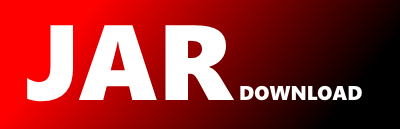
org.opencb.biodata.models.clinical.pedigree.Pedigree Maven / Gradle / Ivy
The newest version!
/*
*
*
*/
package org.opencb.biodata.models.clinical.pedigree;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.opencb.biodata.models.clinical.Disorder;
import org.opencb.biodata.models.clinical.Phenotype;
import java.util.*;
/**
* Created by imedina on 10/10/16.
*/
public class Pedigree {
private String name;
private List phenotypes;
private List disorders;
private List members;
private Member proband;
private Map attributes;
/**
* Empty constructor.
*/
public Pedigree() {
}
/**
* Constructor.
*
* @param name Family name
* @param members Individuals belonging to this family
* @param attributes Family attributes
*/
public Pedigree(String name, List members, Map attributes) {
this(name, members, null, Collections.emptyList(), Collections.emptyList(), attributes);
}
/**
* Constructor.
* @param name Family name
* @param members Individuals belonging to this family
* @param phenotypes List of phenotypes present in the members of the family
* @param disorders List of disorders present in the members of the family
* @param attributes Family attributes
*/
public Pedigree(String name, List members, List phenotypes, List disorders,
Map attributes) {
this(name, members, null, phenotypes, disorders, attributes);
}
/**
* Constructor.
* @param name Family name
* @param members Individuals belonging to this family
* @param proband Proband individual
* @param phenotypes List of phenotypes present in the members of the family
* @param disorders List of disorders present in the members of the family
* @param attributes Family attributes
*/
public Pedigree(String name, List members, Member proband, List phenotypes, List disorders,
Map attributes) {
this.name = name;
this.members = members;
this.proband = proband;
this.phenotypes = phenotypes;
this.disorders = disorders;
this.attributes = attributes;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("Pedigree{");
sb.append("name='").append(name).append('\'');
sb.append(", phenotypes=").append(phenotypes);
sb.append(", disorders=").append(disorders);
sb.append(", members=").append(members);
sb.append(", proband=").append(proband);
sb.append(", attributes=").append(attributes);
sb.append('}');
return sb.toString();
}
public String getName() {
return name;
}
public Pedigree setName(String name) {
this.name = name;
return this;
}
public List getPhenotypes() {
return phenotypes;
}
public Pedigree setPhenotypes(List phenotypes) {
this.phenotypes = phenotypes;
return this;
}
public List getDisorders() {
return disorders;
}
public Pedigree setDisorders(List disorders) {
this.disorders = disorders;
return this;
}
public List getMembers() {
return members;
}
public Pedigree setMembers(List members) {
this.members = members;
return this;
}
public Member getProband() {
return proband;
}
public Pedigree setProband(Member proband) {
this.proband = proband;
return this;
}
public Map getAttributes() {
return attributes;
}
public Pedigree setAttributes(Map attributes) {
this.attributes = attributes;
return this;
}
public String toJSON() {
try {
ObjectMapper mapper = new ObjectMapper();
return mapper.writerWithDefaultPrettyPrinter().writeValueAsString(this).toString();
} catch (Exception e) {
return "";
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy