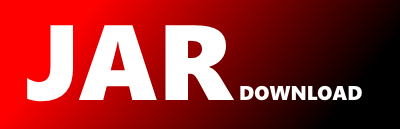
swift6.JSONValue.mustache Maven / Gradle / Ivy
//
// JSONValue.swift
//
// Generated by openapi-generator
// https://openapi-generator.tech
//
import Foundation
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} enum JSONValue: Sendable, Codable, Hashable {
case string(String)
case int(Int)
case double(Double)
case bool(Bool)
case array([JSONValue])
case dictionary([String: JSONValue])
case null
// MARK: - Decoding Logic
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(from decoder: Decoder) throws {
let container = try decoder.singleValueContainer()
if let stringValue = try? container.decode(String.self) {
self = .string(stringValue)
} else if let intValue = try? container.decode(Int.self) {
self = .int(intValue)
} else if let doubleValue = try? container.decode(Double.self) {
self = .double(doubleValue)
} else if let boolValue = try? container.decode(Bool.self) {
self = .bool(boolValue)
} else if let arrayValue = try? container.decode([JSONValue].self) {
self = .array(arrayValue)
} else if let dictionaryValue = try? container.decode([String: JSONValue].self) {
self = .dictionary(dictionaryValue)
} else if container.decodeNil() {
self = .null
} else {
throw DecodingError.dataCorruptedError(in: container, debugDescription: "Unknown JSON value")
}
}
// MARK: - Encoding Logic
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} func encode(to encoder: Encoder) throws {
var container = encoder.singleValueContainer()
switch self {
case .string(let value):
try container.encode(value)
case .int(let value):
try container.encode(value)
case .double(let value):
try container.encode(value)
case .bool(let value):
try container.encode(value)
case .array(let value):
try container.encode(value)
case .dictionary(let value):
try container.encode(value)
case .null:
try container.encodeNil()
}
}
}
extension JSONValue {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ value: String) {
self = .string(value)
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ value: Int) {
self = .int(value)
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ value: Double) {
self = .double(value)
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ value: Bool) {
self = .bool(value)
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ value: [JSONValue]) {
self = .array(value)
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ value: [String: JSONValue]) {
self = .dictionary(value)
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(_ codable: T) throws {
let encoder = JSONEncoder()
let encodedData = try encoder.encode(codable)
let decoder = JSONDecoder()
let decodedValue = try decoder.decode(JSONValue.self, from: encodedData)
self = decodedValue
}
}
extension JSONValue {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isString: Bool {
if case .string = self { return true }
return false
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isInt: Bool {
if case .int = self { return true }
return false
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isDouble: Bool {
if case .double = self { return true }
return false
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isBool: Bool {
if case .bool = self { return true }
return false
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isArray: Bool {
if case .array = self { return true }
return false
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isDictionary: Bool {
if case .dictionary = self { return true }
return false
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var isNull: Bool {
return self == .null
}
}
extension JSONValue {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var stringValue: String? {
switch self {
case .string(let value):
return value
default:
return nil
}
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var intValue: Int? {
switch self {
case .int(let value):
return value
default:
return nil
}
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var doubleValue: Double? {
switch self {
case .double(let value):
return value
default:
return nil
}
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var boolValue: Bool? {
switch self {
case .bool(let value):
return value
default:
return nil
}
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var arrayValue: [JSONValue]? {
if case let .array(value) = self {
return value
}
return nil
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} var dictionaryValue: [String: JSONValue]? {
if case let .dictionary(value) = self {
return value
}
return nil
}
}
extension JSONValue {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} subscript(key: String) -> JSONValue? {
return dictionaryValue?[key]
}
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} subscript(index: Int) -> JSONValue? {
guard case let .array(array) = self, index >= 0 && index < array.count else {
return nil
}
return array[index]
}
}
extension JSONValue: ExpressibleByStringLiteral, ExpressibleByStringInterpolation {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(stringLiteral value: StringLiteralType) {
self = .string(value)
}
}
extension JSONValue: ExpressibleByIntegerLiteral {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(integerLiteral value: IntegerLiteralType) {
self = .int(value)
}
}
extension JSONValue: ExpressibleByFloatLiteral {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(floatLiteral value: FloatLiteralType) {
self = .double(value)
}
}
extension JSONValue: ExpressibleByBooleanLiteral {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(booleanLiteral value: BooleanLiteralType) {
self = .bool(value)
}
}
extension JSONValue: ExpressibleByArrayLiteral {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(arrayLiteral elements: JSONValue...) {
self = .array(elements)
}
}
extension JSONValue: ExpressibleByDictionaryLiteral {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(dictionaryLiteral elements: (String, JSONValue)...) {
var dict: [String: JSONValue] = [:]
for (key, value) in elements {
dict[key] = value
}
self = .dictionary(dict)
}
}
extension JSONValue: ExpressibleByNilLiteral {
{{#nonPublicApi}}internal{{/nonPublicApi}}{{^nonPublicApi}}public{{/nonPublicApi}} init(nilLiteral: ()) {
self = .null
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy