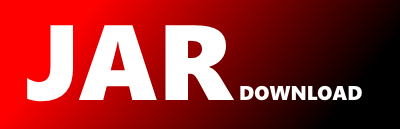
kotlin-client.libraries.jvm-volley.api.mustache Maven / Gradle / Ivy
package {{apiPackage}}
import android.content.Context
import com.android.volley.DefaultRetryPolicy
import com.android.volley.Request
import com.android.volley.RequestQueue
import com.android.volley.Response
import com.android.volley.toolbox.BaseHttpStack
import com.android.volley.toolbox.Volley
import java.util.*
import kotlin.coroutines.resume
import kotlin.coroutines.resumeWithException
import kotlin.coroutines.suspendCoroutine
import com.google.gson.reflect.TypeToken
import {{packageName}}.request.IRequestFactory
import {{packageName}}.request.RequestFactory
import {{packageName}}.infrastructure.CollectionFormats.*
{{#imports}}import {{import}}
{{/imports}}
{{#operations}}
/*
* If you wish to use a custom http stack with your client you
* can pass that to the request queue like:
* Volley.newRequestQueue(context.applicationContext, myCustomHttpStack)
*/
class {{classname}} (
private val context: Context,
private val requestQueue: Lazy = lazy(initializer = {
Volley.newRequestQueue(context.applicationContext)
}),
private val requestFactory: IRequestFactory = RequestFactory(),
private val basePath: String = "{{{basePath}}}",
private val postProcessors :List <(Request<*>) -> Unit> = listOf()) {
{{#operation}}
/**
* {{summary}}
* {{notes}}
{{#allParams}}* @param {{{paramName}}} {{description}}{{^required}} (optional{{#defaultValue}}, default to {{{.}}}{{/defaultValue}}){{/required}}{{#required}}{{#defaultValue}} (default to {{{.}}}){{/defaultValue}}{{/required}}
{{/allParams}}* @return {{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}void{{/returnType}}
*/
{{#isDeprecated}}
@Deprecated("This api was deprecated")
{{/isDeprecated}}
suspend fun {{operationId}}({{^allParams}}){{/allParams}}{{#allParams}}{{>queryParams}}{{>pathParams}}{{>headerParams}}{{>bodyParams}}{{>formParams}}{{^-last}}, {{/-last}}{{#-last}}{{#operationAuthMethod}}, opAuthHeaderFactory = () -> map{{/operationAuthMethod}}){{/-last}}{{/allParams}}: {{#returnType}}{{{returnType}}}?{{/returnType}}{{^returnType}}Unit{{/returnType}} {
{{#bodyParam}}
val body: Any? = {{paramName}}
{{/bodyParam}}
{{^bodyParam}}
val body: Any? = null
{{/bodyParam}}
val contentTypes : Array = arrayOf({{#consumes}}"{{{mediaType}}}"{{^-last}},{{/-last}}{{/consumes}})
val contentType: String = if (contentTypes.isNotEmpty()) { contentTypes.first() } else { "application/json" }
// Do some work or avoid some work based on what we know about the model,
// before we delegate to a pluggable request factory template
// The request factory template contains only pure code and no templates
// to make it easy to override with your own.
// create path and map variables
val path = "{{{path}}}"{{#pathParams}}.replace("{" + "{{baseName}}" + "}", {{#isContainer}}{{paramName}}.joinToString(","){{/isContainer}}{{^isContainer}}IRequestFactory.escapeString({{{paramName}}}.toString()){{/isContainer}}){{/pathParams}}
val formParams = mapOf({{^formParams}}){{/formParams}}{{#formParams}}
"{{baseName}}" to IRequestFactory.parameterToString({{paramName}}){{^-last}},{{/-last}}{{#-last}}
){{/-last}}{{/formParams}}
// TODO: Cater for allowing empty values
// TODO, if its apikey auth, then add the header names here and the hardcoded auth key
// Only support hard coded apikey in query param auth for when we do this first path
val queryParams = mapOf({{^queryParams}}){{/queryParams}}{{#queryParams}}
"{{baseName}}" to IRequestFactory.parameterToString({{paramName}}){{^-last}},{{/-last}}{{#-last}}
){{/-last}}{{/queryParams}}
.filter { it.value.isNotEmpty() }
val headerParams: Map = mapOf({{^headerParams}}){{/headerParams}}{{#headerParams}}
"{{baseName}}" to IRequestFactory.parameterToString({{paramName}}){{^-last}},{{/-last}}{{#-last}}
){{/-last}}{{/headerParams}}
return suspendCoroutine { continuation ->
val responseListener = Response.Listener<{{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}Unit{{/returnType}}> { response ->
continuation.resume(response)
}
val errorListener = Response.ErrorListener { error ->
continuation.resumeWithException(error)
}
val responseType = object : TypeToken<{{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}Unit{{/returnType}}>() {}.type
// Call the correct request builder based on whether we have a return type or a body.
// All other switching on types must be done in code inside the builder
val request: Request<{{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}Unit{{/returnType}}> = requestFactory.build(
Request.Method.{{httpMethod}},
"$basePath$path",
body,
headerParams,
queryParams,
formParams,
contentType,
responseType,
responseListener,
errorListener)
postProcessors.forEach { it.invoke(request) }
requestQueue.value.add(request)
}
}
{{/operation}}
}
{{/operations}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy