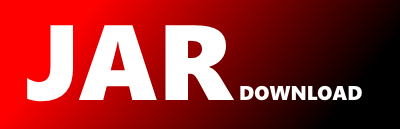
org.opencb.biodata.models.pedigree.Pedigree Maven / Gradle / Ivy
The newest version!
/*
*
*
*/
package org.opencb.biodata.models.pedigree;
import java.util.*;
/**
* @author Alejandro Aleman Ramos <[email protected]>
*/
public class Pedigree {
private Map individuals;
private Map> families;
private Map fields;
public Pedigree() {
individuals = new LinkedHashMap<>(100);
families = new LinkedHashMap<>(100);
fields = new LinkedHashMap<>(5);
}
public Set getFamily(String familyId) {
return families.get(familyId);
}
public Individual getIndividual(String id) {
return individuals.get(id);
}
public Map getIndividuals() {
return individuals;
}
public void setIndividuals(Map individuals) {
this.individuals = individuals;
}
public Map> getFamilies() {
return families;
}
public void setFamilies(Map> families) {
this.families = families;
}
public Map getFields() {
return fields;
}
public void setFields(Map fields) {
this.fields = fields;
}
public void addIndividual(Individual ind) {
this.individuals.put(ind.getId(), ind);
}
public void addIndividualToFamily(String familyId, Individual ind) {
this.families.get(familyId).add(ind);
}
public void addFamily(String familyId, Set family) {
this.getFamilies().put(familyId, family);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Pedigree\n");
if (fields.size() > 0) {
sb.append("fields = " + fields.keySet().toString() + "\n");
}
for (Map.Entry> elem : this.families.entrySet()) {
sb.append(elem.getKey() + "\n");
for (Individual ind : elem.getValue()) {
sb.append("\t" + ind.toString() + "\n");
}
}
return sb.toString();
}
public List getFamiliesTDT() {
List families = new ArrayList<>();
Individual ind;
for (Map.Entry entry : this.individuals.entrySet()) {
ind = entry.getValue();
if (ind.getFather() != null && ind.getMother() != null) {
addIndividualToFamily(ind, ind.getFather(), ind.getMother(), families);
}
}
return families;
}
private void addIndividualToFamily(Individual ind, Individual father, Individual mother, List families) {
boolean b = false;
Iterator it = families.iterator();
Family fam;
while (it.hasNext() && !b) {
fam = it.next();
if (fam.getFather().equals(father) && fam.getMother().equals(mother)) {
fam.addChild(ind);
b = true;
}
}
if (!b) {
fam = new Family(father, mother);
fam.addChild(ind);
families.add(fam);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy