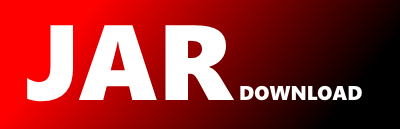
org.gel.models.participant.avro.Ancestries Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.participant.avro;
@SuppressWarnings("all")
/** Ancestries, defined as Ethnic category(ies) and Chi-square test */
@org.apache.avro.specific.AvroGenerated
public class Ancestries extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"Ancestries\",\"namespace\":\"org.gel.models.participant.avro\",\"doc\":\"Ancestries, defined as Ethnic category(ies) and Chi-square test\",\"fields\":[{\"name\":\"mothersEthnicOrigin\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"EthnicCategory\",\"doc\":\"This is the list of ethnicities in ONS16\\n\\n * `D`: Mixed: White and Black Caribbean\\n * `E`: Mixed: White and Black African\\n * `F`: Mixed: White and Asian\\n * `G`: Mixed: Any other mixed background\\n * `A`: White: British\\n * `B`: White: Irish\\n * `C`: White: Any other White background\\n * `L`: Asian or Asian British: Any other Asian background\\n * `M`: Black or Black British: Caribbean\\n * `N`: Black or Black British: African\\n * `H`: Asian or Asian British: Indian\\n * `J`: Asian or Asian British: Pakistani\\n * `K`: Asian or Asian British: Bangladeshi\\n * `P`: Black or Black British: Any other Black background\\n * `S`: Other Ethnic Groups: Any other ethnic group\\n * `R`: Other Ethnic Groups: Chinese\\n * `Z`: Not stated\",\"symbols\":[\"D\",\"E\",\"F\",\"G\",\"A\",\"B\",\"C\",\"L\",\"M\",\"N\",\"H\",\"J\",\"K\",\"P\",\"S\",\"R\",\"Z\"]}],\"doc\":\"Mother's Ethnic Origin\"},{\"name\":\"mothersOtherRelevantAncestry\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Mother's Ethnic Origin Description\"},{\"name\":\"fathersEthnicOrigin\",\"type\":[\"null\",\"EthnicCategory\"],\"doc\":\"Father's Ethnic Origin\"},{\"name\":\"fathersOtherRelevantAncestry\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Father's Ethnic Origin Description\"},{\"name\":\"chiSquare1KGenomesPhase3Pop\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"ChiSquare1KGenomesPhase3Pop\",\"doc\":\"Chi-square test for goodness of fit of this sample to 1000 Genomes Phase 3 populations\",\"fields\":[{\"name\":\"kgSuperPopCategory\",\"type\":{\"type\":\"enum\",\"name\":\"KgSuperPopCategory\",\"doc\":\"1K Genomes project super populations\",\"symbols\":[\"AFR\",\"AMR\",\"EAS\",\"EUR\",\"SAS\"]},\"doc\":\"1K Super Population\"},{\"name\":\"kgPopCategory\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"KgPopCategory\",\"doc\":\"1K Genomes project populations\",\"symbols\":[\"ACB\",\"ASW\",\"BEB\",\"CDX\",\"CEU\",\"CHB\",\"CHS\",\"CLM\",\"ESN\",\"FIN\",\"GBR\",\"GIH\",\"GWD\",\"IBS\",\"ITU\",\"JPT\",\"KHV\",\"LWK\",\"MSL\",\"MXL\",\"PEL\",\"PJL\",\"PUR\",\"STU\",\"TSI\",\"YRI\"]}],\"doc\":\"1K Population\"},{\"name\":\"chiSquare\",\"type\":\"double\",\"doc\":\"Chi-square test for goodness of fit of this sample to this 1000 Genomes Phase 3 population\"}]}}],\"doc\":\"Chi-square test for goodness of fit of this sample to 1000 Genomes Phase 3 populations\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** Mother's Ethnic Origin */
private org.gel.models.participant.avro.EthnicCategory mothersEthnicOrigin;
/** Mother's Ethnic Origin Description */
private java.lang.String mothersOtherRelevantAncestry;
/** Father's Ethnic Origin */
private org.gel.models.participant.avro.EthnicCategory fathersEthnicOrigin;
/** Father's Ethnic Origin Description */
private java.lang.String fathersOtherRelevantAncestry;
/** Chi-square test for goodness of fit of this sample to 1000 Genomes Phase 3 populations */
private java.util.List chiSquare1KGenomesPhase3Pop;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public Ancestries() {}
/**
* All-args constructor.
*/
public Ancestries(org.gel.models.participant.avro.EthnicCategory mothersEthnicOrigin, java.lang.String mothersOtherRelevantAncestry, org.gel.models.participant.avro.EthnicCategory fathersEthnicOrigin, java.lang.String fathersOtherRelevantAncestry, java.util.List chiSquare1KGenomesPhase3Pop) {
this.mothersEthnicOrigin = mothersEthnicOrigin;
this.mothersOtherRelevantAncestry = mothersOtherRelevantAncestry;
this.fathersEthnicOrigin = fathersEthnicOrigin;
this.fathersOtherRelevantAncestry = fathersOtherRelevantAncestry;
this.chiSquare1KGenomesPhase3Pop = chiSquare1KGenomesPhase3Pop;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return mothersEthnicOrigin;
case 1: return mothersOtherRelevantAncestry;
case 2: return fathersEthnicOrigin;
case 3: return fathersOtherRelevantAncestry;
case 4: return chiSquare1KGenomesPhase3Pop;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: mothersEthnicOrigin = (org.gel.models.participant.avro.EthnicCategory)value$; break;
case 1: mothersOtherRelevantAncestry = (java.lang.String)value$; break;
case 2: fathersEthnicOrigin = (org.gel.models.participant.avro.EthnicCategory)value$; break;
case 3: fathersOtherRelevantAncestry = (java.lang.String)value$; break;
case 4: chiSquare1KGenomesPhase3Pop = (java.util.List)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'mothersEthnicOrigin' field.
* Mother's Ethnic Origin */
public org.gel.models.participant.avro.EthnicCategory getMothersEthnicOrigin() {
return mothersEthnicOrigin;
}
/**
* Sets the value of the 'mothersEthnicOrigin' field.
* Mother's Ethnic Origin * @param value the value to set.
*/
public void setMothersEthnicOrigin(org.gel.models.participant.avro.EthnicCategory value) {
this.mothersEthnicOrigin = value;
}
/**
* Gets the value of the 'mothersOtherRelevantAncestry' field.
* Mother's Ethnic Origin Description */
public java.lang.String getMothersOtherRelevantAncestry() {
return mothersOtherRelevantAncestry;
}
/**
* Sets the value of the 'mothersOtherRelevantAncestry' field.
* Mother's Ethnic Origin Description * @param value the value to set.
*/
public void setMothersOtherRelevantAncestry(java.lang.String value) {
this.mothersOtherRelevantAncestry = value;
}
/**
* Gets the value of the 'fathersEthnicOrigin' field.
* Father's Ethnic Origin */
public org.gel.models.participant.avro.EthnicCategory getFathersEthnicOrigin() {
return fathersEthnicOrigin;
}
/**
* Sets the value of the 'fathersEthnicOrigin' field.
* Father's Ethnic Origin * @param value the value to set.
*/
public void setFathersEthnicOrigin(org.gel.models.participant.avro.EthnicCategory value) {
this.fathersEthnicOrigin = value;
}
/**
* Gets the value of the 'fathersOtherRelevantAncestry' field.
* Father's Ethnic Origin Description */
public java.lang.String getFathersOtherRelevantAncestry() {
return fathersOtherRelevantAncestry;
}
/**
* Sets the value of the 'fathersOtherRelevantAncestry' field.
* Father's Ethnic Origin Description * @param value the value to set.
*/
public void setFathersOtherRelevantAncestry(java.lang.String value) {
this.fathersOtherRelevantAncestry = value;
}
/**
* Gets the value of the 'chiSquare1KGenomesPhase3Pop' field.
* Chi-square test for goodness of fit of this sample to 1000 Genomes Phase 3 populations */
public java.util.List getChiSquare1KGenomesPhase3Pop() {
return chiSquare1KGenomesPhase3Pop;
}
/**
* Sets the value of the 'chiSquare1KGenomesPhase3Pop' field.
* Chi-square test for goodness of fit of this sample to 1000 Genomes Phase 3 populations * @param value the value to set.
*/
public void setChiSquare1KGenomesPhase3Pop(java.util.List value) {
this.chiSquare1KGenomesPhase3Pop = value;
}
/** Creates a new Ancestries RecordBuilder */
public static org.gel.models.participant.avro.Ancestries.Builder newBuilder() {
return new org.gel.models.participant.avro.Ancestries.Builder();
}
/** Creates a new Ancestries RecordBuilder by copying an existing Builder */
public static org.gel.models.participant.avro.Ancestries.Builder newBuilder(org.gel.models.participant.avro.Ancestries.Builder other) {
return new org.gel.models.participant.avro.Ancestries.Builder(other);
}
/** Creates a new Ancestries RecordBuilder by copying an existing Ancestries instance */
public static org.gel.models.participant.avro.Ancestries.Builder newBuilder(org.gel.models.participant.avro.Ancestries other) {
return new org.gel.models.participant.avro.Ancestries.Builder(other);
}
/**
* RecordBuilder for Ancestries instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private org.gel.models.participant.avro.EthnicCategory mothersEthnicOrigin;
private java.lang.String mothersOtherRelevantAncestry;
private org.gel.models.participant.avro.EthnicCategory fathersEthnicOrigin;
private java.lang.String fathersOtherRelevantAncestry;
private java.util.List chiSquare1KGenomesPhase3Pop;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.participant.avro.Ancestries.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.participant.avro.Ancestries.Builder other) {
super(other);
if (isValidValue(fields()[0], other.mothersEthnicOrigin)) {
this.mothersEthnicOrigin = data().deepCopy(fields()[0].schema(), other.mothersEthnicOrigin);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.mothersOtherRelevantAncestry)) {
this.mothersOtherRelevantAncestry = data().deepCopy(fields()[1].schema(), other.mothersOtherRelevantAncestry);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.fathersEthnicOrigin)) {
this.fathersEthnicOrigin = data().deepCopy(fields()[2].schema(), other.fathersEthnicOrigin);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.fathersOtherRelevantAncestry)) {
this.fathersOtherRelevantAncestry = data().deepCopy(fields()[3].schema(), other.fathersOtherRelevantAncestry);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.chiSquare1KGenomesPhase3Pop)) {
this.chiSquare1KGenomesPhase3Pop = data().deepCopy(fields()[4].schema(), other.chiSquare1KGenomesPhase3Pop);
fieldSetFlags()[4] = true;
}
}
/** Creates a Builder by copying an existing Ancestries instance */
private Builder(org.gel.models.participant.avro.Ancestries other) {
super(org.gel.models.participant.avro.Ancestries.SCHEMA$);
if (isValidValue(fields()[0], other.mothersEthnicOrigin)) {
this.mothersEthnicOrigin = data().deepCopy(fields()[0].schema(), other.mothersEthnicOrigin);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.mothersOtherRelevantAncestry)) {
this.mothersOtherRelevantAncestry = data().deepCopy(fields()[1].schema(), other.mothersOtherRelevantAncestry);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.fathersEthnicOrigin)) {
this.fathersEthnicOrigin = data().deepCopy(fields()[2].schema(), other.fathersEthnicOrigin);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.fathersOtherRelevantAncestry)) {
this.fathersOtherRelevantAncestry = data().deepCopy(fields()[3].schema(), other.fathersOtherRelevantAncestry);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.chiSquare1KGenomesPhase3Pop)) {
this.chiSquare1KGenomesPhase3Pop = data().deepCopy(fields()[4].schema(), other.chiSquare1KGenomesPhase3Pop);
fieldSetFlags()[4] = true;
}
}
/** Gets the value of the 'mothersEthnicOrigin' field */
public org.gel.models.participant.avro.EthnicCategory getMothersEthnicOrigin() {
return mothersEthnicOrigin;
}
/** Sets the value of the 'mothersEthnicOrigin' field */
public org.gel.models.participant.avro.Ancestries.Builder setMothersEthnicOrigin(org.gel.models.participant.avro.EthnicCategory value) {
validate(fields()[0], value);
this.mothersEthnicOrigin = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'mothersEthnicOrigin' field has been set */
public boolean hasMothersEthnicOrigin() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'mothersEthnicOrigin' field */
public org.gel.models.participant.avro.Ancestries.Builder clearMothersEthnicOrigin() {
mothersEthnicOrigin = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'mothersOtherRelevantAncestry' field */
public java.lang.String getMothersOtherRelevantAncestry() {
return mothersOtherRelevantAncestry;
}
/** Sets the value of the 'mothersOtherRelevantAncestry' field */
public org.gel.models.participant.avro.Ancestries.Builder setMothersOtherRelevantAncestry(java.lang.String value) {
validate(fields()[1], value);
this.mothersOtherRelevantAncestry = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'mothersOtherRelevantAncestry' field has been set */
public boolean hasMothersOtherRelevantAncestry() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'mothersOtherRelevantAncestry' field */
public org.gel.models.participant.avro.Ancestries.Builder clearMothersOtherRelevantAncestry() {
mothersOtherRelevantAncestry = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'fathersEthnicOrigin' field */
public org.gel.models.participant.avro.EthnicCategory getFathersEthnicOrigin() {
return fathersEthnicOrigin;
}
/** Sets the value of the 'fathersEthnicOrigin' field */
public org.gel.models.participant.avro.Ancestries.Builder setFathersEthnicOrigin(org.gel.models.participant.avro.EthnicCategory value) {
validate(fields()[2], value);
this.fathersEthnicOrigin = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'fathersEthnicOrigin' field has been set */
public boolean hasFathersEthnicOrigin() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'fathersEthnicOrigin' field */
public org.gel.models.participant.avro.Ancestries.Builder clearFathersEthnicOrigin() {
fathersEthnicOrigin = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'fathersOtherRelevantAncestry' field */
public java.lang.String getFathersOtherRelevantAncestry() {
return fathersOtherRelevantAncestry;
}
/** Sets the value of the 'fathersOtherRelevantAncestry' field */
public org.gel.models.participant.avro.Ancestries.Builder setFathersOtherRelevantAncestry(java.lang.String value) {
validate(fields()[3], value);
this.fathersOtherRelevantAncestry = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'fathersOtherRelevantAncestry' field has been set */
public boolean hasFathersOtherRelevantAncestry() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'fathersOtherRelevantAncestry' field */
public org.gel.models.participant.avro.Ancestries.Builder clearFathersOtherRelevantAncestry() {
fathersOtherRelevantAncestry = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'chiSquare1KGenomesPhase3Pop' field */
public java.util.List getChiSquare1KGenomesPhase3Pop() {
return chiSquare1KGenomesPhase3Pop;
}
/** Sets the value of the 'chiSquare1KGenomesPhase3Pop' field */
public org.gel.models.participant.avro.Ancestries.Builder setChiSquare1KGenomesPhase3Pop(java.util.List value) {
validate(fields()[4], value);
this.chiSquare1KGenomesPhase3Pop = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'chiSquare1KGenomesPhase3Pop' field has been set */
public boolean hasChiSquare1KGenomesPhase3Pop() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'chiSquare1KGenomesPhase3Pop' field */
public org.gel.models.participant.avro.Ancestries.Builder clearChiSquare1KGenomesPhase3Pop() {
chiSquare1KGenomesPhase3Pop = null;
fieldSetFlags()[4] = false;
return this;
}
@Override
public Ancestries build() {
try {
Ancestries record = new Ancestries();
record.mothersEthnicOrigin = fieldSetFlags()[0] ? this.mothersEthnicOrigin : (org.gel.models.participant.avro.EthnicCategory) defaultValue(fields()[0]);
record.mothersOtherRelevantAncestry = fieldSetFlags()[1] ? this.mothersOtherRelevantAncestry : (java.lang.String) defaultValue(fields()[1]);
record.fathersEthnicOrigin = fieldSetFlags()[2] ? this.fathersEthnicOrigin : (org.gel.models.participant.avro.EthnicCategory) defaultValue(fields()[2]);
record.fathersOtherRelevantAncestry = fieldSetFlags()[3] ? this.fathersOtherRelevantAncestry : (java.lang.String) defaultValue(fields()[3]);
record.chiSquare1KGenomesPhase3Pop = fieldSetFlags()[4] ? this.chiSquare1KGenomesPhase3Pop : (java.util.List) defaultValue(fields()[4]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy