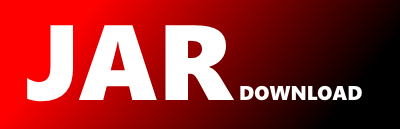
org.openbp.cockpit.modeler.figures.generic.SimpleImageFigure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openbp-cockpit Show documentation
Show all versions of openbp-cockpit Show documentation
OpenBP Cockpit (graphical process modeler)
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openbp.cockpit.modeler.figures.generic;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Point;
import java.awt.Rectangle;
import java.util.Vector;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import org.openbp.common.icon.MultiIcon;
import CH.ifa.draw.standard.AbstractFigure;
import CH.ifa.draw.standard.BoxHandleKit;
/**
* A simple image figure.
*
* @author Heiko Erhardt
*/
public class SimpleImageFigure extends AbstractFigure
{
/** Image */
private transient Image image;
/** Display box */
protected Rectangle box;
//////////////////////////////////////////////////
// @@ Construction
//////////////////////////////////////////////////
/**
* Default constructor.
*/
public SimpleImageFigure()
{
box = new Rectangle();
}
/**
* Default constructor.
*
* @param image Image
*/
public SimpleImageFigure(Image image)
{
this();
setImage(image);
}
/**
* Default constructor.
*
* @param icon Icon
*/
public SimpleImageFigure(Icon icon)
{
this();
setIcon(icon);
}
//////////////////////////////////////////////////
// @@ AbstractFigure overrides
//////////////////////////////////////////////////
public void basicDisplayBox(Point origin, Point corner)
{
box = new Rectangle(origin);
box.add(corner);
}
public Vector handles()
{
Vector handles = new Vector();
BoxHandleKit.addHandles(this, handles);
return handles;
}
public Rectangle displayBox()
{
return new Rectangle(box);
}
protected void basicMoveBy(int x, int y)
{
box.translate(x, y);
}
//////////////////////////////////////////////////
// @@ Drawing
//////////////////////////////////////////////////
public void draw(Graphics g)
{
if (image == null)
return;
g.drawImage(image, box.x, box.y, box.width, box.height, null);
}
/**
* Gets the image.
*/
public Image getImage()
{
return image;
}
/**
* Sets the image.
*/
public void setImage(Image image)
{
this.image = image;
}
/**
* Sets the icon.
*/
public void setIcon(Icon icon)
{
if (icon instanceof ImageIcon)
{
image = ((ImageIcon) icon).getImage();
}
else if (icon instanceof MultiIcon)
{
image = ((MultiIcon) icon).getImage();
}
box.width = icon.getIconWidth();
box.height = icon.getIconHeight();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy