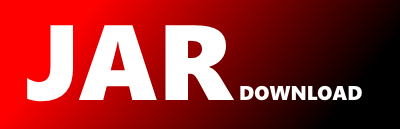
org.gel.models.participant.avro.HpoTerm Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.participant.avro;
@SuppressWarnings("all")
/** This defines an HPO term and its modifiers (possibly multiple)
If HPO term presence is unknown we don't have a entry on the list */
@org.apache.avro.specific.AvroGenerated
public class HpoTerm extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"HpoTerm\",\"namespace\":\"org.gel.models.participant.avro\",\"doc\":\"This defines an HPO term and its modifiers (possibly multiple)\\n If HPO term presence is unknown we don't have a entry on the list\",\"fields\":[{\"name\":\"term\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Identifier of the HPO term\"},{\"name\":\"termPresence\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"TernaryOption\",\"doc\":\"This defines a yes/no/unknown case\",\"symbols\":[\"yes\",\"no\",\"unknown\"]}],\"doc\":\"This is whether the term is present in the participant (default is unknown) yes=term is present in participant,\\n no=term is not present\"},{\"name\":\"hpoBuildNumber\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"hpoBuildNumber\"},{\"name\":\"modifiers\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"HpoTermModifiers\",\"doc\":\"HPO Modifiers\\n For GMS, hpoModifierCode and hpoModifierVersion will be used\",\"fields\":[{\"name\":\"laterality\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"Laterality\",\"symbols\":[\"RIGHT\",\"UNILATERAL\",\"BILATERAL\",\"LEFT\"]}]},{\"name\":\"progression\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"Progression\",\"symbols\":[\"PROGRESSIVE\",\"NONPROGRESSIVE\"]}]},{\"name\":\"severity\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"Severity\",\"symbols\":[\"BORDERLINE\",\"MILD\",\"MODERATE\",\"SEVERE\",\"PROFOUND\"]}]},{\"name\":\"spatialPattern\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"SpatialPattern\",\"symbols\":[\"DISTAL\",\"GENERALIZED\",\"LOCALIZED\",\"PROXIMAL\"]}]},{\"name\":\"hpoModifierCode\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"hpoModifierVersion\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]}]}}],\"doc\":\"Modifier associated with the HPO term\"},{\"name\":\"ageOfOnset\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"AgeOfOnset\",\"symbols\":[\"EMBRYONAL_ONSET\",\"FETAL_ONSET\",\"NEONATAL_ONSET\",\"INFANTILE_ONSET\",\"CHILDHOOD_ONSET\",\"JUVENILE_ONSET\",\"YOUNG_ADULT_ONSET\",\"LATE_ONSET\",\"MIDDLE_AGE_ONSET\"]}],\"doc\":\"Age of onset in months\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** Identifier of the HPO term */
private java.lang.String term;
/** This is whether the term is present in the participant (default is unknown) yes=term is present in participant,
no=term is not present */
private org.gel.models.participant.avro.TernaryOption termPresence;
/** hpoBuildNumber */
private java.lang.String hpoBuildNumber;
/** Modifier associated with the HPO term */
private java.util.List modifiers;
/** Age of onset in months */
private org.gel.models.participant.avro.AgeOfOnset ageOfOnset;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public HpoTerm() {}
/**
* All-args constructor.
*/
public HpoTerm(java.lang.String term, org.gel.models.participant.avro.TernaryOption termPresence, java.lang.String hpoBuildNumber, java.util.List modifiers, org.gel.models.participant.avro.AgeOfOnset ageOfOnset) {
this.term = term;
this.termPresence = termPresence;
this.hpoBuildNumber = hpoBuildNumber;
this.modifiers = modifiers;
this.ageOfOnset = ageOfOnset;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return term;
case 1: return termPresence;
case 2: return hpoBuildNumber;
case 3: return modifiers;
case 4: return ageOfOnset;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: term = (java.lang.String)value$; break;
case 1: termPresence = (org.gel.models.participant.avro.TernaryOption)value$; break;
case 2: hpoBuildNumber = (java.lang.String)value$; break;
case 3: modifiers = (java.util.List)value$; break;
case 4: ageOfOnset = (org.gel.models.participant.avro.AgeOfOnset)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'term' field.
* Identifier of the HPO term */
public java.lang.String getTerm() {
return term;
}
/**
* Sets the value of the 'term' field.
* Identifier of the HPO term * @param value the value to set.
*/
public void setTerm(java.lang.String value) {
this.term = value;
}
/**
* Gets the value of the 'termPresence' field.
* This is whether the term is present in the participant (default is unknown) yes=term is present in participant,
no=term is not present */
public org.gel.models.participant.avro.TernaryOption getTermPresence() {
return termPresence;
}
/**
* Sets the value of the 'termPresence' field.
* This is whether the term is present in the participant (default is unknown) yes=term is present in participant,
no=term is not present * @param value the value to set.
*/
public void setTermPresence(org.gel.models.participant.avro.TernaryOption value) {
this.termPresence = value;
}
/**
* Gets the value of the 'hpoBuildNumber' field.
* hpoBuildNumber */
public java.lang.String getHpoBuildNumber() {
return hpoBuildNumber;
}
/**
* Sets the value of the 'hpoBuildNumber' field.
* hpoBuildNumber * @param value the value to set.
*/
public void setHpoBuildNumber(java.lang.String value) {
this.hpoBuildNumber = value;
}
/**
* Gets the value of the 'modifiers' field.
* Modifier associated with the HPO term */
public java.util.List getModifiers() {
return modifiers;
}
/**
* Sets the value of the 'modifiers' field.
* Modifier associated with the HPO term * @param value the value to set.
*/
public void setModifiers(java.util.List value) {
this.modifiers = value;
}
/**
* Gets the value of the 'ageOfOnset' field.
* Age of onset in months */
public org.gel.models.participant.avro.AgeOfOnset getAgeOfOnset() {
return ageOfOnset;
}
/**
* Sets the value of the 'ageOfOnset' field.
* Age of onset in months * @param value the value to set.
*/
public void setAgeOfOnset(org.gel.models.participant.avro.AgeOfOnset value) {
this.ageOfOnset = value;
}
/** Creates a new HpoTerm RecordBuilder */
public static org.gel.models.participant.avro.HpoTerm.Builder newBuilder() {
return new org.gel.models.participant.avro.HpoTerm.Builder();
}
/** Creates a new HpoTerm RecordBuilder by copying an existing Builder */
public static org.gel.models.participant.avro.HpoTerm.Builder newBuilder(org.gel.models.participant.avro.HpoTerm.Builder other) {
return new org.gel.models.participant.avro.HpoTerm.Builder(other);
}
/** Creates a new HpoTerm RecordBuilder by copying an existing HpoTerm instance */
public static org.gel.models.participant.avro.HpoTerm.Builder newBuilder(org.gel.models.participant.avro.HpoTerm other) {
return new org.gel.models.participant.avro.HpoTerm.Builder(other);
}
/**
* RecordBuilder for HpoTerm instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String term;
private org.gel.models.participant.avro.TernaryOption termPresence;
private java.lang.String hpoBuildNumber;
private java.util.List modifiers;
private org.gel.models.participant.avro.AgeOfOnset ageOfOnset;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.participant.avro.HpoTerm.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.participant.avro.HpoTerm.Builder other) {
super(other);
if (isValidValue(fields()[0], other.term)) {
this.term = data().deepCopy(fields()[0].schema(), other.term);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.termPresence)) {
this.termPresence = data().deepCopy(fields()[1].schema(), other.termPresence);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.hpoBuildNumber)) {
this.hpoBuildNumber = data().deepCopy(fields()[2].schema(), other.hpoBuildNumber);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.modifiers)) {
this.modifiers = data().deepCopy(fields()[3].schema(), other.modifiers);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.ageOfOnset)) {
this.ageOfOnset = data().deepCopy(fields()[4].schema(), other.ageOfOnset);
fieldSetFlags()[4] = true;
}
}
/** Creates a Builder by copying an existing HpoTerm instance */
private Builder(org.gel.models.participant.avro.HpoTerm other) {
super(org.gel.models.participant.avro.HpoTerm.SCHEMA$);
if (isValidValue(fields()[0], other.term)) {
this.term = data().deepCopy(fields()[0].schema(), other.term);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.termPresence)) {
this.termPresence = data().deepCopy(fields()[1].schema(), other.termPresence);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.hpoBuildNumber)) {
this.hpoBuildNumber = data().deepCopy(fields()[2].schema(), other.hpoBuildNumber);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.modifiers)) {
this.modifiers = data().deepCopy(fields()[3].schema(), other.modifiers);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.ageOfOnset)) {
this.ageOfOnset = data().deepCopy(fields()[4].schema(), other.ageOfOnset);
fieldSetFlags()[4] = true;
}
}
/** Gets the value of the 'term' field */
public java.lang.String getTerm() {
return term;
}
/** Sets the value of the 'term' field */
public org.gel.models.participant.avro.HpoTerm.Builder setTerm(java.lang.String value) {
validate(fields()[0], value);
this.term = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'term' field has been set */
public boolean hasTerm() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'term' field */
public org.gel.models.participant.avro.HpoTerm.Builder clearTerm() {
term = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'termPresence' field */
public org.gel.models.participant.avro.TernaryOption getTermPresence() {
return termPresence;
}
/** Sets the value of the 'termPresence' field */
public org.gel.models.participant.avro.HpoTerm.Builder setTermPresence(org.gel.models.participant.avro.TernaryOption value) {
validate(fields()[1], value);
this.termPresence = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'termPresence' field has been set */
public boolean hasTermPresence() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'termPresence' field */
public org.gel.models.participant.avro.HpoTerm.Builder clearTermPresence() {
termPresence = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'hpoBuildNumber' field */
public java.lang.String getHpoBuildNumber() {
return hpoBuildNumber;
}
/** Sets the value of the 'hpoBuildNumber' field */
public org.gel.models.participant.avro.HpoTerm.Builder setHpoBuildNumber(java.lang.String value) {
validate(fields()[2], value);
this.hpoBuildNumber = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'hpoBuildNumber' field has been set */
public boolean hasHpoBuildNumber() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'hpoBuildNumber' field */
public org.gel.models.participant.avro.HpoTerm.Builder clearHpoBuildNumber() {
hpoBuildNumber = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'modifiers' field */
public java.util.List getModifiers() {
return modifiers;
}
/** Sets the value of the 'modifiers' field */
public org.gel.models.participant.avro.HpoTerm.Builder setModifiers(java.util.List value) {
validate(fields()[3], value);
this.modifiers = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'modifiers' field has been set */
public boolean hasModifiers() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'modifiers' field */
public org.gel.models.participant.avro.HpoTerm.Builder clearModifiers() {
modifiers = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'ageOfOnset' field */
public org.gel.models.participant.avro.AgeOfOnset getAgeOfOnset() {
return ageOfOnset;
}
/** Sets the value of the 'ageOfOnset' field */
public org.gel.models.participant.avro.HpoTerm.Builder setAgeOfOnset(org.gel.models.participant.avro.AgeOfOnset value) {
validate(fields()[4], value);
this.ageOfOnset = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'ageOfOnset' field has been set */
public boolean hasAgeOfOnset() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'ageOfOnset' field */
public org.gel.models.participant.avro.HpoTerm.Builder clearAgeOfOnset() {
ageOfOnset = null;
fieldSetFlags()[4] = false;
return this;
}
@Override
public HpoTerm build() {
try {
HpoTerm record = new HpoTerm();
record.term = fieldSetFlags()[0] ? this.term : (java.lang.String) defaultValue(fields()[0]);
record.termPresence = fieldSetFlags()[1] ? this.termPresence : (org.gel.models.participant.avro.TernaryOption) defaultValue(fields()[1]);
record.hpoBuildNumber = fieldSetFlags()[2] ? this.hpoBuildNumber : (java.lang.String) defaultValue(fields()[2]);
record.modifiers = fieldSetFlags()[3] ? this.modifiers : (java.util.List) defaultValue(fields()[3]);
record.ageOfOnset = fieldSetFlags()[4] ? this.ageOfOnset : (org.gel.models.participant.avro.AgeOfOnset) defaultValue(fields()[4]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy