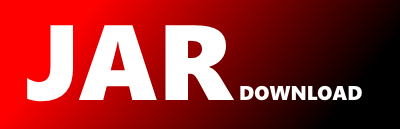
org.opencastproject.assetmanager.impl.query.SelectQueryContribution Maven / Gradle / Ivy
/**
* Licensed to The Apereo Foundation under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional
* information regarding copyright ownership.
*
*
* The Apereo Foundation licenses this file to you under the Educational
* Community License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License
* at:
*
* http://opensource.org/licenses/ecl2.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
*/
package org.opencastproject.assetmanager.impl.query;
import static com.entwinemedia.fn.Stream.$;
import com.entwinemedia.fn.Fn;
import com.entwinemedia.fn.Stream;
import com.entwinemedia.fn.data.Opt;
import com.mysema.query.types.EntityPath;
import com.mysema.query.types.Expression;
import com.mysema.query.types.OrderSpecifier;
import com.mysema.query.types.expr.BooleanExpression;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
/**
* Collect contributions to a JPA query.
* Each of the builder methods creates a new instance.
*/
@ParametersAreNonnullByDefault
public final class SelectQueryContribution {
// CHECKSTYLE:OFF
final Stream> fetch;
final Stream> from;
final Stream join;
final Opt where;
final Opt offset;
final Opt limit;
final Stream> order;
// CHECKSTYLE:ON
public SelectQueryContribution(
Stream> fetch,
Stream> from,
Stream join,
Opt where,
Opt offset,
Opt limit,
Stream> order) {
this.fetch = fetch;
this.from = from;
this.join = join;
this.where = where;
this.offset = offset;
this.limit = limit;
this.order = order;
}
/** Create a new, empty contribution. */
public static SelectQueryContribution mk() {
return new SelectQueryContribution(
Stream.>empty(),
Stream.>empty(),
Stream.empty(),
Opt.none(),
Opt.none(),
Opt.none(),
Stream.>empty()
);
}
/** Set the `fetch` contribution. */
SelectQueryContribution fetch(Stream extends Expression>> fetch) {
return new SelectQueryContribution((Stream>) fetch, from, join, where, offset, limit, order);
}
/** Add to the `from` contribution. */
SelectQueryContribution addFetch(Stream extends Expression>> fetch) {
return new SelectQueryContribution(this.fetch.append(fetch), from, join, where, offset, limit, order);
}
/** Set the `from` contribution. */
SelectQueryContribution from(Stream extends EntityPath>> from) {
return new SelectQueryContribution(fetch, (Stream>) from, join, where, offset, limit, order);
}
/** Add to the `from` contribution. */
SelectQueryContribution addFrom(Stream extends EntityPath>> from) {
return new SelectQueryContribution(fetch, this.from.append(from), join, where, offset, limit, order);
}
/** Set the `join` contribution. */
SelectQueryContribution join(Stream join) {
return new SelectQueryContribution(fetch, from, join, where, offset, limit, order);
}
/** Set the `join` contribution. */
SelectQueryContribution join(Join join) {
return join($(join));
}
/** Add to the `join` contribution. */
SelectQueryContribution addJoin(Stream join) {
return new SelectQueryContribution(fetch, from, this.join.append(join), where, offset, limit, order);
}
/** Add to the `join` contribution. */
SelectQueryContribution addJoin(Join join) {
return addJoin($(join));
}
/* -------------------------------------------------------------------------------------------------------------- */
/** Set the `where` contribution. */
SelectQueryContribution where(Opt where) {
return new SelectQueryContribution(fetch, from, join, where, offset, limit, order);
}
/** Set the `where` contribution. */
SelectQueryContribution where(@Nullable BooleanExpression where) {
return where(Opt.nul(where));
}
/** Add to the `where` contribution using boolean "and". */
SelectQueryContribution andWhere(Opt where) {
return where(JpaFns.allOf(this.where, where));
}
/** Add to the `where` contribution using boolean "and". */
SelectQueryContribution andWhere(@Nullable BooleanExpression where) {
return andWhere(Opt.nul(where));
}
/* -------------------------------------------------------------------------------------------------------------- */
/** Set the `offset` contribution. */
SelectQueryContribution offset(Opt offset) {
return new SelectQueryContribution(fetch, from, join, where, offset, limit, order);
}
/** Set the `offset` contribution. */
SelectQueryContribution offset(Integer offset) {
return offset(Opt.some(offset));
}
/** Set the `limit` contribution. */
SelectQueryContribution limit(Opt limit) {
return new SelectQueryContribution(fetch, from, join, where, offset, limit, order);
}
/** Set the `limit` contribution. */
SelectQueryContribution limit(Integer limit) {
return limit(Opt.some(limit));
}
/** Set the `order` contribution. */
SelectQueryContribution order(Stream extends OrderSpecifier>> order) {
return new SelectQueryContribution(fetch, from, join, where, offset, limit, (Stream>) order);
}
/** Add to the `order` contribution. */
SelectQueryContribution addOrder(Stream extends OrderSpecifier>> order) {
return new SelectQueryContribution(fetch, from, join, where, offset, limit, this.order.append(order));
}
static final Fn>> getFetch
= new Fn>>() {
@Override public Stream> apply(SelectQueryContribution c) {
return c.fetch;
}
};
static final Fn>> getFrom
= new Fn>>() {
@Override public Stream> apply(SelectQueryContribution c) {
return c.from;
}
};
static final Fn> getJoin
= new Fn>() {
@Override public Stream apply(SelectQueryContribution c) {
return c.join;
}
};
static final Fn> getWhere
= new Fn>() {
@Override public Opt apply(SelectQueryContribution c) {
return c.where;
}
};
static final Fn>> getOrder
= new Fn>>() {
@Override public Stream> apply(SelectQueryContribution c) {
return c.order;
}
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy