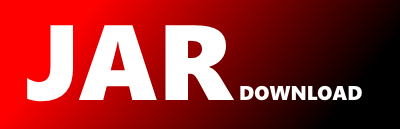
org.opencb.biodata.models.variant.avro.GwasAssociation Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.opencb.biodata.models.variant.avro;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class GwasAssociation extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"GwasAssociation\",\"namespace\":\"org.opencb.biodata.models.variant.avro\",\"fields\":[{\"name\":\"source\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"region\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"snpId\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"riskAllele\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"riskAlleleFrequency\",\"type\":[\"null\",\"double\"]},{\"name\":\"studies\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"GwasAssociationStudy\",\"fields\":[{\"name\":\"pubmedid\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"study\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"studyAccession\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"initialSampleSizeDescription\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"initialSampleSize\",\"type\":[\"null\",\"int\"]},{\"name\":\"platform\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"genotypingTechnology\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"traits\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"GwasAssociationStudyTrait\",\"fields\":[{\"name\":\"diseaseTrait\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"strongestSnpRiskAllele\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"string\",\"avro.java.string\":\"String\"}}]},{\"name\":\"ontologies\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"OntologyTermAnnotation\",\"fields\":[{\"name\":\"id\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"name\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"description\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"source\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"url\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"attributes\",\"type\":[\"null\",{\"type\":\"map\",\"values\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"avro.java.string\":\"String\"}]}]}}]},{\"name\":\"scores\",\"type\":[\"null\",{\"type\":\"array\",\"items\":{\"type\":\"record\",\"name\":\"GwasAssociationStudyTraitScores\",\"fields\":[{\"name\":\"pValue\",\"type\":[\"null\",\"double\"]},{\"name\":\"pValueMlog\",\"type\":[\"null\",\"double\"]},{\"name\":\"pValueText\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"orBeta\",\"type\":[\"null\",\"double\"]},{\"name\":\"percentCI\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]}]}}]}]}}]}]}}]}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private java.lang.String source;
private java.lang.String region;
private java.lang.String snpId;
private java.lang.String riskAllele;
private java.lang.Double riskAlleleFrequency;
private java.util.List studies;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public GwasAssociation() {}
/**
* All-args constructor.
*/
public GwasAssociation(java.lang.String source, java.lang.String region, java.lang.String snpId, java.lang.String riskAllele, java.lang.Double riskAlleleFrequency, java.util.List studies) {
this.source = source;
this.region = region;
this.snpId = snpId;
this.riskAllele = riskAllele;
this.riskAlleleFrequency = riskAlleleFrequency;
this.studies = studies;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return source;
case 1: return region;
case 2: return snpId;
case 3: return riskAllele;
case 4: return riskAlleleFrequency;
case 5: return studies;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: source = (java.lang.String)value$; break;
case 1: region = (java.lang.String)value$; break;
case 2: snpId = (java.lang.String)value$; break;
case 3: riskAllele = (java.lang.String)value$; break;
case 4: riskAlleleFrequency = (java.lang.Double)value$; break;
case 5: studies = (java.util.List)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'source' field.
*/
public java.lang.String getSource() {
return source;
}
/**
* Sets the value of the 'source' field.
* @param value the value to set.
*/
public void setSource(java.lang.String value) {
this.source = value;
}
/**
* Gets the value of the 'region' field.
*/
public java.lang.String getRegion() {
return region;
}
/**
* Sets the value of the 'region' field.
* @param value the value to set.
*/
public void setRegion(java.lang.String value) {
this.region = value;
}
/**
* Gets the value of the 'snpId' field.
*/
public java.lang.String getSnpId() {
return snpId;
}
/**
* Sets the value of the 'snpId' field.
* @param value the value to set.
*/
public void setSnpId(java.lang.String value) {
this.snpId = value;
}
/**
* Gets the value of the 'riskAllele' field.
*/
public java.lang.String getRiskAllele() {
return riskAllele;
}
/**
* Sets the value of the 'riskAllele' field.
* @param value the value to set.
*/
public void setRiskAllele(java.lang.String value) {
this.riskAllele = value;
}
/**
* Gets the value of the 'riskAlleleFrequency' field.
*/
public java.lang.Double getRiskAlleleFrequency() {
return riskAlleleFrequency;
}
/**
* Sets the value of the 'riskAlleleFrequency' field.
* @param value the value to set.
*/
public void setRiskAlleleFrequency(java.lang.Double value) {
this.riskAlleleFrequency = value;
}
/**
* Gets the value of the 'studies' field.
*/
public java.util.List getStudies() {
return studies;
}
/**
* Sets the value of the 'studies' field.
* @param value the value to set.
*/
public void setStudies(java.util.List value) {
this.studies = value;
}
/** Creates a new GwasAssociation RecordBuilder */
public static org.opencb.biodata.models.variant.avro.GwasAssociation.Builder newBuilder() {
return new org.opencb.biodata.models.variant.avro.GwasAssociation.Builder();
}
/** Creates a new GwasAssociation RecordBuilder by copying an existing Builder */
public static org.opencb.biodata.models.variant.avro.GwasAssociation.Builder newBuilder(org.opencb.biodata.models.variant.avro.GwasAssociation.Builder other) {
return new org.opencb.biodata.models.variant.avro.GwasAssociation.Builder(other);
}
/** Creates a new GwasAssociation RecordBuilder by copying an existing GwasAssociation instance */
public static org.opencb.biodata.models.variant.avro.GwasAssociation.Builder newBuilder(org.opencb.biodata.models.variant.avro.GwasAssociation other) {
return new org.opencb.biodata.models.variant.avro.GwasAssociation.Builder(other);
}
/**
* RecordBuilder for GwasAssociation instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String source;
private java.lang.String region;
private java.lang.String snpId;
private java.lang.String riskAllele;
private java.lang.Double riskAlleleFrequency;
private java.util.List studies;
/** Creates a new Builder */
private Builder() {
super(org.opencb.biodata.models.variant.avro.GwasAssociation.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.opencb.biodata.models.variant.avro.GwasAssociation.Builder other) {
super(other);
if (isValidValue(fields()[0], other.source)) {
this.source = data().deepCopy(fields()[0].schema(), other.source);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.region)) {
this.region = data().deepCopy(fields()[1].schema(), other.region);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.snpId)) {
this.snpId = data().deepCopy(fields()[2].schema(), other.snpId);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.riskAllele)) {
this.riskAllele = data().deepCopy(fields()[3].schema(), other.riskAllele);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.riskAlleleFrequency)) {
this.riskAlleleFrequency = data().deepCopy(fields()[4].schema(), other.riskAlleleFrequency);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.studies)) {
this.studies = data().deepCopy(fields()[5].schema(), other.studies);
fieldSetFlags()[5] = true;
}
}
/** Creates a Builder by copying an existing GwasAssociation instance */
private Builder(org.opencb.biodata.models.variant.avro.GwasAssociation other) {
super(org.opencb.biodata.models.variant.avro.GwasAssociation.SCHEMA$);
if (isValidValue(fields()[0], other.source)) {
this.source = data().deepCopy(fields()[0].schema(), other.source);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.region)) {
this.region = data().deepCopy(fields()[1].schema(), other.region);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.snpId)) {
this.snpId = data().deepCopy(fields()[2].schema(), other.snpId);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.riskAllele)) {
this.riskAllele = data().deepCopy(fields()[3].schema(), other.riskAllele);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.riskAlleleFrequency)) {
this.riskAlleleFrequency = data().deepCopy(fields()[4].schema(), other.riskAlleleFrequency);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.studies)) {
this.studies = data().deepCopy(fields()[5].schema(), other.studies);
fieldSetFlags()[5] = true;
}
}
/** Gets the value of the 'source' field */
public java.lang.String getSource() {
return source;
}
/** Sets the value of the 'source' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder setSource(java.lang.String value) {
validate(fields()[0], value);
this.source = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'source' field has been set */
public boolean hasSource() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'source' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder clearSource() {
source = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'region' field */
public java.lang.String getRegion() {
return region;
}
/** Sets the value of the 'region' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder setRegion(java.lang.String value) {
validate(fields()[1], value);
this.region = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'region' field has been set */
public boolean hasRegion() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'region' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder clearRegion() {
region = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'snpId' field */
public java.lang.String getSnpId() {
return snpId;
}
/** Sets the value of the 'snpId' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder setSnpId(java.lang.String value) {
validate(fields()[2], value);
this.snpId = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'snpId' field has been set */
public boolean hasSnpId() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'snpId' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder clearSnpId() {
snpId = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'riskAllele' field */
public java.lang.String getRiskAllele() {
return riskAllele;
}
/** Sets the value of the 'riskAllele' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder setRiskAllele(java.lang.String value) {
validate(fields()[3], value);
this.riskAllele = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'riskAllele' field has been set */
public boolean hasRiskAllele() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'riskAllele' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder clearRiskAllele() {
riskAllele = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'riskAlleleFrequency' field */
public java.lang.Double getRiskAlleleFrequency() {
return riskAlleleFrequency;
}
/** Sets the value of the 'riskAlleleFrequency' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder setRiskAlleleFrequency(java.lang.Double value) {
validate(fields()[4], value);
this.riskAlleleFrequency = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'riskAlleleFrequency' field has been set */
public boolean hasRiskAlleleFrequency() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'riskAlleleFrequency' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder clearRiskAlleleFrequency() {
riskAlleleFrequency = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'studies' field */
public java.util.List getStudies() {
return studies;
}
/** Sets the value of the 'studies' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder setStudies(java.util.List value) {
validate(fields()[5], value);
this.studies = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'studies' field has been set */
public boolean hasStudies() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'studies' field */
public org.opencb.biodata.models.variant.avro.GwasAssociation.Builder clearStudies() {
studies = null;
fieldSetFlags()[5] = false;
return this;
}
@Override
public GwasAssociation build() {
try {
GwasAssociation record = new GwasAssociation();
record.source = fieldSetFlags()[0] ? this.source : (java.lang.String) defaultValue(fields()[0]);
record.region = fieldSetFlags()[1] ? this.region : (java.lang.String) defaultValue(fields()[1]);
record.snpId = fieldSetFlags()[2] ? this.snpId : (java.lang.String) defaultValue(fields()[2]);
record.riskAllele = fieldSetFlags()[3] ? this.riskAllele : (java.lang.String) defaultValue(fields()[3]);
record.riskAlleleFrequency = fieldSetFlags()[4] ? this.riskAlleleFrequency : (java.lang.Double) defaultValue(fields()[4]);
record.studies = fieldSetFlags()[5] ? this.studies : (java.util.List) defaultValue(fields()[5]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy