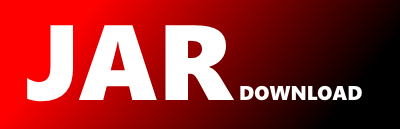
csharp.modelOneOf.mustache Maven / Gradle / Ivy
{{#model}}
///
/// {{description}}{{^description}}{{classname}}{{/description}}
///
{{#vendorExtensions.x-cls-compliant}}
[CLSCompliant({{{.}}})]
{{/vendorExtensions.x-cls-compliant}}
{{#vendorExtensions.x-com-visible}}
[ComVisible({{{.}}})]
{{/vendorExtensions.x-com-visible}}
[JsonConverter(typeof({{classname}}JsonConverter))]
[DataContract(Name = "{{{name}}}")]
{{>visibility}} partial class {{classname}} : {{#lambda.joinWithComma}}AbstractOpenAPISchema {{#parent}}{{{.}}} {{/parent}}{{#equatable}}IEquatable<{{classname}}> {{/equatable}}{{#validatable}}IValidatableObject {{/validatable}}{{/lambda.joinWithComma}}
{
{{#isNullable}}
///
/// Initializes a new instance of the class.
///
public {{classname}}()
{
this.IsNullable = true;
this.SchemaType= "oneOf";
}
{{/isNullable}}
{{#composedSchemas.oneOf}}
{{^vendorExtensions.x-duplicated-data-type}}
{{^isNull}}
///
/// Initializes a new instance of the class
/// with the class
///
/// An instance of {{dataType}}.
public {{classname}}({{{dataType}}} actualInstance)
{
this.IsNullable = {{#model.isNullable}}true{{/model.isNullable}}{{^model.isNullable}}false{{/model.isNullable}};
this.SchemaType= "oneOf";
this.ActualInstance = actualInstance{{^model.isNullable}}{{^isPrimitiveType}} ?? throw new ArgumentException("Invalid instance found. Must not be null."){{/isPrimitiveType}}{{#isPrimitiveType}}{{#isArray}} ?? throw new ArgumentException("Invalid instance found. Must not be null."){{/isArray}}{{/isPrimitiveType}}{{#isPrimitiveType}}{{#isFreeFormObject}} ?? throw new ArgumentException("Invalid instance found. Must not be null."){{/isFreeFormObject}}{{/isPrimitiveType}}{{#isPrimitiveType}}{{#isString}} ?? throw new ArgumentException("Invalid instance found. Must not be null."){{/isString}}{{/isPrimitiveType}}{{/model.isNullable}};
}
{{/isNull}}
{{/vendorExtensions.x-duplicated-data-type}}
{{/composedSchemas.oneOf}}
private Object _actualInstance;
///
/// Gets or Sets ActualInstance
///
public override Object ActualInstance
{
get
{
return _actualInstance;
}
set
{
{{#oneOf}}
{{^-first}}else {{/-first}}if (value.GetType() == typeof({{{.}}}))
{
this._actualInstance = value;
}
{{/oneOf}}
else
{
throw new ArgumentException("Invalid instance found. Must be the following types:{{#oneOf}} {{{.}}}{{^-last}},{{/-last}}{{/oneOf}}");
}
}
}
{{#composedSchemas.oneOf}}
{{^vendorExtensions.x-duplicated-data-type}}
{{^isNull}}
///
/// Get the actual instance of `{{dataType}}`. If the actual instance is not `{{dataType}}`,
/// the InvalidClassException will be thrown
///
/// An instance of {{dataType}}
public {{{dataType}}} Get{{#lambda.titlecase}}{{baseType}}{{/lambda.titlecase}}{{#isArray}}{{#lambda.titlecase}}{{{dataFormat}}}{{/lambda.titlecase}}{{/isArray}}()
{
return ({{{dataType}}})this.ActualInstance;
}
{{/isNull}}
{{/vendorExtensions.x-duplicated-data-type}}
{{/composedSchemas.oneOf}}
///
/// Returns the string presentation of the object
///
/// String presentation of the object
public override string ToString()
{
var sb = new StringBuilder();
sb.Append("class {{classname}} {\n");
sb.Append(" ActualInstance: ").Append(this.ActualInstance).Append("\n");
sb.Append("}\n");
return sb.ToString();
}
///
/// Returns the JSON string presentation of the object
///
/// JSON string presentation of the object
public override string ToJson()
{
return JsonConvert.SerializeObject(this.ActualInstance, {{classname}}.SerializerSettings);
}
///
/// Converts the JSON string into an instance of {{classname}}
///
/// JSON string
/// An instance of {{classname}}
public static {{classname}} FromJson(string jsonString)
{
{{classname}} new{{classname}} = null;
if (string.IsNullOrEmpty(jsonString))
{
return new{{classname}};
}
{{#useOneOfDiscriminatorLookup}}
{{#discriminator}}
try
{
var discriminatorObj = JObject.Parse(jsonString)["{{{propertyBaseName}}}"];
string discriminatorValue = discriminatorObj == null ?string.Empty :discriminatorObj.ToString();
switch (discriminatorValue)
{
{{#mappedModels}}
case "{{{mappingName}}}":
new{{classname}} = new {{classname}}(JsonConvert.DeserializeObject<{{{modelName}}}>(jsonString, {{classname}}.AdditionalPropertiesSerializerSettings));
return new{{classname}};
{{/mappedModels}}
default:
System.Diagnostics.Debug.WriteLine(string.Format("Failed to lookup discriminator value `{0}` for {{classname}}. Possible values:{{#mappedModels}} {{{mappingName}}}{{/mappedModels}}", discriminatorValue));
break;
}
}
catch (Exception ex)
{
System.Diagnostics.Debug.WriteLine(string.Format("Failed to parse the json data : `{0}` {1}", jsonString, ex.ToString()));
}
{{/discriminator}}
{{/useOneOfDiscriminatorLookup}}
int match = 0;
List matchedTypes = new List();
{{#oneOf}}
try
{
// if it does not contains "AdditionalProperties", use SerializerSettings to deserialize
if (typeof({{{.}}}).GetProperty("AdditionalProperties") == null)
{
new{{classname}} = new {{classname}}(JsonConvert.DeserializeObject<{{{.}}}>(jsonString, {{classname}}.SerializerSettings));
}
else
{
new{{classname}} = new {{classname}}(JsonConvert.DeserializeObject<{{{.}}}>(jsonString, {{classname}}.AdditionalPropertiesSerializerSettings));
}
matchedTypes.Add("{{{.}}}");
match++;
}
catch (Exception exception)
{
// deserialization failed, try the next one
System.Diagnostics.Debug.WriteLine(string.Format("Failed to deserialize `{0}` into {{{.}}}: {1}", jsonString, exception.ToString()));
}
{{/oneOf}}
if (match == 0)
{
throw new InvalidDataException("The JSON string `" + jsonString + "` cannot be deserialized into any schema defined.");
}
else if (match > 1)
{
throw new InvalidDataException("The JSON string `" + jsonString + "` incorrectly matches more than one schema (should be exactly one match): " + String.Join(",", matchedTypes));
}
// deserialization is considered successful at this point if no exception has been thrown.
return new{{classname}};
}
{{#equatable}}
///
/// Returns true if objects are equal
///
/// Object to be compared
/// Boolean
public override bool Equals(object input)
{
{{#useCompareNetObjects}}
return OpenAPIClientUtils.compareLogic.Compare(this, input as {{classname}}).AreEqual;
{{/useCompareNetObjects}}
{{^useCompareNetObjects}}
return this.Equals(input as {{classname}});
{{/useCompareNetObjects}}
}
///
/// Returns true if {{classname}} instances are equal
///
/// Instance of {{classname}} to be compared
/// Boolean
public bool Equals({{classname}} input)
{
{{#useCompareNetObjects}}
return OpenAPIClientUtils.compareLogic.Compare(this, input).AreEqual;
{{/useCompareNetObjects}}
{{^useCompareNetObjects}}
if (input == null)
return false;
return this.ActualInstance.Equals(input.ActualInstance);
{{/useCompareNetObjects}}
}
///
/// Gets the hash code
///
/// Hash code
public override int GetHashCode()
{
unchecked // Overflow is fine, just wrap
{
int hashCode = 41;
if (this.ActualInstance != null)
hashCode = hashCode * 59 + this.ActualInstance.GetHashCode();
return hashCode;
}
}
{{/equatable}}
{{#validatable}}
///
/// To validate all properties of the instance
///
/// Validation context
/// Validation Result
IEnumerable IValidatableObject.Validate(ValidationContext validationContext)
{
yield break;
}
{{/validatable}}
}
///
/// Custom JSON converter for {{classname}}
///
public class {{classname}}JsonConverter : JsonConverter
{
///
/// To write the JSON string
///
/// JSON writer
/// Object to be converted into a JSON string
/// JSON Serializer
public override void WriteJson(JsonWriter writer, object value, JsonSerializer serializer)
{
writer.WriteRawValue((string)(typeof({{classname}}).GetMethod("ToJson").Invoke(value, null)));
}
///
/// To convert a JSON string into an object
///
/// JSON reader
/// Object type
/// Existing value
/// JSON Serializer
/// The object converted from the JSON string
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, JsonSerializer serializer)
{
switch(reader.TokenType)
{
{{#composedSchemas.oneOf}}
{{^vendorExtensions.x-duplicated-data-type}}
{{#isInteger}}
case JsonToken.Integer:
return new {{classname}}(Convert.ToInt32(reader.Value));
{{/isInteger}}
{{#isNumber}}
case JsonToken.Float:
return new {{classname}}(Convert.ToDecimal(reader.Value));
{{/isNumber}}
{{#isString}}
case JsonToken.String:
return new {{classname}}(Convert.ToString(reader.Value));
{{/isString}}
{{#isBoolean}}
case JsonToken.Boolean:
return new {{classname}}(Convert.ToBoolean(reader.Value));
{{/isBoolean}}
{{#isDate}}
case JsonToken.Date:
return new {{classname}}(Convert.ToDateTime(reader.Value));
{{/isDate}}
{{/vendorExtensions.x-duplicated-data-type}}
{{/composedSchemas.oneOf}}
case JsonToken.StartObject:
return {{classname}}.FromJson(JObject.Load(reader).ToString(Formatting.None));
case JsonToken.StartArray:
return {{classname}}.FromJson(JArray.Load(reader).ToString(Formatting.None));
default:
return null;
}
}
///
/// Check if the object can be converted
///
/// Object type
/// True if the object can be converted
public override bool CanConvert(Type objectType)
{
return false;
}
}
{{/model}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy