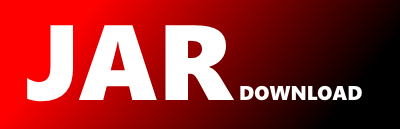
org.openbp.cockpit.modeler.figures.process.ProcessVariableFigure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openbp-cockpit Show documentation
Show all versions of openbp-cockpit Show documentation
OpenBP Cockpit (graphical process modeler)
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openbp.cockpit.modeler.figures.process;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.Stroke;
import java.awt.datatransfer.Transferable;
import java.awt.event.MouseEvent;
import java.util.List;
import java.util.Vector;
import org.openbp.cockpit.modeler.ViewModeMgr;
import org.openbp.cockpit.modeler.drawing.ProcessDrawing;
import org.openbp.cockpit.modeler.figures.VisualElement;
import org.openbp.cockpit.modeler.figures.VisualElementEvent;
import org.openbp.cockpit.modeler.figures.generic.SimpleImageFigure;
import org.openbp.cockpit.modeler.figures.generic.XFigure;
import org.openbp.common.icon.FlexibleSize;
import org.openbp.common.util.ToStringHelper;
import org.openbp.core.model.item.process.ProcessObject;
import org.openbp.core.model.item.process.ProcessVariable;
import org.openbp.guiclient.model.item.ItemIconMgr;
import org.openbp.jaspira.decoration.DecorationMgr;
import org.openbp.jaspira.gui.interaction.DropClientUtil;
import CH.ifa.draw.framework.Figure;
/**
* This is the figurative representation of a process variable.
*
* @author Stephan Moritz
*/
public class ProcessVariableFigure extends SimpleImageFigure
implements ProcessElementContainer
{
/** The param that this figure represents */
private ProcessVariable param;
/** Parent figure (the param tag figure we are associated with) */
private ParamFigure parent;
/**
* Constructor.
*
* @param param Param the figure belongs to
* @param parent Parent figure
*/
public ProcessVariableFigure(ProcessVariable param, ParamFigure parent)
{
super(ItemIconMgr.getInstance().getTypeIcon(parent.getDrawing().getProcessSkin().getName(), param.getDataType(), FlexibleSize.SMALL));
this.param = param;
param.setRepresentation(this);
this.parent = parent;
}
/**
* Gets the the param that this figure represents.
*/
public ProcessVariable getProcessVariable()
{
return param;
}
/**
* Returns a string representation of this object.
*/
public String toString()
{
return ToStringHelper.toString(this, "paramFigure", "ProcessVariable");
}
/////////////////////////////////////////////////////////////////////////
// @@ Figure overrides
/////////////////////////////////////////////////////////////////////////
public boolean canConnect()
{
return false;
}
public Vector handles()
{
return parent.handles();
}
/**
* Draws the icon. Checks via the decoration manager for stroke and framecolor decorations,
* if so, draws a frame around the image using the given parameters.
*
* @param g The graphics object to draw upon
*/
public void draw(Graphics g)
{
if (!ViewModeMgr.getInstance().isDataLinkVisible(this))
{
// Don't paint if data link display is turned off
return;
}
super.draw(g);
Stroke stroke = (Stroke) DecorationMgr.decorate(this, XFigure.DECO_FRAMESTROKE, null);
Color frame = (Color) DecorationMgr.decorate(this, XFigure.DECO_FRAMECOLOR, null);
if (frame != null && stroke != null)
{
Graphics2D g2 = (Graphics2D) g;
g2.setStroke(stroke);
g.setColor(frame);
Rectangle r = displayBox();
g.drawRoundRect(r.x, r.y, r.width - 1, r.height - 1, 8, 8);
}
}
public boolean containsPoint(int x, int y)
{
if (!ViewModeMgr.getInstance().isDataLinkVisible(this))
{
// We won't react on user interaction if we are not displayed
return false;
}
return super.containsPoint(x, y);
}
public void release()
{
super.release();
parent.removeProcessVariableConnection();
}
/////////////////////////////////////////////////////////////////////////
// @@ ProcessElementContainer implementation
/////////////////////////////////////////////////////////////////////////
public ProcessObject getProcessElement()
{
return param;
}
public ProcessObject getReferredProcessElement()
{
return getProcessElement();
}
/**
* No object should be selected on deletion.
*/
public Figure selectionOnDelete()
{
return null;
}
//////////////////////////////////////////////////
// @@ VisualElement implementation
//////////////////////////////////////////////////
public void setDrawing(ProcessDrawing drawing)
{
}
public ProcessDrawing getDrawing()
{
return parent.getDrawing();
}
public VisualElement getParentElement()
{
return parent;
}
public Figure getPresentationFigure()
{
return this;
}
public void updatePresentationFigure()
{
// No dynamic presentation figure, so do nothing
}
public boolean isVisible()
{
return false;
}
public void setVisible(boolean visible)
{
}
public boolean handleEvent(VisualElementEvent event)
{
return false;
}
/////////////////////////////////////////////////////////////////////////
// @@ InteractionClient implementation
/////////////////////////////////////////////////////////////////////////
public List getDropRegions(List flavors, Transferable data, MouseEvent mouseEvent)
{
return null;
}
public List getImportersAt(Point p)
{
return null;
}
public List getAllImportersAt(Point p)
{
return DropClientUtil.getAllImportersAt(this, p);
}
public List getAllDropRegions(List flavors, Transferable data, MouseEvent mouseEvent)
{
return null;
}
public boolean importData(Object regionId, Transferable data, Point p)
{
return false;
}
public void dragStarted(Transferable transferable)
{
DropClientUtil.dragStarted(this, transferable);
}
public void dragEnded(Transferable transferable)
{
DropClientUtil.dragEnded(this, transferable);
}
public void dragActionTriggered(Object regionId, Point p)
{
}
public List getSubClients()
{
return null;
}
//////////////////////////////////////////////////
// @@ UpdatableFigure implementation
//////////////////////////////////////////////////
public void updateFigure()
{
// Nothing changed
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy