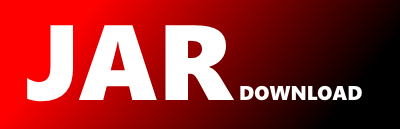
org.gel.models.report.avro.File Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.report.avro;
@SuppressWarnings("all")
/** This defines a file
This record is uniquely defined by the sample identfier and an URI
Currently sample identifier can be a single string or a list of strings if multiple samples are associated with the same file
* */
@org.apache.avro.specific.AvroGenerated
public class File extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"File\",\"namespace\":\"org.gel.models.report.avro\",\"doc\":\"This defines a file\\n This record is uniquely defined by the sample identfier and an URI\\n Currently sample identifier can be a single string or a list of strings if multiple samples are associated with the same file\\n *\",\"fields\":[{\"name\":\"sampleId\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"string\",\"avro.java.string\":\"String\"}}],\"doc\":\"Unique identifier(s) of the sample. For example in a multisample vcf this would have an array of all the sample identifiers\"},{\"name\":\"uriFile\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"URI path of the file\"},{\"name\":\"fileType\",\"type\":{\"type\":\"enum\",\"name\":\"FileType\",\"symbols\":[\"BAM\",\"gVCF\",\"VCF_small\",\"VCF_somatic_small\",\"VCF_CNV\",\"VCF_somatic_CNV\",\"VCF_SV\",\"VCF_somatic_SV\",\"VCF_SV_CNV\",\"SVG\",\"ANN\",\"BigWig\",\"MD5Sum\",\"ROH\",\"OTHER\",\"PARTITION\",\"VARIANT_FREQUENCIES\",\"COVERAGE\"]},\"doc\":\"The type of the file\"},{\"name\":\"md5Sum\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The MD5 checksum\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** Unique identifier(s) of the sample. For example in a multisample vcf this would have an array of all the sample identifiers */
private java.util.List sampleId;
/** URI path of the file */
private java.lang.String uriFile;
/** The type of the file */
private org.gel.models.report.avro.FileType fileType;
/** The MD5 checksum */
private java.lang.String md5Sum;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public File() {}
/**
* All-args constructor.
*/
public File(java.util.List sampleId, java.lang.String uriFile, org.gel.models.report.avro.FileType fileType, java.lang.String md5Sum) {
this.sampleId = sampleId;
this.uriFile = uriFile;
this.fileType = fileType;
this.md5Sum = md5Sum;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return sampleId;
case 1: return uriFile;
case 2: return fileType;
case 3: return md5Sum;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: sampleId = (java.util.List)value$; break;
case 1: uriFile = (java.lang.String)value$; break;
case 2: fileType = (org.gel.models.report.avro.FileType)value$; break;
case 3: md5Sum = (java.lang.String)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'sampleId' field.
* Unique identifier(s) of the sample. For example in a multisample vcf this would have an array of all the sample identifiers */
public java.util.List getSampleId() {
return sampleId;
}
/**
* Sets the value of the 'sampleId' field.
* Unique identifier(s) of the sample. For example in a multisample vcf this would have an array of all the sample identifiers * @param value the value to set.
*/
public void setSampleId(java.util.List value) {
this.sampleId = value;
}
/**
* Gets the value of the 'uriFile' field.
* URI path of the file */
public java.lang.String getUriFile() {
return uriFile;
}
/**
* Sets the value of the 'uriFile' field.
* URI path of the file * @param value the value to set.
*/
public void setUriFile(java.lang.String value) {
this.uriFile = value;
}
/**
* Gets the value of the 'fileType' field.
* The type of the file */
public org.gel.models.report.avro.FileType getFileType() {
return fileType;
}
/**
* Sets the value of the 'fileType' field.
* The type of the file * @param value the value to set.
*/
public void setFileType(org.gel.models.report.avro.FileType value) {
this.fileType = value;
}
/**
* Gets the value of the 'md5Sum' field.
* The MD5 checksum */
public java.lang.String getMd5Sum() {
return md5Sum;
}
/**
* Sets the value of the 'md5Sum' field.
* The MD5 checksum * @param value the value to set.
*/
public void setMd5Sum(java.lang.String value) {
this.md5Sum = value;
}
/** Creates a new File RecordBuilder */
public static org.gel.models.report.avro.File.Builder newBuilder() {
return new org.gel.models.report.avro.File.Builder();
}
/** Creates a new File RecordBuilder by copying an existing Builder */
public static org.gel.models.report.avro.File.Builder newBuilder(org.gel.models.report.avro.File.Builder other) {
return new org.gel.models.report.avro.File.Builder(other);
}
/** Creates a new File RecordBuilder by copying an existing File instance */
public static org.gel.models.report.avro.File.Builder newBuilder(org.gel.models.report.avro.File other) {
return new org.gel.models.report.avro.File.Builder(other);
}
/**
* RecordBuilder for File instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.util.List sampleId;
private java.lang.String uriFile;
private org.gel.models.report.avro.FileType fileType;
private java.lang.String md5Sum;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.report.avro.File.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.report.avro.File.Builder other) {
super(other);
if (isValidValue(fields()[0], other.sampleId)) {
this.sampleId = data().deepCopy(fields()[0].schema(), other.sampleId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.uriFile)) {
this.uriFile = data().deepCopy(fields()[1].schema(), other.uriFile);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.fileType)) {
this.fileType = data().deepCopy(fields()[2].schema(), other.fileType);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.md5Sum)) {
this.md5Sum = data().deepCopy(fields()[3].schema(), other.md5Sum);
fieldSetFlags()[3] = true;
}
}
/** Creates a Builder by copying an existing File instance */
private Builder(org.gel.models.report.avro.File other) {
super(org.gel.models.report.avro.File.SCHEMA$);
if (isValidValue(fields()[0], other.sampleId)) {
this.sampleId = data().deepCopy(fields()[0].schema(), other.sampleId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.uriFile)) {
this.uriFile = data().deepCopy(fields()[1].schema(), other.uriFile);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.fileType)) {
this.fileType = data().deepCopy(fields()[2].schema(), other.fileType);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.md5Sum)) {
this.md5Sum = data().deepCopy(fields()[3].schema(), other.md5Sum);
fieldSetFlags()[3] = true;
}
}
/** Gets the value of the 'sampleId' field */
public java.util.List getSampleId() {
return sampleId;
}
/** Sets the value of the 'sampleId' field */
public org.gel.models.report.avro.File.Builder setSampleId(java.util.List value) {
validate(fields()[0], value);
this.sampleId = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'sampleId' field has been set */
public boolean hasSampleId() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'sampleId' field */
public org.gel.models.report.avro.File.Builder clearSampleId() {
sampleId = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'uriFile' field */
public java.lang.String getUriFile() {
return uriFile;
}
/** Sets the value of the 'uriFile' field */
public org.gel.models.report.avro.File.Builder setUriFile(java.lang.String value) {
validate(fields()[1], value);
this.uriFile = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'uriFile' field has been set */
public boolean hasUriFile() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'uriFile' field */
public org.gel.models.report.avro.File.Builder clearUriFile() {
uriFile = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'fileType' field */
public org.gel.models.report.avro.FileType getFileType() {
return fileType;
}
/** Sets the value of the 'fileType' field */
public org.gel.models.report.avro.File.Builder setFileType(org.gel.models.report.avro.FileType value) {
validate(fields()[2], value);
this.fileType = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'fileType' field has been set */
public boolean hasFileType() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'fileType' field */
public org.gel.models.report.avro.File.Builder clearFileType() {
fileType = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'md5Sum' field */
public java.lang.String getMd5Sum() {
return md5Sum;
}
/** Sets the value of the 'md5Sum' field */
public org.gel.models.report.avro.File.Builder setMd5Sum(java.lang.String value) {
validate(fields()[3], value);
this.md5Sum = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'md5Sum' field has been set */
public boolean hasMd5Sum() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'md5Sum' field */
public org.gel.models.report.avro.File.Builder clearMd5Sum() {
md5Sum = null;
fieldSetFlags()[3] = false;
return this;
}
@Override
public File build() {
try {
File record = new File();
record.sampleId = fieldSetFlags()[0] ? this.sampleId : (java.util.List) defaultValue(fields()[0]);
record.uriFile = fieldSetFlags()[1] ? this.uriFile : (java.lang.String) defaultValue(fields()[1]);
record.fileType = fieldSetFlags()[2] ? this.fileType : (org.gel.models.report.avro.FileType) defaultValue(fields()[2]);
record.md5Sum = fieldSetFlags()[3] ? this.md5Sum : (java.lang.String) defaultValue(fields()[3]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy