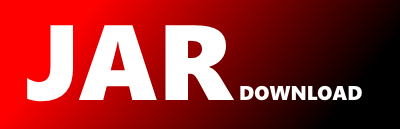
org.opencb.biodata.models.variant.avro.StructuralVariation Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.opencb.biodata.models.variant.avro;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class StructuralVariation extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"StructuralVariation\",\"namespace\":\"org.opencb.biodata.models.variant.avro\",\"fields\":[{\"name\":\"ciStartLeft\",\"type\":[\"null\",\"int\"]},{\"name\":\"ciStartRight\",\"type\":[\"null\",\"int\"]},{\"name\":\"ciEndLeft\",\"type\":[\"null\",\"int\"]},{\"name\":\"ciEndRight\",\"type\":[\"null\",\"int\"]},{\"name\":\"copyNumber\",\"type\":[\"null\",\"int\"],\"doc\":\"* Number of copies for CNV variants.\"},{\"name\":\"leftSvInsSeq\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"* Inserted sequence for long INS\\n *\"},{\"name\":\"rightSvInsSeq\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"type\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"StructuralVariantType\",\"doc\":\"* @Deprecated, use VariantType instead\",\"symbols\":[\"COPY_NUMBER_GAIN\",\"COPY_NUMBER_LOSS\",\"TANDEM_DUPLICATION\"],\"javaAnnotation\":\"Deprecated\"}],\"doc\":\"* @deprecated\",\"javaAnnotation\":\"Deprecated\"},{\"name\":\"breakend\",\"type\":[\"null\",{\"type\":\"record\",\"name\":\"Breakend\",\"fields\":[{\"name\":\"mate\",\"type\":[\"null\",{\"type\":\"record\",\"name\":\"BreakendMate\",\"fields\":[{\"name\":\"chromosome\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"position\",\"type\":[\"null\",\"int\"]},{\"name\":\"ciPositionLeft\",\"type\":[\"null\",\"int\"]},{\"name\":\"ciPositionRight\",\"type\":[\"null\",\"int\"]}]}]},{\"name\":\"orientation\",\"type\":[\"null\",{\"type\":\"enum\",\"name\":\"BreakendOrientation\",\"doc\":\"* SE | (Start -> End) | s | t[p[ | piece extending to the right of p is joined after t\\n * SS | (Start -> Start) | s | t]p] | reverse comp piece extending left of p is joined after t\\n * ES | (End -> Start) | s | ]p]t | piece extending to the left of p is joined before t\\n * EE | (End -> End) | s | [p[t | reverse comp piece extending right of p is joined before t\",\"symbols\":[\"SE\",\"SS\",\"ES\",\"EE\"]}]},{\"name\":\"insSeq\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]}]}],\"default\":null}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private java.lang.Integer ciStartLeft;
private java.lang.Integer ciStartRight;
private java.lang.Integer ciEndLeft;
private java.lang.Integer ciEndRight;
/** * Number of copies for CNV variants. */
private java.lang.Integer copyNumber;
/** * Inserted sequence for long INS
* */
private java.lang.String leftSvInsSeq;
private java.lang.String rightSvInsSeq;
/** * @deprecated */
@Deprecated
private org.opencb.biodata.models.variant.avro.StructuralVariantType type;
private org.opencb.biodata.models.variant.avro.Breakend breakend;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public StructuralVariation() {}
/**
* All-args constructor.
*/
public StructuralVariation(java.lang.Integer ciStartLeft, java.lang.Integer ciStartRight, java.lang.Integer ciEndLeft, java.lang.Integer ciEndRight, java.lang.Integer copyNumber, java.lang.String leftSvInsSeq, java.lang.String rightSvInsSeq, org.opencb.biodata.models.variant.avro.StructuralVariantType type, org.opencb.biodata.models.variant.avro.Breakend breakend) {
this.ciStartLeft = ciStartLeft;
this.ciStartRight = ciStartRight;
this.ciEndLeft = ciEndLeft;
this.ciEndRight = ciEndRight;
this.copyNumber = copyNumber;
this.leftSvInsSeq = leftSvInsSeq;
this.rightSvInsSeq = rightSvInsSeq;
this.type = type;
this.breakend = breakend;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return ciStartLeft;
case 1: return ciStartRight;
case 2: return ciEndLeft;
case 3: return ciEndRight;
case 4: return copyNumber;
case 5: return leftSvInsSeq;
case 6: return rightSvInsSeq;
case 7: return type;
case 8: return breakend;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: ciStartLeft = (java.lang.Integer)value$; break;
case 1: ciStartRight = (java.lang.Integer)value$; break;
case 2: ciEndLeft = (java.lang.Integer)value$; break;
case 3: ciEndRight = (java.lang.Integer)value$; break;
case 4: copyNumber = (java.lang.Integer)value$; break;
case 5: leftSvInsSeq = (java.lang.String)value$; break;
case 6: rightSvInsSeq = (java.lang.String)value$; break;
case 7: type = (org.opencb.biodata.models.variant.avro.StructuralVariantType)value$; break;
case 8: breakend = (org.opencb.biodata.models.variant.avro.Breakend)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'ciStartLeft' field.
*/
public java.lang.Integer getCiStartLeft() {
return ciStartLeft;
}
/**
* Sets the value of the 'ciStartLeft' field.
* @param value the value to set.
*/
public void setCiStartLeft(java.lang.Integer value) {
this.ciStartLeft = value;
}
/**
* Gets the value of the 'ciStartRight' field.
*/
public java.lang.Integer getCiStartRight() {
return ciStartRight;
}
/**
* Sets the value of the 'ciStartRight' field.
* @param value the value to set.
*/
public void setCiStartRight(java.lang.Integer value) {
this.ciStartRight = value;
}
/**
* Gets the value of the 'ciEndLeft' field.
*/
public java.lang.Integer getCiEndLeft() {
return ciEndLeft;
}
/**
* Sets the value of the 'ciEndLeft' field.
* @param value the value to set.
*/
public void setCiEndLeft(java.lang.Integer value) {
this.ciEndLeft = value;
}
/**
* Gets the value of the 'ciEndRight' field.
*/
public java.lang.Integer getCiEndRight() {
return ciEndRight;
}
/**
* Sets the value of the 'ciEndRight' field.
* @param value the value to set.
*/
public void setCiEndRight(java.lang.Integer value) {
this.ciEndRight = value;
}
/**
* Gets the value of the 'copyNumber' field.
* * Number of copies for CNV variants. */
public java.lang.Integer getCopyNumber() {
return copyNumber;
}
/**
* Sets the value of the 'copyNumber' field.
* * Number of copies for CNV variants. * @param value the value to set.
*/
public void setCopyNumber(java.lang.Integer value) {
this.copyNumber = value;
}
/**
* Gets the value of the 'leftSvInsSeq' field.
* * Inserted sequence for long INS
* */
public java.lang.String getLeftSvInsSeq() {
return leftSvInsSeq;
}
/**
* Sets the value of the 'leftSvInsSeq' field.
* * Inserted sequence for long INS
* * @param value the value to set.
*/
public void setLeftSvInsSeq(java.lang.String value) {
this.leftSvInsSeq = value;
}
/**
* Gets the value of the 'rightSvInsSeq' field.
*/
public java.lang.String getRightSvInsSeq() {
return rightSvInsSeq;
}
/**
* Sets the value of the 'rightSvInsSeq' field.
* @param value the value to set.
*/
public void setRightSvInsSeq(java.lang.String value) {
this.rightSvInsSeq = value;
}
/**
* Gets the value of the 'type' field.
* * @deprecated */
public org.opencb.biodata.models.variant.avro.StructuralVariantType getType() {
return type;
}
/**
* Sets the value of the 'type' field.
* * @deprecated * @param value the value to set.
*/
public void setType(org.opencb.biodata.models.variant.avro.StructuralVariantType value) {
this.type = value;
}
/**
* Gets the value of the 'breakend' field.
*/
public org.opencb.biodata.models.variant.avro.Breakend getBreakend() {
return breakend;
}
/**
* Sets the value of the 'breakend' field.
* @param value the value to set.
*/
public void setBreakend(org.opencb.biodata.models.variant.avro.Breakend value) {
this.breakend = value;
}
/** Creates a new StructuralVariation RecordBuilder */
public static org.opencb.biodata.models.variant.avro.StructuralVariation.Builder newBuilder() {
return new org.opencb.biodata.models.variant.avro.StructuralVariation.Builder();
}
/** Creates a new StructuralVariation RecordBuilder by copying an existing Builder */
public static org.opencb.biodata.models.variant.avro.StructuralVariation.Builder newBuilder(org.opencb.biodata.models.variant.avro.StructuralVariation.Builder other) {
return new org.opencb.biodata.models.variant.avro.StructuralVariation.Builder(other);
}
/** Creates a new StructuralVariation RecordBuilder by copying an existing StructuralVariation instance */
public static org.opencb.biodata.models.variant.avro.StructuralVariation.Builder newBuilder(org.opencb.biodata.models.variant.avro.StructuralVariation other) {
return new org.opencb.biodata.models.variant.avro.StructuralVariation.Builder(other);
}
/**
* RecordBuilder for StructuralVariation instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.Integer ciStartLeft;
private java.lang.Integer ciStartRight;
private java.lang.Integer ciEndLeft;
private java.lang.Integer ciEndRight;
private java.lang.Integer copyNumber;
private java.lang.String leftSvInsSeq;
private java.lang.String rightSvInsSeq;
private org.opencb.biodata.models.variant.avro.StructuralVariantType type;
private org.opencb.biodata.models.variant.avro.Breakend breakend;
/** Creates a new Builder */
private Builder() {
super(org.opencb.biodata.models.variant.avro.StructuralVariation.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.opencb.biodata.models.variant.avro.StructuralVariation.Builder other) {
super(other);
if (isValidValue(fields()[0], other.ciStartLeft)) {
this.ciStartLeft = data().deepCopy(fields()[0].schema(), other.ciStartLeft);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.ciStartRight)) {
this.ciStartRight = data().deepCopy(fields()[1].schema(), other.ciStartRight);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.ciEndLeft)) {
this.ciEndLeft = data().deepCopy(fields()[2].schema(), other.ciEndLeft);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.ciEndRight)) {
this.ciEndRight = data().deepCopy(fields()[3].schema(), other.ciEndRight);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.copyNumber)) {
this.copyNumber = data().deepCopy(fields()[4].schema(), other.copyNumber);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.leftSvInsSeq)) {
this.leftSvInsSeq = data().deepCopy(fields()[5].schema(), other.leftSvInsSeq);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.rightSvInsSeq)) {
this.rightSvInsSeq = data().deepCopy(fields()[6].schema(), other.rightSvInsSeq);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.type)) {
this.type = data().deepCopy(fields()[7].schema(), other.type);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.breakend)) {
this.breakend = data().deepCopy(fields()[8].schema(), other.breakend);
fieldSetFlags()[8] = true;
}
}
/** Creates a Builder by copying an existing StructuralVariation instance */
private Builder(org.opencb.biodata.models.variant.avro.StructuralVariation other) {
super(org.opencb.biodata.models.variant.avro.StructuralVariation.SCHEMA$);
if (isValidValue(fields()[0], other.ciStartLeft)) {
this.ciStartLeft = data().deepCopy(fields()[0].schema(), other.ciStartLeft);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.ciStartRight)) {
this.ciStartRight = data().deepCopy(fields()[1].schema(), other.ciStartRight);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.ciEndLeft)) {
this.ciEndLeft = data().deepCopy(fields()[2].schema(), other.ciEndLeft);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.ciEndRight)) {
this.ciEndRight = data().deepCopy(fields()[3].schema(), other.ciEndRight);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.copyNumber)) {
this.copyNumber = data().deepCopy(fields()[4].schema(), other.copyNumber);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.leftSvInsSeq)) {
this.leftSvInsSeq = data().deepCopy(fields()[5].schema(), other.leftSvInsSeq);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.rightSvInsSeq)) {
this.rightSvInsSeq = data().deepCopy(fields()[6].schema(), other.rightSvInsSeq);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.type)) {
this.type = data().deepCopy(fields()[7].schema(), other.type);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.breakend)) {
this.breakend = data().deepCopy(fields()[8].schema(), other.breakend);
fieldSetFlags()[8] = true;
}
}
/** Gets the value of the 'ciStartLeft' field */
public java.lang.Integer getCiStartLeft() {
return ciStartLeft;
}
/** Sets the value of the 'ciStartLeft' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setCiStartLeft(java.lang.Integer value) {
validate(fields()[0], value);
this.ciStartLeft = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'ciStartLeft' field has been set */
public boolean hasCiStartLeft() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'ciStartLeft' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearCiStartLeft() {
ciStartLeft = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'ciStartRight' field */
public java.lang.Integer getCiStartRight() {
return ciStartRight;
}
/** Sets the value of the 'ciStartRight' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setCiStartRight(java.lang.Integer value) {
validate(fields()[1], value);
this.ciStartRight = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'ciStartRight' field has been set */
public boolean hasCiStartRight() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'ciStartRight' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearCiStartRight() {
ciStartRight = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'ciEndLeft' field */
public java.lang.Integer getCiEndLeft() {
return ciEndLeft;
}
/** Sets the value of the 'ciEndLeft' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setCiEndLeft(java.lang.Integer value) {
validate(fields()[2], value);
this.ciEndLeft = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'ciEndLeft' field has been set */
public boolean hasCiEndLeft() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'ciEndLeft' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearCiEndLeft() {
ciEndLeft = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'ciEndRight' field */
public java.lang.Integer getCiEndRight() {
return ciEndRight;
}
/** Sets the value of the 'ciEndRight' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setCiEndRight(java.lang.Integer value) {
validate(fields()[3], value);
this.ciEndRight = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'ciEndRight' field has been set */
public boolean hasCiEndRight() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'ciEndRight' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearCiEndRight() {
ciEndRight = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'copyNumber' field */
public java.lang.Integer getCopyNumber() {
return copyNumber;
}
/** Sets the value of the 'copyNumber' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setCopyNumber(java.lang.Integer value) {
validate(fields()[4], value);
this.copyNumber = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'copyNumber' field has been set */
public boolean hasCopyNumber() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'copyNumber' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearCopyNumber() {
copyNumber = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'leftSvInsSeq' field */
public java.lang.String getLeftSvInsSeq() {
return leftSvInsSeq;
}
/** Sets the value of the 'leftSvInsSeq' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setLeftSvInsSeq(java.lang.String value) {
validate(fields()[5], value);
this.leftSvInsSeq = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'leftSvInsSeq' field has been set */
public boolean hasLeftSvInsSeq() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'leftSvInsSeq' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearLeftSvInsSeq() {
leftSvInsSeq = null;
fieldSetFlags()[5] = false;
return this;
}
/** Gets the value of the 'rightSvInsSeq' field */
public java.lang.String getRightSvInsSeq() {
return rightSvInsSeq;
}
/** Sets the value of the 'rightSvInsSeq' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setRightSvInsSeq(java.lang.String value) {
validate(fields()[6], value);
this.rightSvInsSeq = value;
fieldSetFlags()[6] = true;
return this;
}
/** Checks whether the 'rightSvInsSeq' field has been set */
public boolean hasRightSvInsSeq() {
return fieldSetFlags()[6];
}
/** Clears the value of the 'rightSvInsSeq' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearRightSvInsSeq() {
rightSvInsSeq = null;
fieldSetFlags()[6] = false;
return this;
}
/** Gets the value of the 'type' field */
public org.opencb.biodata.models.variant.avro.StructuralVariantType getType() {
return type;
}
/** Sets the value of the 'type' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setType(org.opencb.biodata.models.variant.avro.StructuralVariantType value) {
validate(fields()[7], value);
this.type = value;
fieldSetFlags()[7] = true;
return this;
}
/** Checks whether the 'type' field has been set */
public boolean hasType() {
return fieldSetFlags()[7];
}
/** Clears the value of the 'type' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearType() {
type = null;
fieldSetFlags()[7] = false;
return this;
}
/** Gets the value of the 'breakend' field */
public org.opencb.biodata.models.variant.avro.Breakend getBreakend() {
return breakend;
}
/** Sets the value of the 'breakend' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder setBreakend(org.opencb.biodata.models.variant.avro.Breakend value) {
validate(fields()[8], value);
this.breakend = value;
fieldSetFlags()[8] = true;
return this;
}
/** Checks whether the 'breakend' field has been set */
public boolean hasBreakend() {
return fieldSetFlags()[8];
}
/** Clears the value of the 'breakend' field */
public org.opencb.biodata.models.variant.avro.StructuralVariation.Builder clearBreakend() {
breakend = null;
fieldSetFlags()[8] = false;
return this;
}
@Override
public StructuralVariation build() {
try {
StructuralVariation record = new StructuralVariation();
record.ciStartLeft = fieldSetFlags()[0] ? this.ciStartLeft : (java.lang.Integer) defaultValue(fields()[0]);
record.ciStartRight = fieldSetFlags()[1] ? this.ciStartRight : (java.lang.Integer) defaultValue(fields()[1]);
record.ciEndLeft = fieldSetFlags()[2] ? this.ciEndLeft : (java.lang.Integer) defaultValue(fields()[2]);
record.ciEndRight = fieldSetFlags()[3] ? this.ciEndRight : (java.lang.Integer) defaultValue(fields()[3]);
record.copyNumber = fieldSetFlags()[4] ? this.copyNumber : (java.lang.Integer) defaultValue(fields()[4]);
record.leftSvInsSeq = fieldSetFlags()[5] ? this.leftSvInsSeq : (java.lang.String) defaultValue(fields()[5]);
record.rightSvInsSeq = fieldSetFlags()[6] ? this.rightSvInsSeq : (java.lang.String) defaultValue(fields()[6]);
record.type = fieldSetFlags()[7] ? this.type : (org.opencb.biodata.models.variant.avro.StructuralVariantType) defaultValue(fields()[7]);
record.breakend = fieldSetFlags()[8] ? this.breakend : (org.opencb.biodata.models.variant.avro.Breakend) defaultValue(fields()[8]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy