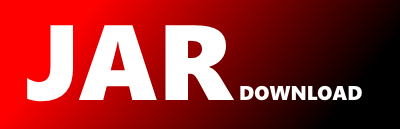
org.opencb.biodata.models.variant.metadata.VariantStudyStats Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.opencb.biodata.models.variant.metadata;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class VariantStudyStats extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"VariantStudyStats\",\"namespace\":\"org.opencb.biodata.models.variant.metadata\",\"fields\":[{\"name\":\"sampleStats\",\"type\":{\"type\":\"map\",\"values\":{\"type\":\"record\",\"name\":\"VariantSetStats\",\"doc\":\"Variant statistics for a set of variants.\\n The variants set can be contain a whole study, a cohort, a sample, a region, ...\",\"fields\":[{\"name\":\"variantCount\",\"type\":\"long\",\"doc\":\"Number of variants in the variant set\"},{\"name\":\"sampleCount\",\"type\":\"long\",\"doc\":\"Number of samples in the variant set\"},{\"name\":\"filterCount\",\"type\":{\"type\":\"map\",\"values\":\"long\",\"avro.java.string\":\"String\"},\"doc\":\"* The number of occurrences for each FILTER value in files from this set.\\n * Each file can contain more than one filter value (usually separated by ';').\\n *\"},{\"name\":\"genotypeCount\",\"type\":{\"type\":\"map\",\"values\":\"long\",\"avro.java.string\":\"String\"},\"doc\":\"Number of genotypes found for all samples in variants set\",\"default\":{}},{\"name\":\"filesCount\",\"type\":\"long\",\"doc\":\"Number of files in the variant set\"},{\"name\":\"tiTvRatio\",\"type\":\"float\",\"doc\":\"TiTvRatio = num. transitions / num. transversions\"},{\"name\":\"qualityAvg\",\"type\":\"float\",\"doc\":\"Mean Quality for all the variants with quality\"},{\"name\":\"qualityStdDev\",\"type\":\"float\",\"doc\":\"Standard Deviation of the quality\"},{\"name\":\"typeCount\",\"type\":{\"type\":\"map\",\"values\":\"long\",\"avro.java.string\":\"String\"},\"doc\":\"Variants count group by type. e.g. SNP, INDEL, MNP, SNV, ...\",\"default\":{}},{\"name\":\"biotypeCount\",\"type\":{\"type\":\"map\",\"values\":\"long\",\"avro.java.string\":\"String\"},\"doc\":\"Variants count group by biotype. e.g. protein-coding, miRNA, lncRNA, ...\",\"default\":{}},{\"name\":\"consequenceTypeCount\",\"type\":{\"type\":\"map\",\"values\":\"long\",\"avro.java.string\":\"String\"},\"doc\":\"Variants count group by consequence type. e.g. synonymous_variant, missense_variant, stop_lost, ...\",\"default\":{}},{\"name\":\"chromosomeCount\",\"type\":{\"type\":\"map\",\"values\":\"long\",\"avro.java.string\":\"String\"},\"doc\":\"Number of variants per chromosome\",\"default\":{}},{\"name\":\"chromosomeDensity\",\"type\":{\"type\":\"map\",\"values\":\"float\",\"avro.java.string\":\"String\"},\"doc\":\"Total density of variants within the chromosome. counts / chromosome.length\",\"default\":{}}]},\"avro.java.string\":\"String\"},\"default\":{}},{\"name\":\"cohortStats\",\"type\":{\"type\":\"map\",\"values\":\"VariantSetStats\",\"avro.java.string\":\"String\"},\"default\":{}}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private java.util.Map sampleStats;
private java.util.Map cohortStats;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public VariantStudyStats() {}
/**
* All-args constructor.
*/
public VariantStudyStats(java.util.Map sampleStats, java.util.Map cohortStats) {
this.sampleStats = sampleStats;
this.cohortStats = cohortStats;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return sampleStats;
case 1: return cohortStats;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: sampleStats = (java.util.Map)value$; break;
case 1: cohortStats = (java.util.Map)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'sampleStats' field.
*/
public java.util.Map getSampleStats() {
return sampleStats;
}
/**
* Sets the value of the 'sampleStats' field.
* @param value the value to set.
*/
public void setSampleStats(java.util.Map value) {
this.sampleStats = value;
}
/**
* Gets the value of the 'cohortStats' field.
*/
public java.util.Map getCohortStats() {
return cohortStats;
}
/**
* Sets the value of the 'cohortStats' field.
* @param value the value to set.
*/
public void setCohortStats(java.util.Map value) {
this.cohortStats = value;
}
/** Creates a new VariantStudyStats RecordBuilder */
public static org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder newBuilder() {
return new org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder();
}
/** Creates a new VariantStudyStats RecordBuilder by copying an existing Builder */
public static org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder newBuilder(org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder other) {
return new org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder(other);
}
/** Creates a new VariantStudyStats RecordBuilder by copying an existing VariantStudyStats instance */
public static org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder newBuilder(org.opencb.biodata.models.variant.metadata.VariantStudyStats other) {
return new org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder(other);
}
/**
* RecordBuilder for VariantStudyStats instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.util.Map sampleStats;
private java.util.Map cohortStats;
/** Creates a new Builder */
private Builder() {
super(org.opencb.biodata.models.variant.metadata.VariantStudyStats.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder other) {
super(other);
if (isValidValue(fields()[0], other.sampleStats)) {
this.sampleStats = data().deepCopy(fields()[0].schema(), other.sampleStats);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.cohortStats)) {
this.cohortStats = data().deepCopy(fields()[1].schema(), other.cohortStats);
fieldSetFlags()[1] = true;
}
}
/** Creates a Builder by copying an existing VariantStudyStats instance */
private Builder(org.opencb.biodata.models.variant.metadata.VariantStudyStats other) {
super(org.opencb.biodata.models.variant.metadata.VariantStudyStats.SCHEMA$);
if (isValidValue(fields()[0], other.sampleStats)) {
this.sampleStats = data().deepCopy(fields()[0].schema(), other.sampleStats);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.cohortStats)) {
this.cohortStats = data().deepCopy(fields()[1].schema(), other.cohortStats);
fieldSetFlags()[1] = true;
}
}
/** Gets the value of the 'sampleStats' field */
public java.util.Map getSampleStats() {
return sampleStats;
}
/** Sets the value of the 'sampleStats' field */
public org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder setSampleStats(java.util.Map value) {
validate(fields()[0], value);
this.sampleStats = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'sampleStats' field has been set */
public boolean hasSampleStats() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'sampleStats' field */
public org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder clearSampleStats() {
sampleStats = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'cohortStats' field */
public java.util.Map getCohortStats() {
return cohortStats;
}
/** Sets the value of the 'cohortStats' field */
public org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder setCohortStats(java.util.Map value) {
validate(fields()[1], value);
this.cohortStats = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'cohortStats' field has been set */
public boolean hasCohortStats() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'cohortStats' field */
public org.opencb.biodata.models.variant.metadata.VariantStudyStats.Builder clearCohortStats() {
cohortStats = null;
fieldSetFlags()[1] = false;
return this;
}
@Override
public VariantStudyStats build() {
try {
VariantStudyStats record = new VariantStudyStats();
record.sampleStats = fieldSetFlags()[0] ? this.sampleStats : (java.util.Map) defaultValue(fields()[0]);
record.cohortStats = fieldSetFlags()[1] ? this.cohortStats : (java.util.Map) defaultValue(fields()[1]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy