#hasHttpBearerMethods}}
///
/// A token provider of type
///
public TokenProvider BearerTokenProvider { get; }{{/hasHttpBearerMethods}}{{#hasHttpBasicMethods}}
///
/// A token provider of type
///
public TokenProvider BasicTokenProvider { get; }{{/hasHttpBasicMethods}}{{#hasHttpSignatureMethods}}
///
/// A token provider of type
///
public TokenProvider HttpSignatureTokenProvider { get; }{{/hasHttpSignatureMethods}}{{#hasOAuthMethods}}
///
/// A token provider of type
///
public TokenProvider OauthTokenProvider { get; }{{/hasOAuthMethods}}
{{#net80OrLater}}
{{#lambda.unique}}
{{#operation}}
{{#vendorExtensions.x-set-cookie}}
///
/// The token cookie container
///
public {{packageName}}.{{clientPackage}}.CookieContainer CookieContainer { get; }
{{/vendorExtensions.x-set-cookie}}
{{/operation}}
{{/lambda.unique}}
{{/net80OrLater}}
///
/// Initializes a new instance of the class.
///
///
public {{classname}}(ILogger<{{classname}}> logger, ILoggerFactory loggerFactory, HttpClient httpClient, JsonSerializerOptionsProvider jsonSerializerOptionsProvider, {{classname}}Events {{#lambda.camelcase_sanitize_param}}{{classname}}Events{{/lambda.camelcase_sanitize_param}}{{#hasApiKeyMethods}},
TokenProvider apiKeyProvider{{/hasApiKeyMethods}}{{#hasHttpBearerMethods}},
TokenProvider bearerTokenProvider{{/hasHttpBearerMethods}}{{#hasHttpBasicMethods}},
TokenProvider basicTokenProvider{{/hasHttpBasicMethods}}{{#hasHttpSignatureMethods}},
TokenProvider httpSignatureTokenProvider{{/hasHttpSignatureMethods}}{{#hasOAuthMethods}},
TokenProvider oauthTokenProvider{{/hasOAuthMethods}}{{#net80OrLater}}{{#operation}}{{#lambda.uniqueLines}}{{#vendorExtensions.x-set-cookie}},
{{packageName}}.{{clientPackage}}.CookieContainer cookieContainer{{/vendorExtensions.x-set-cookie}}{{/lambda.uniqueLines}}{{/operation}}{{/net80OrLater}})
{
_jsonSerializerOptions = jsonSerializerOptionsProvider.Options;
LoggerFactory = loggerFactory;
Logger = LoggerFactory.CreateLogger<{{classname}}>();
HttpClient = httpClient;
Events = {{#lambda.camelcase_sanitize_param}}{{classname}}Events{{/lambda.camelcase_sanitize_param}};{{#hasApiKeyMethods}}
ApiKeyProvider = apiKeyProvider;{{/hasApiKeyMethods}}{{#hasHttpBearerMethods}}
BearerTokenProvider = bearerTokenProvider;{{/hasHttpBearerMethods}}{{#hasHttpBasicMethods}}
BasicTokenProvider = basicTokenProvider;{{/hasHttpBasicMethods}}{{#hasHttpSignatureMethods}}
HttpSignatureTokenProvider = httpSignatureTokenProvider;{{/hasHttpSignatureMethods}}{{#hasOAuthMethods}}
OauthTokenProvider = oauthTokenProvider;{{/hasOAuthMethods}}{{#net80OrLater}}{{#operation}}{{#lambda.uniqueLines}}{{#vendorExtensions.x-set-cookie}}
CookieContainer = cookieContainer;{{/vendorExtensions.x-set-cookie}}{{/lambda.uniqueLines}}{{/operation}}{{/net80OrLater}}
}
{{#operation}}
{{#allParams}}
{{#-first}}
partial void Format{{operationId}}({{#allParams}}{{#isPrimitiveType}}ref {{/isPrimitiveType}}{{^required}}Option<{{/required}}{{{dataType}}}{{>NullConditionalParameter}}{{^required}}>{{/required}} {{paramName}}{{^-last}}, {{/-last}}{{/allParams}});
{{/-first}}
{{/allParams}}
{{#vendorExtensions.x-has-not-nullable-reference-types}}
///
/// Validates the request parameters
///
{{#vendorExtensions.x-not-nullable-reference-types}}
///
{{/vendorExtensions.x-not-nullable-reference-types}}
///
private void Validate{{operationId}}({{#vendorExtensions.x-not-nullable-reference-types}}{{^required}}Option<{{/required}}{{{dataType}}}{{>NullConditionalParameter}}{{^required}}>{{/required}} {{paramName}}{{^-last}}, {{/-last}}{{/vendorExtensions.x-not-nullable-reference-types}})
{
{{#lambda.trimTrailingWithNewLine}}
{{#vendorExtensions.x-not-nullable-reference-types}}
{{#required}}
{{^vendorExtensions.x-is-value-type}}
if ({{paramName}} == null)
throw new ArgumentNullException(nameof({{paramName}}));
{{/vendorExtensions.x-is-value-type}}
{{/required}}
{{^required}}
{{^vendorExtensions.x-is-value-type}}
if ({{paramName}}.IsSet && {{paramName}}.Value == null)
throw new ArgumentNullException(nameof({{paramName}}));
{{/vendorExtensions.x-is-value-type}}
{{/required}}
{{/vendorExtensions.x-not-nullable-reference-types}}
{{/lambda.trimTrailingWithNewLine}}
}
{{/vendorExtensions.x-has-not-nullable-reference-types}}
///
/// Processes the server response
///
///
{{#allParams}}
///
{{/allParams}}
private void After{{operationId}}DefaultImplementation({{#lambda.joinWithComma}}{{interfacePrefix}}{{operationId}}ApiResponse apiResponseLocalVar {{#allParams}}{{^required}}Option<{{/required}}{{{dataType}}}{{>NullConditionalParameter}}{{^required}}>{{/required}} {{paramName}} {{/allParams}}{{/lambda.joinWithComma}})
{
bool suppressDefaultLog = false;
After{{operationId}}({{#lambda.joinWithComma}}ref suppressDefaultLog apiResponseLocalVar {{#allParams}}{{paramName}} {{/allParams}}{{/lambda.joinWithComma}});
{{>AfterOperationDefaultImplementation}}
}
///
/// Processes the server response
///
///
///
{{#allParams}}
///
{{/allParams}}
partial void After{{operationId}}({{#lambda.joinWithComma}}ref bool suppressDefaultLog {{interfacePrefix}}{{operationId}}ApiResponse apiResponseLocalVar {{#allParams}}{{^required}}Option<{{/required}}{{{dataType}}}{{>NullConditionalParameter}}{{^required}}>{{/required}} {{paramName}} {{/allParams}}{{/lambda.joinWithComma}});
///
/// Logs exceptions that occur while retrieving the server response
///
///
///
///
{{#allParams}}
///
{{/allParams}}
private void OnError{{operationId}}DefaultImplementation({{#lambda.joinWithComma}}Exception exceptionLocalVar string pathFormatLocalVar string pathLocalVar {{#allParams}}{{^required}}Option<{{/required}}{{{dataType}}}{{>NullConditionalParameter}}{{^required}}>{{/required}} {{paramName}} {{/allParams}}{{/lambda.joinWithComma}})
{
bool suppressDefaultLogLocalVar = false;
OnError{{operationId}}({{#lambda.joinWithComma}}ref suppressDefaultLogLocalVar exceptionLocalVar pathFormatLocalVar pathLocalVar {{#allParams}}{{paramName}} {{/allParams}}{{/lambda.joinWithComma}});
{{>OnErrorDefaultImplementation}}
}
///
/// A partial method that gives developers a way to provide customized exception handling
///
///
///
///
///
{{#allParams}}
///
{{/allParams}}
partial void OnError{{operationId}}({{#lambda.joinWithComma}}ref bool suppressDefaultLogLocalVar Exception exceptionLocalVar string pathFormatLocalVar string pathLocalVar {{#allParams}}{{^required}}Option<{{/required}}{{{dataType}}}{{>NullConditionalParameter}}{{^required}}>{{/required}} {{paramName}} {{/allParams}}{{/lambda.joinWithComma}});
///
/// {{summary}} {{notes}}
///
{{#allParams}}
/// {{description}}{{^required}} (optional{{#defaultValue}}, default to {{.}}{{/defaultValue}}){{/required}}
{{/allParams}}
/// Cancellation Token to cancel the request.
/// < >
public async Task<{{interfacePrefix}}{{operationId}}ApiResponse{{nrt?}}> {{operationId}}OrDefaultAsync({{>OperationSignature}})
{
try
{
return await {{operationId}}Async({{#allParams}}{{paramName}}{{^-last}}, {{/-last}}{{/allParams}}{{#allParams.0}}, {{/allParams.0}}cancellationToken).ConfigureAwait(false);
}
catch (Exception)
{
return null;
}
}
///
/// {{summary}} {{notes}}
///
/// Thrown when fails to make API call
{{#allParams}}
/// {{description}}{{^required}} (optional{{#defaultValue}}, default to {{.}}{{/defaultValue}}){{/required}}
{{/allParams}}
/// Cancellation Token to cancel the request.
/// < >
public async Task<{{interfacePrefix}}{{operationId}}ApiResponse> {{operationId}}Async({{>OperationSignature}})
{
{{#lambda.trimLineBreaks}}
UriBuilder uriBuilderLocalVar = new UriBuilder();
try
{
{{#vendorExtensions.x-has-not-nullable-reference-types}}
Validate{{operationId}}({{#vendorExtensions.x-not-nullable-reference-types}}{{paramName}}{{^-last}}, {{/-last}}{{/vendorExtensions.x-not-nullable-reference-types}});
{{/vendorExtensions.x-has-not-nullable-reference-types}}
{{#allParams}}
{{#-first}}
Format{{operationId}}({{#allParams}}{{#isPrimitiveType}}ref {{/isPrimitiveType}}{{paramName}}{{^-last}}, {{/-last}}{{/allParams}});
{{/-first}}
{{/allParams}}
using (HttpRequestMessage httpRequestMessageLocalVar = new HttpRequestMessage())
{
{{^servers}}
uriBuilderLocalVar.Host = HttpClient.BaseAddress{{nrt!}}.Host;
uriBuilderLocalVar.Port = HttpClient.BaseAddress.Port;
uriBuilderLocalVar.Scheme = HttpClient.BaseAddress.Scheme;
uriBuilderLocalVar.Path = ClientUtils.CONTEXT_PATH + "{{{path}}}";
{{/servers}}
{{#servers}}
{{#-first}}
Uri urlLocalVar = httpRequestMessageLocalVar.RequestUri = new Uri("{{url}}");
uriBuilderLocalVar.Host = urlLocalVar.Authority;
uriBuilderLocalVar.Scheme = urlLocalVar.Scheme;
uriBuilderLocalVar.Path = urlLocalVar.AbsolutePath;
{{/-first}}
{{/servers}}
{{#constantParams}}
{{#isPathParam}}
// Set client side default value of Path Param "{{baseName}}".
uriBuilderLocalVar.Path = uriBuilderLocalVar.Path.Replace("%7B{{baseName}}%7D", Uri.EscapeDataString(ClientUtils.ParameterToString({{#_enum}}"{{{.}}}"{{/_enum}}))); // Constant path parameter
{{/isPathParam}}
{{/constantParams}}
{{#pathParams}}
uriBuilderLocalVar.Path = uriBuilderLocalVar.Path.Replace("%7B{{baseName}}%7D", Uri.EscapeDataString({{paramName}}.ToString()));
{{#-last}}
{{/-last}}
{{/pathParams}}
{{#queryParams}}
{{#-first}}
System.Collections.Specialized.NameValueCollection parseQueryStringLocalVar = System.Web.HttpUtility.ParseQueryString(string.Empty);
{{/-first}}
{{/queryParams}}
{{^queryParams}}
{{#authMethods}}
{{#isApiKey}}
{{#isKeyInQuery}}
System.Collections.Specialized.NameValueCollection parseQueryStringLocalVar = System.Web.HttpUtility.ParseQueryString(string.Empty);
{{/isKeyInQuery}}
{{/isApiKey}}
{{/authMethods}}
{{/queryParams}}
{{#queryParams}}
{{#required}}
{{#-first}}
{{/-first}}
parseQueryStringLocalVar["{{baseName}}"] = ClientUtils.ParameterToString({{paramName}});
{{/required}}
{{/queryParams}}
{{#constantParams}}
{{#isQueryParam}}
// Set client side default value of Query Param "{{baseName}}".
parseQueryStringLocalVar["{{baseName}}"] = ClientUtils.ParameterToString({{#_enum}}"{{{.}}}"{{/_enum}}); // Constant query parameter
{{/isQueryParam}}
{{/constantParams}}
{{#queryParams}}
{{^required}}
if ({{paramName}}.IsSet)
parseQueryStringLocalVar["{{baseName}}"] = ClientUtils.ParameterToString({{paramName}}.Value);
{{/required}}
{{#-last}}
uriBuilderLocalVar.Query = parseQueryStringLocalVar.ToString();
{{/-last}}
{{/queryParams}}
{{#constantParams}}
{{#isHeaderParam}}
// Set client side default value of Header Param "{{baseName}}".
httpRequestMessageLocalVar.Headers.Add("{{baseName}}", ClientUtils.ParameterToString({{#_enum}}"{{{.}}}"{{/_enum}})); // Constant header parameter
{{/isHeaderParam}}
{{/constantParams}}
{{#headerParams}}
{{#required}}
httpRequestMessageLocalVar.Headers.Add("{{baseName}}", ClientUtils.ParameterToString({{paramName}}));
{{/required}}
{{^required}}
if ({{paramName}}.IsSet)
httpRequestMessageLocalVar.Headers.Add("{{baseName}}", ClientUtils.ParameterToString({{paramName}}.Value));
{{/required}}
{{/headerParams}}
{{#formParams}}
{{#-first}}
MultipartContent multipartContentLocalVar = new MultipartContent();
httpRequestMessageLocalVar.Content = multipartContentLocalVar;
List> formParameterLocalVars = new List>();
multipartContentLocalVar.Add(new FormUrlEncodedContent(formParameterLocalVars));{{/-first}}{{^isFile}}{{#required}}
formParameterLocalVars.Add(new KeyValuePair("{{baseName}}", ClientUtils.ParameterToString({{paramName}})));
{{/required}}
{{^required}}
if ({{paramName}}.IsSet)
formParameterLocalVars.Add(new KeyValuePair("{{baseName}}", ClientUtils.ParameterToString({{paramName}}.Value)));
{{/required}}
{{/isFile}}
{{#isFile}}
{{#required}}
multipartContentLocalVar.Add(new StreamContent({{paramName}}));
{{/required}}
{{^required}}
if ({{paramName}}.IsSet)
multipartContentLocalVar.Add(new StreamContent({{paramName}}.Value));
{{/required}}
{{/isFile}}
{{/formParams}}
{{#bodyParam}}
{{#required}}
httpRequestMessageLocalVar.Content = ({{paramName}}{{^required}}.Value{{/required}} as object) is System.IO.Stream stream
? httpRequestMessageLocalVar.Content = new StreamContent(stream)
: httpRequestMessageLocalVar.Content = new StringContent(JsonSerializer.Serialize({{paramName}}{{^required}}.Value{{/required}}, _jsonSerializerOptions));
{{/required}}
{{^required}}
if ({{paramName}}.IsSet)
httpRequestMessageLocalVar.Content = ({{paramName}}{{^required}}.Value{{/required}} as object) is System.IO.Stream stream
? httpRequestMessageLocalVar.Content = new StreamContent(stream)
: httpRequestMessageLocalVar.Content = new StringContent(JsonSerializer.Serialize({{paramName}}{{^required}}.Value{{/required}}, _jsonSerializerOptions));
{{/required}}
{{/bodyParam}}
{{#authMethods}}
{{#-first}}
List tokenBaseLocalVars = new List();
{{/-first}}
{{#isApiKey}}
{{^isKeyInCookie}}
ApiKeyToken apiKeyTokenLocalVar{{-index}} = (ApiKeyToken) await ApiKeyProvider.GetAsync("{{keyParamName}}", cancellationToken).ConfigureAwait(false);
tokenBaseLocalVars.Add(apiKeyTokenLocalVar{{-index}});
{{#isKeyInHeader}}
apiKeyTokenLocalVar{{-index}}.UseInHeader(httpRequestMessageLocalVar);
{{/isKeyInHeader}}
{{/isKeyInCookie}}
{{#isKeyInQuery}}
apiKeyTokenLocalVar{{-index}}.UseInQuery(httpRequestMessageLocalVar, uriBuilderLocalVar, parseQueryStringLocalVar);
uriBuilderLocalVar.Query = parseQueryStringLocalVar.ToString();
{{/isKeyInQuery}}
{{/isApiKey}}
{{/authMethods}}
httpRequestMessageLocalVar.RequestUri = uriBuilderLocalVar.Uri;
{{#authMethods}}
{{#isBasicBasic}}
BasicToken basicTokenLocalVar{{-index}} = (BasicToken) await BasicTokenProvider.GetAsync(cancellation: cancellationToken).ConfigureAwait(false);
tokenBaseLocalVars.Add(basicTokenLocalVar{{-index}});
basicTokenLocalVar{{-index}}.UseInHeader(httpRequestMessageLocalVar, "{{keyParamName}}");
{{/isBasicBasic}}
{{#isBasicBearer}}
BearerToken bearerTokenLocalVar{{-index}} = (BearerToken) await BearerTokenProvider.GetAsync(cancellation: cancellationToken).ConfigureAwait(false);
tokenBaseLocalVars.Add(bearerTokenLocalVar{{-index}});
bearerTokenLocalVar{{-index}}.UseInHeader(httpRequestMessageLocalVar, "{{keyParamName}}");
{{/isBasicBearer}}
{{#isOAuth}}
OAuthToken oauthTokenLocalVar{{-index}} = (OAuthToken) await OauthTokenProvider.GetAsync(cancellation: cancellationToken).ConfigureAwait(false);
tokenBaseLocalVars.Add(oauthTokenLocalVar{{-index}});
oauthTokenLocalVar{{-index}}.UseInHeader(httpRequestMessageLocalVar, "{{keyParamName}}");
{{/isOAuth}}
{{#isHttpSignature}}
HttpSignatureToken httpSignatureTokenLocalVar{{-index}} = (HttpSignatureToken) await HttpSignatureTokenProvider.GetAsync(cancellation: cancellationToken).ConfigureAwait(false);
tokenBaseLocalVars.Add(httpSignatureTokenLocalVar{{-index}});
if (httpRequestMessageLocalVar.Content != null) {
string requestBodyLocalVar = await httpRequestMessageLocalVar.Content.ReadAsStringAsync({{#net60OrLater}}cancellationToken{{/net60OrLater}}).ConfigureAwait(false);
httpSignatureTokenLocalVar{{-index}}.UseInHeader(httpRequestMessageLocalVar, requestBodyLocalVar, cancellationToken);
}
{{/isHttpSignature}}
{{/authMethods}}
{{#consumes}}
{{#-first}}
{{=<% %>=}}
string[] contentTypes = new string[] {<%/-first%>
<%={{ }}=%>
"{{{mediaType}}}"{{^-last}},{{/-last}}{{#-last}}
};
{{/-last}}
{{/consumes}}
{{#consumes}}
{{#-first}}
string{{nrt?}} contentTypeLocalVar = ClientUtils.SelectHeaderContentType(contentTypes);
if (contentTypeLocalVar != null && httpRequestMessageLocalVar.Content != null)
httpRequestMessageLocalVar.Content.Headers.ContentType = new MediaTypeHeaderValue(contentTypeLocalVar);
{{/-first}}
{{/consumes}}
{{#produces}}
{{#-first}}
{{=<% %>=}}
string[] acceptLocalVars = new string[] {<%/-first%>
<%={{ }}=%>
"{{{mediaType}}}"{{^-last}},{{/-last}}{{#-last}}
};
{{/-last}}
{{/produces}}
{{#produces}}
{{#-first}}
string{{nrt?}} acceptLocalVar = ClientUtils.SelectHeaderAccept(acceptLocalVars);
if (acceptLocalVar != null)
httpRequestMessageLocalVar.Headers.Accept.Add(new MediaTypeWithQualityHeaderValue(acceptLocalVar));
{{/-first}}
{{/produces}}
{{#net60OrLater}}
httpRequestMessageLocalVar.Method = HttpMethod.{{#lambda.titlecase}}{{#lambda.lowercase}}{{httpMethod}}{{/lambda.lowercase}}{{/lambda.titlecase}};
{{/net60OrLater}}
{{^net60OrLater}}
httpRequestMessageLocalVar.Method = new HttpMethod("{{#lambda.uppercase}}{{httpMethod}}{{/lambda.uppercase}}");
{{/net60OrLater}}
DateTime requestedAtLocalVar = DateTime.UtcNow;
using (HttpResponseMessage httpResponseMessageLocalVar = await HttpClient.SendAsync(httpRequestMessageLocalVar, cancellationToken).ConfigureAwait(false))
{
string responseContentLocalVar = await httpResponseMessageLocalVar.Content.ReadAsStringAsync({{#net60OrLater}}cancellationToken{{/net60OrLater}}).ConfigureAwait(false);
ILogger<{{#vendorExtensions.x-duplicates}}{{.}}.{{/vendorExtensions.x-duplicates}}{{operationId}}ApiResponse> apiResponseLoggerLocalVar = LoggerFactory.CreateLogger<{{#vendorExtensions.x-duplicates}}{{.}}.{{/vendorExtensions.x-duplicates}}{{operationId}}ApiResponse>();
{{#vendorExtensions.x-duplicates}}{{.}}.{{/vendorExtensions.x-duplicates}}{{operationId}}ApiResponse apiResponseLocalVar = new{{^net60OrLater}} {{operationId}}ApiResponse{{/net60OrLater}}(apiResponseLoggerLocalVar, httpRequestMessageLocalVar, httpResponseMessageLocalVar, responseContentLocalVar, "{{{path}}}", requestedAtLocalVar, _jsonSerializerOptions);
After{{operationId}}DefaultImplementation({{#lambda.joinWithComma}}apiResponseLocalVar {{#allParams}}{{paramName}} {{/allParams}}{{/lambda.joinWithComma}});
Events.ExecuteOn{{operationId}}(apiResponseLocalVar);
{{#authMethods}}
{{#-first}}
if (apiResponseLocalVar.StatusCode == (HttpStatusCode) 429)
foreach(TokenBase tokenBaseLocalVar in tokenBaseLocalVars)
tokenBaseLocalVar.BeginRateLimit();
{{/-first}}
{{/authMethods}}
{{#net80OrLater}}
{{#responses}}
{{#vendorExtensions.x-set-cookie}}
if (httpResponseMessageLocalVar.StatusCode == (HttpStatusCode) {{code}} && httpResponseMessageLocalVar.Headers.TryGetValues("Set-Cookie", out var cookieHeadersLocalVar))
{
foreach(string cookieHeader in cookieHeadersLocalVar)
{
IList setCookieHeaderValuesLocalVar = Microsoft.Net.Http.Headers.SetCookieHeaderValue.ParseList(cookieHeadersLocalVar.ToArray());
foreach(Microsoft.Net.Http.Headers.SetCookieHeaderValue setCookieHeaderValueLocalVar in setCookieHeaderValuesLocalVar)
{
Cookie cookieLocalVar = new Cookie(setCookieHeaderValueLocalVar.Name.ToString(), setCookieHeaderValueLocalVar.Value.ToString())
{
HttpOnly = setCookieHeaderValueLocalVar.HttpOnly
};
if (setCookieHeaderValueLocalVar.Expires.HasValue)
cookieLocalVar.Expires = setCookieHeaderValueLocalVar.Expires.Value.UtcDateTime;
if (setCookieHeaderValueLocalVar.Path.HasValue)
cookieLocalVar.Path = setCookieHeaderValueLocalVar.Path.Value;
if (setCookieHeaderValueLocalVar.Domain.HasValue)
cookieLocalVar.Domain = setCookieHeaderValueLocalVar.Domain.Value;
CookieContainer.Value.Add(new Uri($"{uriBuilderLocalVar.Scheme}://{uriBuilderLocalVar.Host}"), cookieLocalVar);
}
}
}
{{/vendorExtensions.x-set-cookie}}
{{/responses}}
{{/net80OrLater}}
return apiResponseLocalVar;
}
}
}
catch(Exception e)
{
OnError{{operationId}}DefaultImplementation({{#lambda.joinWithComma}}e "{{{path}}}" uriBuilderLocalVar.Path {{#allParams}}{{paramName}} {{/allParams}}{{/lambda.joinWithComma}});
Events.ExecuteOnError{{operationId}}(e);
throw;
}
{{/lambda.trimLineBreaks}}
}
{{^vendorExtensions.x-duplicates}}
{{#responses}}
{{#-first}}
///
/// The
///
{{>visibility}} partial class {{operationId}}ApiResponse : {{packageName}}.{{clientPackage}}.ApiResponse, {{interfacePrefix}}{{operationId}}ApiResponse
{
///
/// The logger
///
public ILogger<{{operationId}}ApiResponse> Logger { get; }
///
/// The
///
///
///
///
///
///
///
///
public {{operationId}}ApiResponse(ILogger<{{operationId}}ApiResponse> logger, System.Net.Http.HttpRequestMessage httpRequestMessage, System.Net.Http.HttpResponseMessage httpResponseMessage, string rawContent, string path, DateTime requestedAt, System.Text.Json.JsonSerializerOptions jsonSerializerOptions) : base(httpRequestMessage, httpResponseMessage, rawContent, path, requestedAt, jsonSerializerOptions)
{
Logger = logger;
OnCreated(httpRequestMessage, httpResponseMessage);
}
partial void OnCreated(global::System.Net.Http.HttpRequestMessage httpRequestMessage, System.Net.Http.HttpResponseMessage httpResponseMessage);
{{#responses}}
{{#vendorExtensions.x-http-status-is-default}}
///
/// Returns true if the response is the default response type
///
///
public bool Is{{vendorExtensions.x-http-status}} => {{#vendorExtensions.x-only-default}}true{{/vendorExtensions.x-only-default}}{{^vendorExtensions.x-only-default}}{{#lambda.joinConditions}}{{#responses}}{{^vendorExtensions.x-http-status-is-default}}!Is{{vendorExtensions.x-http-status}} {{/vendorExtensions.x-http-status-is-default}}{{/responses}}{{/lambda.joinConditions}}{{/vendorExtensions.x-only-default}};
{{/vendorExtensions.x-http-status-is-default}}
{{^vendorExtensions.x-http-status-is-default}}
///
/// Returns true if the response is {{code}} {{vendorExtensions.x-http-status}}
///
///
{{#vendorExtensions.x-http-status-range}}
public bool Is{{vendorExtensions.x-http-status}}
{
get
{
int statusCode = (int)StatusCode;
return {{vendorExtensions.x-http-status-range}}00 >= statusCode && {{vendorExtensions.x-http-status-range}}99 <= statusCode;
}
}
{{/vendorExtensions.x-http-status-range}}
{{^vendorExtensions.x-http-status-range}}
public bool Is{{vendorExtensions.x-http-status}} => {{code}} == (int)StatusCode;
{{/vendorExtensions.x-http-status-range}}
{{/vendorExtensions.x-http-status-is-default}}
{{#dataType}}
///
/// Deserializes the response if the response is {{code}} {{vendorExtensions.x-http-status}}
///
///
public {{#isModel}}{{^containerType}}{{packageName}}.{{modelPackage}}.{{/containerType}}{{/isModel}}{{{dataType}}}{{#nrt}}?{{/nrt}}{{^nrt}}{{#vendorExtensions.x-is-value-type}}?{{/vendorExtensions.x-is-value-type}}{{/nrt}} {{vendorExtensions.x-http-status}}()
{
{{#lambda.trimTrailingWithNewLine}}
{{#lambda.indent4}}
{{>AsModel}}
{{/lambda.indent4}}
{{/lambda.trimTrailingWithNewLine}}
}
///
/// Returns true if the response is {{code}} {{vendorExtensions.x-http-status}} and the deserialized response is not null
///
///
///
public bool Try{{vendorExtensions.x-http-status}}({{#net60OrLater}}[NotNullWhen(true)]{{/net60OrLater}}out {{#isModel}}{{^containerType}}{{packageName}}.{{modelPackage}}.{{/containerType}}{{/isModel}}{{{dataType}}}{{#nrt}}?{{/nrt}}{{^nrt}}{{#vendorExtensions.x-is-value-type}}?{{/vendorExtensions.x-is-value-type}}{{/nrt}} result)
{
result = null;
try
{
result = {{vendorExtensions.x-http-status}}();
} catch (Exception e)
{
OnDeserializationErrorDefaultImplementation(e, (HttpStatusCode){{#vendorExtensions.x-http-status-range}}{{.}}{{/vendorExtensions.x-http-status-range}}{{^vendorExtensions.x-http-status-range}}{{code}}{{/vendorExtensions.x-http-status-range}});
}
return result != null;
}
{{/dataType}}
{{#-last}}
private void OnDeserializationErrorDefaultImplementation(Exception exception, HttpStatusCode httpStatusCode)
{
bool suppressDefaultLog = false;
OnDeserializationError(ref suppressDefaultLog, exception, httpStatusCode);
{{#lambda.trimTrailingWithNewLine}}
{{#lambda.indent4}}
{{>OnDeserializationError}}
{{/lambda.indent4}}
{{/lambda.trimTrailingWithNewLine}}
}
partial void OnDeserializationError(ref bool suppressDefaultLog, Exception exception, HttpStatusCode httpStatusCode);
{{/-last}}
{{/responses}}
}
{{/-first}}
{{/responses}}
{{/vendorExtensions.x-duplicates}}
{{/operation}}
}
{{/operations}}
}
{{/lambda.trimLineBreaks}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy