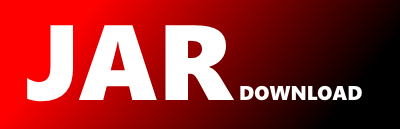
cpp-pistache-server.helpers-header.mustache Maven / Gradle / Ivy
{{>licenseInfo}}
/*
* {{prefix}}Helpers.h
*
* This is the helper class for models and primitives
*/
#ifndef {{prefix}}Helpers_H_
#define {{prefix}}Helpers_H_
#include
#include
#include
#include
#include
© 2015 - 2025 Weber Informatics LLC | Privacy Policy