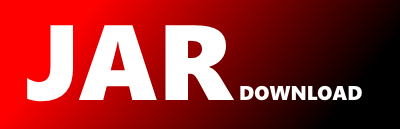
org.openbp.cockpit.modeler.figures.process.TextElementFigure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openbp-cockpit Show documentation
Show all versions of openbp-cockpit Show documentation
OpenBP Cockpit (graphical process modeler)
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openbp.cockpit.modeler.figures.process;
import java.awt.Color;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.awt.event.MouseEvent;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
import java.util.Vector;
import org.openbp.cockpit.modeler.drawing.ProcessDrawing;
import org.openbp.cockpit.modeler.drawing.WorkspaceDrawingView;
import org.openbp.cockpit.modeler.figures.VisualElement;
import org.openbp.cockpit.modeler.figures.VisualElementEvent;
import org.openbp.cockpit.modeler.figures.generic.XFontSizeHandle;
import org.openbp.cockpit.modeler.figures.generic.XTextFigure;
import org.openbp.cockpit.modeler.util.ModelerFlavors;
import org.openbp.common.util.ToStringHelper;
import org.openbp.core.model.item.process.ProcessObject;
import org.openbp.core.model.item.process.TextElement;
import org.openbp.jaspira.gui.interaction.Importer;
import org.openbp.jaspira.gui.interaction.ViewDropRegion;
import org.openbp.swing.SwingUtil;
import CH.ifa.draw.framework.Figure;
import CH.ifa.draw.standard.AbstractLocator;
/**
* The text element figure represents a simple text rectangle.
* The size of the figure is computed automatically based on the text and font settings.
*
* @author Stephan Moritz
*/
public class TextElementFigure extends XTextFigure
implements ProcessElementContainer
{
/** Region id for color region */
public static final String REGION_COLOR = "color";
/** Text element */
private TextElement textElement;
/** The visual status as defined in org.openbp.cockpit.modeler.figures.VisualElement */
private int visualStatus = VISUAL_VISIBLE;
/** Process drawing we belong to */
private ProcessDrawing drawing;
/**
* Constructor.
*/
public TextElementFigure()
{
super();
}
/**
* Connects the figure to a process object.
*
* @param textElement Text element
* @param drawing Process drawing that owns the figure
*/
public void connect(TextElement textElement, ProcessDrawing drawing)
{
// Text figure that adjusts its size according to the text
setAutoSize(true);
this.textElement = textElement;
this.drawing = drawing;
String text = textElement.getDescription();
if (text == null)
text = "Text";
setText(text);
decodeGeometry();
}
/**
* Gets the text element.
*/
public TextElement getTextElement()
{
return textElement;
}
/**
* Returns a string representation of this object.
*/
public String toString()
{
return ToStringHelper.toString(this, "textElement");
}
//////////////////////////////////////////////////
// @@ Figure overrides
//////////////////////////////////////////////////
/**
* We display a single font size handle in the top left corner only.
*/
public Vector handles()
{
Vector handles = new Vector();
handles.addElement(new XFontSizeHandle(this, new SizeHandleLocator(this)));
return handles;
}
public void release()
{
super.release();
// Remove the element from the process
getDrawing().getProcess().removeTextElement(textElement);
}
//////////////////////////////////////////////////
// @@ Geometry serialization support
//////////////////////////////////////////////////
/**
* Decodes the enclosed geometry information of the asssociated process element.
*/
public void decodeGeometry()
{
parseGeometry(textElement.getGeometry());
}
/**
* Encodes the geometry information of this object into the associated process element.
*/
public void encodeGeometry()
{
textElement.setGeometry(createGeometry());
}
//////////////////////////////////////////////////
// @@ ProcessElementContainer implementation
//////////////////////////////////////////////////
public ProcessObject getProcessElement()
{
return textElement;
}
public ProcessObject getReferredProcessElement()
{
return getProcessElement();
}
/**
* No object should be selected on deletion.
*/
public Figure selectionOnDelete()
{
return null;
}
//////////////////////////////////////////////////
// @@ VisualElement implementation
//////////////////////////////////////////////////
public void setDrawing(ProcessDrawing drawing)
{
this.drawing = drawing;
}
public ProcessDrawing getDrawing()
{
return drawing;
}
public VisualElement getParentElement()
{
return getDrawing();
}
public Figure getPresentationFigure()
{
return this;
}
public void updatePresentationFigure()
{
// No dynamic presentation figure, so do nothing
}
public boolean isVisible()
{
return (visualStatus & VisualElement.VISUAL_VISIBLE) != 0;
}
public void setVisible(boolean visible)
{
willChange();
if (visible)
{
visualStatus |= VisualElement.VISUAL_VISIBLE;
}
else
{
visualStatus &= ~ VisualElement.VISUAL_VISIBLE;
}
changed();
}
public boolean handleEvent(VisualElementEvent event)
{
return false;
}
//////////////////////////////////////////////////
// @@ UpdatableFigure implementation
//////////////////////////////////////////////////
public void updateFigure()
{
String newText = textElement.getDescription();
if (newText == null)
newText = "Text";
setText(newText);
}
/////////////////////////////////////////////////////////////////////////
// @@ InteractionClient implementation
/////////////////////////////////////////////////////////////////////////
public void dragActionTriggered(Object regionId, Point p)
{
}
public void dragEnded(Transferable transferable)
{
}
public void dragStarted(Transferable transferable)
{
}
public List getAllDropRegions(List flavors, Transferable data, MouseEvent mouseEvent)
{
return getDropRegions(flavors, data, mouseEvent);
}
public List getDropRegions(List flavors, Transferable data, MouseEvent mouseEvent)
{
if (flavors.contains(ModelerFlavors.COLOR))
{
// Use the whole figure as target
WorkspaceDrawingView view = getDrawing().getView();
Rectangle r = view.applyScale(displayBox(), false);
return Collections.singletonList(new ViewDropRegion(REGION_COLOR, this, r, view));
}
return null;
}
public List getImportersAt(Point p)
{
WorkspaceDrawingView view = getDrawing().getView();
Point docPoint = SwingUtil.convertFromGlassCoords(p, view);
if (containsPoint(docPoint.x, docPoint.y))
return Collections.singletonList(new Importer(REGION_COLOR, this, new DataFlavor[]
{
ModelerFlavors.COLOR
}));
return null;
}
public List getAllImportersAt(Point p)
{
return getImportersAt(p);
}
public List getSubClients()
{
return null;
}
public boolean importData(Object regionId, Transferable data, Point p)
{
try
{
if (regionId.equals(REGION_COLOR))
{
// Set the color
Color color = (Color) data.getTransferData(ModelerFlavors.COLOR);
getDrawing().getEditor().startUndo("Set Color");
setFillColor(color);
invalidate();
getDrawing().getEditor().endUndo();
return true;
}
}
catch (UnsupportedFlavorException e)
{
return false;
}
catch (IOException e)
{
return false;
}
return false;
}
/////////////////////////////////////////////////////////////////////////
// @@ Inner classes
/////////////////////////////////////////////////////////////////////////
/**
* Locator that returns a postion 5 pixels from the upper left of the display box.
*/
private class SizeHandleLocator extends AbstractLocator
{
/** Owner figure */
private final Figure owner;
/**
* Constructor
*
* @param owner Owner figure
*/
public SizeHandleLocator(Figure owner)
{
super();
this.owner = owner;
}
/**
* Returns the position.
*
* @param figure Is ignored
*/
public Point locate(Figure figure)
{
Rectangle r = owner.displayBox();
return new Point(r.x - HANDLE_DISTANCE, r.y - HANDLE_DISTANCE);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy