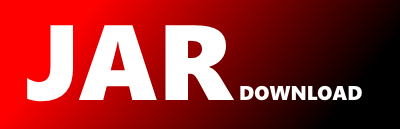
org.opencb.biodata.models.variant.avro.Drug Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.opencb.biodata.models.variant.avro;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class Drug extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"Drug\",\"namespace\":\"org.opencb.biodata.models.variant.avro\",\"fields\":[{\"name\":\"therapeuticContext\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"pathway\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"effect\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"association\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"status\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"evidence\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"bibliography\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"string\",\"avro.java.string\":\"String\"}}]}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private java.lang.String therapeuticContext;
private java.lang.String pathway;
private java.lang.String effect;
private java.lang.String association;
private java.lang.String status;
private java.lang.String evidence;
private java.util.List bibliography;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public Drug() {}
/**
* All-args constructor.
*/
public Drug(java.lang.String therapeuticContext, java.lang.String pathway, java.lang.String effect, java.lang.String association, java.lang.String status, java.lang.String evidence, java.util.List bibliography) {
this.therapeuticContext = therapeuticContext;
this.pathway = pathway;
this.effect = effect;
this.association = association;
this.status = status;
this.evidence = evidence;
this.bibliography = bibliography;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return therapeuticContext;
case 1: return pathway;
case 2: return effect;
case 3: return association;
case 4: return status;
case 5: return evidence;
case 6: return bibliography;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: therapeuticContext = (java.lang.String)value$; break;
case 1: pathway = (java.lang.String)value$; break;
case 2: effect = (java.lang.String)value$; break;
case 3: association = (java.lang.String)value$; break;
case 4: status = (java.lang.String)value$; break;
case 5: evidence = (java.lang.String)value$; break;
case 6: bibliography = (java.util.List)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'therapeuticContext' field.
*/
public java.lang.String getTherapeuticContext() {
return therapeuticContext;
}
/**
* Sets the value of the 'therapeuticContext' field.
* @param value the value to set.
*/
public void setTherapeuticContext(java.lang.String value) {
this.therapeuticContext = value;
}
/**
* Gets the value of the 'pathway' field.
*/
public java.lang.String getPathway() {
return pathway;
}
/**
* Sets the value of the 'pathway' field.
* @param value the value to set.
*/
public void setPathway(java.lang.String value) {
this.pathway = value;
}
/**
* Gets the value of the 'effect' field.
*/
public java.lang.String getEffect() {
return effect;
}
/**
* Sets the value of the 'effect' field.
* @param value the value to set.
*/
public void setEffect(java.lang.String value) {
this.effect = value;
}
/**
* Gets the value of the 'association' field.
*/
public java.lang.String getAssociation() {
return association;
}
/**
* Sets the value of the 'association' field.
* @param value the value to set.
*/
public void setAssociation(java.lang.String value) {
this.association = value;
}
/**
* Gets the value of the 'status' field.
*/
public java.lang.String getStatus() {
return status;
}
/**
* Sets the value of the 'status' field.
* @param value the value to set.
*/
public void setStatus(java.lang.String value) {
this.status = value;
}
/**
* Gets the value of the 'evidence' field.
*/
public java.lang.String getEvidence() {
return evidence;
}
/**
* Sets the value of the 'evidence' field.
* @param value the value to set.
*/
public void setEvidence(java.lang.String value) {
this.evidence = value;
}
/**
* Gets the value of the 'bibliography' field.
*/
public java.util.List getBibliography() {
return bibliography;
}
/**
* Sets the value of the 'bibliography' field.
* @param value the value to set.
*/
public void setBibliography(java.util.List value) {
this.bibliography = value;
}
/** Creates a new Drug RecordBuilder */
public static org.opencb.biodata.models.variant.avro.Drug.Builder newBuilder() {
return new org.opencb.biodata.models.variant.avro.Drug.Builder();
}
/** Creates a new Drug RecordBuilder by copying an existing Builder */
public static org.opencb.biodata.models.variant.avro.Drug.Builder newBuilder(org.opencb.biodata.models.variant.avro.Drug.Builder other) {
return new org.opencb.biodata.models.variant.avro.Drug.Builder(other);
}
/** Creates a new Drug RecordBuilder by copying an existing Drug instance */
public static org.opencb.biodata.models.variant.avro.Drug.Builder newBuilder(org.opencb.biodata.models.variant.avro.Drug other) {
return new org.opencb.biodata.models.variant.avro.Drug.Builder(other);
}
/**
* RecordBuilder for Drug instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String therapeuticContext;
private java.lang.String pathway;
private java.lang.String effect;
private java.lang.String association;
private java.lang.String status;
private java.lang.String evidence;
private java.util.List bibliography;
/** Creates a new Builder */
private Builder() {
super(org.opencb.biodata.models.variant.avro.Drug.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.opencb.biodata.models.variant.avro.Drug.Builder other) {
super(other);
if (isValidValue(fields()[0], other.therapeuticContext)) {
this.therapeuticContext = data().deepCopy(fields()[0].schema(), other.therapeuticContext);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.pathway)) {
this.pathway = data().deepCopy(fields()[1].schema(), other.pathway);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.effect)) {
this.effect = data().deepCopy(fields()[2].schema(), other.effect);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.association)) {
this.association = data().deepCopy(fields()[3].schema(), other.association);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.status)) {
this.status = data().deepCopy(fields()[4].schema(), other.status);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.evidence)) {
this.evidence = data().deepCopy(fields()[5].schema(), other.evidence);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.bibliography)) {
this.bibliography = data().deepCopy(fields()[6].schema(), other.bibliography);
fieldSetFlags()[6] = true;
}
}
/** Creates a Builder by copying an existing Drug instance */
private Builder(org.opencb.biodata.models.variant.avro.Drug other) {
super(org.opencb.biodata.models.variant.avro.Drug.SCHEMA$);
if (isValidValue(fields()[0], other.therapeuticContext)) {
this.therapeuticContext = data().deepCopy(fields()[0].schema(), other.therapeuticContext);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.pathway)) {
this.pathway = data().deepCopy(fields()[1].schema(), other.pathway);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.effect)) {
this.effect = data().deepCopy(fields()[2].schema(), other.effect);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.association)) {
this.association = data().deepCopy(fields()[3].schema(), other.association);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.status)) {
this.status = data().deepCopy(fields()[4].schema(), other.status);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.evidence)) {
this.evidence = data().deepCopy(fields()[5].schema(), other.evidence);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.bibliography)) {
this.bibliography = data().deepCopy(fields()[6].schema(), other.bibliography);
fieldSetFlags()[6] = true;
}
}
/** Gets the value of the 'therapeuticContext' field */
public java.lang.String getTherapeuticContext() {
return therapeuticContext;
}
/** Sets the value of the 'therapeuticContext' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setTherapeuticContext(java.lang.String value) {
validate(fields()[0], value);
this.therapeuticContext = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'therapeuticContext' field has been set */
public boolean hasTherapeuticContext() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'therapeuticContext' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearTherapeuticContext() {
therapeuticContext = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'pathway' field */
public java.lang.String getPathway() {
return pathway;
}
/** Sets the value of the 'pathway' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setPathway(java.lang.String value) {
validate(fields()[1], value);
this.pathway = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'pathway' field has been set */
public boolean hasPathway() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'pathway' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearPathway() {
pathway = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'effect' field */
public java.lang.String getEffect() {
return effect;
}
/** Sets the value of the 'effect' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setEffect(java.lang.String value) {
validate(fields()[2], value);
this.effect = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'effect' field has been set */
public boolean hasEffect() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'effect' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearEffect() {
effect = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'association' field */
public java.lang.String getAssociation() {
return association;
}
/** Sets the value of the 'association' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setAssociation(java.lang.String value) {
validate(fields()[3], value);
this.association = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'association' field has been set */
public boolean hasAssociation() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'association' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearAssociation() {
association = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'status' field */
public java.lang.String getStatus() {
return status;
}
/** Sets the value of the 'status' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setStatus(java.lang.String value) {
validate(fields()[4], value);
this.status = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'status' field has been set */
public boolean hasStatus() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'status' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearStatus() {
status = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'evidence' field */
public java.lang.String getEvidence() {
return evidence;
}
/** Sets the value of the 'evidence' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setEvidence(java.lang.String value) {
validate(fields()[5], value);
this.evidence = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'evidence' field has been set */
public boolean hasEvidence() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'evidence' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearEvidence() {
evidence = null;
fieldSetFlags()[5] = false;
return this;
}
/** Gets the value of the 'bibliography' field */
public java.util.List getBibliography() {
return bibliography;
}
/** Sets the value of the 'bibliography' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder setBibliography(java.util.List value) {
validate(fields()[6], value);
this.bibliography = value;
fieldSetFlags()[6] = true;
return this;
}
/** Checks whether the 'bibliography' field has been set */
public boolean hasBibliography() {
return fieldSetFlags()[6];
}
/** Clears the value of the 'bibliography' field */
public org.opencb.biodata.models.variant.avro.Drug.Builder clearBibliography() {
bibliography = null;
fieldSetFlags()[6] = false;
return this;
}
@Override
public Drug build() {
try {
Drug record = new Drug();
record.therapeuticContext = fieldSetFlags()[0] ? this.therapeuticContext : (java.lang.String) defaultValue(fields()[0]);
record.pathway = fieldSetFlags()[1] ? this.pathway : (java.lang.String) defaultValue(fields()[1]);
record.effect = fieldSetFlags()[2] ? this.effect : (java.lang.String) defaultValue(fields()[2]);
record.association = fieldSetFlags()[3] ? this.association : (java.lang.String) defaultValue(fields()[3]);
record.status = fieldSetFlags()[4] ? this.status : (java.lang.String) defaultValue(fields()[4]);
record.evidence = fieldSetFlags()[5] ? this.evidence : (java.lang.String) defaultValue(fields()[5]);
record.bibliography = fieldSetFlags()[6] ? this.bibliography : (java.util.List) defaultValue(fields()[6]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy