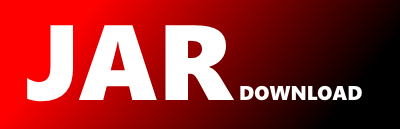
org.gel.models.report.avro.VariantCall Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.gel.models.report.avro;
@SuppressWarnings("all")
/** This is intended to hold the genotypes for the family members. This assumes that varinats have been split before.
In principle it is a phased zygosity as in VCF spec and called by the analysis provider if further phasing is conducted */
@org.apache.avro.specific.AvroGenerated
public class VariantCall extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"VariantCall\",\"namespace\":\"org.gel.models.report.avro\",\"doc\":\"This is intended to hold the genotypes for the family members. This assumes that varinats have been split before.\\n In principle it is a phased zygosity as in VCF spec and called by the analysis provider if further phasing is conducted\",\"fields\":[{\"name\":\"participantId\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Participant id\"},{\"name\":\"sampleId\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Sample Id\"},{\"name\":\"zygosity\",\"type\":{\"type\":\"enum\",\"name\":\"Zygosity\",\"doc\":\"It is a representation of the zygosity\\n\\n* `reference_homozygous`: 0/0, 0|0\\n* `heterozygous`: 0/1, 1/0, 1|0, 0|1\\n* `alternate_homozygous`: 1/1, 1|1\\n* `missing`: ./., .|.\\n* `half_missing_reference`: ./0, 0/., 0|., .|0\\n* `half_missing_alternate`: ./1, 1/., 1|., .|1\\n* `alternate_hemizigous`: 1\\n* `reference_hemizigous`: 0\\n* `unk`: Anything unexpected\",\"symbols\":[\"reference_homozygous\",\"heterozygous\",\"alternate_homozygous\",\"missing\",\"half_missing_reference\",\"half_missing_alternate\",\"alternate_hemizigous\",\"reference_hemizigous\",\"unk\",\"na\"]},\"doc\":\"Zygosity. For somatic variants, or variants without zygosity use `na`\"},{\"name\":\"phaseGenotype\",\"type\":[\"null\",{\"type\":\"record\",\"name\":\"PhaseGenotype\",\"fields\":[{\"name\":\"sortedAlleles\",\"type\":{\"type\":\"array\",\"items\":{\"type\":\"string\",\"avro.java.string\":\"String\"}}},{\"name\":\"phaseSet\",\"type\":\"int\"}]}],\"doc\":\"phase alleles for those in phase\"},{\"name\":\"sampleVariantAlleleFrequency\",\"type\":[\"null\",\"double\"],\"doc\":\"Sample Variant Allele Frequency\"},{\"name\":\"depthReference\",\"type\":[\"null\",\"int\"],\"doc\":\"Depth for Reference Allele\"},{\"name\":\"depthAlternate\",\"type\":[\"null\",\"int\"],\"doc\":\"Depth for Alternate Allele\"},{\"name\":\"numberOfCopies\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"NumberOfCopies\",\"fields\":[{\"name\":\"numberOfCopies\",\"type\":\"int\",\"doc\":\"Number of copies given by the caller in one of the allele\"},{\"name\":\"confidenceIntervalMaximum\",\"type\":[\"null\",\"int\"]},{\"name\":\"confidenceIntervalMinimum\",\"type\":[\"null\",\"int\"]}]}}],\"doc\":\"Alleles for copy number variation - add doc\"},{\"name\":\"alleleOrigins\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"enum\",\"name\":\"AlleleOrigin\",\"doc\":\"Allele origin.\\n\\n* `SO_0001781`: de novo variant. http://purl.obolibrary.org/obo/SO_0001781\\n* `SO_0001778`: germline variant. http://purl.obolibrary.org/obo/SO_0001778\\n* `SO_0001775`: maternal variant. http://purl.obolibrary.org/obo/SO_0001775\\n* `SO_0001776`: paternal variant. http://purl.obolibrary.org/obo/SO_0001776\\n* `SO_0001779`: pedigree specific variant. http://purl.obolibrary.org/obo/SO_0001779\\n* `SO_0001780`: population specific variant. http://purl.obolibrary.org/obo/SO_0001780\\n* `SO_0001777`: somatic variant. http://purl.obolibrary.org/obo/SO_0001777\",\"symbols\":[\"de_novo_variant\",\"germline_variant\",\"maternal_variant\",\"paternal_variant\",\"pedigree_specific_variant\",\"population_specific_variant\",\"somatic_variant\"]}}],\"doc\":\"Describe whether this is a somatic or Germline variant\"},{\"name\":\"supportingReadTypes\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"enum\",\"name\":\"SupportingReadType\",\"symbols\":[\"spanning\",\"flanking\",\"inrepeat\"]}}]}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
/** Participant id */
private java.lang.String participantId;
/** Sample Id */
private java.lang.String sampleId;
/** Zygosity. For somatic variants, or variants without zygosity use `na` */
private org.gel.models.report.avro.Zygosity zygosity;
/** phase alleles for those in phase */
private org.gel.models.report.avro.PhaseGenotype phaseGenotype;
/** Sample Variant Allele Frequency */
private java.lang.Double sampleVariantAlleleFrequency;
/** Depth for Reference Allele */
private java.lang.Integer depthReference;
/** Depth for Alternate Allele */
private java.lang.Integer depthAlternate;
/** Alleles for copy number variation - add doc */
private java.util.List numberOfCopies;
/** Describe whether this is a somatic or Germline variant */
private java.util.List alleleOrigins;
private java.util.List supportingReadTypes;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public VariantCall() {}
/**
* All-args constructor.
*/
public VariantCall(java.lang.String participantId, java.lang.String sampleId, org.gel.models.report.avro.Zygosity zygosity, org.gel.models.report.avro.PhaseGenotype phaseGenotype, java.lang.Double sampleVariantAlleleFrequency, java.lang.Integer depthReference, java.lang.Integer depthAlternate, java.util.List numberOfCopies, java.util.List alleleOrigins, java.util.List supportingReadTypes) {
this.participantId = participantId;
this.sampleId = sampleId;
this.zygosity = zygosity;
this.phaseGenotype = phaseGenotype;
this.sampleVariantAlleleFrequency = sampleVariantAlleleFrequency;
this.depthReference = depthReference;
this.depthAlternate = depthAlternate;
this.numberOfCopies = numberOfCopies;
this.alleleOrigins = alleleOrigins;
this.supportingReadTypes = supportingReadTypes;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return participantId;
case 1: return sampleId;
case 2: return zygosity;
case 3: return phaseGenotype;
case 4: return sampleVariantAlleleFrequency;
case 5: return depthReference;
case 6: return depthAlternate;
case 7: return numberOfCopies;
case 8: return alleleOrigins;
case 9: return supportingReadTypes;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: participantId = (java.lang.String)value$; break;
case 1: sampleId = (java.lang.String)value$; break;
case 2: zygosity = (org.gel.models.report.avro.Zygosity)value$; break;
case 3: phaseGenotype = (org.gel.models.report.avro.PhaseGenotype)value$; break;
case 4: sampleVariantAlleleFrequency = (java.lang.Double)value$; break;
case 5: depthReference = (java.lang.Integer)value$; break;
case 6: depthAlternate = (java.lang.Integer)value$; break;
case 7: numberOfCopies = (java.util.List)value$; break;
case 8: alleleOrigins = (java.util.List)value$; break;
case 9: supportingReadTypes = (java.util.List)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'participantId' field.
* Participant id */
public java.lang.String getParticipantId() {
return participantId;
}
/**
* Sets the value of the 'participantId' field.
* Participant id * @param value the value to set.
*/
public void setParticipantId(java.lang.String value) {
this.participantId = value;
}
/**
* Gets the value of the 'sampleId' field.
* Sample Id */
public java.lang.String getSampleId() {
return sampleId;
}
/**
* Sets the value of the 'sampleId' field.
* Sample Id * @param value the value to set.
*/
public void setSampleId(java.lang.String value) {
this.sampleId = value;
}
/**
* Gets the value of the 'zygosity' field.
* Zygosity. For somatic variants, or variants without zygosity use `na` */
public org.gel.models.report.avro.Zygosity getZygosity() {
return zygosity;
}
/**
* Sets the value of the 'zygosity' field.
* Zygosity. For somatic variants, or variants without zygosity use `na` * @param value the value to set.
*/
public void setZygosity(org.gel.models.report.avro.Zygosity value) {
this.zygosity = value;
}
/**
* Gets the value of the 'phaseGenotype' field.
* phase alleles for those in phase */
public org.gel.models.report.avro.PhaseGenotype getPhaseGenotype() {
return phaseGenotype;
}
/**
* Sets the value of the 'phaseGenotype' field.
* phase alleles for those in phase * @param value the value to set.
*/
public void setPhaseGenotype(org.gel.models.report.avro.PhaseGenotype value) {
this.phaseGenotype = value;
}
/**
* Gets the value of the 'sampleVariantAlleleFrequency' field.
* Sample Variant Allele Frequency */
public java.lang.Double getSampleVariantAlleleFrequency() {
return sampleVariantAlleleFrequency;
}
/**
* Sets the value of the 'sampleVariantAlleleFrequency' field.
* Sample Variant Allele Frequency * @param value the value to set.
*/
public void setSampleVariantAlleleFrequency(java.lang.Double value) {
this.sampleVariantAlleleFrequency = value;
}
/**
* Gets the value of the 'depthReference' field.
* Depth for Reference Allele */
public java.lang.Integer getDepthReference() {
return depthReference;
}
/**
* Sets the value of the 'depthReference' field.
* Depth for Reference Allele * @param value the value to set.
*/
public void setDepthReference(java.lang.Integer value) {
this.depthReference = value;
}
/**
* Gets the value of the 'depthAlternate' field.
* Depth for Alternate Allele */
public java.lang.Integer getDepthAlternate() {
return depthAlternate;
}
/**
* Sets the value of the 'depthAlternate' field.
* Depth for Alternate Allele * @param value the value to set.
*/
public void setDepthAlternate(java.lang.Integer value) {
this.depthAlternate = value;
}
/**
* Gets the value of the 'numberOfCopies' field.
* Alleles for copy number variation - add doc */
public java.util.List getNumberOfCopies() {
return numberOfCopies;
}
/**
* Sets the value of the 'numberOfCopies' field.
* Alleles for copy number variation - add doc * @param value the value to set.
*/
public void setNumberOfCopies(java.util.List value) {
this.numberOfCopies = value;
}
/**
* Gets the value of the 'alleleOrigins' field.
* Describe whether this is a somatic or Germline variant */
public java.util.List getAlleleOrigins() {
return alleleOrigins;
}
/**
* Sets the value of the 'alleleOrigins' field.
* Describe whether this is a somatic or Germline variant * @param value the value to set.
*/
public void setAlleleOrigins(java.util.List value) {
this.alleleOrigins = value;
}
/**
* Gets the value of the 'supportingReadTypes' field.
*/
public java.util.List getSupportingReadTypes() {
return supportingReadTypes;
}
/**
* Sets the value of the 'supportingReadTypes' field.
* @param value the value to set.
*/
public void setSupportingReadTypes(java.util.List value) {
this.supportingReadTypes = value;
}
/** Creates a new VariantCall RecordBuilder */
public static org.gel.models.report.avro.VariantCall.Builder newBuilder() {
return new org.gel.models.report.avro.VariantCall.Builder();
}
/** Creates a new VariantCall RecordBuilder by copying an existing Builder */
public static org.gel.models.report.avro.VariantCall.Builder newBuilder(org.gel.models.report.avro.VariantCall.Builder other) {
return new org.gel.models.report.avro.VariantCall.Builder(other);
}
/** Creates a new VariantCall RecordBuilder by copying an existing VariantCall instance */
public static org.gel.models.report.avro.VariantCall.Builder newBuilder(org.gel.models.report.avro.VariantCall other) {
return new org.gel.models.report.avro.VariantCall.Builder(other);
}
/**
* RecordBuilder for VariantCall instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String participantId;
private java.lang.String sampleId;
private org.gel.models.report.avro.Zygosity zygosity;
private org.gel.models.report.avro.PhaseGenotype phaseGenotype;
private java.lang.Double sampleVariantAlleleFrequency;
private java.lang.Integer depthReference;
private java.lang.Integer depthAlternate;
private java.util.List numberOfCopies;
private java.util.List alleleOrigins;
private java.util.List supportingReadTypes;
/** Creates a new Builder */
private Builder() {
super(org.gel.models.report.avro.VariantCall.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.gel.models.report.avro.VariantCall.Builder other) {
super(other);
if (isValidValue(fields()[0], other.participantId)) {
this.participantId = data().deepCopy(fields()[0].schema(), other.participantId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.sampleId)) {
this.sampleId = data().deepCopy(fields()[1].schema(), other.sampleId);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.zygosity)) {
this.zygosity = data().deepCopy(fields()[2].schema(), other.zygosity);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.phaseGenotype)) {
this.phaseGenotype = data().deepCopy(fields()[3].schema(), other.phaseGenotype);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.sampleVariantAlleleFrequency)) {
this.sampleVariantAlleleFrequency = data().deepCopy(fields()[4].schema(), other.sampleVariantAlleleFrequency);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.depthReference)) {
this.depthReference = data().deepCopy(fields()[5].schema(), other.depthReference);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.depthAlternate)) {
this.depthAlternate = data().deepCopy(fields()[6].schema(), other.depthAlternate);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.numberOfCopies)) {
this.numberOfCopies = data().deepCopy(fields()[7].schema(), other.numberOfCopies);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.alleleOrigins)) {
this.alleleOrigins = data().deepCopy(fields()[8].schema(), other.alleleOrigins);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.supportingReadTypes)) {
this.supportingReadTypes = data().deepCopy(fields()[9].schema(), other.supportingReadTypes);
fieldSetFlags()[9] = true;
}
}
/** Creates a Builder by copying an existing VariantCall instance */
private Builder(org.gel.models.report.avro.VariantCall other) {
super(org.gel.models.report.avro.VariantCall.SCHEMA$);
if (isValidValue(fields()[0], other.participantId)) {
this.participantId = data().deepCopy(fields()[0].schema(), other.participantId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.sampleId)) {
this.sampleId = data().deepCopy(fields()[1].schema(), other.sampleId);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.zygosity)) {
this.zygosity = data().deepCopy(fields()[2].schema(), other.zygosity);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.phaseGenotype)) {
this.phaseGenotype = data().deepCopy(fields()[3].schema(), other.phaseGenotype);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.sampleVariantAlleleFrequency)) {
this.sampleVariantAlleleFrequency = data().deepCopy(fields()[4].schema(), other.sampleVariantAlleleFrequency);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.depthReference)) {
this.depthReference = data().deepCopy(fields()[5].schema(), other.depthReference);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.depthAlternate)) {
this.depthAlternate = data().deepCopy(fields()[6].schema(), other.depthAlternate);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.numberOfCopies)) {
this.numberOfCopies = data().deepCopy(fields()[7].schema(), other.numberOfCopies);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.alleleOrigins)) {
this.alleleOrigins = data().deepCopy(fields()[8].schema(), other.alleleOrigins);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.supportingReadTypes)) {
this.supportingReadTypes = data().deepCopy(fields()[9].schema(), other.supportingReadTypes);
fieldSetFlags()[9] = true;
}
}
/** Gets the value of the 'participantId' field */
public java.lang.String getParticipantId() {
return participantId;
}
/** Sets the value of the 'participantId' field */
public org.gel.models.report.avro.VariantCall.Builder setParticipantId(java.lang.String value) {
validate(fields()[0], value);
this.participantId = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'participantId' field has been set */
public boolean hasParticipantId() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'participantId' field */
public org.gel.models.report.avro.VariantCall.Builder clearParticipantId() {
participantId = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'sampleId' field */
public java.lang.String getSampleId() {
return sampleId;
}
/** Sets the value of the 'sampleId' field */
public org.gel.models.report.avro.VariantCall.Builder setSampleId(java.lang.String value) {
validate(fields()[1], value);
this.sampleId = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'sampleId' field has been set */
public boolean hasSampleId() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'sampleId' field */
public org.gel.models.report.avro.VariantCall.Builder clearSampleId() {
sampleId = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'zygosity' field */
public org.gel.models.report.avro.Zygosity getZygosity() {
return zygosity;
}
/** Sets the value of the 'zygosity' field */
public org.gel.models.report.avro.VariantCall.Builder setZygosity(org.gel.models.report.avro.Zygosity value) {
validate(fields()[2], value);
this.zygosity = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'zygosity' field has been set */
public boolean hasZygosity() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'zygosity' field */
public org.gel.models.report.avro.VariantCall.Builder clearZygosity() {
zygosity = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'phaseGenotype' field */
public org.gel.models.report.avro.PhaseGenotype getPhaseGenotype() {
return phaseGenotype;
}
/** Sets the value of the 'phaseGenotype' field */
public org.gel.models.report.avro.VariantCall.Builder setPhaseGenotype(org.gel.models.report.avro.PhaseGenotype value) {
validate(fields()[3], value);
this.phaseGenotype = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'phaseGenotype' field has been set */
public boolean hasPhaseGenotype() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'phaseGenotype' field */
public org.gel.models.report.avro.VariantCall.Builder clearPhaseGenotype() {
phaseGenotype = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'sampleVariantAlleleFrequency' field */
public java.lang.Double getSampleVariantAlleleFrequency() {
return sampleVariantAlleleFrequency;
}
/** Sets the value of the 'sampleVariantAlleleFrequency' field */
public org.gel.models.report.avro.VariantCall.Builder setSampleVariantAlleleFrequency(java.lang.Double value) {
validate(fields()[4], value);
this.sampleVariantAlleleFrequency = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'sampleVariantAlleleFrequency' field has been set */
public boolean hasSampleVariantAlleleFrequency() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'sampleVariantAlleleFrequency' field */
public org.gel.models.report.avro.VariantCall.Builder clearSampleVariantAlleleFrequency() {
sampleVariantAlleleFrequency = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'depthReference' field */
public java.lang.Integer getDepthReference() {
return depthReference;
}
/** Sets the value of the 'depthReference' field */
public org.gel.models.report.avro.VariantCall.Builder setDepthReference(java.lang.Integer value) {
validate(fields()[5], value);
this.depthReference = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'depthReference' field has been set */
public boolean hasDepthReference() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'depthReference' field */
public org.gel.models.report.avro.VariantCall.Builder clearDepthReference() {
depthReference = null;
fieldSetFlags()[5] = false;
return this;
}
/** Gets the value of the 'depthAlternate' field */
public java.lang.Integer getDepthAlternate() {
return depthAlternate;
}
/** Sets the value of the 'depthAlternate' field */
public org.gel.models.report.avro.VariantCall.Builder setDepthAlternate(java.lang.Integer value) {
validate(fields()[6], value);
this.depthAlternate = value;
fieldSetFlags()[6] = true;
return this;
}
/** Checks whether the 'depthAlternate' field has been set */
public boolean hasDepthAlternate() {
return fieldSetFlags()[6];
}
/** Clears the value of the 'depthAlternate' field */
public org.gel.models.report.avro.VariantCall.Builder clearDepthAlternate() {
depthAlternate = null;
fieldSetFlags()[6] = false;
return this;
}
/** Gets the value of the 'numberOfCopies' field */
public java.util.List getNumberOfCopies() {
return numberOfCopies;
}
/** Sets the value of the 'numberOfCopies' field */
public org.gel.models.report.avro.VariantCall.Builder setNumberOfCopies(java.util.List value) {
validate(fields()[7], value);
this.numberOfCopies = value;
fieldSetFlags()[7] = true;
return this;
}
/** Checks whether the 'numberOfCopies' field has been set */
public boolean hasNumberOfCopies() {
return fieldSetFlags()[7];
}
/** Clears the value of the 'numberOfCopies' field */
public org.gel.models.report.avro.VariantCall.Builder clearNumberOfCopies() {
numberOfCopies = null;
fieldSetFlags()[7] = false;
return this;
}
/** Gets the value of the 'alleleOrigins' field */
public java.util.List getAlleleOrigins() {
return alleleOrigins;
}
/** Sets the value of the 'alleleOrigins' field */
public org.gel.models.report.avro.VariantCall.Builder setAlleleOrigins(java.util.List value) {
validate(fields()[8], value);
this.alleleOrigins = value;
fieldSetFlags()[8] = true;
return this;
}
/** Checks whether the 'alleleOrigins' field has been set */
public boolean hasAlleleOrigins() {
return fieldSetFlags()[8];
}
/** Clears the value of the 'alleleOrigins' field */
public org.gel.models.report.avro.VariantCall.Builder clearAlleleOrigins() {
alleleOrigins = null;
fieldSetFlags()[8] = false;
return this;
}
/** Gets the value of the 'supportingReadTypes' field */
public java.util.List getSupportingReadTypes() {
return supportingReadTypes;
}
/** Sets the value of the 'supportingReadTypes' field */
public org.gel.models.report.avro.VariantCall.Builder setSupportingReadTypes(java.util.List value) {
validate(fields()[9], value);
this.supportingReadTypes = value;
fieldSetFlags()[9] = true;
return this;
}
/** Checks whether the 'supportingReadTypes' field has been set */
public boolean hasSupportingReadTypes() {
return fieldSetFlags()[9];
}
/** Clears the value of the 'supportingReadTypes' field */
public org.gel.models.report.avro.VariantCall.Builder clearSupportingReadTypes() {
supportingReadTypes = null;
fieldSetFlags()[9] = false;
return this;
}
@Override
public VariantCall build() {
try {
VariantCall record = new VariantCall();
record.participantId = fieldSetFlags()[0] ? this.participantId : (java.lang.String) defaultValue(fields()[0]);
record.sampleId = fieldSetFlags()[1] ? this.sampleId : (java.lang.String) defaultValue(fields()[1]);
record.zygosity = fieldSetFlags()[2] ? this.zygosity : (org.gel.models.report.avro.Zygosity) defaultValue(fields()[2]);
record.phaseGenotype = fieldSetFlags()[3] ? this.phaseGenotype : (org.gel.models.report.avro.PhaseGenotype) defaultValue(fields()[3]);
record.sampleVariantAlleleFrequency = fieldSetFlags()[4] ? this.sampleVariantAlleleFrequency : (java.lang.Double) defaultValue(fields()[4]);
record.depthReference = fieldSetFlags()[5] ? this.depthReference : (java.lang.Integer) defaultValue(fields()[5]);
record.depthAlternate = fieldSetFlags()[6] ? this.depthAlternate : (java.lang.Integer) defaultValue(fields()[6]);
record.numberOfCopies = fieldSetFlags()[7] ? this.numberOfCopies : (java.util.List) defaultValue(fields()[7]);
record.alleleOrigins = fieldSetFlags()[8] ? this.alleleOrigins : (java.util.List) defaultValue(fields()[8]);
record.supportingReadTypes = fieldSetFlags()[9] ? this.supportingReadTypes : (java.util.List) defaultValue(fields()[9]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy