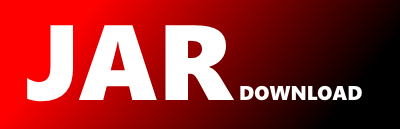
org.gel.models.participant.avro.Tumour Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.participant.avro;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class Tumour extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"Tumour\",\"namespace\":\"org.gel.models.participant.avro\",\"fields\":[{\"name\":\"tumourId\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"TumourId in GMS\"},{\"name\":\"tumourLocalId\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Local hospital tumour ID from the GLH Laboratory Information Management System (LIMS) in GMS\"},{\"name\":\"tumourType\",\"type\":{\"type\":\"enum\",\"name\":\"TumourType\",\"doc\":\"NOTE: This has been changed completely, the previous tumour type has been split into TumourPresentation and PrimaryOrMetastatic\",\"symbols\":[\"BRAIN_TUMOUR\",\"HAEMATOLOGICAL_MALIGNANCY_SOLID_SAMPLE\",\"HAEMATOLOGICAL_MALIGNANCY_LIQUID_SAMPLE\",\"SOLID_TUMOUR_METASTATIC\",\"SOLID_TUMOUR_PRIMARY\",\"SOLID_TUMOUR\",\"UNKNOWN\"]},\"doc\":\"tumourType\"},{\"name\":\"tumourParentId\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Parent Tumour UID if this tumour is metastatic\"},{\"name\":\"tumourDiagnosisDate\",\"type\":[\"null\",{\"type\":\"record\",\"name\":\"Date\",\"doc\":\"This defines a date record\",\"fields\":[{\"name\":\"year\",\"type\":\"int\",\"doc\":\"Format YYYY\"},{\"name\":\"month\",\"type\":[\"null\",\"int\"],\"doc\":\"Format MM. e.g June is 06\"},{\"name\":\"day\",\"type\":[\"null\",\"int\"],\"doc\":\"Format DD e.g. 12th of October is 12\"}]}],\"doc\":\"Date of Diagnosis of the specific tumour\"},{\"name\":\"tumourDescription\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Description of the tumour\"},{\"name\":\"tumourMorphologies\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"Morphology\",\"fields\":[{\"name\":\"id\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The ontology term id or accession in OBO format ${ONTOLOGY_ID}:${TERM_ID} (http://www.obofoundry.org/id-policy.html)\"},{\"name\":\"name\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The ontology term name\"},{\"name\":\"value\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Optional value for the ontology term, the type of the value is not checked\\n (i.e.: we could set the pvalue term to \\\"significant\\\" or to \\\"0.0001\\\")\"},{\"name\":\"version\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Ontology version\"}]}}],\"doc\":\"Morphology of the tumour\"},{\"name\":\"tumourTopographies\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"Topography\",\"fields\":[{\"name\":\"id\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The ontology term id or accession in OBO format ${ONTOLOGY_ID}:${TERM_ID} (http://www.obofoundry.org/id-policy.html)\"},{\"name\":\"name\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The ontology term name\"},{\"name\":\"value\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Optional value for the ontology term, the type of the value is not checked\\n (i.e.: we could set the pvalue term to \\\"significant\\\" or to \\\"0.0001\\\")\"},{\"name\":\"version\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Ontology version\"}]}}],\"doc\":\"Topography of the tumour\"},{\"name\":\"tumourPrimaryTopographies\",\"type\":[\"null\",{\"type\":\"array\",\"items\":\"Topography\"}],\"doc\":\"Associated primary topography for metastatic tumours\"},{\"name\":\"tumourGrade\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Grade of the Tumour\"},{\"name\":\"tumourStage\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Stage of the Tumour\"},{\"name\":\"tumourPrognosticScore\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Prognostic Score of the Tumour\"},{\"name\":\"tumourPresentation\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"TumourPresentation\",\"symbols\":[\"FIRST_PRESENTATION\",\"RECURRENCE\",\"UNKNOWN\"]}],\"doc\":\"In GMS, tumour presentation\"},{\"name\":\"primaryOrMetastatic\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"PrimaryOrMetastatic\",\"symbols\":[\"PRIMARY\",\"METASTATIC\",\"UNKNOWN\",\"NOT_APPLICABLE\"]}],\"doc\":\"In GMS, primary or metastatic\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** TumourId in GMS */
private java.lang.String tumourId;
/** Local hospital tumour ID from the GLH Laboratory Information Management System (LIMS) in GMS */
private java.lang.String tumourLocalId;
/** tumourType */
private org.gel.models.participant.avro.TumourType tumourType;
/** Parent Tumour UID if this tumour is metastatic */
private java.lang.String tumourParentId;
/** Date of Diagnosis of the specific tumour */
private org.gel.models.participant.avro.Date tumourDiagnosisDate;
/** Description of the tumour */
private java.lang.String tumourDescription;
/** Morphology of the tumour */
private java.util.List tumourMorphologies;
/** Topography of the tumour */
private java.util.List tumourTopographies;
/** Associated primary topography for metastatic tumours */
private java.util.List tumourPrimaryTopographies;
/** Grade of the Tumour */
private java.lang.String tumourGrade;
/** Stage of the Tumour */
private java.lang.String tumourStage;
/** Prognostic Score of the Tumour */
private java.lang.String tumourPrognosticScore;
/** In GMS, tumour presentation */
private org.gel.models.participant.avro.TumourPresentation tumourPresentation;
/** In GMS, primary or metastatic */
private org.gel.models.participant.avro.PrimaryOrMetastatic primaryOrMetastatic;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public Tumour() {}
/**
* All-args constructor.
*/
public Tumour(java.lang.String tumourId, java.lang.String tumourLocalId, org.gel.models.participant.avro.TumourType tumourType, java.lang.String tumourParentId, org.gel.models.participant.avro.Date tumourDiagnosisDate, java.lang.String tumourDescription, java.util.List tumourMorphologies, java.util.List tumourTopographies, java.util.List tumourPrimaryTopographies, java.lang.String tumourGrade, java.lang.String tumourStage, java.lang.String tumourPrognosticScore, org.gel.models.participant.avro.TumourPresentation tumourPresentation, org.gel.models.participant.avro.PrimaryOrMetastatic primaryOrMetastatic) {
this.tumourId = tumourId;
this.tumourLocalId = tumourLocalId;
this.tumourType = tumourType;
this.tumourParentId = tumourParentId;
this.tumourDiagnosisDate = tumourDiagnosisDate;
this.tumourDescription = tumourDescription;
this.tumourMorphologies = tumourMorphologies;
this.tumourTopographies = tumourTopographies;
this.tumourPrimaryTopographies = tumourPrimaryTopographies;
this.tumourGrade = tumourGrade;
this.tumourStage = tumourStage;
this.tumourPrognosticScore = tumourPrognosticScore;
this.tumourPresentation = tumourPresentation;
this.primaryOrMetastatic = primaryOrMetastatic;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return tumourId;
case 1: return tumourLocalId;
case 2: return tumourType;
case 3: return tumourParentId;
case 4: return tumourDiagnosisDate;
case 5: return tumourDescription;
case 6: return tumourMorphologies;
case 7: return tumourTopographies;
case 8: return tumourPrimaryTopographies;
case 9: return tumourGrade;
case 10: return tumourStage;
case 11: return tumourPrognosticScore;
case 12: return tumourPresentation;
case 13: return primaryOrMetastatic;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: tumourId = (java.lang.String)value$; break;
case 1: tumourLocalId = (java.lang.String)value$; break;
case 2: tumourType = (org.gel.models.participant.avro.TumourType)value$; break;
case 3: tumourParentId = (java.lang.String)value$; break;
case 4: tumourDiagnosisDate = (org.gel.models.participant.avro.Date)value$; break;
case 5: tumourDescription = (java.lang.String)value$; break;
case 6: tumourMorphologies = (java.util.List)value$; break;
case 7: tumourTopographies = (java.util.List)value$; break;
case 8: tumourPrimaryTopographies = (java.util.List)value$; break;
case 9: tumourGrade = (java.lang.String)value$; break;
case 10: tumourStage = (java.lang.String)value$; break;
case 11: tumourPrognosticScore = (java.lang.String)value$; break;
case 12: tumourPresentation = (org.gel.models.participant.avro.TumourPresentation)value$; break;
case 13: primaryOrMetastatic = (org.gel.models.participant.avro.PrimaryOrMetastatic)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'tumourId' field.
* TumourId in GMS */
public java.lang.String getTumourId() {
return tumourId;
}
/**
* Sets the value of the 'tumourId' field.
* TumourId in GMS * @param value the value to set.
*/
public void setTumourId(java.lang.String value) {
this.tumourId = value;
}
/**
* Gets the value of the 'tumourLocalId' field.
* Local hospital tumour ID from the GLH Laboratory Information Management System (LIMS) in GMS */
public java.lang.String getTumourLocalId() {
return tumourLocalId;
}
/**
* Sets the value of the 'tumourLocalId' field.
* Local hospital tumour ID from the GLH Laboratory Information Management System (LIMS) in GMS * @param value the value to set.
*/
public void setTumourLocalId(java.lang.String value) {
this.tumourLocalId = value;
}
/**
* Gets the value of the 'tumourType' field.
* tumourType */
public org.gel.models.participant.avro.TumourType getTumourType() {
return tumourType;
}
/**
* Sets the value of the 'tumourType' field.
* tumourType * @param value the value to set.
*/
public void setTumourType(org.gel.models.participant.avro.TumourType value) {
this.tumourType = value;
}
/**
* Gets the value of the 'tumourParentId' field.
* Parent Tumour UID if this tumour is metastatic */
public java.lang.String getTumourParentId() {
return tumourParentId;
}
/**
* Sets the value of the 'tumourParentId' field.
* Parent Tumour UID if this tumour is metastatic * @param value the value to set.
*/
public void setTumourParentId(java.lang.String value) {
this.tumourParentId = value;
}
/**
* Gets the value of the 'tumourDiagnosisDate' field.
* Date of Diagnosis of the specific tumour */
public org.gel.models.participant.avro.Date getTumourDiagnosisDate() {
return tumourDiagnosisDate;
}
/**
* Sets the value of the 'tumourDiagnosisDate' field.
* Date of Diagnosis of the specific tumour * @param value the value to set.
*/
public void setTumourDiagnosisDate(org.gel.models.participant.avro.Date value) {
this.tumourDiagnosisDate = value;
}
/**
* Gets the value of the 'tumourDescription' field.
* Description of the tumour */
public java.lang.String getTumourDescription() {
return tumourDescription;
}
/**
* Sets the value of the 'tumourDescription' field.
* Description of the tumour * @param value the value to set.
*/
public void setTumourDescription(java.lang.String value) {
this.tumourDescription = value;
}
/**
* Gets the value of the 'tumourMorphologies' field.
* Morphology of the tumour */
public java.util.List getTumourMorphologies() {
return tumourMorphologies;
}
/**
* Sets the value of the 'tumourMorphologies' field.
* Morphology of the tumour * @param value the value to set.
*/
public void setTumourMorphologies(java.util.List value) {
this.tumourMorphologies = value;
}
/**
* Gets the value of the 'tumourTopographies' field.
* Topography of the tumour */
public java.util.List getTumourTopographies() {
return tumourTopographies;
}
/**
* Sets the value of the 'tumourTopographies' field.
* Topography of the tumour * @param value the value to set.
*/
public void setTumourTopographies(java.util.List value) {
this.tumourTopographies = value;
}
/**
* Gets the value of the 'tumourPrimaryTopographies' field.
* Associated primary topography for metastatic tumours */
public java.util.List getTumourPrimaryTopographies() {
return tumourPrimaryTopographies;
}
/**
* Sets the value of the 'tumourPrimaryTopographies' field.
* Associated primary topography for metastatic tumours * @param value the value to set.
*/
public void setTumourPrimaryTopographies(java.util.List value) {
this.tumourPrimaryTopographies = value;
}
/**
* Gets the value of the 'tumourGrade' field.
* Grade of the Tumour */
public java.lang.String getTumourGrade() {
return tumourGrade;
}
/**
* Sets the value of the 'tumourGrade' field.
* Grade of the Tumour * @param value the value to set.
*/
public void setTumourGrade(java.lang.String value) {
this.tumourGrade = value;
}
/**
* Gets the value of the 'tumourStage' field.
* Stage of the Tumour */
public java.lang.String getTumourStage() {
return tumourStage;
}
/**
* Sets the value of the 'tumourStage' field.
* Stage of the Tumour * @param value the value to set.
*/
public void setTumourStage(java.lang.String value) {
this.tumourStage = value;
}
/**
* Gets the value of the 'tumourPrognosticScore' field.
* Prognostic Score of the Tumour */
public java.lang.String getTumourPrognosticScore() {
return tumourPrognosticScore;
}
/**
* Sets the value of the 'tumourPrognosticScore' field.
* Prognostic Score of the Tumour * @param value the value to set.
*/
public void setTumourPrognosticScore(java.lang.String value) {
this.tumourPrognosticScore = value;
}
/**
* Gets the value of the 'tumourPresentation' field.
* In GMS, tumour presentation */
public org.gel.models.participant.avro.TumourPresentation getTumourPresentation() {
return tumourPresentation;
}
/**
* Sets the value of the 'tumourPresentation' field.
* In GMS, tumour presentation * @param value the value to set.
*/
public void setTumourPresentation(org.gel.models.participant.avro.TumourPresentation value) {
this.tumourPresentation = value;
}
/**
* Gets the value of the 'primaryOrMetastatic' field.
* In GMS, primary or metastatic */
public org.gel.models.participant.avro.PrimaryOrMetastatic getPrimaryOrMetastatic() {
return primaryOrMetastatic;
}
/**
* Sets the value of the 'primaryOrMetastatic' field.
* In GMS, primary or metastatic * @param value the value to set.
*/
public void setPrimaryOrMetastatic(org.gel.models.participant.avro.PrimaryOrMetastatic value) {
this.primaryOrMetastatic = value;
}
/** Creates a new Tumour RecordBuilder */
public static org.gel.models.participant.avro.Tumour.Builder newBuilder() {
return new org.gel.models.participant.avro.Tumour.Builder();
}
/** Creates a new Tumour RecordBuilder by copying an existing Builder */
public static org.gel.models.participant.avro.Tumour.Builder newBuilder(org.gel.models.participant.avro.Tumour.Builder other) {
return new org.gel.models.participant.avro.Tumour.Builder(other);
}
/** Creates a new Tumour RecordBuilder by copying an existing Tumour instance */
public static org.gel.models.participant.avro.Tumour.Builder newBuilder(org.gel.models.participant.avro.Tumour other) {
return new org.gel.models.participant.avro.Tumour.Builder(other);
}
/**
* RecordBuilder for Tumour instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String tumourId;
private java.lang.String tumourLocalId;
private org.gel.models.participant.avro.TumourType tumourType;
private java.lang.String tumourParentId;
private org.gel.models.participant.avro.Date tumourDiagnosisDate;
private java.lang.String tumourDescription;
private java.util.List tumourMorphologies;
private java.util.List tumourTopographies;
private java.util.List tumourPrimaryTopographies;
private java.lang.String tumourGrade;
private java.lang.String tumourStage;
private java.lang.String tumourPrognosticScore;
private org.gel.models.participant.avro.TumourPresentation tumourPresentation;
private org.gel.models.participant.avro.PrimaryOrMetastatic primaryOrMetastatic;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.participant.avro.Tumour.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.participant.avro.Tumour.Builder other) {
super(other);
if (isValidValue(fields()[0], other.tumourId)) {
this.tumourId = data().deepCopy(fields()[0].schema(), other.tumourId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.tumourLocalId)) {
this.tumourLocalId = data().deepCopy(fields()[1].schema(), other.tumourLocalId);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.tumourType)) {
this.tumourType = data().deepCopy(fields()[2].schema(), other.tumourType);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.tumourParentId)) {
this.tumourParentId = data().deepCopy(fields()[3].schema(), other.tumourParentId);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.tumourDiagnosisDate)) {
this.tumourDiagnosisDate = data().deepCopy(fields()[4].schema(), other.tumourDiagnosisDate);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.tumourDescription)) {
this.tumourDescription = data().deepCopy(fields()[5].schema(), other.tumourDescription);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.tumourMorphologies)) {
this.tumourMorphologies = data().deepCopy(fields()[6].schema(), other.tumourMorphologies);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.tumourTopographies)) {
this.tumourTopographies = data().deepCopy(fields()[7].schema(), other.tumourTopographies);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.tumourPrimaryTopographies)) {
this.tumourPrimaryTopographies = data().deepCopy(fields()[8].schema(), other.tumourPrimaryTopographies);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.tumourGrade)) {
this.tumourGrade = data().deepCopy(fields()[9].schema(), other.tumourGrade);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.tumourStage)) {
this.tumourStage = data().deepCopy(fields()[10].schema(), other.tumourStage);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.tumourPrognosticScore)) {
this.tumourPrognosticScore = data().deepCopy(fields()[11].schema(), other.tumourPrognosticScore);
fieldSetFlags()[11] = true;
}
if (isValidValue(fields()[12], other.tumourPresentation)) {
this.tumourPresentation = data().deepCopy(fields()[12].schema(), other.tumourPresentation);
fieldSetFlags()[12] = true;
}
if (isValidValue(fields()[13], other.primaryOrMetastatic)) {
this.primaryOrMetastatic = data().deepCopy(fields()[13].schema(), other.primaryOrMetastatic);
fieldSetFlags()[13] = true;
}
}
/** Creates a Builder by copying an existing Tumour instance */
private Builder(org.gel.models.participant.avro.Tumour other) {
super(org.gel.models.participant.avro.Tumour.SCHEMA$);
if (isValidValue(fields()[0], other.tumourId)) {
this.tumourId = data().deepCopy(fields()[0].schema(), other.tumourId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.tumourLocalId)) {
this.tumourLocalId = data().deepCopy(fields()[1].schema(), other.tumourLocalId);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.tumourType)) {
this.tumourType = data().deepCopy(fields()[2].schema(), other.tumourType);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.tumourParentId)) {
this.tumourParentId = data().deepCopy(fields()[3].schema(), other.tumourParentId);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.tumourDiagnosisDate)) {
this.tumourDiagnosisDate = data().deepCopy(fields()[4].schema(), other.tumourDiagnosisDate);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.tumourDescription)) {
this.tumourDescription = data().deepCopy(fields()[5].schema(), other.tumourDescription);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.tumourMorphologies)) {
this.tumourMorphologies = data().deepCopy(fields()[6].schema(), other.tumourMorphologies);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.tumourTopographies)) {
this.tumourTopographies = data().deepCopy(fields()[7].schema(), other.tumourTopographies);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.tumourPrimaryTopographies)) {
this.tumourPrimaryTopographies = data().deepCopy(fields()[8].schema(), other.tumourPrimaryTopographies);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.tumourGrade)) {
this.tumourGrade = data().deepCopy(fields()[9].schema(), other.tumourGrade);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.tumourStage)) {
this.tumourStage = data().deepCopy(fields()[10].schema(), other.tumourStage);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.tumourPrognosticScore)) {
this.tumourPrognosticScore = data().deepCopy(fields()[11].schema(), other.tumourPrognosticScore);
fieldSetFlags()[11] = true;
}
if (isValidValue(fields()[12], other.tumourPresentation)) {
this.tumourPresentation = data().deepCopy(fields()[12].schema(), other.tumourPresentation);
fieldSetFlags()[12] = true;
}
if (isValidValue(fields()[13], other.primaryOrMetastatic)) {
this.primaryOrMetastatic = data().deepCopy(fields()[13].schema(), other.primaryOrMetastatic);
fieldSetFlags()[13] = true;
}
}
/** Gets the value of the 'tumourId' field */
public java.lang.String getTumourId() {
return tumourId;
}
/** Sets the value of the 'tumourId' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourId(java.lang.String value) {
validate(fields()[0], value);
this.tumourId = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'tumourId' field has been set */
public boolean hasTumourId() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'tumourId' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourId() {
tumourId = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'tumourLocalId' field */
public java.lang.String getTumourLocalId() {
return tumourLocalId;
}
/** Sets the value of the 'tumourLocalId' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourLocalId(java.lang.String value) {
validate(fields()[1], value);
this.tumourLocalId = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'tumourLocalId' field has been set */
public boolean hasTumourLocalId() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'tumourLocalId' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourLocalId() {
tumourLocalId = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'tumourType' field */
public org.gel.models.participant.avro.TumourType getTumourType() {
return tumourType;
}
/** Sets the value of the 'tumourType' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourType(org.gel.models.participant.avro.TumourType value) {
validate(fields()[2], value);
this.tumourType = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'tumourType' field has been set */
public boolean hasTumourType() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'tumourType' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourType() {
tumourType = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'tumourParentId' field */
public java.lang.String getTumourParentId() {
return tumourParentId;
}
/** Sets the value of the 'tumourParentId' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourParentId(java.lang.String value) {
validate(fields()[3], value);
this.tumourParentId = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'tumourParentId' field has been set */
public boolean hasTumourParentId() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'tumourParentId' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourParentId() {
tumourParentId = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'tumourDiagnosisDate' field */
public org.gel.models.participant.avro.Date getTumourDiagnosisDate() {
return tumourDiagnosisDate;
}
/** Sets the value of the 'tumourDiagnosisDate' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourDiagnosisDate(org.gel.models.participant.avro.Date value) {
validate(fields()[4], value);
this.tumourDiagnosisDate = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'tumourDiagnosisDate' field has been set */
public boolean hasTumourDiagnosisDate() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'tumourDiagnosisDate' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourDiagnosisDate() {
tumourDiagnosisDate = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'tumourDescription' field */
public java.lang.String getTumourDescription() {
return tumourDescription;
}
/** Sets the value of the 'tumourDescription' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourDescription(java.lang.String value) {
validate(fields()[5], value);
this.tumourDescription = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'tumourDescription' field has been set */
public boolean hasTumourDescription() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'tumourDescription' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourDescription() {
tumourDescription = null;
fieldSetFlags()[5] = false;
return this;
}
/** Gets the value of the 'tumourMorphologies' field */
public java.util.List getTumourMorphologies() {
return tumourMorphologies;
}
/** Sets the value of the 'tumourMorphologies' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourMorphologies(java.util.List value) {
validate(fields()[6], value);
this.tumourMorphologies = value;
fieldSetFlags()[6] = true;
return this;
}
/** Checks whether the 'tumourMorphologies' field has been set */
public boolean hasTumourMorphologies() {
return fieldSetFlags()[6];
}
/** Clears the value of the 'tumourMorphologies' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourMorphologies() {
tumourMorphologies = null;
fieldSetFlags()[6] = false;
return this;
}
/** Gets the value of the 'tumourTopographies' field */
public java.util.List getTumourTopographies() {
return tumourTopographies;
}
/** Sets the value of the 'tumourTopographies' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourTopographies(java.util.List value) {
validate(fields()[7], value);
this.tumourTopographies = value;
fieldSetFlags()[7] = true;
return this;
}
/** Checks whether the 'tumourTopographies' field has been set */
public boolean hasTumourTopographies() {
return fieldSetFlags()[7];
}
/** Clears the value of the 'tumourTopographies' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourTopographies() {
tumourTopographies = null;
fieldSetFlags()[7] = false;
return this;
}
/** Gets the value of the 'tumourPrimaryTopographies' field */
public java.util.List getTumourPrimaryTopographies() {
return tumourPrimaryTopographies;
}
/** Sets the value of the 'tumourPrimaryTopographies' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourPrimaryTopographies(java.util.List value) {
validate(fields()[8], value);
this.tumourPrimaryTopographies = value;
fieldSetFlags()[8] = true;
return this;
}
/** Checks whether the 'tumourPrimaryTopographies' field has been set */
public boolean hasTumourPrimaryTopographies() {
return fieldSetFlags()[8];
}
/** Clears the value of the 'tumourPrimaryTopographies' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourPrimaryTopographies() {
tumourPrimaryTopographies = null;
fieldSetFlags()[8] = false;
return this;
}
/** Gets the value of the 'tumourGrade' field */
public java.lang.String getTumourGrade() {
return tumourGrade;
}
/** Sets the value of the 'tumourGrade' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourGrade(java.lang.String value) {
validate(fields()[9], value);
this.tumourGrade = value;
fieldSetFlags()[9] = true;
return this;
}
/** Checks whether the 'tumourGrade' field has been set */
public boolean hasTumourGrade() {
return fieldSetFlags()[9];
}
/** Clears the value of the 'tumourGrade' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourGrade() {
tumourGrade = null;
fieldSetFlags()[9] = false;
return this;
}
/** Gets the value of the 'tumourStage' field */
public java.lang.String getTumourStage() {
return tumourStage;
}
/** Sets the value of the 'tumourStage' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourStage(java.lang.String value) {
validate(fields()[10], value);
this.tumourStage = value;
fieldSetFlags()[10] = true;
return this;
}
/** Checks whether the 'tumourStage' field has been set */
public boolean hasTumourStage() {
return fieldSetFlags()[10];
}
/** Clears the value of the 'tumourStage' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourStage() {
tumourStage = null;
fieldSetFlags()[10] = false;
return this;
}
/** Gets the value of the 'tumourPrognosticScore' field */
public java.lang.String getTumourPrognosticScore() {
return tumourPrognosticScore;
}
/** Sets the value of the 'tumourPrognosticScore' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourPrognosticScore(java.lang.String value) {
validate(fields()[11], value);
this.tumourPrognosticScore = value;
fieldSetFlags()[11] = true;
return this;
}
/** Checks whether the 'tumourPrognosticScore' field has been set */
public boolean hasTumourPrognosticScore() {
return fieldSetFlags()[11];
}
/** Clears the value of the 'tumourPrognosticScore' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourPrognosticScore() {
tumourPrognosticScore = null;
fieldSetFlags()[11] = false;
return this;
}
/** Gets the value of the 'tumourPresentation' field */
public org.gel.models.participant.avro.TumourPresentation getTumourPresentation() {
return tumourPresentation;
}
/** Sets the value of the 'tumourPresentation' field */
public org.gel.models.participant.avro.Tumour.Builder setTumourPresentation(org.gel.models.participant.avro.TumourPresentation value) {
validate(fields()[12], value);
this.tumourPresentation = value;
fieldSetFlags()[12] = true;
return this;
}
/** Checks whether the 'tumourPresentation' field has been set */
public boolean hasTumourPresentation() {
return fieldSetFlags()[12];
}
/** Clears the value of the 'tumourPresentation' field */
public org.gel.models.participant.avro.Tumour.Builder clearTumourPresentation() {
tumourPresentation = null;
fieldSetFlags()[12] = false;
return this;
}
/** Gets the value of the 'primaryOrMetastatic' field */
public org.gel.models.participant.avro.PrimaryOrMetastatic getPrimaryOrMetastatic() {
return primaryOrMetastatic;
}
/** Sets the value of the 'primaryOrMetastatic' field */
public org.gel.models.participant.avro.Tumour.Builder setPrimaryOrMetastatic(org.gel.models.participant.avro.PrimaryOrMetastatic value) {
validate(fields()[13], value);
this.primaryOrMetastatic = value;
fieldSetFlags()[13] = true;
return this;
}
/** Checks whether the 'primaryOrMetastatic' field has been set */
public boolean hasPrimaryOrMetastatic() {
return fieldSetFlags()[13];
}
/** Clears the value of the 'primaryOrMetastatic' field */
public org.gel.models.participant.avro.Tumour.Builder clearPrimaryOrMetastatic() {
primaryOrMetastatic = null;
fieldSetFlags()[13] = false;
return this;
}
@Override
public Tumour build() {
try {
Tumour record = new Tumour();
record.tumourId = fieldSetFlags()[0] ? this.tumourId : (java.lang.String) defaultValue(fields()[0]);
record.tumourLocalId = fieldSetFlags()[1] ? this.tumourLocalId : (java.lang.String) defaultValue(fields()[1]);
record.tumourType = fieldSetFlags()[2] ? this.tumourType : (org.gel.models.participant.avro.TumourType) defaultValue(fields()[2]);
record.tumourParentId = fieldSetFlags()[3] ? this.tumourParentId : (java.lang.String) defaultValue(fields()[3]);
record.tumourDiagnosisDate = fieldSetFlags()[4] ? this.tumourDiagnosisDate : (org.gel.models.participant.avro.Date) defaultValue(fields()[4]);
record.tumourDescription = fieldSetFlags()[5] ? this.tumourDescription : (java.lang.String) defaultValue(fields()[5]);
record.tumourMorphologies = fieldSetFlags()[6] ? this.tumourMorphologies : (java.util.List) defaultValue(fields()[6]);
record.tumourTopographies = fieldSetFlags()[7] ? this.tumourTopographies : (java.util.List) defaultValue(fields()[7]);
record.tumourPrimaryTopographies = fieldSetFlags()[8] ? this.tumourPrimaryTopographies : (java.util.List) defaultValue(fields()[8]);
record.tumourGrade = fieldSetFlags()[9] ? this.tumourGrade : (java.lang.String) defaultValue(fields()[9]);
record.tumourStage = fieldSetFlags()[10] ? this.tumourStage : (java.lang.String) defaultValue(fields()[10]);
record.tumourPrognosticScore = fieldSetFlags()[11] ? this.tumourPrognosticScore : (java.lang.String) defaultValue(fields()[11]);
record.tumourPresentation = fieldSetFlags()[12] ? this.tumourPresentation : (org.gel.models.participant.avro.TumourPresentation) defaultValue(fields()[12]);
record.primaryOrMetastatic = fieldSetFlags()[13] ? this.primaryOrMetastatic : (org.gel.models.participant.avro.PrimaryOrMetastatic) defaultValue(fields()[13]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy