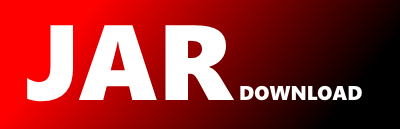
org.opencastproject.util.data.functions.Strings Maven / Gradle / Ivy
/**
* Licensed to The Apereo Foundation under one or more contributor license
* agreements. See the NOTICE file distributed with this work for additional
* information regarding copyright ownership.
*
*
* The Apereo Foundation licenses this file to you under the Educational
* Community License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License
* at:
*
* http://opensource.org/licenses/ecl2.txt
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
*/
package org.opencastproject.util.data.functions;
import static org.opencastproject.util.data.Collections.list;
import static org.opencastproject.util.data.Collections.nil;
import static org.opencastproject.util.data.Option.none;
import static org.opencastproject.util.data.Option.some;
import org.opencastproject.util.data.Function;
import org.opencastproject.util.data.Option;
import java.text.Format;
import java.util.List;
import java.util.regex.Pattern;
/** Functions for strings. */
public final class Strings {
private Strings() {
}
private static final List NIL = nil();
private static final Option NONE = none();
/**
* Trim a string and return either some
or none
if it's empty. The string may be null.
*/
public static final Function> trimToNone = new Function>() {
@Override
public Option apply(String a) {
return trimToNone(a);
}
};
/**
* Trim a string and return either some
or none
if it's empty. The string may be null.
*/
public static Option trimToNone(String a) {
if (a != null) {
final String trimmed = a.trim();
return trimmed.length() > 0 ? some(trimmed) : NONE;
} else {
return none();
}
}
/** Return a.toString()
wrapped in a some if a != null
, none otherwise. */
public static Option asString(Object a) {
return a != null ? some(a.toString()) : NONE;
}
/** Return a.toString()
wrapped in a some if a != null
, none otherwise. */
public static Function> asString() {
return new Function>() {
@Override
public Option apply(A a) {
return asString(a);
}
};
}
/** Return a.toString()
or <null>
if argument is null. */
public static Function asStringNull() {
return new Function() {
@Override
public String apply(A a) {
return a != null ? a.toString() : "";
}
};
}
/** Convert a string into a long if possible. */
public static final Function> toDouble = new Function>() {
@Override
public Option apply(String s) {
try {
return some(Double.parseDouble(s));
} catch (NumberFormatException e) {
return none();
}
}
};
/** Convert a string into an integer if possible. */
public static final Function> toInt = new Function>() {
@Override
public Option apply(String s) {
try {
return some(Integer.parseInt(s));
} catch (NumberFormatException e) {
return none();
}
}
};
/**
* Convert a string into a boolean.
*
* @see Boolean#valueOf(String)
*/
public static final Function toBool = new Function() {
@Override
public Boolean apply(String s) {
return Boolean.valueOf(s);
}
};
public static Function format(final Format f) {
return new Function() {
@Override
public String apply(A a) {
return f.format(a);
}
};
}
public static final Function> trimToNil = new Function>() {
@Override
public List apply(String a) {
if (a != null) {
final String trimmed = a.trim();
return trimmed.length() > 0 ? list(trimmed) : NIL;
} else {
return NIL;
}
}
};
/** Create a {@linkplain Pattern#split(CharSequence) split} function from a regex pattern. */
public static Function split(final Pattern splitter) {
return new Function() {
@Override
public String[] apply(String s) {
return splitter.split(s);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy