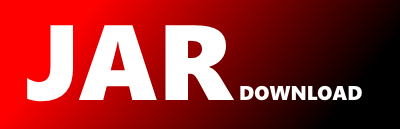
org.gel.models.participant.avro.OrganisationNgis Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.participant.avro;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class OrganisationNgis extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"OrganisationNgis\",\"namespace\":\"org.gel.models.participant.avro\",\"fields\":[{\"name\":\"organisationId\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Organisation Id\"},{\"name\":\"organisationCode\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Ods code\"},{\"name\":\"organisationName\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Organisation Name\"},{\"name\":\"organisationNationalGroupingId\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"National Grouping (GLH) Id\"},{\"name\":\"organisationNationalGroupingName\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"National Grouping (GLH) Name\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** Organisation Id */
private java.lang.String organisationId;
/** Ods code */
private java.lang.String organisationCode;
/** Organisation Name */
private java.lang.String organisationName;
/** National Grouping (GLH) Id */
private java.lang.String organisationNationalGroupingId;
/** National Grouping (GLH) Name */
private java.lang.String organisationNationalGroupingName;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public OrganisationNgis() {}
/**
* All-args constructor.
*/
public OrganisationNgis(java.lang.String organisationId, java.lang.String organisationCode, java.lang.String organisationName, java.lang.String organisationNationalGroupingId, java.lang.String organisationNationalGroupingName) {
this.organisationId = organisationId;
this.organisationCode = organisationCode;
this.organisationName = organisationName;
this.organisationNationalGroupingId = organisationNationalGroupingId;
this.organisationNationalGroupingName = organisationNationalGroupingName;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return organisationId;
case 1: return organisationCode;
case 2: return organisationName;
case 3: return organisationNationalGroupingId;
case 4: return organisationNationalGroupingName;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: organisationId = (java.lang.String)value$; break;
case 1: organisationCode = (java.lang.String)value$; break;
case 2: organisationName = (java.lang.String)value$; break;
case 3: organisationNationalGroupingId = (java.lang.String)value$; break;
case 4: organisationNationalGroupingName = (java.lang.String)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'organisationId' field.
* Organisation Id */
public java.lang.String getOrganisationId() {
return organisationId;
}
/**
* Sets the value of the 'organisationId' field.
* Organisation Id * @param value the value to set.
*/
public void setOrganisationId(java.lang.String value) {
this.organisationId = value;
}
/**
* Gets the value of the 'organisationCode' field.
* Ods code */
public java.lang.String getOrganisationCode() {
return organisationCode;
}
/**
* Sets the value of the 'organisationCode' field.
* Ods code * @param value the value to set.
*/
public void setOrganisationCode(java.lang.String value) {
this.organisationCode = value;
}
/**
* Gets the value of the 'organisationName' field.
* Organisation Name */
public java.lang.String getOrganisationName() {
return organisationName;
}
/**
* Sets the value of the 'organisationName' field.
* Organisation Name * @param value the value to set.
*/
public void setOrganisationName(java.lang.String value) {
this.organisationName = value;
}
/**
* Gets the value of the 'organisationNationalGroupingId' field.
* National Grouping (GLH) Id */
public java.lang.String getOrganisationNationalGroupingId() {
return organisationNationalGroupingId;
}
/**
* Sets the value of the 'organisationNationalGroupingId' field.
* National Grouping (GLH) Id * @param value the value to set.
*/
public void setOrganisationNationalGroupingId(java.lang.String value) {
this.organisationNationalGroupingId = value;
}
/**
* Gets the value of the 'organisationNationalGroupingName' field.
* National Grouping (GLH) Name */
public java.lang.String getOrganisationNationalGroupingName() {
return organisationNationalGroupingName;
}
/**
* Sets the value of the 'organisationNationalGroupingName' field.
* National Grouping (GLH) Name * @param value the value to set.
*/
public void setOrganisationNationalGroupingName(java.lang.String value) {
this.organisationNationalGroupingName = value;
}
/** Creates a new OrganisationNgis RecordBuilder */
public static org.gel.models.participant.avro.OrganisationNgis.Builder newBuilder() {
return new org.gel.models.participant.avro.OrganisationNgis.Builder();
}
/** Creates a new OrganisationNgis RecordBuilder by copying an existing Builder */
public static org.gel.models.participant.avro.OrganisationNgis.Builder newBuilder(org.gel.models.participant.avro.OrganisationNgis.Builder other) {
return new org.gel.models.participant.avro.OrganisationNgis.Builder(other);
}
/** Creates a new OrganisationNgis RecordBuilder by copying an existing OrganisationNgis instance */
public static org.gel.models.participant.avro.OrganisationNgis.Builder newBuilder(org.gel.models.participant.avro.OrganisationNgis other) {
return new org.gel.models.participant.avro.OrganisationNgis.Builder(other);
}
/**
* RecordBuilder for OrganisationNgis instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String organisationId;
private java.lang.String organisationCode;
private java.lang.String organisationName;
private java.lang.String organisationNationalGroupingId;
private java.lang.String organisationNationalGroupingName;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.participant.avro.OrganisationNgis.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.participant.avro.OrganisationNgis.Builder other) {
super(other);
if (isValidValue(fields()[0], other.organisationId)) {
this.organisationId = data().deepCopy(fields()[0].schema(), other.organisationId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.organisationCode)) {
this.organisationCode = data().deepCopy(fields()[1].schema(), other.organisationCode);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.organisationName)) {
this.organisationName = data().deepCopy(fields()[2].schema(), other.organisationName);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.organisationNationalGroupingId)) {
this.organisationNationalGroupingId = data().deepCopy(fields()[3].schema(), other.organisationNationalGroupingId);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.organisationNationalGroupingName)) {
this.organisationNationalGroupingName = data().deepCopy(fields()[4].schema(), other.organisationNationalGroupingName);
fieldSetFlags()[4] = true;
}
}
/** Creates a Builder by copying an existing OrganisationNgis instance */
private Builder(org.gel.models.participant.avro.OrganisationNgis other) {
super(org.gel.models.participant.avro.OrganisationNgis.SCHEMA$);
if (isValidValue(fields()[0], other.organisationId)) {
this.organisationId = data().deepCopy(fields()[0].schema(), other.organisationId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.organisationCode)) {
this.organisationCode = data().deepCopy(fields()[1].schema(), other.organisationCode);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.organisationName)) {
this.organisationName = data().deepCopy(fields()[2].schema(), other.organisationName);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.organisationNationalGroupingId)) {
this.organisationNationalGroupingId = data().deepCopy(fields()[3].schema(), other.organisationNationalGroupingId);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.organisationNationalGroupingName)) {
this.organisationNationalGroupingName = data().deepCopy(fields()[4].schema(), other.organisationNationalGroupingName);
fieldSetFlags()[4] = true;
}
}
/** Gets the value of the 'organisationId' field */
public java.lang.String getOrganisationId() {
return organisationId;
}
/** Sets the value of the 'organisationId' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder setOrganisationId(java.lang.String value) {
validate(fields()[0], value);
this.organisationId = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'organisationId' field has been set */
public boolean hasOrganisationId() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'organisationId' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder clearOrganisationId() {
organisationId = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'organisationCode' field */
public java.lang.String getOrganisationCode() {
return organisationCode;
}
/** Sets the value of the 'organisationCode' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder setOrganisationCode(java.lang.String value) {
validate(fields()[1], value);
this.organisationCode = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'organisationCode' field has been set */
public boolean hasOrganisationCode() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'organisationCode' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder clearOrganisationCode() {
organisationCode = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'organisationName' field */
public java.lang.String getOrganisationName() {
return organisationName;
}
/** Sets the value of the 'organisationName' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder setOrganisationName(java.lang.String value) {
validate(fields()[2], value);
this.organisationName = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'organisationName' field has been set */
public boolean hasOrganisationName() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'organisationName' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder clearOrganisationName() {
organisationName = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'organisationNationalGroupingId' field */
public java.lang.String getOrganisationNationalGroupingId() {
return organisationNationalGroupingId;
}
/** Sets the value of the 'organisationNationalGroupingId' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder setOrganisationNationalGroupingId(java.lang.String value) {
validate(fields()[3], value);
this.organisationNationalGroupingId = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'organisationNationalGroupingId' field has been set */
public boolean hasOrganisationNationalGroupingId() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'organisationNationalGroupingId' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder clearOrganisationNationalGroupingId() {
organisationNationalGroupingId = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'organisationNationalGroupingName' field */
public java.lang.String getOrganisationNationalGroupingName() {
return organisationNationalGroupingName;
}
/** Sets the value of the 'organisationNationalGroupingName' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder setOrganisationNationalGroupingName(java.lang.String value) {
validate(fields()[4], value);
this.organisationNationalGroupingName = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'organisationNationalGroupingName' field has been set */
public boolean hasOrganisationNationalGroupingName() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'organisationNationalGroupingName' field */
public org.gel.models.participant.avro.OrganisationNgis.Builder clearOrganisationNationalGroupingName() {
organisationNationalGroupingName = null;
fieldSetFlags()[4] = false;
return this;
}
@Override
public OrganisationNgis build() {
try {
OrganisationNgis record = new OrganisationNgis();
record.organisationId = fieldSetFlags()[0] ? this.organisationId : (java.lang.String) defaultValue(fields()[0]);
record.organisationCode = fieldSetFlags()[1] ? this.organisationCode : (java.lang.String) defaultValue(fields()[1]);
record.organisationName = fieldSetFlags()[2] ? this.organisationName : (java.lang.String) defaultValue(fields()[2]);
record.organisationNationalGroupingId = fieldSetFlags()[3] ? this.organisationNationalGroupingId : (java.lang.String) defaultValue(fields()[3]);
record.organisationNationalGroupingName = fieldSetFlags()[4] ? this.organisationNationalGroupingName : (java.lang.String) defaultValue(fields()[4]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy