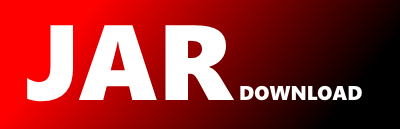
org.gel.models.report.avro.Therapy Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.report.avro;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class Therapy extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"Therapy\",\"namespace\":\"org.gel.models.report.avro\",\"fields\":[{\"name\":\"referenceUrl\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"URL where reference information for this therapy association can be found\"},{\"name\":\"source\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Source\"},{\"name\":\"references\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"string\",\"avro.java.string\":\"String\"}}],\"doc\":\"References\"},{\"name\":\"conditions\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"string\",\"avro.java.string\":\"String\"}}],\"doc\":\"Conditions\"},{\"name\":\"drugResponse\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"DrugResponse\",\"fields\":[{\"name\":\"TreatmentAgent\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Treatment agent\"},{\"name\":\"drugResponseClassification\",\"type\":{\"type\":\"enum\",\"name\":\"DrugResponseClassification\",\"symbols\":[\"altered_sensitivity\",\"reduced_sensitivity\",\"increased_sensitivity\",\"altered_resistance\",\"increased_resistance\",\"reduced_resistance\",\"increased_risk_of_toxicity\",\"reduced_risk_of_toxicity\",\"altered_toxicity\",\"adverse_drug_reaction\",\"indication\",\"contraindication\",\"dosing_alteration\",\"increased_dose\",\"reduced_dose\",\"increased_monitoring\",\"increased_efficacy\",\"reduced_efficacy\",\"altered_efficacy\"]},\"doc\":\"associated effect of the drug\"}]}}],\"doc\":\"Drug responses\"},{\"name\":\"otherInterventions\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"Intervention\",\"doc\":\"A process or action that is the focus of a clinical study.\\n Ref. https://prsinfo.clinicaltrials.gov/definitions.html\",\"fields\":[{\"name\":\"interventionType\",\"type\":{\"type\":\"enum\",\"name\":\"InterventionType\",\"doc\":\"For each intervention studied in the clinical study, the general type of intervention\\n\\n* `drug`: Including placebo\\n* `device`: Including sham\\n* `biological`: Vaccine\\n* `procedure`: Surgery\\n* `radiation`\\n* `behavioral`: For example, psychotherapy, lifestyle counselling\\n* `genetic`: Including gene transfer, stem cell and recombinant DNA\\n* `dietary_supplement`: For example, vitamins, minerals\\n* `combination_product`: Combining a drug and device, a biological product and device; a drug and biological product; or a drug, biological product, and device\\n* `diagnostic_test`: For example, imaging, in-vitro\\n* `other`\\n\\n Ref. https://prsinfo.clinicaltrials.gov/definitions.htm\",\"symbols\":[\"drug\",\"device\",\"procedure\",\"biological\",\"radiation\",\"behavioral\",\"genetic\",\"dietary_supplement\",\"combination_product\",\"diagnostic_test\",\"other\"]},\"doc\":\"Intervention type, i.e drug\"},{\"name\":\"interventionName\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Intervention name: Placebo\"}]}}],\"doc\":\"Any other clinical intervention\"},{\"name\":\"variantActionable\",\"type\":\"boolean\",\"doc\":\"If true, the association was made at the variant level, if not the association was made at Genomic Entity level\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** URL where reference information for this therapy association can be found */
private java.lang.String referenceUrl;
/** Source */
private java.lang.String source;
/** References */
private java.util.List references;
/** Conditions */
private java.util.List conditions;
/** Drug responses */
private java.util.List drugResponse;
/** Any other clinical intervention */
private java.util.List otherInterventions;
/** If true, the association was made at the variant level, if not the association was made at Genomic Entity level */
private boolean variantActionable;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public Therapy() {}
/**
* All-args constructor.
*/
public Therapy(java.lang.String referenceUrl, java.lang.String source, java.util.List references, java.util.List conditions, java.util.List drugResponse, java.util.List otherInterventions, java.lang.Boolean variantActionable) {
this.referenceUrl = referenceUrl;
this.source = source;
this.references = references;
this.conditions = conditions;
this.drugResponse = drugResponse;
this.otherInterventions = otherInterventions;
this.variantActionable = variantActionable;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return referenceUrl;
case 1: return source;
case 2: return references;
case 3: return conditions;
case 4: return drugResponse;
case 5: return otherInterventions;
case 6: return variantActionable;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: referenceUrl = (java.lang.String)value$; break;
case 1: source = (java.lang.String)value$; break;
case 2: references = (java.util.List)value$; break;
case 3: conditions = (java.util.List)value$; break;
case 4: drugResponse = (java.util.List)value$; break;
case 5: otherInterventions = (java.util.List)value$; break;
case 6: variantActionable = (java.lang.Boolean)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'referenceUrl' field.
* URL where reference information for this therapy association can be found */
public java.lang.String getReferenceUrl() {
return referenceUrl;
}
/**
* Sets the value of the 'referenceUrl' field.
* URL where reference information for this therapy association can be found * @param value the value to set.
*/
public void setReferenceUrl(java.lang.String value) {
this.referenceUrl = value;
}
/**
* Gets the value of the 'source' field.
* Source */
public java.lang.String getSource() {
return source;
}
/**
* Sets the value of the 'source' field.
* Source * @param value the value to set.
*/
public void setSource(java.lang.String value) {
this.source = value;
}
/**
* Gets the value of the 'references' field.
* References */
public java.util.List getReferences() {
return references;
}
/**
* Sets the value of the 'references' field.
* References * @param value the value to set.
*/
public void setReferences(java.util.List value) {
this.references = value;
}
/**
* Gets the value of the 'conditions' field.
* Conditions */
public java.util.List getConditions() {
return conditions;
}
/**
* Sets the value of the 'conditions' field.
* Conditions * @param value the value to set.
*/
public void setConditions(java.util.List value) {
this.conditions = value;
}
/**
* Gets the value of the 'drugResponse' field.
* Drug responses */
public java.util.List getDrugResponse() {
return drugResponse;
}
/**
* Sets the value of the 'drugResponse' field.
* Drug responses * @param value the value to set.
*/
public void setDrugResponse(java.util.List value) {
this.drugResponse = value;
}
/**
* Gets the value of the 'otherInterventions' field.
* Any other clinical intervention */
public java.util.List getOtherInterventions() {
return otherInterventions;
}
/**
* Sets the value of the 'otherInterventions' field.
* Any other clinical intervention * @param value the value to set.
*/
public void setOtherInterventions(java.util.List value) {
this.otherInterventions = value;
}
/**
* Gets the value of the 'variantActionable' field.
* If true, the association was made at the variant level, if not the association was made at Genomic Entity level */
public java.lang.Boolean getVariantActionable() {
return variantActionable;
}
/**
* Sets the value of the 'variantActionable' field.
* If true, the association was made at the variant level, if not the association was made at Genomic Entity level * @param value the value to set.
*/
public void setVariantActionable(java.lang.Boolean value) {
this.variantActionable = value;
}
/** Creates a new Therapy RecordBuilder */
public static org.gel.models.report.avro.Therapy.Builder newBuilder() {
return new org.gel.models.report.avro.Therapy.Builder();
}
/** Creates a new Therapy RecordBuilder by copying an existing Builder */
public static org.gel.models.report.avro.Therapy.Builder newBuilder(org.gel.models.report.avro.Therapy.Builder other) {
return new org.gel.models.report.avro.Therapy.Builder(other);
}
/** Creates a new Therapy RecordBuilder by copying an existing Therapy instance */
public static org.gel.models.report.avro.Therapy.Builder newBuilder(org.gel.models.report.avro.Therapy other) {
return new org.gel.models.report.avro.Therapy.Builder(other);
}
/**
* RecordBuilder for Therapy instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String referenceUrl;
private java.lang.String source;
private java.util.List references;
private java.util.List conditions;
private java.util.List drugResponse;
private java.util.List otherInterventions;
private boolean variantActionable;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.report.avro.Therapy.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.report.avro.Therapy.Builder other) {
super(other);
if (isValidValue(fields()[0], other.referenceUrl)) {
this.referenceUrl = data().deepCopy(fields()[0].schema(), other.referenceUrl);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.source)) {
this.source = data().deepCopy(fields()[1].schema(), other.source);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.references)) {
this.references = data().deepCopy(fields()[2].schema(), other.references);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.conditions)) {
this.conditions = data().deepCopy(fields()[3].schema(), other.conditions);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.drugResponse)) {
this.drugResponse = data().deepCopy(fields()[4].schema(), other.drugResponse);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.otherInterventions)) {
this.otherInterventions = data().deepCopy(fields()[5].schema(), other.otherInterventions);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.variantActionable)) {
this.variantActionable = data().deepCopy(fields()[6].schema(), other.variantActionable);
fieldSetFlags()[6] = true;
}
}
/** Creates a Builder by copying an existing Therapy instance */
private Builder(org.gel.models.report.avro.Therapy other) {
super(org.gel.models.report.avro.Therapy.SCHEMA$);
if (isValidValue(fields()[0], other.referenceUrl)) {
this.referenceUrl = data().deepCopy(fields()[0].schema(), other.referenceUrl);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.source)) {
this.source = data().deepCopy(fields()[1].schema(), other.source);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.references)) {
this.references = data().deepCopy(fields()[2].schema(), other.references);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.conditions)) {
this.conditions = data().deepCopy(fields()[3].schema(), other.conditions);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.drugResponse)) {
this.drugResponse = data().deepCopy(fields()[4].schema(), other.drugResponse);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.otherInterventions)) {
this.otherInterventions = data().deepCopy(fields()[5].schema(), other.otherInterventions);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.variantActionable)) {
this.variantActionable = data().deepCopy(fields()[6].schema(), other.variantActionable);
fieldSetFlags()[6] = true;
}
}
/** Gets the value of the 'referenceUrl' field */
public java.lang.String getReferenceUrl() {
return referenceUrl;
}
/** Sets the value of the 'referenceUrl' field */
public org.gel.models.report.avro.Therapy.Builder setReferenceUrl(java.lang.String value) {
validate(fields()[0], value);
this.referenceUrl = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'referenceUrl' field has been set */
public boolean hasReferenceUrl() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'referenceUrl' field */
public org.gel.models.report.avro.Therapy.Builder clearReferenceUrl() {
referenceUrl = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'source' field */
public java.lang.String getSource() {
return source;
}
/** Sets the value of the 'source' field */
public org.gel.models.report.avro.Therapy.Builder setSource(java.lang.String value) {
validate(fields()[1], value);
this.source = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'source' field has been set */
public boolean hasSource() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'source' field */
public org.gel.models.report.avro.Therapy.Builder clearSource() {
source = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'references' field */
public java.util.List getReferences() {
return references;
}
/** Sets the value of the 'references' field */
public org.gel.models.report.avro.Therapy.Builder setReferences(java.util.List value) {
validate(fields()[2], value);
this.references = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'references' field has been set */
public boolean hasReferences() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'references' field */
public org.gel.models.report.avro.Therapy.Builder clearReferences() {
references = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'conditions' field */
public java.util.List getConditions() {
return conditions;
}
/** Sets the value of the 'conditions' field */
public org.gel.models.report.avro.Therapy.Builder setConditions(java.util.List value) {
validate(fields()[3], value);
this.conditions = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'conditions' field has been set */
public boolean hasConditions() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'conditions' field */
public org.gel.models.report.avro.Therapy.Builder clearConditions() {
conditions = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'drugResponse' field */
public java.util.List getDrugResponse() {
return drugResponse;
}
/** Sets the value of the 'drugResponse' field */
public org.gel.models.report.avro.Therapy.Builder setDrugResponse(java.util.List value) {
validate(fields()[4], value);
this.drugResponse = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'drugResponse' field has been set */
public boolean hasDrugResponse() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'drugResponse' field */
public org.gel.models.report.avro.Therapy.Builder clearDrugResponse() {
drugResponse = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'otherInterventions' field */
public java.util.List getOtherInterventions() {
return otherInterventions;
}
/** Sets the value of the 'otherInterventions' field */
public org.gel.models.report.avro.Therapy.Builder setOtherInterventions(java.util.List value) {
validate(fields()[5], value);
this.otherInterventions = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'otherInterventions' field has been set */
public boolean hasOtherInterventions() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'otherInterventions' field */
public org.gel.models.report.avro.Therapy.Builder clearOtherInterventions() {
otherInterventions = null;
fieldSetFlags()[5] = false;
return this;
}
/** Gets the value of the 'variantActionable' field */
public java.lang.Boolean getVariantActionable() {
return variantActionable;
}
/** Sets the value of the 'variantActionable' field */
public org.gel.models.report.avro.Therapy.Builder setVariantActionable(boolean value) {
validate(fields()[6], value);
this.variantActionable = value;
fieldSetFlags()[6] = true;
return this;
}
/** Checks whether the 'variantActionable' field has been set */
public boolean hasVariantActionable() {
return fieldSetFlags()[6];
}
/** Clears the value of the 'variantActionable' field */
public org.gel.models.report.avro.Therapy.Builder clearVariantActionable() {
fieldSetFlags()[6] = false;
return this;
}
@Override
public Therapy build() {
try {
Therapy record = new Therapy();
record.referenceUrl = fieldSetFlags()[0] ? this.referenceUrl : (java.lang.String) defaultValue(fields()[0]);
record.source = fieldSetFlags()[1] ? this.source : (java.lang.String) defaultValue(fields()[1]);
record.references = fieldSetFlags()[2] ? this.references : (java.util.List) defaultValue(fields()[2]);
record.conditions = fieldSetFlags()[3] ? this.conditions : (java.util.List) defaultValue(fields()[3]);
record.drugResponse = fieldSetFlags()[4] ? this.drugResponse : (java.util.List) defaultValue(fields()[4]);
record.otherInterventions = fieldSetFlags()[5] ? this.otherInterventions : (java.util.List) defaultValue(fields()[5]);
record.variantActionable = fieldSetFlags()[6] ? this.variantActionable : (java.lang.Boolean) defaultValue(fields()[6]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy