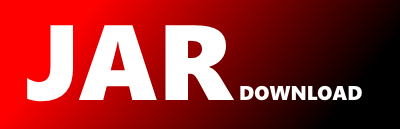
org.opencastproject.util.data.MultiMap Maven / Gradle / Ivy
/**
* Licensed to The Apereo Foundation under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional
* information regarding copyright ownership.
*
*
* The Apereo Foundation licenses this file to you under the Educational
* Community License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License
* at:
*
* http://opensource.org/licenses/ecl2.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
*/
package org.opencastproject.util.data;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public abstract class MultiMap {
private final Map> map;
public MultiMap(Map> map) {
this.map = map;
}
public static MultiMap multiHashMapWithArrayList() {
return new MultiMap(new HashMap>()) {
@Override
protected List newList() {
return new ArrayList();
}
};
}
public Map> value() {
return map;
}
public MultiMap put(A key, B value) {
List current = map.get(key);
if (current == null) {
current = newList();
map.put(key, current);
}
current.add(value);
return this;
}
public MultiMap putAll(A key, List values) {
List current = map.get(key);
if (current == null) {
current = newList();
map.put(key, current);
}
current.addAll(values);
return this;
}
protected abstract List newList();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy