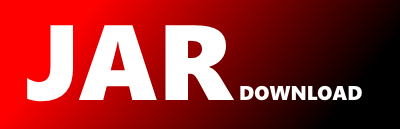
org.opencb.biodata.models.variant.protobuf.VariantProto Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protobuf/opencb/variant.proto
package org.opencb.biodata.models.variant.protobuf;
public final class VariantProto {
private VariantProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
**
* Type of variation, which depends mostly on its length.
* <ul>
* <li>SNVs involve a single nucleotide, without changes in length</li>
* <li>MNVs involve multiple nucleotides, without changes in length</li>
* <li>Indels are insertions or deletions of less than SV_THRESHOLD (50) nucleotides</li>
* <li>Structural variations are large changes of more than SV_THRESHOLD nucleotides</li>
* <li>Copy-number variations alter the number of copies of a region</li>
* </ul>
*
*
* Protobuf enum {@code protobuf.opencb.VariantType}
*/
public enum VariantType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* As the NO_VARIATION is the most common value on gVCFs, being the first value,
* protobuf will use this as default value and save some space.
*
*
* NO_VARIATION = 0;
*/
NO_VARIATION(0),
/**
*
* SO:0001483
*
*
* SNV = 2;
*/
SNV(2),
/**
*
* SO:0002007
*
*
* MNV = 4;
*/
MNV(4),
/**
*
* SO:1000032
*
*
* INDEL = 5;
*/
INDEL(5),
/**
*
* SO:0001537
*
*
* SV = 6;
*/
SV(6),
/**
*
* SO:0001019
*
*
* COPY_NUMBER = 7;
*/
COPY_NUMBER(7),
/**
*
* SO:0001742
*
*
* COPY_NUMBER_GAIN = 16;
*/
COPY_NUMBER_GAIN(16),
/**
*
* SO:0001743
*
*
* COPY_NUMBER_LOSS = 17;
*/
COPY_NUMBER_LOSS(17),
/**
*
* Defined in HTSJDK
*
*
* SYMBOLIC = 8;
*/
SYMBOLIC(8),
/**
*
* Defined in HTSJDK
*
*
* MIXED = 9;
*/
MIXED(9),
/**
*
* SO:0000667
*
*
* INSERTION = 10;
*/
INSERTION(10),
/**
*
* SO:0000159
*
*
* DELETION = 11;
*/
DELETION(11),
/**
*
* SO:0000199
*
*
* TRANSLOCATION = 12;
*/
TRANSLOCATION(12),
/**
*
* SO:1000036
*
*
* INVERSION = 13;
*/
INVERSION(13),
/**
*
* SO:1000035
*
*
* DUPLICATION = 14;
*/
DUPLICATION(14),
/**
*
* SO:1000173
*
*
* TANDEM_DUPLICATION = 18;
*/
TANDEM_DUPLICATION(18),
/**
* BREAKEND = 15;
*/
BREAKEND(15),
/**
*
* Deprecated
*
*
* CNV = 20;
*/
CNV(20),
/**
*
* Deprecated
*
*
* SNP = 1;
*/
SNP(1),
/**
*
* Deprecated
*
*
* MNP = 3;
*/
MNP(3),
UNRECOGNIZED(-1),
;
/**
*
* As the NO_VARIATION is the most common value on gVCFs, being the first value,
* protobuf will use this as default value and save some space.
*
*
* NO_VARIATION = 0;
*/
public static final int NO_VARIATION_VALUE = 0;
/**
*
* SO:0001483
*
*
* SNV = 2;
*/
public static final int SNV_VALUE = 2;
/**
*
* SO:0002007
*
*
* MNV = 4;
*/
public static final int MNV_VALUE = 4;
/**
*
* SO:1000032
*
*
* INDEL = 5;
*/
public static final int INDEL_VALUE = 5;
/**
*
* SO:0001537
*
*
* SV = 6;
*/
public static final int SV_VALUE = 6;
/**
*
* SO:0001019
*
*
* COPY_NUMBER = 7;
*/
public static final int COPY_NUMBER_VALUE = 7;
/**
*
* SO:0001742
*
*
* COPY_NUMBER_GAIN = 16;
*/
public static final int COPY_NUMBER_GAIN_VALUE = 16;
/**
*
* SO:0001743
*
*
* COPY_NUMBER_LOSS = 17;
*/
public static final int COPY_NUMBER_LOSS_VALUE = 17;
/**
*
* Defined in HTSJDK
*
*
* SYMBOLIC = 8;
*/
public static final int SYMBOLIC_VALUE = 8;
/**
*
* Defined in HTSJDK
*
*
* MIXED = 9;
*/
public static final int MIXED_VALUE = 9;
/**
*
* SO:0000667
*
*
* INSERTION = 10;
*/
public static final int INSERTION_VALUE = 10;
/**
*
* SO:0000159
*
*
* DELETION = 11;
*/
public static final int DELETION_VALUE = 11;
/**
*
* SO:0000199
*
*
* TRANSLOCATION = 12;
*/
public static final int TRANSLOCATION_VALUE = 12;
/**
*
* SO:1000036
*
*
* INVERSION = 13;
*/
public static final int INVERSION_VALUE = 13;
/**
*
* SO:1000035
*
*
* DUPLICATION = 14;
*/
public static final int DUPLICATION_VALUE = 14;
/**
*
* SO:1000173
*
*
* TANDEM_DUPLICATION = 18;
*/
public static final int TANDEM_DUPLICATION_VALUE = 18;
/**
* BREAKEND = 15;
*/
public static final int BREAKEND_VALUE = 15;
/**
*
* Deprecated
*
*
* CNV = 20;
*/
public static final int CNV_VALUE = 20;
/**
*
* Deprecated
*
*
* SNP = 1;
*/
public static final int SNP_VALUE = 1;
/**
*
* Deprecated
*
*
* MNP = 3;
*/
public static final int MNP_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static VariantType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static VariantType forNumber(int value) {
switch (value) {
case 0: return NO_VARIATION;
case 2: return SNV;
case 4: return MNV;
case 5: return INDEL;
case 6: return SV;
case 7: return COPY_NUMBER;
case 16: return COPY_NUMBER_GAIN;
case 17: return COPY_NUMBER_LOSS;
case 8: return SYMBOLIC;
case 9: return MIXED;
case 10: return INSERTION;
case 11: return DELETION;
case 12: return TRANSLOCATION;
case 13: return INVERSION;
case 14: return DUPLICATION;
case 18: return TANDEM_DUPLICATION;
case 15: return BREAKEND;
case 20: return CNV;
case 1: return SNP;
case 3: return MNP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
VariantType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public VariantType findValueByNumber(int number) {
return VariantType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.getDescriptor().getEnumTypes().get(0);
}
private static final VariantType[] VALUES = values();
public static VariantType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private VariantType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:protobuf.opencb.VariantType)
}
/**
*
* SE | (Start -> End) | s | t[p[ | piece extending to the right of p is joined after t
* SS | (Start -> Start) | s | t]p] | reverse comp piece extending left of p is joined after t
* ES | (End -> Start) | s | ]p]t | piece extending to the left of p is joined before t
* EE | (End -> End) | s | [p[t | reverse comp piece extending right of p is joined before t
*
*
* Protobuf enum {@code protobuf.opencb.BreakendOrientation}
*/
public enum BreakendOrientation
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SE = 0;
*/
SE(0),
/**
* SS = 1;
*/
SS(1),
/**
* ES = 2;
*/
ES(2),
/**
* EE = 3;
*/
EE(3),
UNRECOGNIZED(-1),
;
/**
* SE = 0;
*/
public static final int SE_VALUE = 0;
/**
* SS = 1;
*/
public static final int SS_VALUE = 1;
/**
* ES = 2;
*/
public static final int ES_VALUE = 2;
/**
* EE = 3;
*/
public static final int EE_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BreakendOrientation valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static BreakendOrientation forNumber(int value) {
switch (value) {
case 0: return SE;
case 1: return SS;
case 2: return ES;
case 3: return EE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BreakendOrientation> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BreakendOrientation findValueByNumber(int number) {
return BreakendOrientation.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.getDescriptor().getEnumTypes().get(1);
}
private static final BreakendOrientation[] VALUES = values();
public static BreakendOrientation valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private BreakendOrientation(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:protobuf.opencb.BreakendOrientation)
}
public interface VariantStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.VariantStats)
com.google.protobuf.MessageOrBuilder {
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return The cohortId.
*/
java.lang.String getCohortId();
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return The bytes for cohortId.
*/
com.google.protobuf.ByteString
getCohortIdBytes();
/**
*
**
* Count of samples with non-missing genotypes in this variant from the cohort.
* This value is used as denominator for genotypeFreq.
*
*
* int32 sampleCount = 18;
* @return The sampleCount.
*/
int getSampleCount();
/**
*
**
* Count of files with samples from the cohort that reported this variant.
* This value is used as denominator for filterFreq.
*
*
* int32 fileCount = 19;
* @return The fileCount.
*/
int getFileCount();
/**
*
**
* Total number of alleles in called genotypes. It does not include missing alleles.
* This value is used as denominator for refAlleleFreq and altAlleleFreq.
*
*
* int32 alleleCount = 1;
* @return The alleleCount.
*/
int getAlleleCount();
/**
*
**
* Number of reference alleles found in this variant.
*
*
* int32 refAlleleCount = 2;
* @return The refAlleleCount.
*/
int getRefAlleleCount();
/**
*
**
* Number of main alternate alleles found in this variants. It does not include secondary alternates.
*
*
* int32 altAlleleCount = 3;
* @return The altAlleleCount.
*/
int getAltAlleleCount();
/**
*
**
* Reference allele frequency calculated from refAlleleCount and alleleCount, in the range [0,1]
*
*
* float refAlleleFreq = 4;
* @return The refAlleleFreq.
*/
float getRefAlleleFreq();
/**
*
**
* Alternate allele frequency calculated from altAlleleCount and alleleCount, in the range [0,1]
*
*
* float altAlleleFreq = 5;
* @return The altAlleleFreq.
*/
float getAltAlleleFreq();
/**
*
**
* Number of missing alleles
*
*
* int32 missingAlleleCount = 8;
* @return The missingAlleleCount.
*/
int getMissingAlleleCount();
/**
*
**
* Number of genotypes with all alleles missing (e.g. ./.). It does not count partially missing genotypes like "./0" or "./1".
*
*
* int32 missingGenotypeCount = 9;
* @return The missingGenotypeCount.
*/
int getMissingGenotypeCount();
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
int getGenotypeCountCount();
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
boolean containsGenotypeCount(
java.lang.String key);
/**
* Use {@link #getGenotypeCountMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getGenotypeCount();
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
java.util.Map
getGenotypeCountMap();
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
int getGenotypeCountOrDefault(
java.lang.String key,
int defaultValue);
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
int getGenotypeCountOrThrow(
java.lang.String key);
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
int getGenotypeFreqCount();
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
boolean containsGenotypeFreq(
java.lang.String key);
/**
* Use {@link #getGenotypeFreqMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getGenotypeFreq();
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
java.util.Map
getGenotypeFreqMap();
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
float getGenotypeFreqOrDefault(
java.lang.String key,
float defaultValue);
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
float getGenotypeFreqOrThrow(
java.lang.String key);
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
int getFilterCountCount();
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
boolean containsFilterCount(
java.lang.String key);
/**
* Use {@link #getFilterCountMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getFilterCount();
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
java.util.Map
getFilterCountMap();
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
int getFilterCountOrDefault(
java.lang.String key,
int defaultValue);
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
int getFilterCountOrThrow(
java.lang.String key);
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
int getFilterFreqCount();
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
boolean containsFilterFreq(
java.lang.String key);
/**
* Use {@link #getFilterFreqMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getFilterFreq();
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
java.util.Map
getFilterFreqMap();
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
float getFilterFreqOrDefault(
java.lang.String key,
float defaultValue);
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
float getFilterFreqOrThrow(
java.lang.String key);
/**
*
**
* The number of files from samples in this cohort reporting this variant with valid QUAL values.
* This value is used as denominator to obtain the qualityAvg.
*
*
* int32 qualityCount = 20;
* @return The qualityCount.
*/
int getQualityCount();
/**
*
**
* The average Quality value for files with valid QUAL values from samples in this cohort reporting this variant.
* Some files may not have defined the QUAL value, so the sampling could be less than the filesCount.
*
*
* float qualityAvg = 16;
* @return The qualityAvg.
*/
float getQualityAvg();
/**
*
**
* Minor allele frequency. Frequency of the less common allele between the reference and the main alternate alleles.
* This value does not take into acconunt secondary alternates.
*
*
* float maf = 10;
* @return The maf.
*/
float getMaf();
/**
*
**
* Minor genotype frequency. Frequency of the less common genotype seen in this variant.
* This value takes into account all values from the genotypeFreq map.
*
*
* float mgf = 11;
* @return The mgf.
*/
float getMgf();
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return The mafAllele.
*/
java.lang.String getMafAllele();
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return The bytes for mafAllele.
*/
com.google.protobuf.ByteString
getMafAlleleBytes();
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return The mgfGenotype.
*/
java.lang.String getMgfGenotype();
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return The bytes for mgfGenotype.
*/
com.google.protobuf.ByteString
getMgfGenotypeBytes();
}
/**
* Protobuf type {@code protobuf.opencb.VariantStats}
*/
public static final class VariantStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.VariantStats)
VariantStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use VariantStats.newBuilder() to construct.
private VariantStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VariantStats() {
cohortId_ = "";
mafAllele_ = "";
mgfGenotype_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new VariantStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VariantStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
alleleCount_ = input.readInt32();
break;
}
case 16: {
refAlleleCount_ = input.readInt32();
break;
}
case 24: {
altAlleleCount_ = input.readInt32();
break;
}
case 37: {
refAlleleFreq_ = input.readFloat();
break;
}
case 45: {
altAlleleFreq_ = input.readFloat();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
genotypeCount_ = com.google.protobuf.MapField.newMapField(
GenotypeCountDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
genotypeCount__ = input.readMessage(
GenotypeCountDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
genotypeCount_.getMutableMap().put(
genotypeCount__.getKey(), genotypeCount__.getValue());
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
genotypeFreq_ = com.google.protobuf.MapField.newMapField(
GenotypeFreqDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
genotypeFreq__ = input.readMessage(
GenotypeFreqDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
genotypeFreq_.getMutableMap().put(
genotypeFreq__.getKey(), genotypeFreq__.getValue());
break;
}
case 64: {
missingAlleleCount_ = input.readInt32();
break;
}
case 72: {
missingGenotypeCount_ = input.readInt32();
break;
}
case 85: {
maf_ = input.readFloat();
break;
}
case 93: {
mgf_ = input.readFloat();
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
mafAllele_ = s;
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
mgfGenotype_ = s;
break;
}
case 114: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
filterCount_ = com.google.protobuf.MapField.newMapField(
FilterCountDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000004;
}
com.google.protobuf.MapEntry
filterCount__ = input.readMessage(
FilterCountDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
filterCount_.getMutableMap().put(
filterCount__.getKey(), filterCount__.getValue());
break;
}
case 122: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
filterFreq_ = com.google.protobuf.MapField.newMapField(
FilterFreqDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000008;
}
com.google.protobuf.MapEntry
filterFreq__ = input.readMessage(
FilterFreqDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
filterFreq_.getMutableMap().put(
filterFreq__.getKey(), filterFreq__.getValue());
break;
}
case 133: {
qualityAvg_ = input.readFloat();
break;
}
case 138: {
java.lang.String s = input.readStringRequireUtf8();
cohortId_ = s;
break;
}
case 144: {
sampleCount_ = input.readInt32();
break;
}
case 152: {
fileCount_ = input.readInt32();
break;
}
case 160: {
qualityCount_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetGenotypeCount();
case 7:
return internalGetGenotypeFreq();
case 14:
return internalGetFilterCount();
case 15:
return internalGetFilterFreq();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.class, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder.class);
}
public static final int COHORTID_FIELD_NUMBER = 17;
private volatile java.lang.Object cohortId_;
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return The cohortId.
*/
public java.lang.String getCohortId() {
java.lang.Object ref = cohortId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cohortId_ = s;
return s;
}
}
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return The bytes for cohortId.
*/
public com.google.protobuf.ByteString
getCohortIdBytes() {
java.lang.Object ref = cohortId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cohortId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SAMPLECOUNT_FIELD_NUMBER = 18;
private int sampleCount_;
/**
*
**
* Count of samples with non-missing genotypes in this variant from the cohort.
* This value is used as denominator for genotypeFreq.
*
*
* int32 sampleCount = 18;
* @return The sampleCount.
*/
public int getSampleCount() {
return sampleCount_;
}
public static final int FILECOUNT_FIELD_NUMBER = 19;
private int fileCount_;
/**
*
**
* Count of files with samples from the cohort that reported this variant.
* This value is used as denominator for filterFreq.
*
*
* int32 fileCount = 19;
* @return The fileCount.
*/
public int getFileCount() {
return fileCount_;
}
public static final int ALLELECOUNT_FIELD_NUMBER = 1;
private int alleleCount_;
/**
*
**
* Total number of alleles in called genotypes. It does not include missing alleles.
* This value is used as denominator for refAlleleFreq and altAlleleFreq.
*
*
* int32 alleleCount = 1;
* @return The alleleCount.
*/
public int getAlleleCount() {
return alleleCount_;
}
public static final int REFALLELECOUNT_FIELD_NUMBER = 2;
private int refAlleleCount_;
/**
*
**
* Number of reference alleles found in this variant.
*
*
* int32 refAlleleCount = 2;
* @return The refAlleleCount.
*/
public int getRefAlleleCount() {
return refAlleleCount_;
}
public static final int ALTALLELECOUNT_FIELD_NUMBER = 3;
private int altAlleleCount_;
/**
*
**
* Number of main alternate alleles found in this variants. It does not include secondary alternates.
*
*
* int32 altAlleleCount = 3;
* @return The altAlleleCount.
*/
public int getAltAlleleCount() {
return altAlleleCount_;
}
public static final int REFALLELEFREQ_FIELD_NUMBER = 4;
private float refAlleleFreq_;
/**
*
**
* Reference allele frequency calculated from refAlleleCount and alleleCount, in the range [0,1]
*
*
* float refAlleleFreq = 4;
* @return The refAlleleFreq.
*/
public float getRefAlleleFreq() {
return refAlleleFreq_;
}
public static final int ALTALLELEFREQ_FIELD_NUMBER = 5;
private float altAlleleFreq_;
/**
*
**
* Alternate allele frequency calculated from altAlleleCount and alleleCount, in the range [0,1]
*
*
* float altAlleleFreq = 5;
* @return The altAlleleFreq.
*/
public float getAltAlleleFreq() {
return altAlleleFreq_;
}
public static final int MISSINGALLELECOUNT_FIELD_NUMBER = 8;
private int missingAlleleCount_;
/**
*
**
* Number of missing alleles
*
*
* int32 missingAlleleCount = 8;
* @return The missingAlleleCount.
*/
public int getMissingAlleleCount() {
return missingAlleleCount_;
}
public static final int MISSINGGENOTYPECOUNT_FIELD_NUMBER = 9;
private int missingGenotypeCount_;
/**
*
**
* Number of genotypes with all alleles missing (e.g. ./.). It does not count partially missing genotypes like "./0" or "./1".
*
*
* int32 missingGenotypeCount = 9;
* @return The missingGenotypeCount.
*/
public int getMissingGenotypeCount() {
return missingGenotypeCount_;
}
public static final int GENOTYPECOUNT_FIELD_NUMBER = 6;
private static final class GenotypeCountDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.Integer> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_GenotypeCountEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.INT32,
0);
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Integer> genotypeCount_;
private com.google.protobuf.MapField
internalGetGenotypeCount() {
if (genotypeCount_ == null) {
return com.google.protobuf.MapField.emptyMapField(
GenotypeCountDefaultEntryHolder.defaultEntry);
}
return genotypeCount_;
}
public int getGenotypeCountCount() {
return internalGetGenotypeCount().getMap().size();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public boolean containsGenotypeCount(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetGenotypeCount().getMap().containsKey(key);
}
/**
* Use {@link #getGenotypeCountMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getGenotypeCount() {
return getGenotypeCountMap();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public java.util.Map getGenotypeCountMap() {
return internalGetGenotypeCount().getMap();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public int getGenotypeCountOrDefault(
java.lang.String key,
int defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeCount().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public int getGenotypeCountOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeCount().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int GENOTYPEFREQ_FIELD_NUMBER = 7;
private static final class GenotypeFreqDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.Float> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_GenotypeFreqEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.FLOAT,
0F);
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Float> genotypeFreq_;
private com.google.protobuf.MapField
internalGetGenotypeFreq() {
if (genotypeFreq_ == null) {
return com.google.protobuf.MapField.emptyMapField(
GenotypeFreqDefaultEntryHolder.defaultEntry);
}
return genotypeFreq_;
}
public int getGenotypeFreqCount() {
return internalGetGenotypeFreq().getMap().size();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public boolean containsGenotypeFreq(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetGenotypeFreq().getMap().containsKey(key);
}
/**
* Use {@link #getGenotypeFreqMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getGenotypeFreq() {
return getGenotypeFreqMap();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public java.util.Map getGenotypeFreqMap() {
return internalGetGenotypeFreq().getMap();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public float getGenotypeFreqOrDefault(
java.lang.String key,
float defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeFreq().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public float getGenotypeFreqOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeFreq().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int FILTERCOUNT_FIELD_NUMBER = 14;
private static final class FilterCountDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.Integer> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_FilterCountEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.INT32,
0);
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Integer> filterCount_;
private com.google.protobuf.MapField
internalGetFilterCount() {
if (filterCount_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FilterCountDefaultEntryHolder.defaultEntry);
}
return filterCount_;
}
public int getFilterCountCount() {
return internalGetFilterCount().getMap().size();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public boolean containsFilterCount(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFilterCount().getMap().containsKey(key);
}
/**
* Use {@link #getFilterCountMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFilterCount() {
return getFilterCountMap();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public java.util.Map getFilterCountMap() {
return internalGetFilterCount().getMap();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public int getFilterCountOrDefault(
java.lang.String key,
int defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterCount().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public int getFilterCountOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterCount().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int FILTERFREQ_FIELD_NUMBER = 15;
private static final class FilterFreqDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.Float> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_FilterFreqEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.FLOAT,
0F);
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Float> filterFreq_;
private com.google.protobuf.MapField
internalGetFilterFreq() {
if (filterFreq_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FilterFreqDefaultEntryHolder.defaultEntry);
}
return filterFreq_;
}
public int getFilterFreqCount() {
return internalGetFilterFreq().getMap().size();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public boolean containsFilterFreq(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFilterFreq().getMap().containsKey(key);
}
/**
* Use {@link #getFilterFreqMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFilterFreq() {
return getFilterFreqMap();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public java.util.Map getFilterFreqMap() {
return internalGetFilterFreq().getMap();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public float getFilterFreqOrDefault(
java.lang.String key,
float defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterFreq().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public float getFilterFreqOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterFreq().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int QUALITYCOUNT_FIELD_NUMBER = 20;
private int qualityCount_;
/**
*
**
* The number of files from samples in this cohort reporting this variant with valid QUAL values.
* This value is used as denominator to obtain the qualityAvg.
*
*
* int32 qualityCount = 20;
* @return The qualityCount.
*/
public int getQualityCount() {
return qualityCount_;
}
public static final int QUALITYAVG_FIELD_NUMBER = 16;
private float qualityAvg_;
/**
*
**
* The average Quality value for files with valid QUAL values from samples in this cohort reporting this variant.
* Some files may not have defined the QUAL value, so the sampling could be less than the filesCount.
*
*
* float qualityAvg = 16;
* @return The qualityAvg.
*/
public float getQualityAvg() {
return qualityAvg_;
}
public static final int MAF_FIELD_NUMBER = 10;
private float maf_;
/**
*
**
* Minor allele frequency. Frequency of the less common allele between the reference and the main alternate alleles.
* This value does not take into acconunt secondary alternates.
*
*
* float maf = 10;
* @return The maf.
*/
public float getMaf() {
return maf_;
}
public static final int MGF_FIELD_NUMBER = 11;
private float mgf_;
/**
*
**
* Minor genotype frequency. Frequency of the less common genotype seen in this variant.
* This value takes into account all values from the genotypeFreq map.
*
*
* float mgf = 11;
* @return The mgf.
*/
public float getMgf() {
return mgf_;
}
public static final int MAFALLELE_FIELD_NUMBER = 12;
private volatile java.lang.Object mafAllele_;
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return The mafAllele.
*/
public java.lang.String getMafAllele() {
java.lang.Object ref = mafAllele_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mafAllele_ = s;
return s;
}
}
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return The bytes for mafAllele.
*/
public com.google.protobuf.ByteString
getMafAlleleBytes() {
java.lang.Object ref = mafAllele_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mafAllele_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MGFGENOTYPE_FIELD_NUMBER = 13;
private volatile java.lang.Object mgfGenotype_;
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return The mgfGenotype.
*/
public java.lang.String getMgfGenotype() {
java.lang.Object ref = mgfGenotype_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mgfGenotype_ = s;
return s;
}
}
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return The bytes for mgfGenotype.
*/
public com.google.protobuf.ByteString
getMgfGenotypeBytes() {
java.lang.Object ref = mgfGenotype_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mgfGenotype_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (alleleCount_ != 0) {
output.writeInt32(1, alleleCount_);
}
if (refAlleleCount_ != 0) {
output.writeInt32(2, refAlleleCount_);
}
if (altAlleleCount_ != 0) {
output.writeInt32(3, altAlleleCount_);
}
if (refAlleleFreq_ != 0F) {
output.writeFloat(4, refAlleleFreq_);
}
if (altAlleleFreq_ != 0F) {
output.writeFloat(5, altAlleleFreq_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetGenotypeCount(),
GenotypeCountDefaultEntryHolder.defaultEntry,
6);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetGenotypeFreq(),
GenotypeFreqDefaultEntryHolder.defaultEntry,
7);
if (missingAlleleCount_ != 0) {
output.writeInt32(8, missingAlleleCount_);
}
if (missingGenotypeCount_ != 0) {
output.writeInt32(9, missingGenotypeCount_);
}
if (maf_ != 0F) {
output.writeFloat(10, maf_);
}
if (mgf_ != 0F) {
output.writeFloat(11, mgf_);
}
if (!getMafAlleleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, mafAllele_);
}
if (!getMgfGenotypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, mgfGenotype_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetFilterCount(),
FilterCountDefaultEntryHolder.defaultEntry,
14);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetFilterFreq(),
FilterFreqDefaultEntryHolder.defaultEntry,
15);
if (qualityAvg_ != 0F) {
output.writeFloat(16, qualityAvg_);
}
if (!getCohortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, cohortId_);
}
if (sampleCount_ != 0) {
output.writeInt32(18, sampleCount_);
}
if (fileCount_ != 0) {
output.writeInt32(19, fileCount_);
}
if (qualityCount_ != 0) {
output.writeInt32(20, qualityCount_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (alleleCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, alleleCount_);
}
if (refAlleleCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, refAlleleCount_);
}
if (altAlleleCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, altAlleleCount_);
}
if (refAlleleFreq_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(4, refAlleleFreq_);
}
if (altAlleleFreq_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(5, altAlleleFreq_);
}
for (java.util.Map.Entry entry
: internalGetGenotypeCount().getMap().entrySet()) {
com.google.protobuf.MapEntry
genotypeCount__ = GenotypeCountDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, genotypeCount__);
}
for (java.util.Map.Entry entry
: internalGetGenotypeFreq().getMap().entrySet()) {
com.google.protobuf.MapEntry
genotypeFreq__ = GenotypeFreqDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, genotypeFreq__);
}
if (missingAlleleCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, missingAlleleCount_);
}
if (missingGenotypeCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, missingGenotypeCount_);
}
if (maf_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(10, maf_);
}
if (mgf_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(11, mgf_);
}
if (!getMafAlleleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, mafAllele_);
}
if (!getMgfGenotypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, mgfGenotype_);
}
for (java.util.Map.Entry entry
: internalGetFilterCount().getMap().entrySet()) {
com.google.protobuf.MapEntry
filterCount__ = FilterCountDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, filterCount__);
}
for (java.util.Map.Entry entry
: internalGetFilterFreq().getMap().entrySet()) {
com.google.protobuf.MapEntry
filterFreq__ = FilterFreqDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, filterFreq__);
}
if (qualityAvg_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(16, qualityAvg_);
}
if (!getCohortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, cohortId_);
}
if (sampleCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(18, sampleCount_);
}
if (fileCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(19, fileCount_);
}
if (qualityCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(20, qualityCount_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats other = (org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats) obj;
if (!getCohortId()
.equals(other.getCohortId())) return false;
if (getSampleCount()
!= other.getSampleCount()) return false;
if (getFileCount()
!= other.getFileCount()) return false;
if (getAlleleCount()
!= other.getAlleleCount()) return false;
if (getRefAlleleCount()
!= other.getRefAlleleCount()) return false;
if (getAltAlleleCount()
!= other.getAltAlleleCount()) return false;
if (java.lang.Float.floatToIntBits(getRefAlleleFreq())
!= java.lang.Float.floatToIntBits(
other.getRefAlleleFreq())) return false;
if (java.lang.Float.floatToIntBits(getAltAlleleFreq())
!= java.lang.Float.floatToIntBits(
other.getAltAlleleFreq())) return false;
if (getMissingAlleleCount()
!= other.getMissingAlleleCount()) return false;
if (getMissingGenotypeCount()
!= other.getMissingGenotypeCount()) return false;
if (!internalGetGenotypeCount().equals(
other.internalGetGenotypeCount())) return false;
if (!internalGetGenotypeFreq().equals(
other.internalGetGenotypeFreq())) return false;
if (!internalGetFilterCount().equals(
other.internalGetFilterCount())) return false;
if (!internalGetFilterFreq().equals(
other.internalGetFilterFreq())) return false;
if (getQualityCount()
!= other.getQualityCount()) return false;
if (java.lang.Float.floatToIntBits(getQualityAvg())
!= java.lang.Float.floatToIntBits(
other.getQualityAvg())) return false;
if (java.lang.Float.floatToIntBits(getMaf())
!= java.lang.Float.floatToIntBits(
other.getMaf())) return false;
if (java.lang.Float.floatToIntBits(getMgf())
!= java.lang.Float.floatToIntBits(
other.getMgf())) return false;
if (!getMafAllele()
.equals(other.getMafAllele())) return false;
if (!getMgfGenotype()
.equals(other.getMgfGenotype())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COHORTID_FIELD_NUMBER;
hash = (53 * hash) + getCohortId().hashCode();
hash = (37 * hash) + SAMPLECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getSampleCount();
hash = (37 * hash) + FILECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getFileCount();
hash = (37 * hash) + ALLELECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAlleleCount();
hash = (37 * hash) + REFALLELECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getRefAlleleCount();
hash = (37 * hash) + ALTALLELECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAltAlleleCount();
hash = (37 * hash) + REFALLELEFREQ_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getRefAlleleFreq());
hash = (37 * hash) + ALTALLELEFREQ_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getAltAlleleFreq());
hash = (37 * hash) + MISSINGALLELECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getMissingAlleleCount();
hash = (37 * hash) + MISSINGGENOTYPECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getMissingGenotypeCount();
if (!internalGetGenotypeCount().getMap().isEmpty()) {
hash = (37 * hash) + GENOTYPECOUNT_FIELD_NUMBER;
hash = (53 * hash) + internalGetGenotypeCount().hashCode();
}
if (!internalGetGenotypeFreq().getMap().isEmpty()) {
hash = (37 * hash) + GENOTYPEFREQ_FIELD_NUMBER;
hash = (53 * hash) + internalGetGenotypeFreq().hashCode();
}
if (!internalGetFilterCount().getMap().isEmpty()) {
hash = (37 * hash) + FILTERCOUNT_FIELD_NUMBER;
hash = (53 * hash) + internalGetFilterCount().hashCode();
}
if (!internalGetFilterFreq().getMap().isEmpty()) {
hash = (37 * hash) + FILTERFREQ_FIELD_NUMBER;
hash = (53 * hash) + internalGetFilterFreq().hashCode();
}
hash = (37 * hash) + QUALITYCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getQualityCount();
hash = (37 * hash) + QUALITYAVG_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getQualityAvg());
hash = (37 * hash) + MAF_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getMaf());
hash = (37 * hash) + MGF_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getMgf());
hash = (37 * hash) + MAFALLELE_FIELD_NUMBER;
hash = (53 * hash) + getMafAllele().hashCode();
hash = (37 * hash) + MGFGENOTYPE_FIELD_NUMBER;
hash = (53 * hash) + getMgfGenotype().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.VariantStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.VariantStats)
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetGenotypeCount();
case 7:
return internalGetGenotypeFreq();
case 14:
return internalGetFilterCount();
case 15:
return internalGetFilterFreq();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 6:
return internalGetMutableGenotypeCount();
case 7:
return internalGetMutableGenotypeFreq();
case 14:
return internalGetMutableFilterCount();
case 15:
return internalGetMutableFilterFreq();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.class, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
cohortId_ = "";
sampleCount_ = 0;
fileCount_ = 0;
alleleCount_ = 0;
refAlleleCount_ = 0;
altAlleleCount_ = 0;
refAlleleFreq_ = 0F;
altAlleleFreq_ = 0F;
missingAlleleCount_ = 0;
missingGenotypeCount_ = 0;
internalGetMutableGenotypeCount().clear();
internalGetMutableGenotypeFreq().clear();
internalGetMutableFilterCount().clear();
internalGetMutableFilterFreq().clear();
qualityCount_ = 0;
qualityAvg_ = 0F;
maf_ = 0F;
mgf_ = 0F;
mafAllele_ = "";
mgfGenotype_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantStats_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats result = new org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats(this);
int from_bitField0_ = bitField0_;
result.cohortId_ = cohortId_;
result.sampleCount_ = sampleCount_;
result.fileCount_ = fileCount_;
result.alleleCount_ = alleleCount_;
result.refAlleleCount_ = refAlleleCount_;
result.altAlleleCount_ = altAlleleCount_;
result.refAlleleFreq_ = refAlleleFreq_;
result.altAlleleFreq_ = altAlleleFreq_;
result.missingAlleleCount_ = missingAlleleCount_;
result.missingGenotypeCount_ = missingGenotypeCount_;
result.genotypeCount_ = internalGetGenotypeCount();
result.genotypeCount_.makeImmutable();
result.genotypeFreq_ = internalGetGenotypeFreq();
result.genotypeFreq_.makeImmutable();
result.filterCount_ = internalGetFilterCount();
result.filterCount_.makeImmutable();
result.filterFreq_ = internalGetFilterFreq();
result.filterFreq_.makeImmutable();
result.qualityCount_ = qualityCount_;
result.qualityAvg_ = qualityAvg_;
result.maf_ = maf_;
result.mgf_ = mgf_;
result.mafAllele_ = mafAllele_;
result.mgfGenotype_ = mgfGenotype_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.getDefaultInstance()) return this;
if (!other.getCohortId().isEmpty()) {
cohortId_ = other.cohortId_;
onChanged();
}
if (other.getSampleCount() != 0) {
setSampleCount(other.getSampleCount());
}
if (other.getFileCount() != 0) {
setFileCount(other.getFileCount());
}
if (other.getAlleleCount() != 0) {
setAlleleCount(other.getAlleleCount());
}
if (other.getRefAlleleCount() != 0) {
setRefAlleleCount(other.getRefAlleleCount());
}
if (other.getAltAlleleCount() != 0) {
setAltAlleleCount(other.getAltAlleleCount());
}
if (other.getRefAlleleFreq() != 0F) {
setRefAlleleFreq(other.getRefAlleleFreq());
}
if (other.getAltAlleleFreq() != 0F) {
setAltAlleleFreq(other.getAltAlleleFreq());
}
if (other.getMissingAlleleCount() != 0) {
setMissingAlleleCount(other.getMissingAlleleCount());
}
if (other.getMissingGenotypeCount() != 0) {
setMissingGenotypeCount(other.getMissingGenotypeCount());
}
internalGetMutableGenotypeCount().mergeFrom(
other.internalGetGenotypeCount());
internalGetMutableGenotypeFreq().mergeFrom(
other.internalGetGenotypeFreq());
internalGetMutableFilterCount().mergeFrom(
other.internalGetFilterCount());
internalGetMutableFilterFreq().mergeFrom(
other.internalGetFilterFreq());
if (other.getQualityCount() != 0) {
setQualityCount(other.getQualityCount());
}
if (other.getQualityAvg() != 0F) {
setQualityAvg(other.getQualityAvg());
}
if (other.getMaf() != 0F) {
setMaf(other.getMaf());
}
if (other.getMgf() != 0F) {
setMgf(other.getMgf());
}
if (!other.getMafAllele().isEmpty()) {
mafAllele_ = other.mafAllele_;
onChanged();
}
if (!other.getMgfGenotype().isEmpty()) {
mgfGenotype_ = other.mgfGenotype_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cohortId_ = "";
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return The cohortId.
*/
public java.lang.String getCohortId() {
java.lang.Object ref = cohortId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cohortId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return The bytes for cohortId.
*/
public com.google.protobuf.ByteString
getCohortIdBytes() {
java.lang.Object ref = cohortId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cohortId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @param value The cohortId to set.
* @return This builder for chaining.
*/
public Builder setCohortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cohortId_ = value;
onChanged();
return this;
}
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @return This builder for chaining.
*/
public Builder clearCohortId() {
cohortId_ = getDefaultInstance().getCohortId();
onChanged();
return this;
}
/**
*
**
* Unique cohort identifier within the study.
*
*
* string cohortId = 17;
* @param value The bytes for cohortId to set.
* @return This builder for chaining.
*/
public Builder setCohortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cohortId_ = value;
onChanged();
return this;
}
private int sampleCount_ ;
/**
*
**
* Count of samples with non-missing genotypes in this variant from the cohort.
* This value is used as denominator for genotypeFreq.
*
*
* int32 sampleCount = 18;
* @return The sampleCount.
*/
public int getSampleCount() {
return sampleCount_;
}
/**
*
**
* Count of samples with non-missing genotypes in this variant from the cohort.
* This value is used as denominator for genotypeFreq.
*
*
* int32 sampleCount = 18;
* @param value The sampleCount to set.
* @return This builder for chaining.
*/
public Builder setSampleCount(int value) {
sampleCount_ = value;
onChanged();
return this;
}
/**
*
**
* Count of samples with non-missing genotypes in this variant from the cohort.
* This value is used as denominator for genotypeFreq.
*
*
* int32 sampleCount = 18;
* @return This builder for chaining.
*/
public Builder clearSampleCount() {
sampleCount_ = 0;
onChanged();
return this;
}
private int fileCount_ ;
/**
*
**
* Count of files with samples from the cohort that reported this variant.
* This value is used as denominator for filterFreq.
*
*
* int32 fileCount = 19;
* @return The fileCount.
*/
public int getFileCount() {
return fileCount_;
}
/**
*
**
* Count of files with samples from the cohort that reported this variant.
* This value is used as denominator for filterFreq.
*
*
* int32 fileCount = 19;
* @param value The fileCount to set.
* @return This builder for chaining.
*/
public Builder setFileCount(int value) {
fileCount_ = value;
onChanged();
return this;
}
/**
*
**
* Count of files with samples from the cohort that reported this variant.
* This value is used as denominator for filterFreq.
*
*
* int32 fileCount = 19;
* @return This builder for chaining.
*/
public Builder clearFileCount() {
fileCount_ = 0;
onChanged();
return this;
}
private int alleleCount_ ;
/**
*
**
* Total number of alleles in called genotypes. It does not include missing alleles.
* This value is used as denominator for refAlleleFreq and altAlleleFreq.
*
*
* int32 alleleCount = 1;
* @return The alleleCount.
*/
public int getAlleleCount() {
return alleleCount_;
}
/**
*
**
* Total number of alleles in called genotypes. It does not include missing alleles.
* This value is used as denominator for refAlleleFreq and altAlleleFreq.
*
*
* int32 alleleCount = 1;
* @param value The alleleCount to set.
* @return This builder for chaining.
*/
public Builder setAlleleCount(int value) {
alleleCount_ = value;
onChanged();
return this;
}
/**
*
**
* Total number of alleles in called genotypes. It does not include missing alleles.
* This value is used as denominator for refAlleleFreq and altAlleleFreq.
*
*
* int32 alleleCount = 1;
* @return This builder for chaining.
*/
public Builder clearAlleleCount() {
alleleCount_ = 0;
onChanged();
return this;
}
private int refAlleleCount_ ;
/**
*
**
* Number of reference alleles found in this variant.
*
*
* int32 refAlleleCount = 2;
* @return The refAlleleCount.
*/
public int getRefAlleleCount() {
return refAlleleCount_;
}
/**
*
**
* Number of reference alleles found in this variant.
*
*
* int32 refAlleleCount = 2;
* @param value The refAlleleCount to set.
* @return This builder for chaining.
*/
public Builder setRefAlleleCount(int value) {
refAlleleCount_ = value;
onChanged();
return this;
}
/**
*
**
* Number of reference alleles found in this variant.
*
*
* int32 refAlleleCount = 2;
* @return This builder for chaining.
*/
public Builder clearRefAlleleCount() {
refAlleleCount_ = 0;
onChanged();
return this;
}
private int altAlleleCount_ ;
/**
*
**
* Number of main alternate alleles found in this variants. It does not include secondary alternates.
*
*
* int32 altAlleleCount = 3;
* @return The altAlleleCount.
*/
public int getAltAlleleCount() {
return altAlleleCount_;
}
/**
*
**
* Number of main alternate alleles found in this variants. It does not include secondary alternates.
*
*
* int32 altAlleleCount = 3;
* @param value The altAlleleCount to set.
* @return This builder for chaining.
*/
public Builder setAltAlleleCount(int value) {
altAlleleCount_ = value;
onChanged();
return this;
}
/**
*
**
* Number of main alternate alleles found in this variants. It does not include secondary alternates.
*
*
* int32 altAlleleCount = 3;
* @return This builder for chaining.
*/
public Builder clearAltAlleleCount() {
altAlleleCount_ = 0;
onChanged();
return this;
}
private float refAlleleFreq_ ;
/**
*
**
* Reference allele frequency calculated from refAlleleCount and alleleCount, in the range [0,1]
*
*
* float refAlleleFreq = 4;
* @return The refAlleleFreq.
*/
public float getRefAlleleFreq() {
return refAlleleFreq_;
}
/**
*
**
* Reference allele frequency calculated from refAlleleCount and alleleCount, in the range [0,1]
*
*
* float refAlleleFreq = 4;
* @param value The refAlleleFreq to set.
* @return This builder for chaining.
*/
public Builder setRefAlleleFreq(float value) {
refAlleleFreq_ = value;
onChanged();
return this;
}
/**
*
**
* Reference allele frequency calculated from refAlleleCount and alleleCount, in the range [0,1]
*
*
* float refAlleleFreq = 4;
* @return This builder for chaining.
*/
public Builder clearRefAlleleFreq() {
refAlleleFreq_ = 0F;
onChanged();
return this;
}
private float altAlleleFreq_ ;
/**
*
**
* Alternate allele frequency calculated from altAlleleCount and alleleCount, in the range [0,1]
*
*
* float altAlleleFreq = 5;
* @return The altAlleleFreq.
*/
public float getAltAlleleFreq() {
return altAlleleFreq_;
}
/**
*
**
* Alternate allele frequency calculated from altAlleleCount and alleleCount, in the range [0,1]
*
*
* float altAlleleFreq = 5;
* @param value The altAlleleFreq to set.
* @return This builder for chaining.
*/
public Builder setAltAlleleFreq(float value) {
altAlleleFreq_ = value;
onChanged();
return this;
}
/**
*
**
* Alternate allele frequency calculated from altAlleleCount and alleleCount, in the range [0,1]
*
*
* float altAlleleFreq = 5;
* @return This builder for chaining.
*/
public Builder clearAltAlleleFreq() {
altAlleleFreq_ = 0F;
onChanged();
return this;
}
private int missingAlleleCount_ ;
/**
*
**
* Number of missing alleles
*
*
* int32 missingAlleleCount = 8;
* @return The missingAlleleCount.
*/
public int getMissingAlleleCount() {
return missingAlleleCount_;
}
/**
*
**
* Number of missing alleles
*
*
* int32 missingAlleleCount = 8;
* @param value The missingAlleleCount to set.
* @return This builder for chaining.
*/
public Builder setMissingAlleleCount(int value) {
missingAlleleCount_ = value;
onChanged();
return this;
}
/**
*
**
* Number of missing alleles
*
*
* int32 missingAlleleCount = 8;
* @return This builder for chaining.
*/
public Builder clearMissingAlleleCount() {
missingAlleleCount_ = 0;
onChanged();
return this;
}
private int missingGenotypeCount_ ;
/**
*
**
* Number of genotypes with all alleles missing (e.g. ./.). It does not count partially missing genotypes like "./0" or "./1".
*
*
* int32 missingGenotypeCount = 9;
* @return The missingGenotypeCount.
*/
public int getMissingGenotypeCount() {
return missingGenotypeCount_;
}
/**
*
**
* Number of genotypes with all alleles missing (e.g. ./.). It does not count partially missing genotypes like "./0" or "./1".
*
*
* int32 missingGenotypeCount = 9;
* @param value The missingGenotypeCount to set.
* @return This builder for chaining.
*/
public Builder setMissingGenotypeCount(int value) {
missingGenotypeCount_ = value;
onChanged();
return this;
}
/**
*
**
* Number of genotypes with all alleles missing (e.g. ./.). It does not count partially missing genotypes like "./0" or "./1".
*
*
* int32 missingGenotypeCount = 9;
* @return This builder for chaining.
*/
public Builder clearMissingGenotypeCount() {
missingGenotypeCount_ = 0;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Integer> genotypeCount_;
private com.google.protobuf.MapField
internalGetGenotypeCount() {
if (genotypeCount_ == null) {
return com.google.protobuf.MapField.emptyMapField(
GenotypeCountDefaultEntryHolder.defaultEntry);
}
return genotypeCount_;
}
private com.google.protobuf.MapField
internalGetMutableGenotypeCount() {
onChanged();;
if (genotypeCount_ == null) {
genotypeCount_ = com.google.protobuf.MapField.newMapField(
GenotypeCountDefaultEntryHolder.defaultEntry);
}
if (!genotypeCount_.isMutable()) {
genotypeCount_ = genotypeCount_.copy();
}
return genotypeCount_;
}
public int getGenotypeCountCount() {
return internalGetGenotypeCount().getMap().size();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public boolean containsGenotypeCount(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetGenotypeCount().getMap().containsKey(key);
}
/**
* Use {@link #getGenotypeCountMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getGenotypeCount() {
return getGenotypeCountMap();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public java.util.Map getGenotypeCountMap() {
return internalGetGenotypeCount().getMap();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public int getGenotypeCountOrDefault(
java.lang.String key,
int defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeCount().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public int getGenotypeCountOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeCount().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearGenotypeCount() {
internalGetMutableGenotypeCount().getMutableMap()
.clear();
return this;
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public Builder removeGenotypeCount(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableGenotypeCount().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableGenotypeCount() {
return internalGetMutableGenotypeCount().getMutableMap();
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public Builder putGenotypeCount(
java.lang.String key,
int value) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableGenotypeCount().getMutableMap()
.put(key, value);
return this;
}
/**
*
**
* Number of occurrences for each genotype.
* This does not include genotype with all alleles missing (e.g. ./.), but it includes partially missing genotypes like "./0" or "./1".
* Total sum of counts should be equal to the count of samples.
*
*
* map<string, int32> genotypeCount = 6;
*/
public Builder putAllGenotypeCount(
java.util.Map values) {
internalGetMutableGenotypeCount().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Float> genotypeFreq_;
private com.google.protobuf.MapField
internalGetGenotypeFreq() {
if (genotypeFreq_ == null) {
return com.google.protobuf.MapField.emptyMapField(
GenotypeFreqDefaultEntryHolder.defaultEntry);
}
return genotypeFreq_;
}
private com.google.protobuf.MapField
internalGetMutableGenotypeFreq() {
onChanged();;
if (genotypeFreq_ == null) {
genotypeFreq_ = com.google.protobuf.MapField.newMapField(
GenotypeFreqDefaultEntryHolder.defaultEntry);
}
if (!genotypeFreq_.isMutable()) {
genotypeFreq_ = genotypeFreq_.copy();
}
return genotypeFreq_;
}
public int getGenotypeFreqCount() {
return internalGetGenotypeFreq().getMap().size();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public boolean containsGenotypeFreq(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetGenotypeFreq().getMap().containsKey(key);
}
/**
* Use {@link #getGenotypeFreqMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getGenotypeFreq() {
return getGenotypeFreqMap();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public java.util.Map getGenotypeFreqMap() {
return internalGetGenotypeFreq().getMap();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public float getGenotypeFreqOrDefault(
java.lang.String key,
float defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeFreq().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public float getGenotypeFreqOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetGenotypeFreq().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearGenotypeFreq() {
internalGetMutableGenotypeFreq().getMutableMap()
.clear();
return this;
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public Builder removeGenotypeFreq(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableGenotypeFreq().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableGenotypeFreq() {
return internalGetMutableGenotypeFreq().getMutableMap();
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public Builder putGenotypeFreq(
java.lang.String key,
float value) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableGenotypeFreq().getMutableMap()
.put(key, value);
return this;
}
/**
*
**
* Genotype frequency for each genotype found calculated from the genotypeCount and samplesCount, in the range [0,1]
* The sum of frequencies should be 1.
*
*
* map<string, float> genotypeFreq = 7;
*/
public Builder putAllGenotypeFreq(
java.util.Map values) {
internalGetMutableGenotypeFreq().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Integer> filterCount_;
private com.google.protobuf.MapField
internalGetFilterCount() {
if (filterCount_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FilterCountDefaultEntryHolder.defaultEntry);
}
return filterCount_;
}
private com.google.protobuf.MapField
internalGetMutableFilterCount() {
onChanged();;
if (filterCount_ == null) {
filterCount_ = com.google.protobuf.MapField.newMapField(
FilterCountDefaultEntryHolder.defaultEntry);
}
if (!filterCount_.isMutable()) {
filterCount_ = filterCount_.copy();
}
return filterCount_;
}
public int getFilterCountCount() {
return internalGetFilterCount().getMap().size();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public boolean containsFilterCount(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFilterCount().getMap().containsKey(key);
}
/**
* Use {@link #getFilterCountMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFilterCount() {
return getFilterCountMap();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public java.util.Map getFilterCountMap() {
return internalGetFilterCount().getMap();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public int getFilterCountOrDefault(
java.lang.String key,
int defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterCount().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public int getFilterCountOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterCount().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearFilterCount() {
internalGetMutableFilterCount().getMutableMap()
.clear();
return this;
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public Builder removeFilterCount(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFilterCount().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableFilterCount() {
return internalGetMutableFilterCount().getMutableMap();
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public Builder putFilterCount(
java.lang.String key,
int value) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFilterCount().getMutableMap()
.put(key, value);
return this;
}
/**
*
**
* The number of occurrences for each FILTER value in files from samples in this cohort reporting this variant.
* As each file can contain more than one filter value (usually separated by ';'), the total sum of counts could be greater than to the count of files.
*
*
* map<string, int32> filterCount = 14;
*/
public Builder putAllFilterCount(
java.util.Map values) {
internalGetMutableFilterCount().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Float> filterFreq_;
private com.google.protobuf.MapField
internalGetFilterFreq() {
if (filterFreq_ == null) {
return com.google.protobuf.MapField.emptyMapField(
FilterFreqDefaultEntryHolder.defaultEntry);
}
return filterFreq_;
}
private com.google.protobuf.MapField
internalGetMutableFilterFreq() {
onChanged();;
if (filterFreq_ == null) {
filterFreq_ = com.google.protobuf.MapField.newMapField(
FilterFreqDefaultEntryHolder.defaultEntry);
}
if (!filterFreq_.isMutable()) {
filterFreq_ = filterFreq_.copy();
}
return filterFreq_;
}
public int getFilterFreqCount() {
return internalGetFilterFreq().getMap().size();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public boolean containsFilterFreq(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetFilterFreq().getMap().containsKey(key);
}
/**
* Use {@link #getFilterFreqMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getFilterFreq() {
return getFilterFreqMap();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public java.util.Map getFilterFreqMap() {
return internalGetFilterFreq().getMap();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public float getFilterFreqOrDefault(
java.lang.String key,
float defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterFreq().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public float getFilterFreqOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetFilterFreq().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearFilterFreq() {
internalGetMutableFilterFreq().getMutableMap()
.clear();
return this;
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public Builder removeFilterFreq(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFilterFreq().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableFilterFreq() {
return internalGetMutableFilterFreq().getMutableMap();
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public Builder putFilterFreq(
java.lang.String key,
float value) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableFilterFreq().getMutableMap()
.put(key, value);
return this;
}
/**
*
**
* Frequency of each filter calculated from the filterCount and filesCount, in the range [0,1]
*
*
* map<string, float> filterFreq = 15;
*/
public Builder putAllFilterFreq(
java.util.Map values) {
internalGetMutableFilterFreq().getMutableMap()
.putAll(values);
return this;
}
private int qualityCount_ ;
/**
*
**
* The number of files from samples in this cohort reporting this variant with valid QUAL values.
* This value is used as denominator to obtain the qualityAvg.
*
*
* int32 qualityCount = 20;
* @return The qualityCount.
*/
public int getQualityCount() {
return qualityCount_;
}
/**
*
**
* The number of files from samples in this cohort reporting this variant with valid QUAL values.
* This value is used as denominator to obtain the qualityAvg.
*
*
* int32 qualityCount = 20;
* @param value The qualityCount to set.
* @return This builder for chaining.
*/
public Builder setQualityCount(int value) {
qualityCount_ = value;
onChanged();
return this;
}
/**
*
**
* The number of files from samples in this cohort reporting this variant with valid QUAL values.
* This value is used as denominator to obtain the qualityAvg.
*
*
* int32 qualityCount = 20;
* @return This builder for chaining.
*/
public Builder clearQualityCount() {
qualityCount_ = 0;
onChanged();
return this;
}
private float qualityAvg_ ;
/**
*
**
* The average Quality value for files with valid QUAL values from samples in this cohort reporting this variant.
* Some files may not have defined the QUAL value, so the sampling could be less than the filesCount.
*
*
* float qualityAvg = 16;
* @return The qualityAvg.
*/
public float getQualityAvg() {
return qualityAvg_;
}
/**
*
**
* The average Quality value for files with valid QUAL values from samples in this cohort reporting this variant.
* Some files may not have defined the QUAL value, so the sampling could be less than the filesCount.
*
*
* float qualityAvg = 16;
* @param value The qualityAvg to set.
* @return This builder for chaining.
*/
public Builder setQualityAvg(float value) {
qualityAvg_ = value;
onChanged();
return this;
}
/**
*
**
* The average Quality value for files with valid QUAL values from samples in this cohort reporting this variant.
* Some files may not have defined the QUAL value, so the sampling could be less than the filesCount.
*
*
* float qualityAvg = 16;
* @return This builder for chaining.
*/
public Builder clearQualityAvg() {
qualityAvg_ = 0F;
onChanged();
return this;
}
private float maf_ ;
/**
*
**
* Minor allele frequency. Frequency of the less common allele between the reference and the main alternate alleles.
* This value does not take into acconunt secondary alternates.
*
*
* float maf = 10;
* @return The maf.
*/
public float getMaf() {
return maf_;
}
/**
*
**
* Minor allele frequency. Frequency of the less common allele between the reference and the main alternate alleles.
* This value does not take into acconunt secondary alternates.
*
*
* float maf = 10;
* @param value The maf to set.
* @return This builder for chaining.
*/
public Builder setMaf(float value) {
maf_ = value;
onChanged();
return this;
}
/**
*
**
* Minor allele frequency. Frequency of the less common allele between the reference and the main alternate alleles.
* This value does not take into acconunt secondary alternates.
*
*
* float maf = 10;
* @return This builder for chaining.
*/
public Builder clearMaf() {
maf_ = 0F;
onChanged();
return this;
}
private float mgf_ ;
/**
*
**
* Minor genotype frequency. Frequency of the less common genotype seen in this variant.
* This value takes into account all values from the genotypeFreq map.
*
*
* float mgf = 11;
* @return The mgf.
*/
public float getMgf() {
return mgf_;
}
/**
*
**
* Minor genotype frequency. Frequency of the less common genotype seen in this variant.
* This value takes into account all values from the genotypeFreq map.
*
*
* float mgf = 11;
* @param value The mgf to set.
* @return This builder for chaining.
*/
public Builder setMgf(float value) {
mgf_ = value;
onChanged();
return this;
}
/**
*
**
* Minor genotype frequency. Frequency of the less common genotype seen in this variant.
* This value takes into account all values from the genotypeFreq map.
*
*
* float mgf = 11;
* @return This builder for chaining.
*/
public Builder clearMgf() {
mgf_ = 0F;
onChanged();
return this;
}
private java.lang.Object mafAllele_ = "";
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return The mafAllele.
*/
public java.lang.String getMafAllele() {
java.lang.Object ref = mafAllele_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mafAllele_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return The bytes for mafAllele.
*/
public com.google.protobuf.ByteString
getMafAlleleBytes() {
java.lang.Object ref = mafAllele_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mafAllele_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @param value The mafAllele to set.
* @return This builder for chaining.
*/
public Builder setMafAllele(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mafAllele_ = value;
onChanged();
return this;
}
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @return This builder for chaining.
*/
public Builder clearMafAllele() {
mafAllele_ = getDefaultInstance().getMafAllele();
onChanged();
return this;
}
/**
*
**
* Allele with minor frequency
*
*
* string mafAllele = 12;
* @param value The bytes for mafAllele to set.
* @return This builder for chaining.
*/
public Builder setMafAlleleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mafAllele_ = value;
onChanged();
return this;
}
private java.lang.Object mgfGenotype_ = "";
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return The mgfGenotype.
*/
public java.lang.String getMgfGenotype() {
java.lang.Object ref = mgfGenotype_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mgfGenotype_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return The bytes for mgfGenotype.
*/
public com.google.protobuf.ByteString
getMgfGenotypeBytes() {
java.lang.Object ref = mgfGenotype_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mgfGenotype_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @param value The mgfGenotype to set.
* @return This builder for chaining.
*/
public Builder setMgfGenotype(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mgfGenotype_ = value;
onChanged();
return this;
}
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @return This builder for chaining.
*/
public Builder clearMgfGenotype() {
mgfGenotype_ = getDefaultInstance().getMgfGenotype();
onChanged();
return this;
}
/**
*
**
* Genotype with minor frequency
*
*
* string mgfGenotype = 13;
* @param value The bytes for mgfGenotype to set.
* @return This builder for chaining.
*/
public Builder setMgfGenotypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mgfGenotype_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.VariantStats)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.VariantStats)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VariantStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VariantStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OriginalCallOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.OriginalCall)
com.google.protobuf.MessageOrBuilder {
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return The variantId.
*/
java.lang.String getVariantId();
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return The bytes for variantId.
*/
com.google.protobuf.ByteString
getVariantIdBytes();
/**
*
**
* Alternate allele index of the original multi-allellic variant call in which was decomposed.
*
*
* int32 alleleIndex = 2;
* @return The alleleIndex.
*/
int getAlleleIndex();
}
/**
* Protobuf type {@code protobuf.opencb.OriginalCall}
*/
public static final class OriginalCall extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.OriginalCall)
OriginalCallOrBuilder {
private static final long serialVersionUID = 0L;
// Use OriginalCall.newBuilder() to construct.
private OriginalCall(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OriginalCall() {
variantId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OriginalCall();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OriginalCall(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
variantId_ = s;
break;
}
case 16: {
alleleIndex_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_OriginalCall_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_OriginalCall_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.class, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder.class);
}
public static final int VARIANTID_FIELD_NUMBER = 1;
private volatile java.lang.Object variantId_;
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return The variantId.
*/
public java.lang.String getVariantId() {
java.lang.Object ref = variantId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
variantId_ = s;
return s;
}
}
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return The bytes for variantId.
*/
public com.google.protobuf.ByteString
getVariantIdBytes() {
java.lang.Object ref = variantId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
variantId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ALLELEINDEX_FIELD_NUMBER = 2;
private int alleleIndex_;
/**
*
**
* Alternate allele index of the original multi-allellic variant call in which was decomposed.
*
*
* int32 alleleIndex = 2;
* @return The alleleIndex.
*/
public int getAlleleIndex() {
return alleleIndex_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getVariantIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, variantId_);
}
if (alleleIndex_ != 0) {
output.writeInt32(2, alleleIndex_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getVariantIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, variantId_);
}
if (alleleIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, alleleIndex_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall other = (org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall) obj;
if (!getVariantId()
.equals(other.getVariantId())) return false;
if (getAlleleIndex()
!= other.getAlleleIndex()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VARIANTID_FIELD_NUMBER;
hash = (53 * hash) + getVariantId().hashCode();
hash = (37 * hash) + ALLELEINDEX_FIELD_NUMBER;
hash = (53 * hash) + getAlleleIndex();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.OriginalCall}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.OriginalCall)
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_OriginalCall_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_OriginalCall_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.class, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
variantId_ = "";
alleleIndex_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_OriginalCall_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall result = new org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall(this);
result.variantId_ = variantId_;
result.alleleIndex_ = alleleIndex_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.getDefaultInstance()) return this;
if (!other.getVariantId().isEmpty()) {
variantId_ = other.variantId_;
onChanged();
}
if (other.getAlleleIndex() != 0) {
setAlleleIndex(other.getAlleleIndex());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object variantId_ = "";
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return The variantId.
*/
public java.lang.String getVariantId() {
java.lang.Object ref = variantId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
variantId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return The bytes for variantId.
*/
public com.google.protobuf.ByteString
getVariantIdBytes() {
java.lang.Object ref = variantId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
variantId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @param value The variantId to set.
* @return This builder for chaining.
*/
public Builder setVariantId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
variantId_ = value;
onChanged();
return this;
}
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @return This builder for chaining.
*/
public Builder clearVariantId() {
variantId_ = getDefaultInstance().getVariantId();
onChanged();
return this;
}
/**
*
**
* Original variant ID before normalization including all secondary alternates.
*
*
* string variantId = 1;
* @param value The bytes for variantId to set.
* @return This builder for chaining.
*/
public Builder setVariantIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
variantId_ = value;
onChanged();
return this;
}
private int alleleIndex_ ;
/**
*
**
* Alternate allele index of the original multi-allellic variant call in which was decomposed.
*
*
* int32 alleleIndex = 2;
* @return The alleleIndex.
*/
public int getAlleleIndex() {
return alleleIndex_;
}
/**
*
**
* Alternate allele index of the original multi-allellic variant call in which was decomposed.
*
*
* int32 alleleIndex = 2;
* @param value The alleleIndex to set.
* @return This builder for chaining.
*/
public Builder setAlleleIndex(int value) {
alleleIndex_ = value;
onChanged();
return this;
}
/**
*
**
* Alternate allele index of the original multi-allellic variant call in which was decomposed.
*
*
* int32 alleleIndex = 2;
* @return This builder for chaining.
*/
public Builder clearAlleleIndex() {
alleleIndex_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.OriginalCall)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.OriginalCall)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OriginalCall parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OriginalCall(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FileEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.FileEntry)
com.google.protobuf.MessageOrBuilder {
/**
* string fileId = 1;
* @return The fileId.
*/
java.lang.String getFileId();
/**
* string fileId = 1;
* @return The bytes for fileId.
*/
com.google.protobuf.ByteString
getFileIdBytes();
/**
* .protobuf.opencb.OriginalCall call = 2;
* @return Whether the call field is set.
*/
boolean hasCall();
/**
* .protobuf.opencb.OriginalCall call = 2;
* @return The call.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall getCall();
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder getCallOrBuilder();
/**
* map<string, string> data = 3;
*/
int getDataCount();
/**
* map<string, string> data = 3;
*/
boolean containsData(
java.lang.String key);
/**
* Use {@link #getDataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getData();
/**
* map<string, string> data = 3;
*/
java.util.Map
getDataMap();
/**
* map<string, string> data = 3;
*/
java.lang.String getDataOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
* map<string, string> data = 3;
*/
java.lang.String getDataOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code protobuf.opencb.FileEntry}
*/
public static final class FileEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.FileEntry)
FileEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use FileEntry.newBuilder() to construct.
private FileEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FileEntry() {
fileId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FileEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FileEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
fileId_ = s;
break;
}
case 18: {
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder subBuilder = null;
if (call_ != null) {
subBuilder = call_.toBuilder();
}
call_ = input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(call_);
call_ = subBuilder.buildPartial();
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
data_ = com.google.protobuf.MapField.newMapField(
DataDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
data__ = input.readMessage(
DataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
data_.getMutableMap().put(
data__.getKey(), data__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_FileEntry_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_FileEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.class, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder.class);
}
public static final int FILEID_FIELD_NUMBER = 1;
private volatile java.lang.Object fileId_;
/**
* string fileId = 1;
* @return The fileId.
*/
public java.lang.String getFileId() {
java.lang.Object ref = fileId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileId_ = s;
return s;
}
}
/**
* string fileId = 1;
* @return The bytes for fileId.
*/
public com.google.protobuf.ByteString
getFileIdBytes() {
java.lang.Object ref = fileId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALL_FIELD_NUMBER = 2;
private org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall call_;
/**
* .protobuf.opencb.OriginalCall call = 2;
* @return Whether the call field is set.
*/
public boolean hasCall() {
return call_ != null;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
* @return The call.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall getCall() {
return call_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.getDefaultInstance() : call_;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder getCallOrBuilder() {
return getCall();
}
public static final int DATA_FIELD_NUMBER = 3;
private static final class DataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_FileEntry_DataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> data_;
private com.google.protobuf.MapField
internalGetData() {
if (data_ == null) {
return com.google.protobuf.MapField.emptyMapField(
DataDefaultEntryHolder.defaultEntry);
}
return data_;
}
public int getDataCount() {
return internalGetData().getMap().size();
}
/**
* map<string, string> data = 3;
*/
public boolean containsData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetData().getMap().containsKey(key);
}
/**
* Use {@link #getDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getData() {
return getDataMap();
}
/**
* map<string, string> data = 3;
*/
public java.util.Map getDataMap() {
return internalGetData().getMap();
}
/**
* map<string, string> data = 3;
*/
public java.lang.String getDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> data = 3;
*/
public java.lang.String getDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getFileIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, fileId_);
}
if (call_ != null) {
output.writeMessage(2, getCall());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetData(),
DataDefaultEntryHolder.defaultEntry,
3);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getFileIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, fileId_);
}
if (call_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCall());
}
for (java.util.Map.Entry entry
: internalGetData().getMap().entrySet()) {
com.google.protobuf.MapEntry
data__ = DataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, data__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry other = (org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry) obj;
if (!getFileId()
.equals(other.getFileId())) return false;
if (hasCall() != other.hasCall()) return false;
if (hasCall()) {
if (!getCall()
.equals(other.getCall())) return false;
}
if (!internalGetData().equals(
other.internalGetData())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FILEID_FIELD_NUMBER;
hash = (53 * hash) + getFileId().hashCode();
if (hasCall()) {
hash = (37 * hash) + CALL_FIELD_NUMBER;
hash = (53 * hash) + getCall().hashCode();
}
if (!internalGetData().getMap().isEmpty()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.FileEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.FileEntry)
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_FileEntry_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 3:
return internalGetMutableData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_FileEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.class, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
fileId_ = "";
if (callBuilder_ == null) {
call_ = null;
} else {
call_ = null;
callBuilder_ = null;
}
internalGetMutableData().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_FileEntry_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry result = new org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry(this);
int from_bitField0_ = bitField0_;
result.fileId_ = fileId_;
if (callBuilder_ == null) {
result.call_ = call_;
} else {
result.call_ = callBuilder_.build();
}
result.data_ = internalGetData();
result.data_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.getDefaultInstance()) return this;
if (!other.getFileId().isEmpty()) {
fileId_ = other.fileId_;
onChanged();
}
if (other.hasCall()) {
mergeCall(other.getCall());
}
internalGetMutableData().mergeFrom(
other.internalGetData());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object fileId_ = "";
/**
* string fileId = 1;
* @return The fileId.
*/
public java.lang.String getFileId() {
java.lang.Object ref = fileId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string fileId = 1;
* @return The bytes for fileId.
*/
public com.google.protobuf.ByteString
getFileIdBytes() {
java.lang.Object ref = fileId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string fileId = 1;
* @param value The fileId to set.
* @return This builder for chaining.
*/
public Builder setFileId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fileId_ = value;
onChanged();
return this;
}
/**
* string fileId = 1;
* @return This builder for chaining.
*/
public Builder clearFileId() {
fileId_ = getDefaultInstance().getFileId();
onChanged();
return this;
}
/**
* string fileId = 1;
* @param value The bytes for fileId to set.
* @return This builder for chaining.
*/
public Builder setFileIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fileId_ = value;
onChanged();
return this;
}
private org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall call_;
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder> callBuilder_;
/**
* .protobuf.opencb.OriginalCall call = 2;
* @return Whether the call field is set.
*/
public boolean hasCall() {
return callBuilder_ != null || call_ != null;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
* @return The call.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall getCall() {
if (callBuilder_ == null) {
return call_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.getDefaultInstance() : call_;
} else {
return callBuilder_.getMessage();
}
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public Builder setCall(org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall value) {
if (callBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
call_ = value;
onChanged();
} else {
callBuilder_.setMessage(value);
}
return this;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public Builder setCall(
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder builderForValue) {
if (callBuilder_ == null) {
call_ = builderForValue.build();
onChanged();
} else {
callBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public Builder mergeCall(org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall value) {
if (callBuilder_ == null) {
if (call_ != null) {
call_ =
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.newBuilder(call_).mergeFrom(value).buildPartial();
} else {
call_ = value;
}
onChanged();
} else {
callBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public Builder clearCall() {
if (callBuilder_ == null) {
call_ = null;
onChanged();
} else {
call_ = null;
callBuilder_ = null;
}
return this;
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder getCallBuilder() {
onChanged();
return getCallFieldBuilder().getBuilder();
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder getCallOrBuilder() {
if (callBuilder_ != null) {
return callBuilder_.getMessageOrBuilder();
} else {
return call_ == null ?
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.getDefaultInstance() : call_;
}
}
/**
* .protobuf.opencb.OriginalCall call = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder>
getCallFieldBuilder() {
if (callBuilder_ == null) {
callBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCall.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.OriginalCallOrBuilder>(
getCall(),
getParentForChildren(),
isClean());
call_ = null;
}
return callBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> data_;
private com.google.protobuf.MapField
internalGetData() {
if (data_ == null) {
return com.google.protobuf.MapField.emptyMapField(
DataDefaultEntryHolder.defaultEntry);
}
return data_;
}
private com.google.protobuf.MapField
internalGetMutableData() {
onChanged();;
if (data_ == null) {
data_ = com.google.protobuf.MapField.newMapField(
DataDefaultEntryHolder.defaultEntry);
}
if (!data_.isMutable()) {
data_ = data_.copy();
}
return data_;
}
public int getDataCount() {
return internalGetData().getMap().size();
}
/**
* map<string, string> data = 3;
*/
public boolean containsData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetData().getMap().containsKey(key);
}
/**
* Use {@link #getDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getData() {
return getDataMap();
}
/**
* map<string, string> data = 3;
*/
public java.util.Map getDataMap() {
return internalGetData().getMap();
}
/**
* map<string, string> data = 3;
*/
public java.lang.String getDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> data = 3;
*/
public java.lang.String getDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearData() {
internalGetMutableData().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> data = 3;
*/
public Builder removeData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableData().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableData() {
return internalGetMutableData().getMutableMap();
}
/**
* map<string, string> data = 3;
*/
public Builder putData(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableData().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> data = 3;
*/
public Builder putAllData(
java.util.Map values) {
internalGetMutableData().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.FileEntry)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.FileEntry)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FileEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FileEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AlternateCoordinateOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.AlternateCoordinate)
com.google.protobuf.MessageOrBuilder {
/**
* string chromosome = 1;
* @return The chromosome.
*/
java.lang.String getChromosome();
/**
* string chromosome = 1;
* @return The bytes for chromosome.
*/
com.google.protobuf.ByteString
getChromosomeBytes();
/**
* int32 start = 2;
* @return The start.
*/
int getStart();
/**
* int32 end = 3;
* @return The end.
*/
int getEnd();
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The reference.
*/
java.lang.String getReference();
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The bytes for reference.
*/
com.google.protobuf.ByteString
getReferenceBytes();
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The alternate.
*/
java.lang.String getAlternate();
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The bytes for alternate.
*/
com.google.protobuf.ByteString
getAlternateBytes();
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return The type.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType getType();
}
/**
* Protobuf type {@code protobuf.opencb.AlternateCoordinate}
*/
public static final class AlternateCoordinate extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.AlternateCoordinate)
AlternateCoordinateOrBuilder {
private static final long serialVersionUID = 0L;
// Use AlternateCoordinate.newBuilder() to construct.
private AlternateCoordinate(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AlternateCoordinate() {
chromosome_ = "";
reference_ = "";
alternate_ = "";
type_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AlternateCoordinate();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AlternateCoordinate(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
chromosome_ = s;
break;
}
case 16: {
start_ = input.readInt32();
break;
}
case 24: {
end_ = input.readInt32();
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
reference_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
alternate_ = s;
break;
}
case 48: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_AlternateCoordinate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_AlternateCoordinate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.class, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder.class);
}
public static final int CHROMOSOME_FIELD_NUMBER = 1;
private volatile java.lang.Object chromosome_;
/**
* string chromosome = 1;
* @return The chromosome.
*/
public java.lang.String getChromosome() {
java.lang.Object ref = chromosome_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
chromosome_ = s;
return s;
}
}
/**
* string chromosome = 1;
* @return The bytes for chromosome.
*/
public com.google.protobuf.ByteString
getChromosomeBytes() {
java.lang.Object ref = chromosome_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
chromosome_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int START_FIELD_NUMBER = 2;
private int start_;
/**
* int32 start = 2;
* @return The start.
*/
public int getStart() {
return start_;
}
public static final int END_FIELD_NUMBER = 3;
private int end_;
/**
* int32 end = 3;
* @return The end.
*/
public int getEnd() {
return end_;
}
public static final int REFERENCE_FIELD_NUMBER = 4;
private volatile java.lang.Object reference_;
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The reference.
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
}
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The bytes for reference.
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ALTERNATE_FIELD_NUMBER = 5;
private volatile java.lang.Object alternate_;
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The alternate.
*/
public java.lang.String getAlternate() {
java.lang.Object ref = alternate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alternate_ = s;
return s;
}
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The bytes for alternate.
*/
public com.google.protobuf.ByteString
getAlternateBytes() {
java.lang.Object ref = alternate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alternate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 6;
private int type_;
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return The enum numeric value on the wire for type.
*/
public int getTypeValue() {
return type_;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return The type.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType getType() {
@SuppressWarnings("deprecation")
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType result = org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.valueOf(type_);
return result == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getChromosomeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, chromosome_);
}
if (start_ != 0) {
output.writeInt32(2, start_);
}
if (end_ != 0) {
output.writeInt32(3, end_);
}
if (!getReferenceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, reference_);
}
if (!getAlternateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, alternate_);
}
if (type_ != org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.NO_VARIATION.getNumber()) {
output.writeEnum(6, type_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getChromosomeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, chromosome_);
}
if (start_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, start_);
}
if (end_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, end_);
}
if (!getReferenceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, reference_);
}
if (!getAlternateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, alternate_);
}
if (type_ != org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.NO_VARIATION.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, type_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate other = (org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate) obj;
if (!getChromosome()
.equals(other.getChromosome())) return false;
if (getStart()
!= other.getStart()) return false;
if (getEnd()
!= other.getEnd()) return false;
if (!getReference()
.equals(other.getReference())) return false;
if (!getAlternate()
.equals(other.getAlternate())) return false;
if (type_ != other.type_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CHROMOSOME_FIELD_NUMBER;
hash = (53 * hash) + getChromosome().hashCode();
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart();
hash = (37 * hash) + END_FIELD_NUMBER;
hash = (53 * hash) + getEnd();
hash = (37 * hash) + REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getReference().hashCode();
hash = (37 * hash) + ALTERNATE_FIELD_NUMBER;
hash = (53 * hash) + getAlternate().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.AlternateCoordinate}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.AlternateCoordinate)
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_AlternateCoordinate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_AlternateCoordinate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.class, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
chromosome_ = "";
start_ = 0;
end_ = 0;
reference_ = "";
alternate_ = "";
type_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_AlternateCoordinate_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate result = new org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate(this);
result.chromosome_ = chromosome_;
result.start_ = start_;
result.end_ = end_;
result.reference_ = reference_;
result.alternate_ = alternate_;
result.type_ = type_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.getDefaultInstance()) return this;
if (!other.getChromosome().isEmpty()) {
chromosome_ = other.chromosome_;
onChanged();
}
if (other.getStart() != 0) {
setStart(other.getStart());
}
if (other.getEnd() != 0) {
setEnd(other.getEnd());
}
if (!other.getReference().isEmpty()) {
reference_ = other.reference_;
onChanged();
}
if (!other.getAlternate().isEmpty()) {
alternate_ = other.alternate_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object chromosome_ = "";
/**
* string chromosome = 1;
* @return The chromosome.
*/
public java.lang.String getChromosome() {
java.lang.Object ref = chromosome_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
chromosome_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string chromosome = 1;
* @return The bytes for chromosome.
*/
public com.google.protobuf.ByteString
getChromosomeBytes() {
java.lang.Object ref = chromosome_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
chromosome_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string chromosome = 1;
* @param value The chromosome to set.
* @return This builder for chaining.
*/
public Builder setChromosome(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
chromosome_ = value;
onChanged();
return this;
}
/**
* string chromosome = 1;
* @return This builder for chaining.
*/
public Builder clearChromosome() {
chromosome_ = getDefaultInstance().getChromosome();
onChanged();
return this;
}
/**
* string chromosome = 1;
* @param value The bytes for chromosome to set.
* @return This builder for chaining.
*/
public Builder setChromosomeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
chromosome_ = value;
onChanged();
return this;
}
private int start_ ;
/**
* int32 start = 2;
* @return The start.
*/
public int getStart() {
return start_;
}
/**
* int32 start = 2;
* @param value The start to set.
* @return This builder for chaining.
*/
public Builder setStart(int value) {
start_ = value;
onChanged();
return this;
}
/**
* int32 start = 2;
* @return This builder for chaining.
*/
public Builder clearStart() {
start_ = 0;
onChanged();
return this;
}
private int end_ ;
/**
* int32 end = 3;
* @return The end.
*/
public int getEnd() {
return end_;
}
/**
* int32 end = 3;
* @param value The end to set.
* @return This builder for chaining.
*/
public Builder setEnd(int value) {
end_ = value;
onChanged();
return this;
}
/**
* int32 end = 3;
* @return This builder for chaining.
*/
public Builder clearEnd() {
end_ = 0;
onChanged();
return this;
}
private java.lang.Object reference_ = "";
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The reference.
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The bytes for reference.
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @param value The reference to set.
* @return This builder for chaining.
*/
public Builder setReference(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
reference_ = value;
onChanged();
return this;
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return This builder for chaining.
*/
public Builder clearReference() {
reference_ = getDefaultInstance().getReference();
onChanged();
return this;
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @param value The bytes for reference to set.
* @return This builder for chaining.
*/
public Builder setReferenceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
reference_ = value;
onChanged();
return this;
}
private java.lang.Object alternate_ = "";
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The alternate.
*/
public java.lang.String getAlternate() {
java.lang.Object ref = alternate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alternate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The bytes for alternate.
*/
public com.google.protobuf.ByteString
getAlternateBytes() {
java.lang.Object ref = alternate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alternate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @param value The alternate to set.
* @return This builder for chaining.
*/
public Builder setAlternate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
alternate_ = value;
onChanged();
return this;
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return This builder for chaining.
*/
public Builder clearAlternate() {
alternate_ = getDefaultInstance().getAlternate();
onChanged();
return this;
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @param value The bytes for alternate to set.
* @return This builder for chaining.
*/
public Builder setAlternateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
alternate_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return The enum numeric value on the wire for type.
*/
public int getTypeValue() {
return type_;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return The type.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType getType() {
@SuppressWarnings("deprecation")
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType result = org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.valueOf(type_);
return result == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.UNRECOGNIZED : result;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 6;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.AlternateCoordinate)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.AlternateCoordinate)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AlternateCoordinate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AlternateCoordinate(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SampleEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.SampleEntry)
com.google.protobuf.MessageOrBuilder {
/**
* string sampleId = 1;
* @return The sampleId.
*/
java.lang.String getSampleId();
/**
* string sampleId = 1;
* @return The bytes for sampleId.
*/
com.google.protobuf.ByteString
getSampleIdBytes();
/**
* int32 fileIndex = 2;
* @return The fileIndex.
*/
int getFileIndex();
/**
* repeated string data = 3;
* @return A list containing the data.
*/
java.util.List
getDataList();
/**
* repeated string data = 3;
* @return The count of data.
*/
int getDataCount();
/**
* repeated string data = 3;
* @param index The index of the element to return.
* @return The data at the given index.
*/
java.lang.String getData(int index);
/**
* repeated string data = 3;
* @param index The index of the value to return.
* @return The bytes of the data at the given index.
*/
com.google.protobuf.ByteString
getDataBytes(int index);
}
/**
* Protobuf type {@code protobuf.opencb.SampleEntry}
*/
public static final class SampleEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.SampleEntry)
SampleEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use SampleEntry.newBuilder() to construct.
private SampleEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SampleEntry() {
sampleId_ = "";
data_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SampleEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SampleEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
sampleId_ = s;
break;
}
case 16: {
fileIndex_ = input.readInt32();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
data_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
data_.add(s);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
data_ = data_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_SampleEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_SampleEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.class, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder.class);
}
public static final int SAMPLEID_FIELD_NUMBER = 1;
private volatile java.lang.Object sampleId_;
/**
* string sampleId = 1;
* @return The sampleId.
*/
public java.lang.String getSampleId() {
java.lang.Object ref = sampleId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sampleId_ = s;
return s;
}
}
/**
* string sampleId = 1;
* @return The bytes for sampleId.
*/
public com.google.protobuf.ByteString
getSampleIdBytes() {
java.lang.Object ref = sampleId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sampleId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILEINDEX_FIELD_NUMBER = 2;
private int fileIndex_;
/**
* int32 fileIndex = 2;
* @return The fileIndex.
*/
public int getFileIndex() {
return fileIndex_;
}
public static final int DATA_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList data_;
/**
* repeated string data = 3;
* @return A list containing the data.
*/
public com.google.protobuf.ProtocolStringList
getDataList() {
return data_;
}
/**
* repeated string data = 3;
* @return The count of data.
*/
public int getDataCount() {
return data_.size();
}
/**
* repeated string data = 3;
* @param index The index of the element to return.
* @return The data at the given index.
*/
public java.lang.String getData(int index) {
return data_.get(index);
}
/**
* repeated string data = 3;
* @param index The index of the value to return.
* @return The bytes of the data at the given index.
*/
public com.google.protobuf.ByteString
getDataBytes(int index) {
return data_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getSampleIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, sampleId_);
}
if (fileIndex_ != 0) {
output.writeInt32(2, fileIndex_);
}
for (int i = 0; i < data_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, data_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getSampleIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, sampleId_);
}
if (fileIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, fileIndex_);
}
{
int dataSize = 0;
for (int i = 0; i < data_.size(); i++) {
dataSize += computeStringSizeNoTag(data_.getRaw(i));
}
size += dataSize;
size += 1 * getDataList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry other = (org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry) obj;
if (!getSampleId()
.equals(other.getSampleId())) return false;
if (getFileIndex()
!= other.getFileIndex()) return false;
if (!getDataList()
.equals(other.getDataList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SAMPLEID_FIELD_NUMBER;
hash = (53 * hash) + getSampleId().hashCode();
hash = (37 * hash) + FILEINDEX_FIELD_NUMBER;
hash = (53 * hash) + getFileIndex();
if (getDataCount() > 0) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.SampleEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.SampleEntry)
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_SampleEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_SampleEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.class, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sampleId_ = "";
fileIndex_ = 0;
data_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_SampleEntry_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry result = new org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry(this);
int from_bitField0_ = bitField0_;
result.sampleId_ = sampleId_;
result.fileIndex_ = fileIndex_;
if (((bitField0_ & 0x00000001) != 0)) {
data_ = data_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.data_ = data_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.getDefaultInstance()) return this;
if (!other.getSampleId().isEmpty()) {
sampleId_ = other.sampleId_;
onChanged();
}
if (other.getFileIndex() != 0) {
setFileIndex(other.getFileIndex());
}
if (!other.data_.isEmpty()) {
if (data_.isEmpty()) {
data_ = other.data_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDataIsMutable();
data_.addAll(other.data_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object sampleId_ = "";
/**
* string sampleId = 1;
* @return The sampleId.
*/
public java.lang.String getSampleId() {
java.lang.Object ref = sampleId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sampleId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sampleId = 1;
* @return The bytes for sampleId.
*/
public com.google.protobuf.ByteString
getSampleIdBytes() {
java.lang.Object ref = sampleId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sampleId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sampleId = 1;
* @param value The sampleId to set.
* @return This builder for chaining.
*/
public Builder setSampleId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sampleId_ = value;
onChanged();
return this;
}
/**
* string sampleId = 1;
* @return This builder for chaining.
*/
public Builder clearSampleId() {
sampleId_ = getDefaultInstance().getSampleId();
onChanged();
return this;
}
/**
* string sampleId = 1;
* @param value The bytes for sampleId to set.
* @return This builder for chaining.
*/
public Builder setSampleIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sampleId_ = value;
onChanged();
return this;
}
private int fileIndex_ ;
/**
* int32 fileIndex = 2;
* @return The fileIndex.
*/
public int getFileIndex() {
return fileIndex_;
}
/**
* int32 fileIndex = 2;
* @param value The fileIndex to set.
* @return This builder for chaining.
*/
public Builder setFileIndex(int value) {
fileIndex_ = value;
onChanged();
return this;
}
/**
* int32 fileIndex = 2;
* @return This builder for chaining.
*/
public Builder clearFileIndex() {
fileIndex_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList data_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureDataIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
data_ = new com.google.protobuf.LazyStringArrayList(data_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string data = 3;
* @return A list containing the data.
*/
public com.google.protobuf.ProtocolStringList
getDataList() {
return data_.getUnmodifiableView();
}
/**
* repeated string data = 3;
* @return The count of data.
*/
public int getDataCount() {
return data_.size();
}
/**
* repeated string data = 3;
* @param index The index of the element to return.
* @return The data at the given index.
*/
public java.lang.String getData(int index) {
return data_.get(index);
}
/**
* repeated string data = 3;
* @param index The index of the value to return.
* @return The bytes of the data at the given index.
*/
public com.google.protobuf.ByteString
getDataBytes(int index) {
return data_.getByteString(index);
}
/**
* repeated string data = 3;
* @param index The index to set the value at.
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureDataIsMutable();
data_.set(index, value);
onChanged();
return this;
}
/**
* repeated string data = 3;
* @param value The data to add.
* @return This builder for chaining.
*/
public Builder addData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureDataIsMutable();
data_.add(value);
onChanged();
return this;
}
/**
* repeated string data = 3;
* @param values The data to add.
* @return This builder for chaining.
*/
public Builder addAllData(
java.lang.Iterable values) {
ensureDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, data_);
onChanged();
return this;
}
/**
* repeated string data = 3;
* @return This builder for chaining.
*/
public Builder clearData() {
data_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string data = 3;
* @param value The bytes of the data to add.
* @return This builder for chaining.
*/
public Builder addDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureDataIsMutable();
data_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.SampleEntry)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.SampleEntry)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SampleEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SampleEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StudyEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.StudyEntry)
com.google.protobuf.MessageOrBuilder {
/**
* string studyId = 1;
* @return The studyId.
*/
java.lang.String getStudyId();
/**
* string studyId = 1;
* @return The bytes for studyId.
*/
com.google.protobuf.ByteString
getStudyIdBytes();
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
java.util.List
getFilesList();
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry getFiles(int index);
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
int getFilesCount();
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder>
getFilesOrBuilderList();
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder getFilesOrBuilder(
int index);
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
java.util.List
getSecondaryAlternatesList();
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate getSecondaryAlternates(int index);
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
int getSecondaryAlternatesCount();
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder>
getSecondaryAlternatesOrBuilderList();
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder getSecondaryAlternatesOrBuilder(
int index);
/**
* repeated string sampleDataKeys = 4;
* @return A list containing the sampleDataKeys.
*/
java.util.List
getSampleDataKeysList();
/**
* repeated string sampleDataKeys = 4;
* @return The count of sampleDataKeys.
*/
int getSampleDataKeysCount();
/**
* repeated string sampleDataKeys = 4;
* @param index The index of the element to return.
* @return The sampleDataKeys at the given index.
*/
java.lang.String getSampleDataKeys(int index);
/**
* repeated string sampleDataKeys = 4;
* @param index The index of the value to return.
* @return The bytes of the sampleDataKeys at the given index.
*/
com.google.protobuf.ByteString
getSampleDataKeysBytes(int index);
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
java.util.List
getSamplesList();
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry getSamples(int index);
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
int getSamplesCount();
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder>
getSamplesOrBuilderList();
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder getSamplesOrBuilder(
int index);
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
java.util.List
getStatsList();
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats getStats(int index);
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
int getStatsCount();
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder>
getStatsOrBuilderList();
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder getStatsOrBuilder(
int index);
}
/**
* Protobuf type {@code protobuf.opencb.StudyEntry}
*/
public static final class StudyEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.StudyEntry)
StudyEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use StudyEntry.newBuilder() to construct.
private StudyEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StudyEntry() {
studyId_ = "";
files_ = java.util.Collections.emptyList();
secondaryAlternates_ = java.util.Collections.emptyList();
sampleDataKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
samples_ = java.util.Collections.emptyList();
stats_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StudyEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StudyEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
studyId_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
files_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
files_.add(
input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.parser(), extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
secondaryAlternates_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
secondaryAlternates_.add(
input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.parser(), extensionRegistry));
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
sampleDataKeys_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
sampleDataKeys_.add(s);
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
samples_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
samples_.add(
input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.parser(), extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
stats_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
stats_.add(
input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
files_ = java.util.Collections.unmodifiableList(files_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
secondaryAlternates_ = java.util.Collections.unmodifiableList(secondaryAlternates_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
sampleDataKeys_ = sampleDataKeys_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
samples_ = java.util.Collections.unmodifiableList(samples_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
stats_ = java.util.Collections.unmodifiableList(stats_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StudyEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StudyEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.class, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder.class);
}
public static final int STUDYID_FIELD_NUMBER = 1;
private volatile java.lang.Object studyId_;
/**
* string studyId = 1;
* @return The studyId.
*/
public java.lang.String getStudyId() {
java.lang.Object ref = studyId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
studyId_ = s;
return s;
}
}
/**
* string studyId = 1;
* @return The bytes for studyId.
*/
public com.google.protobuf.ByteString
getStudyIdBytes() {
java.lang.Object ref = studyId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
studyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILES_FIELD_NUMBER = 2;
private java.util.List files_;
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public java.util.List getFilesList() {
return files_;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder>
getFilesOrBuilderList() {
return files_;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public int getFilesCount() {
return files_.size();
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry getFiles(int index) {
return files_.get(index);
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder getFilesOrBuilder(
int index) {
return files_.get(index);
}
public static final int SECONDARYALTERNATES_FIELD_NUMBER = 3;
private java.util.List secondaryAlternates_;
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public java.util.List getSecondaryAlternatesList() {
return secondaryAlternates_;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder>
getSecondaryAlternatesOrBuilderList() {
return secondaryAlternates_;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public int getSecondaryAlternatesCount() {
return secondaryAlternates_.size();
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate getSecondaryAlternates(int index) {
return secondaryAlternates_.get(index);
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder getSecondaryAlternatesOrBuilder(
int index) {
return secondaryAlternates_.get(index);
}
public static final int SAMPLEDATAKEYS_FIELD_NUMBER = 4;
private com.google.protobuf.LazyStringList sampleDataKeys_;
/**
* repeated string sampleDataKeys = 4;
* @return A list containing the sampleDataKeys.
*/
public com.google.protobuf.ProtocolStringList
getSampleDataKeysList() {
return sampleDataKeys_;
}
/**
* repeated string sampleDataKeys = 4;
* @return The count of sampleDataKeys.
*/
public int getSampleDataKeysCount() {
return sampleDataKeys_.size();
}
/**
* repeated string sampleDataKeys = 4;
* @param index The index of the element to return.
* @return The sampleDataKeys at the given index.
*/
public java.lang.String getSampleDataKeys(int index) {
return sampleDataKeys_.get(index);
}
/**
* repeated string sampleDataKeys = 4;
* @param index The index of the value to return.
* @return The bytes of the sampleDataKeys at the given index.
*/
public com.google.protobuf.ByteString
getSampleDataKeysBytes(int index) {
return sampleDataKeys_.getByteString(index);
}
public static final int SAMPLES_FIELD_NUMBER = 5;
private java.util.List samples_;
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public java.util.List getSamplesList() {
return samples_;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder>
getSamplesOrBuilderList() {
return samples_;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public int getSamplesCount() {
return samples_.size();
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry getSamples(int index) {
return samples_.get(index);
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder getSamplesOrBuilder(
int index) {
return samples_.get(index);
}
public static final int STATS_FIELD_NUMBER = 6;
private java.util.List stats_;
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public java.util.List getStatsList() {
return stats_;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder>
getStatsOrBuilderList() {
return stats_;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public int getStatsCount() {
return stats_.size();
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats getStats(int index) {
return stats_.get(index);
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder getStatsOrBuilder(
int index) {
return stats_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getStudyIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, studyId_);
}
for (int i = 0; i < files_.size(); i++) {
output.writeMessage(2, files_.get(i));
}
for (int i = 0; i < secondaryAlternates_.size(); i++) {
output.writeMessage(3, secondaryAlternates_.get(i));
}
for (int i = 0; i < sampleDataKeys_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, sampleDataKeys_.getRaw(i));
}
for (int i = 0; i < samples_.size(); i++) {
output.writeMessage(5, samples_.get(i));
}
for (int i = 0; i < stats_.size(); i++) {
output.writeMessage(6, stats_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getStudyIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, studyId_);
}
for (int i = 0; i < files_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, files_.get(i));
}
for (int i = 0; i < secondaryAlternates_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, secondaryAlternates_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < sampleDataKeys_.size(); i++) {
dataSize += computeStringSizeNoTag(sampleDataKeys_.getRaw(i));
}
size += dataSize;
size += 1 * getSampleDataKeysList().size();
}
for (int i = 0; i < samples_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, samples_.get(i));
}
for (int i = 0; i < stats_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, stats_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry other = (org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry) obj;
if (!getStudyId()
.equals(other.getStudyId())) return false;
if (!getFilesList()
.equals(other.getFilesList())) return false;
if (!getSecondaryAlternatesList()
.equals(other.getSecondaryAlternatesList())) return false;
if (!getSampleDataKeysList()
.equals(other.getSampleDataKeysList())) return false;
if (!getSamplesList()
.equals(other.getSamplesList())) return false;
if (!getStatsList()
.equals(other.getStatsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STUDYID_FIELD_NUMBER;
hash = (53 * hash) + getStudyId().hashCode();
if (getFilesCount() > 0) {
hash = (37 * hash) + FILES_FIELD_NUMBER;
hash = (53 * hash) + getFilesList().hashCode();
}
if (getSecondaryAlternatesCount() > 0) {
hash = (37 * hash) + SECONDARYALTERNATES_FIELD_NUMBER;
hash = (53 * hash) + getSecondaryAlternatesList().hashCode();
}
if (getSampleDataKeysCount() > 0) {
hash = (37 * hash) + SAMPLEDATAKEYS_FIELD_NUMBER;
hash = (53 * hash) + getSampleDataKeysList().hashCode();
}
if (getSamplesCount() > 0) {
hash = (37 * hash) + SAMPLES_FIELD_NUMBER;
hash = (53 * hash) + getSamplesList().hashCode();
}
if (getStatsCount() > 0) {
hash = (37 * hash) + STATS_FIELD_NUMBER;
hash = (53 * hash) + getStatsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.StudyEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.StudyEntry)
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StudyEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StudyEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.class, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFilesFieldBuilder();
getSecondaryAlternatesFieldBuilder();
getSamplesFieldBuilder();
getStatsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
studyId_ = "";
if (filesBuilder_ == null) {
files_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
filesBuilder_.clear();
}
if (secondaryAlternatesBuilder_ == null) {
secondaryAlternates_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
secondaryAlternatesBuilder_.clear();
}
sampleDataKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
if (samplesBuilder_ == null) {
samples_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
samplesBuilder_.clear();
}
if (statsBuilder_ == null) {
stats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
statsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StudyEntry_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry result = new org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry(this);
int from_bitField0_ = bitField0_;
result.studyId_ = studyId_;
if (filesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
files_ = java.util.Collections.unmodifiableList(files_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.files_ = files_;
} else {
result.files_ = filesBuilder_.build();
}
if (secondaryAlternatesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
secondaryAlternates_ = java.util.Collections.unmodifiableList(secondaryAlternates_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.secondaryAlternates_ = secondaryAlternates_;
} else {
result.secondaryAlternates_ = secondaryAlternatesBuilder_.build();
}
if (((bitField0_ & 0x00000004) != 0)) {
sampleDataKeys_ = sampleDataKeys_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.sampleDataKeys_ = sampleDataKeys_;
if (samplesBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
samples_ = java.util.Collections.unmodifiableList(samples_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.samples_ = samples_;
} else {
result.samples_ = samplesBuilder_.build();
}
if (statsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
stats_ = java.util.Collections.unmodifiableList(stats_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.stats_ = stats_;
} else {
result.stats_ = statsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.getDefaultInstance()) return this;
if (!other.getStudyId().isEmpty()) {
studyId_ = other.studyId_;
onChanged();
}
if (filesBuilder_ == null) {
if (!other.files_.isEmpty()) {
if (files_.isEmpty()) {
files_ = other.files_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFilesIsMutable();
files_.addAll(other.files_);
}
onChanged();
}
} else {
if (!other.files_.isEmpty()) {
if (filesBuilder_.isEmpty()) {
filesBuilder_.dispose();
filesBuilder_ = null;
files_ = other.files_;
bitField0_ = (bitField0_ & ~0x00000001);
filesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFilesFieldBuilder() : null;
} else {
filesBuilder_.addAllMessages(other.files_);
}
}
}
if (secondaryAlternatesBuilder_ == null) {
if (!other.secondaryAlternates_.isEmpty()) {
if (secondaryAlternates_.isEmpty()) {
secondaryAlternates_ = other.secondaryAlternates_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.addAll(other.secondaryAlternates_);
}
onChanged();
}
} else {
if (!other.secondaryAlternates_.isEmpty()) {
if (secondaryAlternatesBuilder_.isEmpty()) {
secondaryAlternatesBuilder_.dispose();
secondaryAlternatesBuilder_ = null;
secondaryAlternates_ = other.secondaryAlternates_;
bitField0_ = (bitField0_ & ~0x00000002);
secondaryAlternatesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSecondaryAlternatesFieldBuilder() : null;
} else {
secondaryAlternatesBuilder_.addAllMessages(other.secondaryAlternates_);
}
}
}
if (!other.sampleDataKeys_.isEmpty()) {
if (sampleDataKeys_.isEmpty()) {
sampleDataKeys_ = other.sampleDataKeys_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureSampleDataKeysIsMutable();
sampleDataKeys_.addAll(other.sampleDataKeys_);
}
onChanged();
}
if (samplesBuilder_ == null) {
if (!other.samples_.isEmpty()) {
if (samples_.isEmpty()) {
samples_ = other.samples_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureSamplesIsMutable();
samples_.addAll(other.samples_);
}
onChanged();
}
} else {
if (!other.samples_.isEmpty()) {
if (samplesBuilder_.isEmpty()) {
samplesBuilder_.dispose();
samplesBuilder_ = null;
samples_ = other.samples_;
bitField0_ = (bitField0_ & ~0x00000008);
samplesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSamplesFieldBuilder() : null;
} else {
samplesBuilder_.addAllMessages(other.samples_);
}
}
}
if (statsBuilder_ == null) {
if (!other.stats_.isEmpty()) {
if (stats_.isEmpty()) {
stats_ = other.stats_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureStatsIsMutable();
stats_.addAll(other.stats_);
}
onChanged();
}
} else {
if (!other.stats_.isEmpty()) {
if (statsBuilder_.isEmpty()) {
statsBuilder_.dispose();
statsBuilder_ = null;
stats_ = other.stats_;
bitField0_ = (bitField0_ & ~0x00000010);
statsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStatsFieldBuilder() : null;
} else {
statsBuilder_.addAllMessages(other.stats_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object studyId_ = "";
/**
* string studyId = 1;
* @return The studyId.
*/
public java.lang.String getStudyId() {
java.lang.Object ref = studyId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
studyId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string studyId = 1;
* @return The bytes for studyId.
*/
public com.google.protobuf.ByteString
getStudyIdBytes() {
java.lang.Object ref = studyId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
studyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string studyId = 1;
* @param value The studyId to set.
* @return This builder for chaining.
*/
public Builder setStudyId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
studyId_ = value;
onChanged();
return this;
}
/**
* string studyId = 1;
* @return This builder for chaining.
*/
public Builder clearStudyId() {
studyId_ = getDefaultInstance().getStudyId();
onChanged();
return this;
}
/**
* string studyId = 1;
* @param value The bytes for studyId to set.
* @return This builder for chaining.
*/
public Builder setStudyIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
studyId_ = value;
onChanged();
return this;
}
private java.util.List files_ =
java.util.Collections.emptyList();
private void ensureFilesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
files_ = new java.util.ArrayList(files_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder> filesBuilder_;
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public java.util.List getFilesList() {
if (filesBuilder_ == null) {
return java.util.Collections.unmodifiableList(files_);
} else {
return filesBuilder_.getMessageList();
}
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public int getFilesCount() {
if (filesBuilder_ == null) {
return files_.size();
} else {
return filesBuilder_.getCount();
}
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry getFiles(int index) {
if (filesBuilder_ == null) {
return files_.get(index);
} else {
return filesBuilder_.getMessage(index);
}
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder setFiles(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.set(index, value);
onChanged();
} else {
filesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder setFiles(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.set(index, builderForValue.build());
onChanged();
} else {
filesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder addFiles(org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(value);
onChanged();
} else {
filesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder addFiles(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(index, value);
onChanged();
} else {
filesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder addFiles(
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(builderForValue.build());
onChanged();
} else {
filesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder addFiles(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(index, builderForValue.build());
onChanged();
} else {
filesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder addAllFiles(
java.lang.Iterable extends org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry> values) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, files_);
onChanged();
} else {
filesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder clearFiles() {
if (filesBuilder_ == null) {
files_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
filesBuilder_.clear();
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public Builder removeFiles(int index) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.remove(index);
onChanged();
} else {
filesBuilder_.remove(index);
}
return this;
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder getFilesBuilder(
int index) {
return getFilesFieldBuilder().getBuilder(index);
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder getFilesOrBuilder(
int index) {
if (filesBuilder_ == null) {
return files_.get(index); } else {
return filesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder>
getFilesOrBuilderList() {
if (filesBuilder_ != null) {
return filesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(files_);
}
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder addFilesBuilder() {
return getFilesFieldBuilder().addBuilder(
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.getDefaultInstance());
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder addFilesBuilder(
int index) {
return getFilesFieldBuilder().addBuilder(
index, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.getDefaultInstance());
}
/**
* repeated .protobuf.opencb.FileEntry files = 2;
*/
public java.util.List
getFilesBuilderList() {
return getFilesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder>
getFilesFieldBuilder() {
if (filesBuilder_ == null) {
filesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.FileEntryOrBuilder>(
files_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
files_ = null;
}
return filesBuilder_;
}
private java.util.List secondaryAlternates_ =
java.util.Collections.emptyList();
private void ensureSecondaryAlternatesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
secondaryAlternates_ = new java.util.ArrayList(secondaryAlternates_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder> secondaryAlternatesBuilder_;
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public java.util.List getSecondaryAlternatesList() {
if (secondaryAlternatesBuilder_ == null) {
return java.util.Collections.unmodifiableList(secondaryAlternates_);
} else {
return secondaryAlternatesBuilder_.getMessageList();
}
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public int getSecondaryAlternatesCount() {
if (secondaryAlternatesBuilder_ == null) {
return secondaryAlternates_.size();
} else {
return secondaryAlternatesBuilder_.getCount();
}
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate getSecondaryAlternates(int index) {
if (secondaryAlternatesBuilder_ == null) {
return secondaryAlternates_.get(index);
} else {
return secondaryAlternatesBuilder_.getMessage(index);
}
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder setSecondaryAlternates(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate value) {
if (secondaryAlternatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.set(index, value);
onChanged();
} else {
secondaryAlternatesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder setSecondaryAlternates(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder builderForValue) {
if (secondaryAlternatesBuilder_ == null) {
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.set(index, builderForValue.build());
onChanged();
} else {
secondaryAlternatesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder addSecondaryAlternates(org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate value) {
if (secondaryAlternatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.add(value);
onChanged();
} else {
secondaryAlternatesBuilder_.addMessage(value);
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder addSecondaryAlternates(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate value) {
if (secondaryAlternatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.add(index, value);
onChanged();
} else {
secondaryAlternatesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder addSecondaryAlternates(
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder builderForValue) {
if (secondaryAlternatesBuilder_ == null) {
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.add(builderForValue.build());
onChanged();
} else {
secondaryAlternatesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder addSecondaryAlternates(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder builderForValue) {
if (secondaryAlternatesBuilder_ == null) {
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.add(index, builderForValue.build());
onChanged();
} else {
secondaryAlternatesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder addAllSecondaryAlternates(
java.lang.Iterable extends org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate> values) {
if (secondaryAlternatesBuilder_ == null) {
ensureSecondaryAlternatesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, secondaryAlternates_);
onChanged();
} else {
secondaryAlternatesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder clearSecondaryAlternates() {
if (secondaryAlternatesBuilder_ == null) {
secondaryAlternates_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
secondaryAlternatesBuilder_.clear();
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public Builder removeSecondaryAlternates(int index) {
if (secondaryAlternatesBuilder_ == null) {
ensureSecondaryAlternatesIsMutable();
secondaryAlternates_.remove(index);
onChanged();
} else {
secondaryAlternatesBuilder_.remove(index);
}
return this;
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder getSecondaryAlternatesBuilder(
int index) {
return getSecondaryAlternatesFieldBuilder().getBuilder(index);
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder getSecondaryAlternatesOrBuilder(
int index) {
if (secondaryAlternatesBuilder_ == null) {
return secondaryAlternates_.get(index); } else {
return secondaryAlternatesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder>
getSecondaryAlternatesOrBuilderList() {
if (secondaryAlternatesBuilder_ != null) {
return secondaryAlternatesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(secondaryAlternates_);
}
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder addSecondaryAlternatesBuilder() {
return getSecondaryAlternatesFieldBuilder().addBuilder(
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.getDefaultInstance());
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder addSecondaryAlternatesBuilder(
int index) {
return getSecondaryAlternatesFieldBuilder().addBuilder(
index, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.getDefaultInstance());
}
/**
*
**
* Alternate alleles that appear along with a variant alternate.
*
*
* repeated .protobuf.opencb.AlternateCoordinate secondaryAlternates = 3;
*/
public java.util.List
getSecondaryAlternatesBuilderList() {
return getSecondaryAlternatesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder>
getSecondaryAlternatesFieldBuilder() {
if (secondaryAlternatesBuilder_ == null) {
secondaryAlternatesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinate.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.AlternateCoordinateOrBuilder>(
secondaryAlternates_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
secondaryAlternates_ = null;
}
return secondaryAlternatesBuilder_;
}
private com.google.protobuf.LazyStringList sampleDataKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSampleDataKeysIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
sampleDataKeys_ = new com.google.protobuf.LazyStringArrayList(sampleDataKeys_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated string sampleDataKeys = 4;
* @return A list containing the sampleDataKeys.
*/
public com.google.protobuf.ProtocolStringList
getSampleDataKeysList() {
return sampleDataKeys_.getUnmodifiableView();
}
/**
* repeated string sampleDataKeys = 4;
* @return The count of sampleDataKeys.
*/
public int getSampleDataKeysCount() {
return sampleDataKeys_.size();
}
/**
* repeated string sampleDataKeys = 4;
* @param index The index of the element to return.
* @return The sampleDataKeys at the given index.
*/
public java.lang.String getSampleDataKeys(int index) {
return sampleDataKeys_.get(index);
}
/**
* repeated string sampleDataKeys = 4;
* @param index The index of the value to return.
* @return The bytes of the sampleDataKeys at the given index.
*/
public com.google.protobuf.ByteString
getSampleDataKeysBytes(int index) {
return sampleDataKeys_.getByteString(index);
}
/**
* repeated string sampleDataKeys = 4;
* @param index The index to set the value at.
* @param value The sampleDataKeys to set.
* @return This builder for chaining.
*/
public Builder setSampleDataKeys(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSampleDataKeysIsMutable();
sampleDataKeys_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sampleDataKeys = 4;
* @param value The sampleDataKeys to add.
* @return This builder for chaining.
*/
public Builder addSampleDataKeys(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSampleDataKeysIsMutable();
sampleDataKeys_.add(value);
onChanged();
return this;
}
/**
* repeated string sampleDataKeys = 4;
* @param values The sampleDataKeys to add.
* @return This builder for chaining.
*/
public Builder addAllSampleDataKeys(
java.lang.Iterable values) {
ensureSampleDataKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sampleDataKeys_);
onChanged();
return this;
}
/**
* repeated string sampleDataKeys = 4;
* @return This builder for chaining.
*/
public Builder clearSampleDataKeys() {
sampleDataKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* repeated string sampleDataKeys = 4;
* @param value The bytes of the sampleDataKeys to add.
* @return This builder for chaining.
*/
public Builder addSampleDataKeysBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSampleDataKeysIsMutable();
sampleDataKeys_.add(value);
onChanged();
return this;
}
private java.util.List samples_ =
java.util.Collections.emptyList();
private void ensureSamplesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
samples_ = new java.util.ArrayList(samples_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder> samplesBuilder_;
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public java.util.List getSamplesList() {
if (samplesBuilder_ == null) {
return java.util.Collections.unmodifiableList(samples_);
} else {
return samplesBuilder_.getMessageList();
}
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public int getSamplesCount() {
if (samplesBuilder_ == null) {
return samples_.size();
} else {
return samplesBuilder_.getCount();
}
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry getSamples(int index) {
if (samplesBuilder_ == null) {
return samples_.get(index);
} else {
return samplesBuilder_.getMessage(index);
}
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder setSamples(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry value) {
if (samplesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSamplesIsMutable();
samples_.set(index, value);
onChanged();
} else {
samplesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder setSamples(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder builderForValue) {
if (samplesBuilder_ == null) {
ensureSamplesIsMutable();
samples_.set(index, builderForValue.build());
onChanged();
} else {
samplesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder addSamples(org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry value) {
if (samplesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSamplesIsMutable();
samples_.add(value);
onChanged();
} else {
samplesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder addSamples(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry value) {
if (samplesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSamplesIsMutable();
samples_.add(index, value);
onChanged();
} else {
samplesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder addSamples(
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder builderForValue) {
if (samplesBuilder_ == null) {
ensureSamplesIsMutable();
samples_.add(builderForValue.build());
onChanged();
} else {
samplesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder addSamples(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder builderForValue) {
if (samplesBuilder_ == null) {
ensureSamplesIsMutable();
samples_.add(index, builderForValue.build());
onChanged();
} else {
samplesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder addAllSamples(
java.lang.Iterable extends org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry> values) {
if (samplesBuilder_ == null) {
ensureSamplesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, samples_);
onChanged();
} else {
samplesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder clearSamples() {
if (samplesBuilder_ == null) {
samples_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
samplesBuilder_.clear();
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public Builder removeSamples(int index) {
if (samplesBuilder_ == null) {
ensureSamplesIsMutable();
samples_.remove(index);
onChanged();
} else {
samplesBuilder_.remove(index);
}
return this;
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder getSamplesBuilder(
int index) {
return getSamplesFieldBuilder().getBuilder(index);
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder getSamplesOrBuilder(
int index) {
if (samplesBuilder_ == null) {
return samples_.get(index); } else {
return samplesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder>
getSamplesOrBuilderList() {
if (samplesBuilder_ != null) {
return samplesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(samples_);
}
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder addSamplesBuilder() {
return getSamplesFieldBuilder().addBuilder(
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.getDefaultInstance());
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder addSamplesBuilder(
int index) {
return getSamplesFieldBuilder().addBuilder(
index, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.getDefaultInstance());
}
/**
* repeated .protobuf.opencb.SampleEntry samples = 5;
*/
public java.util.List
getSamplesBuilderList() {
return getSamplesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder>
getSamplesFieldBuilder() {
if (samplesBuilder_ == null) {
samplesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.SampleEntryOrBuilder>(
samples_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
samples_ = null;
}
return samplesBuilder_;
}
private java.util.List stats_ =
java.util.Collections.emptyList();
private void ensureStatsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
stats_ = new java.util.ArrayList(stats_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder> statsBuilder_;
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public java.util.List getStatsList() {
if (statsBuilder_ == null) {
return java.util.Collections.unmodifiableList(stats_);
} else {
return statsBuilder_.getMessageList();
}
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public int getStatsCount() {
if (statsBuilder_ == null) {
return stats_.size();
} else {
return statsBuilder_.getCount();
}
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats getStats(int index) {
if (statsBuilder_ == null) {
return stats_.get(index);
} else {
return statsBuilder_.getMessage(index);
}
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder setStats(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStatsIsMutable();
stats_.set(index, value);
onChanged();
} else {
statsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder setStats(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder builderForValue) {
if (statsBuilder_ == null) {
ensureStatsIsMutable();
stats_.set(index, builderForValue.build());
onChanged();
} else {
statsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder addStats(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStatsIsMutable();
stats_.add(value);
onChanged();
} else {
statsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder addStats(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStatsIsMutable();
stats_.add(index, value);
onChanged();
} else {
statsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder addStats(
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder builderForValue) {
if (statsBuilder_ == null) {
ensureStatsIsMutable();
stats_.add(builderForValue.build());
onChanged();
} else {
statsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder addStats(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder builderForValue) {
if (statsBuilder_ == null) {
ensureStatsIsMutable();
stats_.add(index, builderForValue.build());
onChanged();
} else {
statsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder addAllStats(
java.lang.Iterable extends org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats> values) {
if (statsBuilder_ == null) {
ensureStatsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, stats_);
onChanged();
} else {
statsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder clearStats() {
if (statsBuilder_ == null) {
stats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
statsBuilder_.clear();
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public Builder removeStats(int index) {
if (statsBuilder_ == null) {
ensureStatsIsMutable();
stats_.remove(index);
onChanged();
} else {
statsBuilder_.remove(index);
}
return this;
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder getStatsBuilder(
int index) {
return getStatsFieldBuilder().getBuilder(index);
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder getStatsOrBuilder(
int index) {
if (statsBuilder_ == null) {
return stats_.get(index); } else {
return statsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder>
getStatsOrBuilderList() {
if (statsBuilder_ != null) {
return statsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(stats_);
}
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder addStatsBuilder() {
return getStatsFieldBuilder().addBuilder(
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.getDefaultInstance());
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder addStatsBuilder(
int index) {
return getStatsFieldBuilder().addBuilder(
index, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.getDefaultInstance());
}
/**
* repeated .protobuf.opencb.VariantStats stats = 6;
*/
public java.util.List
getStatsBuilderList() {
return getStatsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder>
getStatsFieldBuilder() {
if (statsBuilder_ == null) {
statsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStats.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantStatsOrBuilder>(
stats_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
stats_ = null;
}
return statsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.StudyEntry)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.StudyEntry)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StudyEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StudyEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BreakendMateOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.BreakendMate)
com.google.protobuf.MessageOrBuilder {
/**
* string chromosome = 1;
* @return The chromosome.
*/
java.lang.String getChromosome();
/**
* string chromosome = 1;
* @return The bytes for chromosome.
*/
com.google.protobuf.ByteString
getChromosomeBytes();
/**
* int32 position = 2;
* @return The position.
*/
int getPosition();
/**
* int32 ciPositionLeft = 3;
* @return The ciPositionLeft.
*/
int getCiPositionLeft();
/**
* int32 ciPositionRight = 4;
* @return The ciPositionRight.
*/
int getCiPositionRight();
}
/**
* Protobuf type {@code protobuf.opencb.BreakendMate}
*/
public static final class BreakendMate extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.BreakendMate)
BreakendMateOrBuilder {
private static final long serialVersionUID = 0L;
// Use BreakendMate.newBuilder() to construct.
private BreakendMate(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BreakendMate() {
chromosome_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BreakendMate();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BreakendMate(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
chromosome_ = s;
break;
}
case 16: {
position_ = input.readInt32();
break;
}
case 24: {
ciPositionLeft_ = input.readInt32();
break;
}
case 32: {
ciPositionRight_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_BreakendMate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_BreakendMate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.class, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder.class);
}
public static final int CHROMOSOME_FIELD_NUMBER = 1;
private volatile java.lang.Object chromosome_;
/**
* string chromosome = 1;
* @return The chromosome.
*/
public java.lang.String getChromosome() {
java.lang.Object ref = chromosome_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
chromosome_ = s;
return s;
}
}
/**
* string chromosome = 1;
* @return The bytes for chromosome.
*/
public com.google.protobuf.ByteString
getChromosomeBytes() {
java.lang.Object ref = chromosome_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
chromosome_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POSITION_FIELD_NUMBER = 2;
private int position_;
/**
* int32 position = 2;
* @return The position.
*/
public int getPosition() {
return position_;
}
public static final int CIPOSITIONLEFT_FIELD_NUMBER = 3;
private int ciPositionLeft_;
/**
* int32 ciPositionLeft = 3;
* @return The ciPositionLeft.
*/
public int getCiPositionLeft() {
return ciPositionLeft_;
}
public static final int CIPOSITIONRIGHT_FIELD_NUMBER = 4;
private int ciPositionRight_;
/**
* int32 ciPositionRight = 4;
* @return The ciPositionRight.
*/
public int getCiPositionRight() {
return ciPositionRight_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getChromosomeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, chromosome_);
}
if (position_ != 0) {
output.writeInt32(2, position_);
}
if (ciPositionLeft_ != 0) {
output.writeInt32(3, ciPositionLeft_);
}
if (ciPositionRight_ != 0) {
output.writeInt32(4, ciPositionRight_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getChromosomeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, chromosome_);
}
if (position_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, position_);
}
if (ciPositionLeft_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, ciPositionLeft_);
}
if (ciPositionRight_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, ciPositionRight_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate other = (org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate) obj;
if (!getChromosome()
.equals(other.getChromosome())) return false;
if (getPosition()
!= other.getPosition()) return false;
if (getCiPositionLeft()
!= other.getCiPositionLeft()) return false;
if (getCiPositionRight()
!= other.getCiPositionRight()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CHROMOSOME_FIELD_NUMBER;
hash = (53 * hash) + getChromosome().hashCode();
hash = (37 * hash) + POSITION_FIELD_NUMBER;
hash = (53 * hash) + getPosition();
hash = (37 * hash) + CIPOSITIONLEFT_FIELD_NUMBER;
hash = (53 * hash) + getCiPositionLeft();
hash = (37 * hash) + CIPOSITIONRIGHT_FIELD_NUMBER;
hash = (53 * hash) + getCiPositionRight();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.BreakendMate}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.BreakendMate)
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_BreakendMate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_BreakendMate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.class, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
chromosome_ = "";
position_ = 0;
ciPositionLeft_ = 0;
ciPositionRight_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_BreakendMate_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate result = new org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate(this);
result.chromosome_ = chromosome_;
result.position_ = position_;
result.ciPositionLeft_ = ciPositionLeft_;
result.ciPositionRight_ = ciPositionRight_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.getDefaultInstance()) return this;
if (!other.getChromosome().isEmpty()) {
chromosome_ = other.chromosome_;
onChanged();
}
if (other.getPosition() != 0) {
setPosition(other.getPosition());
}
if (other.getCiPositionLeft() != 0) {
setCiPositionLeft(other.getCiPositionLeft());
}
if (other.getCiPositionRight() != 0) {
setCiPositionRight(other.getCiPositionRight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object chromosome_ = "";
/**
* string chromosome = 1;
* @return The chromosome.
*/
public java.lang.String getChromosome() {
java.lang.Object ref = chromosome_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
chromosome_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string chromosome = 1;
* @return The bytes for chromosome.
*/
public com.google.protobuf.ByteString
getChromosomeBytes() {
java.lang.Object ref = chromosome_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
chromosome_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string chromosome = 1;
* @param value The chromosome to set.
* @return This builder for chaining.
*/
public Builder setChromosome(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
chromosome_ = value;
onChanged();
return this;
}
/**
* string chromosome = 1;
* @return This builder for chaining.
*/
public Builder clearChromosome() {
chromosome_ = getDefaultInstance().getChromosome();
onChanged();
return this;
}
/**
* string chromosome = 1;
* @param value The bytes for chromosome to set.
* @return This builder for chaining.
*/
public Builder setChromosomeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
chromosome_ = value;
onChanged();
return this;
}
private int position_ ;
/**
* int32 position = 2;
* @return The position.
*/
public int getPosition() {
return position_;
}
/**
* int32 position = 2;
* @param value The position to set.
* @return This builder for chaining.
*/
public Builder setPosition(int value) {
position_ = value;
onChanged();
return this;
}
/**
* int32 position = 2;
* @return This builder for chaining.
*/
public Builder clearPosition() {
position_ = 0;
onChanged();
return this;
}
private int ciPositionLeft_ ;
/**
* int32 ciPositionLeft = 3;
* @return The ciPositionLeft.
*/
public int getCiPositionLeft() {
return ciPositionLeft_;
}
/**
* int32 ciPositionLeft = 3;
* @param value The ciPositionLeft to set.
* @return This builder for chaining.
*/
public Builder setCiPositionLeft(int value) {
ciPositionLeft_ = value;
onChanged();
return this;
}
/**
* int32 ciPositionLeft = 3;
* @return This builder for chaining.
*/
public Builder clearCiPositionLeft() {
ciPositionLeft_ = 0;
onChanged();
return this;
}
private int ciPositionRight_ ;
/**
* int32 ciPositionRight = 4;
* @return The ciPositionRight.
*/
public int getCiPositionRight() {
return ciPositionRight_;
}
/**
* int32 ciPositionRight = 4;
* @param value The ciPositionRight to set.
* @return This builder for chaining.
*/
public Builder setCiPositionRight(int value) {
ciPositionRight_ = value;
onChanged();
return this;
}
/**
* int32 ciPositionRight = 4;
* @return This builder for chaining.
*/
public Builder clearCiPositionRight() {
ciPositionRight_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.BreakendMate)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.BreakendMate)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BreakendMate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BreakendMate(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BreakendOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.Breakend)
com.google.protobuf.MessageOrBuilder {
/**
* .protobuf.opencb.BreakendMate mate = 1;
* @return Whether the mate field is set.
*/
boolean hasMate();
/**
* .protobuf.opencb.BreakendMate mate = 1;
* @return The mate.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate getMate();
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder getMateOrBuilder();
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return The enum numeric value on the wire for orientation.
*/
int getOrientationValue();
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return The orientation.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation getOrientation();
/**
* string insSeq = 3;
* @return The insSeq.
*/
java.lang.String getInsSeq();
/**
* string insSeq = 3;
* @return The bytes for insSeq.
*/
com.google.protobuf.ByteString
getInsSeqBytes();
}
/**
* Protobuf type {@code protobuf.opencb.Breakend}
*/
public static final class Breakend extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.Breakend)
BreakendOrBuilder {
private static final long serialVersionUID = 0L;
// Use Breakend.newBuilder() to construct.
private Breakend(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Breakend() {
orientation_ = 0;
insSeq_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Breakend();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Breakend(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder subBuilder = null;
if (mate_ != null) {
subBuilder = mate_.toBuilder();
}
mate_ = input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(mate_);
mate_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
orientation_ = rawValue;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
insSeq_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Breakend_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Breakend_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.class, org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder.class);
}
public static final int MATE_FIELD_NUMBER = 1;
private org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate mate_;
/**
* .protobuf.opencb.BreakendMate mate = 1;
* @return Whether the mate field is set.
*/
public boolean hasMate() {
return mate_ != null;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
* @return The mate.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate getMate() {
return mate_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.getDefaultInstance() : mate_;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder getMateOrBuilder() {
return getMate();
}
public static final int ORIENTATION_FIELD_NUMBER = 2;
private int orientation_;
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return The enum numeric value on the wire for orientation.
*/
public int getOrientationValue() {
return orientation_;
}
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return The orientation.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation getOrientation() {
@SuppressWarnings("deprecation")
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation result = org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation.valueOf(orientation_);
return result == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation.UNRECOGNIZED : result;
}
public static final int INSSEQ_FIELD_NUMBER = 3;
private volatile java.lang.Object insSeq_;
/**
* string insSeq = 3;
* @return The insSeq.
*/
public java.lang.String getInsSeq() {
java.lang.Object ref = insSeq_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
insSeq_ = s;
return s;
}
}
/**
* string insSeq = 3;
* @return The bytes for insSeq.
*/
public com.google.protobuf.ByteString
getInsSeqBytes() {
java.lang.Object ref = insSeq_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
insSeq_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (mate_ != null) {
output.writeMessage(1, getMate());
}
if (orientation_ != org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation.SE.getNumber()) {
output.writeEnum(2, orientation_);
}
if (!getInsSeqBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, insSeq_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (mate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMate());
}
if (orientation_ != org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation.SE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, orientation_);
}
if (!getInsSeqBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, insSeq_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend other = (org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend) obj;
if (hasMate() != other.hasMate()) return false;
if (hasMate()) {
if (!getMate()
.equals(other.getMate())) return false;
}
if (orientation_ != other.orientation_) return false;
if (!getInsSeq()
.equals(other.getInsSeq())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMate()) {
hash = (37 * hash) + MATE_FIELD_NUMBER;
hash = (53 * hash) + getMate().hashCode();
}
hash = (37 * hash) + ORIENTATION_FIELD_NUMBER;
hash = (53 * hash) + orientation_;
hash = (37 * hash) + INSSEQ_FIELD_NUMBER;
hash = (53 * hash) + getInsSeq().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.Breakend}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.Breakend)
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Breakend_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Breakend_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.class, org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (mateBuilder_ == null) {
mate_ = null;
} else {
mate_ = null;
mateBuilder_ = null;
}
orientation_ = 0;
insSeq_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Breakend_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend result = new org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend(this);
if (mateBuilder_ == null) {
result.mate_ = mate_;
} else {
result.mate_ = mateBuilder_.build();
}
result.orientation_ = orientation_;
result.insSeq_ = insSeq_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.getDefaultInstance()) return this;
if (other.hasMate()) {
mergeMate(other.getMate());
}
if (other.orientation_ != 0) {
setOrientationValue(other.getOrientationValue());
}
if (!other.getInsSeq().isEmpty()) {
insSeq_ = other.insSeq_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate mate_;
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder> mateBuilder_;
/**
* .protobuf.opencb.BreakendMate mate = 1;
* @return Whether the mate field is set.
*/
public boolean hasMate() {
return mateBuilder_ != null || mate_ != null;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
* @return The mate.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate getMate() {
if (mateBuilder_ == null) {
return mate_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.getDefaultInstance() : mate_;
} else {
return mateBuilder_.getMessage();
}
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public Builder setMate(org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate value) {
if (mateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mate_ = value;
onChanged();
} else {
mateBuilder_.setMessage(value);
}
return this;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public Builder setMate(
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder builderForValue) {
if (mateBuilder_ == null) {
mate_ = builderForValue.build();
onChanged();
} else {
mateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public Builder mergeMate(org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate value) {
if (mateBuilder_ == null) {
if (mate_ != null) {
mate_ =
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.newBuilder(mate_).mergeFrom(value).buildPartial();
} else {
mate_ = value;
}
onChanged();
} else {
mateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public Builder clearMate() {
if (mateBuilder_ == null) {
mate_ = null;
onChanged();
} else {
mate_ = null;
mateBuilder_ = null;
}
return this;
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder getMateBuilder() {
onChanged();
return getMateFieldBuilder().getBuilder();
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder getMateOrBuilder() {
if (mateBuilder_ != null) {
return mateBuilder_.getMessageOrBuilder();
} else {
return mate_ == null ?
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.getDefaultInstance() : mate_;
}
}
/**
* .protobuf.opencb.BreakendMate mate = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder>
getMateFieldBuilder() {
if (mateBuilder_ == null) {
mateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMate.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendMateOrBuilder>(
getMate(),
getParentForChildren(),
isClean());
mate_ = null;
}
return mateBuilder_;
}
private int orientation_ = 0;
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return The enum numeric value on the wire for orientation.
*/
public int getOrientationValue() {
return orientation_;
}
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @param value The enum numeric value on the wire for orientation to set.
* @return This builder for chaining.
*/
public Builder setOrientationValue(int value) {
orientation_ = value;
onChanged();
return this;
}
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return The orientation.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation getOrientation() {
@SuppressWarnings("deprecation")
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation result = org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation.valueOf(orientation_);
return result == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation.UNRECOGNIZED : result;
}
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @param value The orientation to set.
* @return This builder for chaining.
*/
public Builder setOrientation(org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrientation value) {
if (value == null) {
throw new NullPointerException();
}
orientation_ = value.getNumber();
onChanged();
return this;
}
/**
* .protobuf.opencb.BreakendOrientation orientation = 2;
* @return This builder for chaining.
*/
public Builder clearOrientation() {
orientation_ = 0;
onChanged();
return this;
}
private java.lang.Object insSeq_ = "";
/**
* string insSeq = 3;
* @return The insSeq.
*/
public java.lang.String getInsSeq() {
java.lang.Object ref = insSeq_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
insSeq_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string insSeq = 3;
* @return The bytes for insSeq.
*/
public com.google.protobuf.ByteString
getInsSeqBytes() {
java.lang.Object ref = insSeq_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
insSeq_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string insSeq = 3;
* @param value The insSeq to set.
* @return This builder for chaining.
*/
public Builder setInsSeq(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
insSeq_ = value;
onChanged();
return this;
}
/**
* string insSeq = 3;
* @return This builder for chaining.
*/
public Builder clearInsSeq() {
insSeq_ = getDefaultInstance().getInsSeq();
onChanged();
return this;
}
/**
* string insSeq = 3;
* @param value The bytes for insSeq to set.
* @return This builder for chaining.
*/
public Builder setInsSeqBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
insSeq_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.Breakend)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.Breakend)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Breakend parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Breakend(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StructuralVariationOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.StructuralVariation)
com.google.protobuf.MessageOrBuilder {
/**
* int32 ciStartLeft = 1;
* @return The ciStartLeft.
*/
int getCiStartLeft();
/**
* int32 ciStartRight = 2;
* @return The ciStartRight.
*/
int getCiStartRight();
/**
* int32 ciEndLeft = 3;
* @return The ciEndLeft.
*/
int getCiEndLeft();
/**
* int32 ciEndRight = 4;
* @return The ciEndRight.
*/
int getCiEndRight();
/**
*
**
* Number of copies for CNV variants.
*
*
* int32 copyNumber = 5;
* @return The copyNumber.
*/
int getCopyNumber();
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return The leftSvInsSeq.
*/
java.lang.String getLeftSvInsSeq();
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return The bytes for leftSvInsSeq.
*/
com.google.protobuf.ByteString
getLeftSvInsSeqBytes();
/**
* string rightSvInsSeq = 7;
* @return The rightSvInsSeq.
*/
java.lang.String getRightSvInsSeq();
/**
* string rightSvInsSeq = 7;
* @return The bytes for rightSvInsSeq.
*/
com.google.protobuf.ByteString
getRightSvInsSeqBytes();
/**
* .protobuf.opencb.Breakend breakend = 9;
* @return Whether the breakend field is set.
*/
boolean hasBreakend();
/**
* .protobuf.opencb.Breakend breakend = 9;
* @return The breakend.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend getBreakend();
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder getBreakendOrBuilder();
}
/**
* Protobuf type {@code protobuf.opencb.StructuralVariation}
*/
public static final class StructuralVariation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.StructuralVariation)
StructuralVariationOrBuilder {
private static final long serialVersionUID = 0L;
// Use StructuralVariation.newBuilder() to construct.
private StructuralVariation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StructuralVariation() {
leftSvInsSeq_ = "";
rightSvInsSeq_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StructuralVariation();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StructuralVariation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
ciStartLeft_ = input.readInt32();
break;
}
case 16: {
ciStartRight_ = input.readInt32();
break;
}
case 24: {
ciEndLeft_ = input.readInt32();
break;
}
case 32: {
ciEndRight_ = input.readInt32();
break;
}
case 40: {
copyNumber_ = input.readInt32();
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
leftSvInsSeq_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
rightSvInsSeq_ = s;
break;
}
case 74: {
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder subBuilder = null;
if (breakend_ != null) {
subBuilder = breakend_.toBuilder();
}
breakend_ = input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(breakend_);
breakend_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StructuralVariation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StructuralVariation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.class, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder.class);
}
public static final int CISTARTLEFT_FIELD_NUMBER = 1;
private int ciStartLeft_;
/**
* int32 ciStartLeft = 1;
* @return The ciStartLeft.
*/
public int getCiStartLeft() {
return ciStartLeft_;
}
public static final int CISTARTRIGHT_FIELD_NUMBER = 2;
private int ciStartRight_;
/**
* int32 ciStartRight = 2;
* @return The ciStartRight.
*/
public int getCiStartRight() {
return ciStartRight_;
}
public static final int CIENDLEFT_FIELD_NUMBER = 3;
private int ciEndLeft_;
/**
* int32 ciEndLeft = 3;
* @return The ciEndLeft.
*/
public int getCiEndLeft() {
return ciEndLeft_;
}
public static final int CIENDRIGHT_FIELD_NUMBER = 4;
private int ciEndRight_;
/**
* int32 ciEndRight = 4;
* @return The ciEndRight.
*/
public int getCiEndRight() {
return ciEndRight_;
}
public static final int COPYNUMBER_FIELD_NUMBER = 5;
private int copyNumber_;
/**
*
**
* Number of copies for CNV variants.
*
*
* int32 copyNumber = 5;
* @return The copyNumber.
*/
public int getCopyNumber() {
return copyNumber_;
}
public static final int LEFTSVINSSEQ_FIELD_NUMBER = 6;
private volatile java.lang.Object leftSvInsSeq_;
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return The leftSvInsSeq.
*/
public java.lang.String getLeftSvInsSeq() {
java.lang.Object ref = leftSvInsSeq_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
leftSvInsSeq_ = s;
return s;
}
}
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return The bytes for leftSvInsSeq.
*/
public com.google.protobuf.ByteString
getLeftSvInsSeqBytes() {
java.lang.Object ref = leftSvInsSeq_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
leftSvInsSeq_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RIGHTSVINSSEQ_FIELD_NUMBER = 7;
private volatile java.lang.Object rightSvInsSeq_;
/**
* string rightSvInsSeq = 7;
* @return The rightSvInsSeq.
*/
public java.lang.String getRightSvInsSeq() {
java.lang.Object ref = rightSvInsSeq_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rightSvInsSeq_ = s;
return s;
}
}
/**
* string rightSvInsSeq = 7;
* @return The bytes for rightSvInsSeq.
*/
public com.google.protobuf.ByteString
getRightSvInsSeqBytes() {
java.lang.Object ref = rightSvInsSeq_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rightSvInsSeq_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BREAKEND_FIELD_NUMBER = 9;
private org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend breakend_;
/**
* .protobuf.opencb.Breakend breakend = 9;
* @return Whether the breakend field is set.
*/
public boolean hasBreakend() {
return breakend_ != null;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
* @return The breakend.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend getBreakend() {
return breakend_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.getDefaultInstance() : breakend_;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder getBreakendOrBuilder() {
return getBreakend();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (ciStartLeft_ != 0) {
output.writeInt32(1, ciStartLeft_);
}
if (ciStartRight_ != 0) {
output.writeInt32(2, ciStartRight_);
}
if (ciEndLeft_ != 0) {
output.writeInt32(3, ciEndLeft_);
}
if (ciEndRight_ != 0) {
output.writeInt32(4, ciEndRight_);
}
if (copyNumber_ != 0) {
output.writeInt32(5, copyNumber_);
}
if (!getLeftSvInsSeqBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, leftSvInsSeq_);
}
if (!getRightSvInsSeqBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, rightSvInsSeq_);
}
if (breakend_ != null) {
output.writeMessage(9, getBreakend());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (ciStartLeft_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, ciStartLeft_);
}
if (ciStartRight_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, ciStartRight_);
}
if (ciEndLeft_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, ciEndLeft_);
}
if (ciEndRight_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, ciEndRight_);
}
if (copyNumber_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, copyNumber_);
}
if (!getLeftSvInsSeqBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, leftSvInsSeq_);
}
if (!getRightSvInsSeqBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, rightSvInsSeq_);
}
if (breakend_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getBreakend());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation other = (org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation) obj;
if (getCiStartLeft()
!= other.getCiStartLeft()) return false;
if (getCiStartRight()
!= other.getCiStartRight()) return false;
if (getCiEndLeft()
!= other.getCiEndLeft()) return false;
if (getCiEndRight()
!= other.getCiEndRight()) return false;
if (getCopyNumber()
!= other.getCopyNumber()) return false;
if (!getLeftSvInsSeq()
.equals(other.getLeftSvInsSeq())) return false;
if (!getRightSvInsSeq()
.equals(other.getRightSvInsSeq())) return false;
if (hasBreakend() != other.hasBreakend()) return false;
if (hasBreakend()) {
if (!getBreakend()
.equals(other.getBreakend())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CISTARTLEFT_FIELD_NUMBER;
hash = (53 * hash) + getCiStartLeft();
hash = (37 * hash) + CISTARTRIGHT_FIELD_NUMBER;
hash = (53 * hash) + getCiStartRight();
hash = (37 * hash) + CIENDLEFT_FIELD_NUMBER;
hash = (53 * hash) + getCiEndLeft();
hash = (37 * hash) + CIENDRIGHT_FIELD_NUMBER;
hash = (53 * hash) + getCiEndRight();
hash = (37 * hash) + COPYNUMBER_FIELD_NUMBER;
hash = (53 * hash) + getCopyNumber();
hash = (37 * hash) + LEFTSVINSSEQ_FIELD_NUMBER;
hash = (53 * hash) + getLeftSvInsSeq().hashCode();
hash = (37 * hash) + RIGHTSVINSSEQ_FIELD_NUMBER;
hash = (53 * hash) + getRightSvInsSeq().hashCode();
if (hasBreakend()) {
hash = (37 * hash) + BREAKEND_FIELD_NUMBER;
hash = (53 * hash) + getBreakend().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.StructuralVariation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.StructuralVariation)
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StructuralVariation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StructuralVariation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.class, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
ciStartLeft_ = 0;
ciStartRight_ = 0;
ciEndLeft_ = 0;
ciEndRight_ = 0;
copyNumber_ = 0;
leftSvInsSeq_ = "";
rightSvInsSeq_ = "";
if (breakendBuilder_ == null) {
breakend_ = null;
} else {
breakend_ = null;
breakendBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_StructuralVariation_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation result = new org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation(this);
result.ciStartLeft_ = ciStartLeft_;
result.ciStartRight_ = ciStartRight_;
result.ciEndLeft_ = ciEndLeft_;
result.ciEndRight_ = ciEndRight_;
result.copyNumber_ = copyNumber_;
result.leftSvInsSeq_ = leftSvInsSeq_;
result.rightSvInsSeq_ = rightSvInsSeq_;
if (breakendBuilder_ == null) {
result.breakend_ = breakend_;
} else {
result.breakend_ = breakendBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.getDefaultInstance()) return this;
if (other.getCiStartLeft() != 0) {
setCiStartLeft(other.getCiStartLeft());
}
if (other.getCiStartRight() != 0) {
setCiStartRight(other.getCiStartRight());
}
if (other.getCiEndLeft() != 0) {
setCiEndLeft(other.getCiEndLeft());
}
if (other.getCiEndRight() != 0) {
setCiEndRight(other.getCiEndRight());
}
if (other.getCopyNumber() != 0) {
setCopyNumber(other.getCopyNumber());
}
if (!other.getLeftSvInsSeq().isEmpty()) {
leftSvInsSeq_ = other.leftSvInsSeq_;
onChanged();
}
if (!other.getRightSvInsSeq().isEmpty()) {
rightSvInsSeq_ = other.rightSvInsSeq_;
onChanged();
}
if (other.hasBreakend()) {
mergeBreakend(other.getBreakend());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int ciStartLeft_ ;
/**
* int32 ciStartLeft = 1;
* @return The ciStartLeft.
*/
public int getCiStartLeft() {
return ciStartLeft_;
}
/**
* int32 ciStartLeft = 1;
* @param value The ciStartLeft to set.
* @return This builder for chaining.
*/
public Builder setCiStartLeft(int value) {
ciStartLeft_ = value;
onChanged();
return this;
}
/**
* int32 ciStartLeft = 1;
* @return This builder for chaining.
*/
public Builder clearCiStartLeft() {
ciStartLeft_ = 0;
onChanged();
return this;
}
private int ciStartRight_ ;
/**
* int32 ciStartRight = 2;
* @return The ciStartRight.
*/
public int getCiStartRight() {
return ciStartRight_;
}
/**
* int32 ciStartRight = 2;
* @param value The ciStartRight to set.
* @return This builder for chaining.
*/
public Builder setCiStartRight(int value) {
ciStartRight_ = value;
onChanged();
return this;
}
/**
* int32 ciStartRight = 2;
* @return This builder for chaining.
*/
public Builder clearCiStartRight() {
ciStartRight_ = 0;
onChanged();
return this;
}
private int ciEndLeft_ ;
/**
* int32 ciEndLeft = 3;
* @return The ciEndLeft.
*/
public int getCiEndLeft() {
return ciEndLeft_;
}
/**
* int32 ciEndLeft = 3;
* @param value The ciEndLeft to set.
* @return This builder for chaining.
*/
public Builder setCiEndLeft(int value) {
ciEndLeft_ = value;
onChanged();
return this;
}
/**
* int32 ciEndLeft = 3;
* @return This builder for chaining.
*/
public Builder clearCiEndLeft() {
ciEndLeft_ = 0;
onChanged();
return this;
}
private int ciEndRight_ ;
/**
* int32 ciEndRight = 4;
* @return The ciEndRight.
*/
public int getCiEndRight() {
return ciEndRight_;
}
/**
* int32 ciEndRight = 4;
* @param value The ciEndRight to set.
* @return This builder for chaining.
*/
public Builder setCiEndRight(int value) {
ciEndRight_ = value;
onChanged();
return this;
}
/**
* int32 ciEndRight = 4;
* @return This builder for chaining.
*/
public Builder clearCiEndRight() {
ciEndRight_ = 0;
onChanged();
return this;
}
private int copyNumber_ ;
/**
*
**
* Number of copies for CNV variants.
*
*
* int32 copyNumber = 5;
* @return The copyNumber.
*/
public int getCopyNumber() {
return copyNumber_;
}
/**
*
**
* Number of copies for CNV variants.
*
*
* int32 copyNumber = 5;
* @param value The copyNumber to set.
* @return This builder for chaining.
*/
public Builder setCopyNumber(int value) {
copyNumber_ = value;
onChanged();
return this;
}
/**
*
**
* Number of copies for CNV variants.
*
*
* int32 copyNumber = 5;
* @return This builder for chaining.
*/
public Builder clearCopyNumber() {
copyNumber_ = 0;
onChanged();
return this;
}
private java.lang.Object leftSvInsSeq_ = "";
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return The leftSvInsSeq.
*/
public java.lang.String getLeftSvInsSeq() {
java.lang.Object ref = leftSvInsSeq_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
leftSvInsSeq_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return The bytes for leftSvInsSeq.
*/
public com.google.protobuf.ByteString
getLeftSvInsSeqBytes() {
java.lang.Object ref = leftSvInsSeq_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
leftSvInsSeq_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @param value The leftSvInsSeq to set.
* @return This builder for chaining.
*/
public Builder setLeftSvInsSeq(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
leftSvInsSeq_ = value;
onChanged();
return this;
}
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @return This builder for chaining.
*/
public Builder clearLeftSvInsSeq() {
leftSvInsSeq_ = getDefaultInstance().getLeftSvInsSeq();
onChanged();
return this;
}
/**
*
**
* Inserted sequence for long INS
*
*
* string leftSvInsSeq = 6;
* @param value The bytes for leftSvInsSeq to set.
* @return This builder for chaining.
*/
public Builder setLeftSvInsSeqBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
leftSvInsSeq_ = value;
onChanged();
return this;
}
private java.lang.Object rightSvInsSeq_ = "";
/**
* string rightSvInsSeq = 7;
* @return The rightSvInsSeq.
*/
public java.lang.String getRightSvInsSeq() {
java.lang.Object ref = rightSvInsSeq_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rightSvInsSeq_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string rightSvInsSeq = 7;
* @return The bytes for rightSvInsSeq.
*/
public com.google.protobuf.ByteString
getRightSvInsSeqBytes() {
java.lang.Object ref = rightSvInsSeq_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rightSvInsSeq_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string rightSvInsSeq = 7;
* @param value The rightSvInsSeq to set.
* @return This builder for chaining.
*/
public Builder setRightSvInsSeq(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
rightSvInsSeq_ = value;
onChanged();
return this;
}
/**
* string rightSvInsSeq = 7;
* @return This builder for chaining.
*/
public Builder clearRightSvInsSeq() {
rightSvInsSeq_ = getDefaultInstance().getRightSvInsSeq();
onChanged();
return this;
}
/**
* string rightSvInsSeq = 7;
* @param value The bytes for rightSvInsSeq to set.
* @return This builder for chaining.
*/
public Builder setRightSvInsSeqBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
rightSvInsSeq_ = value;
onChanged();
return this;
}
private org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend breakend_;
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend, org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder> breakendBuilder_;
/**
* .protobuf.opencb.Breakend breakend = 9;
* @return Whether the breakend field is set.
*/
public boolean hasBreakend() {
return breakendBuilder_ != null || breakend_ != null;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
* @return The breakend.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend getBreakend() {
if (breakendBuilder_ == null) {
return breakend_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.getDefaultInstance() : breakend_;
} else {
return breakendBuilder_.getMessage();
}
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public Builder setBreakend(org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend value) {
if (breakendBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
breakend_ = value;
onChanged();
} else {
breakendBuilder_.setMessage(value);
}
return this;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public Builder setBreakend(
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder builderForValue) {
if (breakendBuilder_ == null) {
breakend_ = builderForValue.build();
onChanged();
} else {
breakendBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public Builder mergeBreakend(org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend value) {
if (breakendBuilder_ == null) {
if (breakend_ != null) {
breakend_ =
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.newBuilder(breakend_).mergeFrom(value).buildPartial();
} else {
breakend_ = value;
}
onChanged();
} else {
breakendBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public Builder clearBreakend() {
if (breakendBuilder_ == null) {
breakend_ = null;
onChanged();
} else {
breakend_ = null;
breakendBuilder_ = null;
}
return this;
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder getBreakendBuilder() {
onChanged();
return getBreakendFieldBuilder().getBuilder();
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder getBreakendOrBuilder() {
if (breakendBuilder_ != null) {
return breakendBuilder_.getMessageOrBuilder();
} else {
return breakend_ == null ?
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.getDefaultInstance() : breakend_;
}
}
/**
* .protobuf.opencb.Breakend breakend = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend, org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder>
getBreakendFieldBuilder() {
if (breakendBuilder_ == null) {
breakendBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend, org.opencb.biodata.models.variant.protobuf.VariantProto.Breakend.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.BreakendOrBuilder>(
getBreakend(),
getParentForChildren(),
isClean());
breakend_ = null;
}
return breakendBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.StructuralVariation)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.StructuralVariation)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StructuralVariation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StructuralVariation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VariantOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.Variant)
com.google.protobuf.MessageOrBuilder {
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return The chromosome.
*/
java.lang.String getChromosome();
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return The bytes for chromosome.
*/
com.google.protobuf.ByteString
getChromosomeBytes();
/**
*
**
* Normalized position where the genomic variation starts.
* <ul>
* <li>SNVs have the same start and end position</li>
* <li>Insertions start in the last present position: if the first nucleotide
* is inserted in position 6, the start is position 5</li>
* <li>Deletions start in the first previously present position: if the first
* deleted nucleotide is in position 6, the start is position 6</li>
* </ul>
*
*
* int32 start = 2;
* @return The start.
*/
int getStart();
/**
*
**
* Normalized position where the genomic variation ends.
* <ul>
* <li>SNVs have the same start and end positions</li>
* <li>Insertions end in the first present position: if the last nucleotide
* is inserted in position 9, the end is position 10</li>
* <li>Deletions ends in the last previously present position: if the last
* deleted nucleotide is in position 9, the end is position 9</li>
* </ul>
*
*
* int32 end = 3;
* @return The end.
*/
int getEnd();
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The reference.
*/
java.lang.String getReference();
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The bytes for reference.
*/
com.google.protobuf.ByteString
getReferenceBytes();
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The alternate.
*/
java.lang.String getAlternate();
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The bytes for alternate.
*/
com.google.protobuf.ByteString
getAlternateBytes();
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return The strand.
*/
java.lang.String getStrand();
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return The bytes for strand.
*/
com.google.protobuf.ByteString
getStrandBytes();
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
* @return Whether the sv field is set.
*/
boolean hasSv();
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
* @return The sv.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation getSv();
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder getSvOrBuilder();
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return The id.
*/
java.lang.String getId();
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return A list containing the names.
*/
java.util.List
getNamesList();
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return The count of names.
*/
int getNamesCount();
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index of the element to return.
* @return The names at the given index.
*/
java.lang.String getNames(int index);
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index of the value to return.
* @return The bytes of the names at the given index.
*/
com.google.protobuf.ByteString
getNamesBytes(int index);
/**
*
**
* Length of the genomic variation, which depends on the variation type.
* <ul>
* <li>SNVs have a length of 1 nucleotide</li>
* <li>Indels have the length of the largest allele</li>
* </ul>
*
*
* int32 length = 8;
* @return The length.
*/
int getLength();
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return The type.
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType getType();
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
java.util.List
getStudiesList();
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry getStudies(int index);
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
int getStudiesCount();
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder>
getStudiesOrBuilderList();
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder getStudiesOrBuilder(
int index);
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
* @return Whether the annotation field is set.
*/
boolean hasAnnotation();
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
* @return The annotation.
*/
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation getAnnotation();
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotationOrBuilder getAnnotationOrBuilder();
}
/**
* Protobuf type {@code protobuf.opencb.Variant}
*/
public static final class Variant extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.Variant)
VariantOrBuilder {
private static final long serialVersionUID = 0L;
// Use Variant.newBuilder() to construct.
private Variant(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Variant() {
chromosome_ = "";
reference_ = "";
alternate_ = "";
strand_ = "";
id_ = "";
names_ = com.google.protobuf.LazyStringArrayList.EMPTY;
type_ = 0;
studies_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Variant();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Variant(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
chromosome_ = s;
break;
}
case 16: {
start_ = input.readInt32();
break;
}
case 24: {
end_ = input.readInt32();
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
reference_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
alternate_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
strand_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
names_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
names_.add(s);
break;
}
case 64: {
length_ = input.readInt32();
break;
}
case 72: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
studies_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
studies_.add(
input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.parser(), extensionRegistry));
break;
}
case 98: {
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.Builder subBuilder = null;
if (annotation_ != null) {
subBuilder = annotation_.toBuilder();
}
annotation_ = input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(annotation_);
annotation_ = subBuilder.buildPartial();
}
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 114: {
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder subBuilder = null;
if (sv_ != null) {
subBuilder = sv_.toBuilder();
}
sv_ = input.readMessage(org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(sv_);
sv_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
names_ = names_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
studies_ = java.util.Collections.unmodifiableList(studies_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Variant_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Variant_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.class, org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.Builder.class);
}
public static final int CHROMOSOME_FIELD_NUMBER = 1;
private volatile java.lang.Object chromosome_;
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return The chromosome.
*/
public java.lang.String getChromosome() {
java.lang.Object ref = chromosome_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
chromosome_ = s;
return s;
}
}
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return The bytes for chromosome.
*/
public com.google.protobuf.ByteString
getChromosomeBytes() {
java.lang.Object ref = chromosome_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
chromosome_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int START_FIELD_NUMBER = 2;
private int start_;
/**
*
**
* Normalized position where the genomic variation starts.
* <ul>
* <li>SNVs have the same start and end position</li>
* <li>Insertions start in the last present position: if the first nucleotide
* is inserted in position 6, the start is position 5</li>
* <li>Deletions start in the first previously present position: if the first
* deleted nucleotide is in position 6, the start is position 6</li>
* </ul>
*
*
* int32 start = 2;
* @return The start.
*/
public int getStart() {
return start_;
}
public static final int END_FIELD_NUMBER = 3;
private int end_;
/**
*
**
* Normalized position where the genomic variation ends.
* <ul>
* <li>SNVs have the same start and end positions</li>
* <li>Insertions end in the first present position: if the last nucleotide
* is inserted in position 9, the end is position 10</li>
* <li>Deletions ends in the last previously present position: if the last
* deleted nucleotide is in position 9, the end is position 9</li>
* </ul>
*
*
* int32 end = 3;
* @return The end.
*/
public int getEnd() {
return end_;
}
public static final int REFERENCE_FIELD_NUMBER = 4;
private volatile java.lang.Object reference_;
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The reference.
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
}
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The bytes for reference.
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ALTERNATE_FIELD_NUMBER = 5;
private volatile java.lang.Object alternate_;
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The alternate.
*/
public java.lang.String getAlternate() {
java.lang.Object ref = alternate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alternate_ = s;
return s;
}
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The bytes for alternate.
*/
public com.google.protobuf.ByteString
getAlternateBytes() {
java.lang.Object ref = alternate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alternate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STRAND_FIELD_NUMBER = 6;
private volatile java.lang.Object strand_;
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return The strand.
*/
public java.lang.String getStrand() {
java.lang.Object ref = strand_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
strand_ = s;
return s;
}
}
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return The bytes for strand.
*/
public com.google.protobuf.ByteString
getStrandBytes() {
java.lang.Object ref = strand_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
strand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SV_FIELD_NUMBER = 14;
private org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation sv_;
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
* @return Whether the sv field is set.
*/
public boolean hasSv() {
return sv_ != null;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
* @return The sv.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation getSv() {
return sv_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.getDefaultInstance() : sv_;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder getSvOrBuilder() {
return getSv();
}
public static final int ID_FIELD_NUMBER = 13;
private volatile java.lang.Object id_;
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAMES_FIELD_NUMBER = 7;
private com.google.protobuf.LazyStringList names_;
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return A list containing the names.
*/
public com.google.protobuf.ProtocolStringList
getNamesList() {
return names_;
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return The count of names.
*/
public int getNamesCount() {
return names_.size();
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index of the element to return.
* @return The names at the given index.
*/
public java.lang.String getNames(int index) {
return names_.get(index);
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index of the value to return.
* @return The bytes of the names at the given index.
*/
public com.google.protobuf.ByteString
getNamesBytes(int index) {
return names_.getByteString(index);
}
public static final int LENGTH_FIELD_NUMBER = 8;
private int length_;
/**
*
**
* Length of the genomic variation, which depends on the variation type.
* <ul>
* <li>SNVs have a length of 1 nucleotide</li>
* <li>Indels have the length of the largest allele</li>
* </ul>
*
*
* int32 length = 8;
* @return The length.
*/
public int getLength() {
return length_;
}
public static final int TYPE_FIELD_NUMBER = 9;
private int type_;
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return The enum numeric value on the wire for type.
*/
public int getTypeValue() {
return type_;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return The type.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType getType() {
@SuppressWarnings("deprecation")
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType result = org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.valueOf(type_);
return result == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.UNRECOGNIZED : result;
}
public static final int STUDIES_FIELD_NUMBER = 11;
private java.util.List studies_;
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public java.util.List getStudiesList() {
return studies_;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder>
getStudiesOrBuilderList() {
return studies_;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public int getStudiesCount() {
return studies_.size();
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry getStudies(int index) {
return studies_.get(index);
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder getStudiesOrBuilder(
int index) {
return studies_.get(index);
}
public static final int ANNOTATION_FIELD_NUMBER = 12;
private org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation annotation_;
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
* @return Whether the annotation field is set.
*/
public boolean hasAnnotation() {
return annotation_ != null;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
* @return The annotation.
*/
public org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation getAnnotation() {
return annotation_ == null ? org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.getDefaultInstance() : annotation_;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotationOrBuilder getAnnotationOrBuilder() {
return getAnnotation();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getChromosomeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, chromosome_);
}
if (start_ != 0) {
output.writeInt32(2, start_);
}
if (end_ != 0) {
output.writeInt32(3, end_);
}
if (!getReferenceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, reference_);
}
if (!getAlternateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, alternate_);
}
if (!getStrandBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, strand_);
}
for (int i = 0; i < names_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, names_.getRaw(i));
}
if (length_ != 0) {
output.writeInt32(8, length_);
}
if (type_ != org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.NO_VARIATION.getNumber()) {
output.writeEnum(9, type_);
}
for (int i = 0; i < studies_.size(); i++) {
output.writeMessage(11, studies_.get(i));
}
if (annotation_ != null) {
output.writeMessage(12, getAnnotation());
}
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, id_);
}
if (sv_ != null) {
output.writeMessage(14, getSv());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getChromosomeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, chromosome_);
}
if (start_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, start_);
}
if (end_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, end_);
}
if (!getReferenceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, reference_);
}
if (!getAlternateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, alternate_);
}
if (!getStrandBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, strand_);
}
{
int dataSize = 0;
for (int i = 0; i < names_.size(); i++) {
dataSize += computeStringSizeNoTag(names_.getRaw(i));
}
size += dataSize;
size += 1 * getNamesList().size();
}
if (length_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, length_);
}
if (type_ != org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.NO_VARIATION.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, type_);
}
for (int i = 0; i < studies_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, studies_.get(i));
}
if (annotation_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getAnnotation());
}
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, id_);
}
if (sv_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getSv());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.Variant)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.Variant other = (org.opencb.biodata.models.variant.protobuf.VariantProto.Variant) obj;
if (!getChromosome()
.equals(other.getChromosome())) return false;
if (getStart()
!= other.getStart()) return false;
if (getEnd()
!= other.getEnd()) return false;
if (!getReference()
.equals(other.getReference())) return false;
if (!getAlternate()
.equals(other.getAlternate())) return false;
if (!getStrand()
.equals(other.getStrand())) return false;
if (hasSv() != other.hasSv()) return false;
if (hasSv()) {
if (!getSv()
.equals(other.getSv())) return false;
}
if (!getId()
.equals(other.getId())) return false;
if (!getNamesList()
.equals(other.getNamesList())) return false;
if (getLength()
!= other.getLength()) return false;
if (type_ != other.type_) return false;
if (!getStudiesList()
.equals(other.getStudiesList())) return false;
if (hasAnnotation() != other.hasAnnotation()) return false;
if (hasAnnotation()) {
if (!getAnnotation()
.equals(other.getAnnotation())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CHROMOSOME_FIELD_NUMBER;
hash = (53 * hash) + getChromosome().hashCode();
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart();
hash = (37 * hash) + END_FIELD_NUMBER;
hash = (53 * hash) + getEnd();
hash = (37 * hash) + REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getReference().hashCode();
hash = (37 * hash) + ALTERNATE_FIELD_NUMBER;
hash = (53 * hash) + getAlternate().hashCode();
hash = (37 * hash) + STRAND_FIELD_NUMBER;
hash = (53 * hash) + getStrand().hashCode();
if (hasSv()) {
hash = (37 * hash) + SV_FIELD_NUMBER;
hash = (53 * hash) + getSv().hashCode();
}
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
if (getNamesCount() > 0) {
hash = (37 * hash) + NAMES_FIELD_NUMBER;
hash = (53 * hash) + getNamesList().hashCode();
}
hash = (37 * hash) + LENGTH_FIELD_NUMBER;
hash = (53 * hash) + getLength();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (getStudiesCount() > 0) {
hash = (37 * hash) + STUDIES_FIELD_NUMBER;
hash = (53 * hash) + getStudiesList().hashCode();
}
if (hasAnnotation()) {
hash = (37 * hash) + ANNOTATION_FIELD_NUMBER;
hash = (53 * hash) + getAnnotation().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.Variant prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.Variant}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.Variant)
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Variant_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Variant_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.class, org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStudiesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
chromosome_ = "";
start_ = 0;
end_ = 0;
reference_ = "";
alternate_ = "";
strand_ = "";
if (svBuilder_ == null) {
sv_ = null;
} else {
sv_ = null;
svBuilder_ = null;
}
id_ = "";
names_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
length_ = 0;
type_ = 0;
if (studiesBuilder_ == null) {
studies_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
studiesBuilder_.clear();
}
if (annotationBuilder_ == null) {
annotation_ = null;
} else {
annotation_ = null;
annotationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_Variant_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Variant getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Variant build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.Variant result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Variant buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.Variant result = new org.opencb.biodata.models.variant.protobuf.VariantProto.Variant(this);
int from_bitField0_ = bitField0_;
result.chromosome_ = chromosome_;
result.start_ = start_;
result.end_ = end_;
result.reference_ = reference_;
result.alternate_ = alternate_;
result.strand_ = strand_;
if (svBuilder_ == null) {
result.sv_ = sv_;
} else {
result.sv_ = svBuilder_.build();
}
result.id_ = id_;
if (((bitField0_ & 0x00000001) != 0)) {
names_ = names_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.names_ = names_;
result.length_ = length_;
result.type_ = type_;
if (studiesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
studies_ = java.util.Collections.unmodifiableList(studies_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.studies_ = studies_;
} else {
result.studies_ = studiesBuilder_.build();
}
if (annotationBuilder_ == null) {
result.annotation_ = annotation_;
} else {
result.annotation_ = annotationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.Variant) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.Variant)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.Variant other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.Variant.getDefaultInstance()) return this;
if (!other.getChromosome().isEmpty()) {
chromosome_ = other.chromosome_;
onChanged();
}
if (other.getStart() != 0) {
setStart(other.getStart());
}
if (other.getEnd() != 0) {
setEnd(other.getEnd());
}
if (!other.getReference().isEmpty()) {
reference_ = other.reference_;
onChanged();
}
if (!other.getAlternate().isEmpty()) {
alternate_ = other.alternate_;
onChanged();
}
if (!other.getStrand().isEmpty()) {
strand_ = other.strand_;
onChanged();
}
if (other.hasSv()) {
mergeSv(other.getSv());
}
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.names_.isEmpty()) {
if (names_.isEmpty()) {
names_ = other.names_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNamesIsMutable();
names_.addAll(other.names_);
}
onChanged();
}
if (other.getLength() != 0) {
setLength(other.getLength());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (studiesBuilder_ == null) {
if (!other.studies_.isEmpty()) {
if (studies_.isEmpty()) {
studies_ = other.studies_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureStudiesIsMutable();
studies_.addAll(other.studies_);
}
onChanged();
}
} else {
if (!other.studies_.isEmpty()) {
if (studiesBuilder_.isEmpty()) {
studiesBuilder_.dispose();
studiesBuilder_ = null;
studies_ = other.studies_;
bitField0_ = (bitField0_ & ~0x00000002);
studiesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStudiesFieldBuilder() : null;
} else {
studiesBuilder_.addAllMessages(other.studies_);
}
}
}
if (other.hasAnnotation()) {
mergeAnnotation(other.getAnnotation());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.Variant parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.Variant) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object chromosome_ = "";
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return The chromosome.
*/
public java.lang.String getChromosome() {
java.lang.Object ref = chromosome_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
chromosome_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return The bytes for chromosome.
*/
public com.google.protobuf.ByteString
getChromosomeBytes() {
java.lang.Object ref = chromosome_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
chromosome_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @param value The chromosome to set.
* @return This builder for chaining.
*/
public Builder setChromosome(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
chromosome_ = value;
onChanged();
return this;
}
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @return This builder for chaining.
*/
public Builder clearChromosome() {
chromosome_ = getDefaultInstance().getChromosome();
onChanged();
return this;
}
/**
*
**
* Chromosome where the genomic variation occurred.
*
*
* string chromosome = 1;
* @param value The bytes for chromosome to set.
* @return This builder for chaining.
*/
public Builder setChromosomeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
chromosome_ = value;
onChanged();
return this;
}
private int start_ ;
/**
*
**
* Normalized position where the genomic variation starts.
* <ul>
* <li>SNVs have the same start and end position</li>
* <li>Insertions start in the last present position: if the first nucleotide
* is inserted in position 6, the start is position 5</li>
* <li>Deletions start in the first previously present position: if the first
* deleted nucleotide is in position 6, the start is position 6</li>
* </ul>
*
*
* int32 start = 2;
* @return The start.
*/
public int getStart() {
return start_;
}
/**
*
**
* Normalized position where the genomic variation starts.
* <ul>
* <li>SNVs have the same start and end position</li>
* <li>Insertions start in the last present position: if the first nucleotide
* is inserted in position 6, the start is position 5</li>
* <li>Deletions start in the first previously present position: if the first
* deleted nucleotide is in position 6, the start is position 6</li>
* </ul>
*
*
* int32 start = 2;
* @param value The start to set.
* @return This builder for chaining.
*/
public Builder setStart(int value) {
start_ = value;
onChanged();
return this;
}
/**
*
**
* Normalized position where the genomic variation starts.
* <ul>
* <li>SNVs have the same start and end position</li>
* <li>Insertions start in the last present position: if the first nucleotide
* is inserted in position 6, the start is position 5</li>
* <li>Deletions start in the first previously present position: if the first
* deleted nucleotide is in position 6, the start is position 6</li>
* </ul>
*
*
* int32 start = 2;
* @return This builder for chaining.
*/
public Builder clearStart() {
start_ = 0;
onChanged();
return this;
}
private int end_ ;
/**
*
**
* Normalized position where the genomic variation ends.
* <ul>
* <li>SNVs have the same start and end positions</li>
* <li>Insertions end in the first present position: if the last nucleotide
* is inserted in position 9, the end is position 10</li>
* <li>Deletions ends in the last previously present position: if the last
* deleted nucleotide is in position 9, the end is position 9</li>
* </ul>
*
*
* int32 end = 3;
* @return The end.
*/
public int getEnd() {
return end_;
}
/**
*
**
* Normalized position where the genomic variation ends.
* <ul>
* <li>SNVs have the same start and end positions</li>
* <li>Insertions end in the first present position: if the last nucleotide
* is inserted in position 9, the end is position 10</li>
* <li>Deletions ends in the last previously present position: if the last
* deleted nucleotide is in position 9, the end is position 9</li>
* </ul>
*
*
* int32 end = 3;
* @param value The end to set.
* @return This builder for chaining.
*/
public Builder setEnd(int value) {
end_ = value;
onChanged();
return this;
}
/**
*
**
* Normalized position where the genomic variation ends.
* <ul>
* <li>SNVs have the same start and end positions</li>
* <li>Insertions end in the first present position: if the last nucleotide
* is inserted in position 9, the end is position 10</li>
* <li>Deletions ends in the last previously present position: if the last
* deleted nucleotide is in position 9, the end is position 9</li>
* </ul>
*
*
* int32 end = 3;
* @return This builder for chaining.
*/
public Builder clearEnd() {
end_ = 0;
onChanged();
return this;
}
private java.lang.Object reference_ = "";
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The reference.
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return The bytes for reference.
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @param value The reference to set.
* @return This builder for chaining.
*/
public Builder setReference(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
reference_ = value;
onChanged();
return this;
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @return This builder for chaining.
*/
public Builder clearReference() {
reference_ = getDefaultInstance().getReference();
onChanged();
return this;
}
/**
*
**
* Reference allele.
*
*
* string reference = 4;
* @param value The bytes for reference to set.
* @return This builder for chaining.
*/
public Builder setReferenceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
reference_ = value;
onChanged();
return this;
}
private java.lang.Object alternate_ = "";
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The alternate.
*/
public java.lang.String getAlternate() {
java.lang.Object ref = alternate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alternate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return The bytes for alternate.
*/
public com.google.protobuf.ByteString
getAlternateBytes() {
java.lang.Object ref = alternate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alternate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @param value The alternate to set.
* @return This builder for chaining.
*/
public Builder setAlternate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
alternate_ = value;
onChanged();
return this;
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @return This builder for chaining.
*/
public Builder clearAlternate() {
alternate_ = getDefaultInstance().getAlternate();
onChanged();
return this;
}
/**
*
**
* Alternate allele.
*
*
* string alternate = 5;
* @param value The bytes for alternate to set.
* @return This builder for chaining.
*/
public Builder setAlternateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
alternate_ = value;
onChanged();
return this;
}
private java.lang.Object strand_ = "";
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return The strand.
*/
public java.lang.String getStrand() {
java.lang.Object ref = strand_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
strand_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return The bytes for strand.
*/
public com.google.protobuf.ByteString
getStrandBytes() {
java.lang.Object ref = strand_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
strand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @param value The strand to set.
* @return This builder for chaining.
*/
public Builder setStrand(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
strand_ = value;
onChanged();
return this;
}
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @return This builder for chaining.
*/
public Builder clearStrand() {
strand_ = getDefaultInstance().getStrand();
onChanged();
return this;
}
/**
*
**
* Reference strand for this variant
*
*
* string strand = 6;
* @param value The bytes for strand to set.
* @return This builder for chaining.
*/
public Builder setStrandBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
strand_ = value;
onChanged();
return this;
}
private org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation sv_;
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder> svBuilder_;
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
* @return Whether the sv field is set.
*/
public boolean hasSv() {
return svBuilder_ != null || sv_ != null;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
* @return The sv.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation getSv() {
if (svBuilder_ == null) {
return sv_ == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.getDefaultInstance() : sv_;
} else {
return svBuilder_.getMessage();
}
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public Builder setSv(org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation value) {
if (svBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sv_ = value;
onChanged();
} else {
svBuilder_.setMessage(value);
}
return this;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public Builder setSv(
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder builderForValue) {
if (svBuilder_ == null) {
sv_ = builderForValue.build();
onChanged();
} else {
svBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public Builder mergeSv(org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation value) {
if (svBuilder_ == null) {
if (sv_ != null) {
sv_ =
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.newBuilder(sv_).mergeFrom(value).buildPartial();
} else {
sv_ = value;
}
onChanged();
} else {
svBuilder_.mergeFrom(value);
}
return this;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public Builder clearSv() {
if (svBuilder_ == null) {
sv_ = null;
onChanged();
} else {
sv_ = null;
svBuilder_ = null;
}
return this;
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder getSvBuilder() {
onChanged();
return getSvFieldBuilder().getBuilder();
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder getSvOrBuilder() {
if (svBuilder_ != null) {
return svBuilder_.getMessageOrBuilder();
} else {
return sv_ == null ?
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.getDefaultInstance() : sv_;
}
}
/**
*
**
* Information regarding Structural Variants
*
*
* .protobuf.opencb.StructuralVariation sv = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder>
getSvFieldBuilder() {
if (svBuilder_ == null) {
svBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariation.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.StructuralVariationOrBuilder>(
getSv(),
getParentForChildren(),
isClean());
sv_ = null;
}
return svBuilder_;
}
private java.lang.Object id_ = "";
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
**
* The variant ID.
*
*
* string id = 13;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList names_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureNamesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
names_ = new com.google.protobuf.LazyStringArrayList(names_);
bitField0_ |= 0x00000001;
}
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return A list containing the names.
*/
public com.google.protobuf.ProtocolStringList
getNamesList() {
return names_.getUnmodifiableView();
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return The count of names.
*/
public int getNamesCount() {
return names_.size();
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index of the element to return.
* @return The names at the given index.
*/
public java.lang.String getNames(int index) {
return names_.get(index);
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index of the value to return.
* @return The bytes of the names at the given index.
*/
public com.google.protobuf.ByteString
getNamesBytes(int index) {
return names_.getByteString(index);
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param index The index to set the value at.
* @param value The names to set.
* @return This builder for chaining.
*/
public Builder setNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureNamesIsMutable();
names_.set(index, value);
onChanged();
return this;
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param value The names to add.
* @return This builder for chaining.
*/
public Builder addNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureNamesIsMutable();
names_.add(value);
onChanged();
return this;
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param values The names to add.
* @return This builder for chaining.
*/
public Builder addAllNames(
java.lang.Iterable values) {
ensureNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, names_);
onChanged();
return this;
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @return This builder for chaining.
*/
public Builder clearNames() {
names_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
**
* Other names used for this genomic variation.
*
*
* repeated string names = 7;
* @param value The bytes of the names to add.
* @return This builder for chaining.
*/
public Builder addNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureNamesIsMutable();
names_.add(value);
onChanged();
return this;
}
private int length_ ;
/**
*
**
* Length of the genomic variation, which depends on the variation type.
* <ul>
* <li>SNVs have a length of 1 nucleotide</li>
* <li>Indels have the length of the largest allele</li>
* </ul>
*
*
* int32 length = 8;
* @return The length.
*/
public int getLength() {
return length_;
}
/**
*
**
* Length of the genomic variation, which depends on the variation type.
* <ul>
* <li>SNVs have a length of 1 nucleotide</li>
* <li>Indels have the length of the largest allele</li>
* </ul>
*
*
* int32 length = 8;
* @param value The length to set.
* @return This builder for chaining.
*/
public Builder setLength(int value) {
length_ = value;
onChanged();
return this;
}
/**
*
**
* Length of the genomic variation, which depends on the variation type.
* <ul>
* <li>SNVs have a length of 1 nucleotide</li>
* <li>Indels have the length of the largest allele</li>
* </ul>
*
*
* int32 length = 8;
* @return This builder for chaining.
*/
public Builder clearLength() {
length_ = 0;
onChanged();
return this;
}
private int type_ = 0;
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return The enum numeric value on the wire for type.
*/
public int getTypeValue() {
return type_;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return The type.
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType getType() {
@SuppressWarnings("deprecation")
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType result = org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.valueOf(type_);
return result == null ? org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType.UNRECOGNIZED : result;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
**
* Type of variation: single nucleotide, indel or structural variation.
*
*
* .protobuf.opencb.VariantType type = 9;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private java.util.List studies_ =
java.util.Collections.emptyList();
private void ensureStudiesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
studies_ = new java.util.ArrayList(studies_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder> studiesBuilder_;
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public java.util.List getStudiesList() {
if (studiesBuilder_ == null) {
return java.util.Collections.unmodifiableList(studies_);
} else {
return studiesBuilder_.getMessageList();
}
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public int getStudiesCount() {
if (studiesBuilder_ == null) {
return studies_.size();
} else {
return studiesBuilder_.getCount();
}
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry getStudies(int index) {
if (studiesBuilder_ == null) {
return studies_.get(index);
} else {
return studiesBuilder_.getMessage(index);
}
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder setStudies(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry value) {
if (studiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStudiesIsMutable();
studies_.set(index, value);
onChanged();
} else {
studiesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder setStudies(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder builderForValue) {
if (studiesBuilder_ == null) {
ensureStudiesIsMutable();
studies_.set(index, builderForValue.build());
onChanged();
} else {
studiesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder addStudies(org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry value) {
if (studiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStudiesIsMutable();
studies_.add(value);
onChanged();
} else {
studiesBuilder_.addMessage(value);
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder addStudies(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry value) {
if (studiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStudiesIsMutable();
studies_.add(index, value);
onChanged();
} else {
studiesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder addStudies(
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder builderForValue) {
if (studiesBuilder_ == null) {
ensureStudiesIsMutable();
studies_.add(builderForValue.build());
onChanged();
} else {
studiesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder addStudies(
int index, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder builderForValue) {
if (studiesBuilder_ == null) {
ensureStudiesIsMutable();
studies_.add(index, builderForValue.build());
onChanged();
} else {
studiesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder addAllStudies(
java.lang.Iterable extends org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry> values) {
if (studiesBuilder_ == null) {
ensureStudiesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, studies_);
onChanged();
} else {
studiesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder clearStudies() {
if (studiesBuilder_ == null) {
studies_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
studiesBuilder_.clear();
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public Builder removeStudies(int index) {
if (studiesBuilder_ == null) {
ensureStudiesIsMutable();
studies_.remove(index);
onChanged();
} else {
studiesBuilder_.remove(index);
}
return this;
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder getStudiesBuilder(
int index) {
return getStudiesFieldBuilder().getBuilder(index);
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder getStudiesOrBuilder(
int index) {
if (studiesBuilder_ == null) {
return studies_.get(index); } else {
return studiesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public java.util.List extends org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder>
getStudiesOrBuilderList() {
if (studiesBuilder_ != null) {
return studiesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(studies_);
}
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder addStudiesBuilder() {
return getStudiesFieldBuilder().addBuilder(
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.getDefaultInstance());
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder addStudiesBuilder(
int index) {
return getStudiesFieldBuilder().addBuilder(
index, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.getDefaultInstance());
}
/**
*
**
* Information specific to each study the variant was read from, such as
* samples or statistics.
*
*
* repeated .protobuf.opencb.StudyEntry studies = 11;
*/
public java.util.List
getStudiesBuilderList() {
return getStudiesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder>
getStudiesFieldBuilder() {
if (studiesBuilder_ == null) {
studiesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntry.Builder, org.opencb.biodata.models.variant.protobuf.VariantProto.StudyEntryOrBuilder>(
studies_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
studies_ = null;
}
return studiesBuilder_;
}
private org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation annotation_;
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation, org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.Builder, org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotationOrBuilder> annotationBuilder_;
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
* @return Whether the annotation field is set.
*/
public boolean hasAnnotation() {
return annotationBuilder_ != null || annotation_ != null;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
* @return The annotation.
*/
public org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation getAnnotation() {
if (annotationBuilder_ == null) {
return annotation_ == null ? org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.getDefaultInstance() : annotation_;
} else {
return annotationBuilder_.getMessage();
}
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public Builder setAnnotation(org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation value) {
if (annotationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
annotation_ = value;
onChanged();
} else {
annotationBuilder_.setMessage(value);
}
return this;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public Builder setAnnotation(
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.Builder builderForValue) {
if (annotationBuilder_ == null) {
annotation_ = builderForValue.build();
onChanged();
} else {
annotationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public Builder mergeAnnotation(org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation value) {
if (annotationBuilder_ == null) {
if (annotation_ != null) {
annotation_ =
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.newBuilder(annotation_).mergeFrom(value).buildPartial();
} else {
annotation_ = value;
}
onChanged();
} else {
annotationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public Builder clearAnnotation() {
if (annotationBuilder_ == null) {
annotation_ = null;
onChanged();
} else {
annotation_ = null;
annotationBuilder_ = null;
}
return this;
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.Builder getAnnotationBuilder() {
onChanged();
return getAnnotationFieldBuilder().getBuilder();
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
public org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotationOrBuilder getAnnotationOrBuilder() {
if (annotationBuilder_ != null) {
return annotationBuilder_.getMessageOrBuilder();
} else {
return annotation_ == null ?
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.getDefaultInstance() : annotation_;
}
}
/**
*
**
* Annotations of the genomic variation.
*
*
* .protobuf.opencb.VariantAnnotation annotation = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation, org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.Builder, org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotationOrBuilder>
getAnnotationFieldBuilder() {
if (annotationBuilder_ == null) {
annotationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation, org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotation.Builder, org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.VariantAnnotationOrBuilder>(
getAnnotation(),
getParentForChildren(),
isClean());
annotation_ = null;
}
return annotationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.Variant)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.Variant)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.Variant DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.Variant();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.Variant getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Variant parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Variant(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.Variant getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VariantFileMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:protobuf.opencb.VariantFileMetadata)
com.google.protobuf.MessageOrBuilder {
/**
* string fileId = 1;
* @return The fileId.
*/
java.lang.String getFileId();
/**
* string fileId = 1;
* @return The bytes for fileId.
*/
com.google.protobuf.ByteString
getFileIdBytes();
/**
* string studyId = 2;
* @return The studyId.
*/
java.lang.String getStudyId();
/**
* string studyId = 2;
* @return The bytes for studyId.
*/
com.google.protobuf.ByteString
getStudyIdBytes();
/**
* string fileName = 3;
* @return The fileName.
*/
java.lang.String getFileName();
/**
* string fileName = 3;
* @return The bytes for fileName.
*/
com.google.protobuf.ByteString
getFileNameBytes();
/**
* string studyName = 4;
* @return The studyName.
*/
java.lang.String getStudyName();
/**
* string studyName = 4;
* @return The bytes for studyName.
*/
com.google.protobuf.ByteString
getStudyNameBytes();
/**
* repeated string samples = 5;
* @return A list containing the samples.
*/
java.util.List
getSamplesList();
/**
* repeated string samples = 5;
* @return The count of samples.
*/
int getSamplesCount();
/**
* repeated string samples = 5;
* @param index The index of the element to return.
* @return The samples at the given index.
*/
java.lang.String getSamples(int index);
/**
* repeated string samples = 5;
* @param index The index of the value to return.
* @return The bytes of the samples at the given index.
*/
com.google.protobuf.ByteString
getSamplesBytes(int index);
/**
* map<string, string> metadata = 6;
*/
int getMetadataCount();
/**
* map<string, string> metadata = 6;
*/
boolean containsMetadata(
java.lang.String key);
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMetadata();
/**
* map<string, string> metadata = 6;
*/
java.util.Map
getMetadataMap();
/**
* map<string, string> metadata = 6;
*/
java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
* map<string, string> metadata = 6;
*/
java.lang.String getMetadataOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code protobuf.opencb.VariantFileMetadata}
*/
public static final class VariantFileMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protobuf.opencb.VariantFileMetadata)
VariantFileMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use VariantFileMetadata.newBuilder() to construct.
private VariantFileMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VariantFileMetadata() {
fileId_ = "";
studyId_ = "";
fileName_ = "";
studyName_ = "";
samples_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new VariantFileMetadata();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VariantFileMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
fileId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
studyId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
fileName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
studyName_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
samples_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
samples_.add(s);
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
metadata_ = com.google.protobuf.MapField.newMapField(
MetadataDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
metadata__ = input.readMessage(
MetadataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
metadata_.getMutableMap().put(
metadata__.getKey(), metadata__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
samples_ = samples_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantFileMetadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantFileMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.class, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.Builder.class);
}
public static final int FILEID_FIELD_NUMBER = 1;
private volatile java.lang.Object fileId_;
/**
* string fileId = 1;
* @return The fileId.
*/
public java.lang.String getFileId() {
java.lang.Object ref = fileId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileId_ = s;
return s;
}
}
/**
* string fileId = 1;
* @return The bytes for fileId.
*/
public com.google.protobuf.ByteString
getFileIdBytes() {
java.lang.Object ref = fileId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STUDYID_FIELD_NUMBER = 2;
private volatile java.lang.Object studyId_;
/**
* string studyId = 2;
* @return The studyId.
*/
public java.lang.String getStudyId() {
java.lang.Object ref = studyId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
studyId_ = s;
return s;
}
}
/**
* string studyId = 2;
* @return The bytes for studyId.
*/
public com.google.protobuf.ByteString
getStudyIdBytes() {
java.lang.Object ref = studyId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
studyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILENAME_FIELD_NUMBER = 3;
private volatile java.lang.Object fileName_;
/**
* string fileName = 3;
* @return The fileName.
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileName_ = s;
return s;
}
}
/**
* string fileName = 3;
* @return The bytes for fileName.
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STUDYNAME_FIELD_NUMBER = 4;
private volatile java.lang.Object studyName_;
/**
* string studyName = 4;
* @return The studyName.
*/
public java.lang.String getStudyName() {
java.lang.Object ref = studyName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
studyName_ = s;
return s;
}
}
/**
* string studyName = 4;
* @return The bytes for studyName.
*/
public com.google.protobuf.ByteString
getStudyNameBytes() {
java.lang.Object ref = studyName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
studyName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SAMPLES_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList samples_;
/**
* repeated string samples = 5;
* @return A list containing the samples.
*/
public com.google.protobuf.ProtocolStringList
getSamplesList() {
return samples_;
}
/**
* repeated string samples = 5;
* @return The count of samples.
*/
public int getSamplesCount() {
return samples_.size();
}
/**
* repeated string samples = 5;
* @param index The index of the element to return.
* @return The samples at the given index.
*/
public java.lang.String getSamples(int index) {
return samples_.get(index);
}
/**
* repeated string samples = 5;
* @param index The index of the value to return.
* @return The bytes of the samples at the given index.
*/
public com.google.protobuf.ByteString
getSamplesBytes(int index) {
return samples_.getByteString(index);
}
public static final int METADATA_FIELD_NUMBER = 6;
private static final class MetadataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantFileMetadata_MetadataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
* map<string, string> metadata = 6;
*/
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
* map<string, string> metadata = 6;
*/
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
* map<string, string> metadata = 6;
*/
public java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> metadata = 6;
*/
public java.lang.String getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getFileIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, fileId_);
}
if (!getStudyIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, studyId_);
}
if (!getFileNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, fileName_);
}
if (!getStudyNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, studyName_);
}
for (int i = 0; i < samples_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, samples_.getRaw(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMetadata(),
MetadataDefaultEntryHolder.defaultEntry,
6);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getFileIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, fileId_);
}
if (!getStudyIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, studyId_);
}
if (!getFileNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, fileName_);
}
if (!getStudyNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, studyName_);
}
{
int dataSize = 0;
for (int i = 0; i < samples_.size(); i++) {
dataSize += computeStringSizeNoTag(samples_.getRaw(i));
}
size += dataSize;
size += 1 * getSamplesList().size();
}
for (java.util.Map.Entry entry
: internalGetMetadata().getMap().entrySet()) {
com.google.protobuf.MapEntry
metadata__ = MetadataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, metadata__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata)) {
return super.equals(obj);
}
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata other = (org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata) obj;
if (!getFileId()
.equals(other.getFileId())) return false;
if (!getStudyId()
.equals(other.getStudyId())) return false;
if (!getFileName()
.equals(other.getFileName())) return false;
if (!getStudyName()
.equals(other.getStudyName())) return false;
if (!getSamplesList()
.equals(other.getSamplesList())) return false;
if (!internalGetMetadata().equals(
other.internalGetMetadata())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FILEID_FIELD_NUMBER;
hash = (53 * hash) + getFileId().hashCode();
hash = (37 * hash) + STUDYID_FIELD_NUMBER;
hash = (53 * hash) + getStudyId().hashCode();
hash = (37 * hash) + FILENAME_FIELD_NUMBER;
hash = (53 * hash) + getFileName().hashCode();
hash = (37 * hash) + STUDYNAME_FIELD_NUMBER;
hash = (53 * hash) + getStudyName().hashCode();
if (getSamplesCount() > 0) {
hash = (37 * hash) + SAMPLES_FIELD_NUMBER;
hash = (53 * hash) + getSamplesList().hashCode();
}
if (!internalGetMetadata().getMap().isEmpty()) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetMetadata().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protobuf.opencb.VariantFileMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protobuf.opencb.VariantFileMetadata)
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantFileMetadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 6:
return internalGetMutableMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantFileMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.class, org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.Builder.class);
}
// Construct using org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
fileId_ = "";
studyId_ = "";
fileName_ = "";
studyName_ = "";
samples_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
internalGetMutableMetadata().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.internal_static_protobuf_opencb_VariantFileMetadata_descriptor;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata getDefaultInstanceForType() {
return org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.getDefaultInstance();
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata build() {
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata buildPartial() {
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata result = new org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata(this);
int from_bitField0_ = bitField0_;
result.fileId_ = fileId_;
result.studyId_ = studyId_;
result.fileName_ = fileName_;
result.studyName_ = studyName_;
if (((bitField0_ & 0x00000001) != 0)) {
samples_ = samples_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.samples_ = samples_;
result.metadata_ = internalGetMetadata();
result.metadata_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata) {
return mergeFrom((org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata other) {
if (other == org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata.getDefaultInstance()) return this;
if (!other.getFileId().isEmpty()) {
fileId_ = other.fileId_;
onChanged();
}
if (!other.getStudyId().isEmpty()) {
studyId_ = other.studyId_;
onChanged();
}
if (!other.getFileName().isEmpty()) {
fileName_ = other.fileName_;
onChanged();
}
if (!other.getStudyName().isEmpty()) {
studyName_ = other.studyName_;
onChanged();
}
if (!other.samples_.isEmpty()) {
if (samples_.isEmpty()) {
samples_ = other.samples_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSamplesIsMutable();
samples_.addAll(other.samples_);
}
onChanged();
}
internalGetMutableMetadata().mergeFrom(
other.internalGetMetadata());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object fileId_ = "";
/**
* string fileId = 1;
* @return The fileId.
*/
public java.lang.String getFileId() {
java.lang.Object ref = fileId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string fileId = 1;
* @return The bytes for fileId.
*/
public com.google.protobuf.ByteString
getFileIdBytes() {
java.lang.Object ref = fileId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string fileId = 1;
* @param value The fileId to set.
* @return This builder for chaining.
*/
public Builder setFileId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fileId_ = value;
onChanged();
return this;
}
/**
* string fileId = 1;
* @return This builder for chaining.
*/
public Builder clearFileId() {
fileId_ = getDefaultInstance().getFileId();
onChanged();
return this;
}
/**
* string fileId = 1;
* @param value The bytes for fileId to set.
* @return This builder for chaining.
*/
public Builder setFileIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fileId_ = value;
onChanged();
return this;
}
private java.lang.Object studyId_ = "";
/**
* string studyId = 2;
* @return The studyId.
*/
public java.lang.String getStudyId() {
java.lang.Object ref = studyId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
studyId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string studyId = 2;
* @return The bytes for studyId.
*/
public com.google.protobuf.ByteString
getStudyIdBytes() {
java.lang.Object ref = studyId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
studyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string studyId = 2;
* @param value The studyId to set.
* @return This builder for chaining.
*/
public Builder setStudyId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
studyId_ = value;
onChanged();
return this;
}
/**
* string studyId = 2;
* @return This builder for chaining.
*/
public Builder clearStudyId() {
studyId_ = getDefaultInstance().getStudyId();
onChanged();
return this;
}
/**
* string studyId = 2;
* @param value The bytes for studyId to set.
* @return This builder for chaining.
*/
public Builder setStudyIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
studyId_ = value;
onChanged();
return this;
}
private java.lang.Object fileName_ = "";
/**
* string fileName = 3;
* @return The fileName.
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string fileName = 3;
* @return The bytes for fileName.
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string fileName = 3;
* @param value The fileName to set.
* @return This builder for chaining.
*/
public Builder setFileName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fileName_ = value;
onChanged();
return this;
}
/**
* string fileName = 3;
* @return This builder for chaining.
*/
public Builder clearFileName() {
fileName_ = getDefaultInstance().getFileName();
onChanged();
return this;
}
/**
* string fileName = 3;
* @param value The bytes for fileName to set.
* @return This builder for chaining.
*/
public Builder setFileNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fileName_ = value;
onChanged();
return this;
}
private java.lang.Object studyName_ = "";
/**
* string studyName = 4;
* @return The studyName.
*/
public java.lang.String getStudyName() {
java.lang.Object ref = studyName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
studyName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string studyName = 4;
* @return The bytes for studyName.
*/
public com.google.protobuf.ByteString
getStudyNameBytes() {
java.lang.Object ref = studyName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
studyName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string studyName = 4;
* @param value The studyName to set.
* @return This builder for chaining.
*/
public Builder setStudyName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
studyName_ = value;
onChanged();
return this;
}
/**
* string studyName = 4;
* @return This builder for chaining.
*/
public Builder clearStudyName() {
studyName_ = getDefaultInstance().getStudyName();
onChanged();
return this;
}
/**
* string studyName = 4;
* @param value The bytes for studyName to set.
* @return This builder for chaining.
*/
public Builder setStudyNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
studyName_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList samples_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSamplesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
samples_ = new com.google.protobuf.LazyStringArrayList(samples_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string samples = 5;
* @return A list containing the samples.
*/
public com.google.protobuf.ProtocolStringList
getSamplesList() {
return samples_.getUnmodifiableView();
}
/**
* repeated string samples = 5;
* @return The count of samples.
*/
public int getSamplesCount() {
return samples_.size();
}
/**
* repeated string samples = 5;
* @param index The index of the element to return.
* @return The samples at the given index.
*/
public java.lang.String getSamples(int index) {
return samples_.get(index);
}
/**
* repeated string samples = 5;
* @param index The index of the value to return.
* @return The bytes of the samples at the given index.
*/
public com.google.protobuf.ByteString
getSamplesBytes(int index) {
return samples_.getByteString(index);
}
/**
* repeated string samples = 5;
* @param index The index to set the value at.
* @param value The samples to set.
* @return This builder for chaining.
*/
public Builder setSamples(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSamplesIsMutable();
samples_.set(index, value);
onChanged();
return this;
}
/**
* repeated string samples = 5;
* @param value The samples to add.
* @return This builder for chaining.
*/
public Builder addSamples(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSamplesIsMutable();
samples_.add(value);
onChanged();
return this;
}
/**
* repeated string samples = 5;
* @param values The samples to add.
* @return This builder for chaining.
*/
public Builder addAllSamples(
java.lang.Iterable values) {
ensureSamplesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, samples_);
onChanged();
return this;
}
/**
* repeated string samples = 5;
* @return This builder for chaining.
*/
public Builder clearSamples() {
samples_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string samples = 5;
* @param value The bytes of the samples to add.
* @return This builder for chaining.
*/
public Builder addSamplesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSamplesIsMutable();
samples_.add(value);
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
private com.google.protobuf.MapField
internalGetMutableMetadata() {
onChanged();;
if (metadata_ == null) {
metadata_ = com.google.protobuf.MapField.newMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
if (!metadata_.isMutable()) {
metadata_ = metadata_.copy();
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
* map<string, string> metadata = 6;
*/
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
* map<string, string> metadata = 6;
*/
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
* map<string, string> metadata = 6;
*/
public java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> metadata = 6;
*/
public java.lang.String getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearMetadata() {
internalGetMutableMetadata().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> metadata = 6;
*/
public Builder removeMetadata(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMetadata().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableMetadata() {
return internalGetMutableMetadata().getMutableMap();
}
/**
* map<string, string> metadata = 6;
*/
public Builder putMetadata(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMetadata().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> metadata = 6;
*/
public Builder putAllMetadata(
java.util.Map values) {
internalGetMutableMetadata().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protobuf.opencb.VariantFileMetadata)
}
// @@protoc_insertion_point(class_scope:protobuf.opencb.VariantFileMetadata)
private static final org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata();
}
public static org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VariantFileMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VariantFileMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.opencb.biodata.models.variant.protobuf.VariantProto.VariantFileMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantStats_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantStats_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantStats_GenotypeCountEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantStats_GenotypeCountEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantStats_GenotypeFreqEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantStats_GenotypeFreqEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantStats_FilterCountEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantStats_FilterCountEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantStats_FilterFreqEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantStats_FilterFreqEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_OriginalCall_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_OriginalCall_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_FileEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_FileEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_FileEntry_DataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_FileEntry_DataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_AlternateCoordinate_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_AlternateCoordinate_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_SampleEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_SampleEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_StudyEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_StudyEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_BreakendMate_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_BreakendMate_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_Breakend_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_Breakend_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_StructuralVariation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_StructuralVariation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_Variant_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_Variant_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantFileMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantFileMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_protobuf_opencb_VariantFileMetadata_MetadataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_protobuf_opencb_VariantFileMetadata_MetadataEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\035protobuf/opencb/variant.proto\022\017protobu" +
"f.opencb\032(protobuf/opencb/variant_annota" +
"tion.proto\"\313\006\n\014VariantStats\022\020\n\010cohortId\030" +
"\021 \001(\t\022\023\n\013sampleCount\030\022 \001(\005\022\021\n\tfileCount\030" +
"\023 \001(\005\022\023\n\013alleleCount\030\001 \001(\005\022\026\n\016refAlleleC" +
"ount\030\002 \001(\005\022\026\n\016altAlleleCount\030\003 \001(\005\022\025\n\rre" +
"fAlleleFreq\030\004 \001(\002\022\025\n\raltAlleleFreq\030\005 \001(\002" +
"\022\032\n\022missingAlleleCount\030\010 \001(\005\022\034\n\024missingG" +
"enotypeCount\030\t \001(\005\022G\n\rgenotypeCount\030\006 \003(" +
"\01320.protobuf.opencb.VariantStats.Genotyp" +
"eCountEntry\022E\n\014genotypeFreq\030\007 \003(\0132/.prot" +
"obuf.opencb.VariantStats.GenotypeFreqEnt" +
"ry\022C\n\013filterCount\030\016 \003(\0132..protobuf.openc" +
"b.VariantStats.FilterCountEntry\022A\n\nfilte" +
"rFreq\030\017 \003(\0132-.protobuf.opencb.VariantSta" +
"ts.FilterFreqEntry\022\024\n\014qualityCount\030\024 \001(\005" +
"\022\022\n\nqualityAvg\030\020 \001(\002\022\013\n\003maf\030\n \001(\002\022\013\n\003mgf" +
"\030\013 \001(\002\022\021\n\tmafAllele\030\014 \001(\t\022\023\n\013mgfGenotype" +
"\030\r \001(\t\0324\n\022GenotypeCountEntry\022\013\n\003key\030\001 \001(" +
"\t\022\r\n\005value\030\002 \001(\005:\0028\001\0323\n\021GenotypeFreqEntr" +
"y\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\002:\0028\001\0322\n\020Fil" +
"terCountEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(" +
"\005:\0028\001\0321\n\017FilterFreqEntry\022\013\n\003key\030\001 \001(\t\022\r\n" +
"\005value\030\002 \001(\002:\0028\001\"6\n\014OriginalCall\022\021\n\tvari" +
"antId\030\001 \001(\t\022\023\n\013alleleIndex\030\002 \001(\005\"\251\001\n\tFil" +
"eEntry\022\016\n\006fileId\030\001 \001(\t\022+\n\004call\030\002 \001(\0132\035.p" +
"rotobuf.opencb.OriginalCall\0222\n\004data\030\003 \003(" +
"\0132$.protobuf.opencb.FileEntry.DataEntry\032" +
"+\n\tDataEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t" +
":\0028\001\"\227\001\n\023AlternateCoordinate\022\022\n\nchromoso" +
"me\030\001 \001(\t\022\r\n\005start\030\002 \001(\005\022\013\n\003end\030\003 \001(\005\022\021\n\t" +
"reference\030\004 \001(\t\022\021\n\talternate\030\005 \001(\t\022*\n\004ty" +
"pe\030\006 \001(\0162\034.protobuf.opencb.VariantType\"@" +
"\n\013SampleEntry\022\020\n\010sampleId\030\001 \001(\t\022\021\n\tfileI" +
"ndex\030\002 \001(\005\022\014\n\004data\030\003 \003(\t\"\200\002\n\nStudyEntry\022" +
"\017\n\007studyId\030\001 \001(\t\022)\n\005files\030\002 \003(\0132\032.protob" +
"uf.opencb.FileEntry\022A\n\023secondaryAlternat" +
"es\030\003 \003(\0132$.protobuf.opencb.AlternateCoor" +
"dinate\022\026\n\016sampleDataKeys\030\004 \003(\t\022-\n\007sample" +
"s\030\005 \003(\0132\034.protobuf.opencb.SampleEntry\022,\n" +
"\005stats\030\006 \003(\0132\035.protobuf.opencb.VariantSt" +
"ats\"e\n\014BreakendMate\022\022\n\nchromosome\030\001 \001(\t\022" +
"\020\n\010position\030\002 \001(\005\022\026\n\016ciPositionLeft\030\003 \001(" +
"\005\022\027\n\017ciPositionRight\030\004 \001(\005\"\202\001\n\010Breakend\022" +
"+\n\004mate\030\001 \001(\0132\035.protobuf.opencb.Breakend" +
"Mate\0229\n\013orientation\030\002 \001(\0162$.protobuf.ope" +
"ncb.BreakendOrientation\022\016\n\006insSeq\030\003 \001(\t\"" +
"\325\001\n\023StructuralVariation\022\023\n\013ciStartLeft\030\001" +
" \001(\005\022\024\n\014ciStartRight\030\002 \001(\005\022\021\n\tciEndLeft\030" +
"\003 \001(\005\022\022\n\nciEndRight\030\004 \001(\005\022\022\n\ncopyNumber\030" +
"\005 \001(\005\022\024\n\014leftSvInsSeq\030\006 \001(\t\022\025\n\rrightSvIn" +
"sSeq\030\007 \001(\t\022+\n\010breakend\030\t \001(\0132\031.protobuf." +
"opencb.Breakend\"\336\002\n\007Variant\022\022\n\nchromosom" +
"e\030\001 \001(\t\022\r\n\005start\030\002 \001(\005\022\013\n\003end\030\003 \001(\005\022\021\n\tr" +
"eference\030\004 \001(\t\022\021\n\talternate\030\005 \001(\t\022\016\n\006str" +
"and\030\006 \001(\t\0220\n\002sv\030\016 \001(\0132$.protobuf.opencb." +
"StructuralVariation\022\n\n\002id\030\r \001(\t\022\r\n\005names" +
"\030\007 \003(\t\022\016\n\006length\030\010 \001(\005\022*\n\004type\030\t \001(\0162\034.p" +
"rotobuf.opencb.VariantType\022,\n\007studies\030\013 " +
"\003(\0132\033.protobuf.opencb.StudyEntry\0226\n\nanno" +
"tation\030\014 \001(\0132\".protobuf.opencb.VariantAn" +
"notation\"\343\001\n\023VariantFileMetadata\022\016\n\006file" +
"Id\030\001 \001(\t\022\017\n\007studyId\030\002 \001(\t\022\020\n\010fileName\030\003 " +
"\001(\t\022\021\n\tstudyName\030\004 \001(\t\022\017\n\007samples\030\005 \003(\t\022" +
"D\n\010metadata\030\006 \003(\01322.protobuf.opencb.Vari" +
"antFileMetadata.MetadataEntry\032/\n\rMetadat" +
"aEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001*\253" +
"\002\n\013VariantType\022\020\n\014NO_VARIATION\020\000\022\007\n\003SNV\020" +
"\002\022\007\n\003MNV\020\004\022\t\n\005INDEL\020\005\022\006\n\002SV\020\006\022\017\n\013COPY_NU" +
"MBER\020\007\022\024\n\020COPY_NUMBER_GAIN\020\020\022\024\n\020COPY_NUM" +
"BER_LOSS\020\021\022\014\n\010SYMBOLIC\020\010\022\t\n\005MIXED\020\t\022\r\n\tI" +
"NSERTION\020\n\022\014\n\010DELETION\020\013\022\021\n\rTRANSLOCATIO" +
"N\020\014\022\r\n\tINVERSION\020\r\022\017\n\013DUPLICATION\020\016\022\026\n\022T" +
"ANDEM_DUPLICATION\020\022\022\014\n\010BREAKEND\020\017\022\007\n\003CNV" +
"\020\024\022\007\n\003SNP\020\001\022\007\n\003MNP\020\003*5\n\023BreakendOrientat" +
"ion\022\006\n\002SE\020\000\022\006\n\002SS\020\001\022\006\n\002ES\020\002\022\006\n\002EE\020\003B=\n*o" +
"rg.opencb.biodata.models.variant.protobu" +
"fB\014VariantProto\240\001\001b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.getDescriptor(),
});
internal_static_protobuf_opencb_VariantStats_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_protobuf_opencb_VariantStats_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantStats_descriptor,
new java.lang.String[] { "CohortId", "SampleCount", "FileCount", "AlleleCount", "RefAlleleCount", "AltAlleleCount", "RefAlleleFreq", "AltAlleleFreq", "MissingAlleleCount", "MissingGenotypeCount", "GenotypeCount", "GenotypeFreq", "FilterCount", "FilterFreq", "QualityCount", "QualityAvg", "Maf", "Mgf", "MafAllele", "MgfGenotype", });
internal_static_protobuf_opencb_VariantStats_GenotypeCountEntry_descriptor =
internal_static_protobuf_opencb_VariantStats_descriptor.getNestedTypes().get(0);
internal_static_protobuf_opencb_VariantStats_GenotypeCountEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantStats_GenotypeCountEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_protobuf_opencb_VariantStats_GenotypeFreqEntry_descriptor =
internal_static_protobuf_opencb_VariantStats_descriptor.getNestedTypes().get(1);
internal_static_protobuf_opencb_VariantStats_GenotypeFreqEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantStats_GenotypeFreqEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_protobuf_opencb_VariantStats_FilterCountEntry_descriptor =
internal_static_protobuf_opencb_VariantStats_descriptor.getNestedTypes().get(2);
internal_static_protobuf_opencb_VariantStats_FilterCountEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantStats_FilterCountEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_protobuf_opencb_VariantStats_FilterFreqEntry_descriptor =
internal_static_protobuf_opencb_VariantStats_descriptor.getNestedTypes().get(3);
internal_static_protobuf_opencb_VariantStats_FilterFreqEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantStats_FilterFreqEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_protobuf_opencb_OriginalCall_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_protobuf_opencb_OriginalCall_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_OriginalCall_descriptor,
new java.lang.String[] { "VariantId", "AlleleIndex", });
internal_static_protobuf_opencb_FileEntry_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_protobuf_opencb_FileEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_FileEntry_descriptor,
new java.lang.String[] { "FileId", "Call", "Data", });
internal_static_protobuf_opencb_FileEntry_DataEntry_descriptor =
internal_static_protobuf_opencb_FileEntry_descriptor.getNestedTypes().get(0);
internal_static_protobuf_opencb_FileEntry_DataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_FileEntry_DataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_protobuf_opencb_AlternateCoordinate_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_protobuf_opencb_AlternateCoordinate_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_AlternateCoordinate_descriptor,
new java.lang.String[] { "Chromosome", "Start", "End", "Reference", "Alternate", "Type", });
internal_static_protobuf_opencb_SampleEntry_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_protobuf_opencb_SampleEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_SampleEntry_descriptor,
new java.lang.String[] { "SampleId", "FileIndex", "Data", });
internal_static_protobuf_opencb_StudyEntry_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_protobuf_opencb_StudyEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_StudyEntry_descriptor,
new java.lang.String[] { "StudyId", "Files", "SecondaryAlternates", "SampleDataKeys", "Samples", "Stats", });
internal_static_protobuf_opencb_BreakendMate_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_protobuf_opencb_BreakendMate_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_BreakendMate_descriptor,
new java.lang.String[] { "Chromosome", "Position", "CiPositionLeft", "CiPositionRight", });
internal_static_protobuf_opencb_Breakend_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_protobuf_opencb_Breakend_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_Breakend_descriptor,
new java.lang.String[] { "Mate", "Orientation", "InsSeq", });
internal_static_protobuf_opencb_StructuralVariation_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_protobuf_opencb_StructuralVariation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_StructuralVariation_descriptor,
new java.lang.String[] { "CiStartLeft", "CiStartRight", "CiEndLeft", "CiEndRight", "CopyNumber", "LeftSvInsSeq", "RightSvInsSeq", "Breakend", });
internal_static_protobuf_opencb_Variant_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_protobuf_opencb_Variant_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_Variant_descriptor,
new java.lang.String[] { "Chromosome", "Start", "End", "Reference", "Alternate", "Strand", "Sv", "Id", "Names", "Length", "Type", "Studies", "Annotation", });
internal_static_protobuf_opencb_VariantFileMetadata_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_protobuf_opencb_VariantFileMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantFileMetadata_descriptor,
new java.lang.String[] { "FileId", "StudyId", "FileName", "StudyName", "Samples", "Metadata", });
internal_static_protobuf_opencb_VariantFileMetadata_MetadataEntry_descriptor =
internal_static_protobuf_opencb_VariantFileMetadata_descriptor.getNestedTypes().get(0);
internal_static_protobuf_opencb_VariantFileMetadata_MetadataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_protobuf_opencb_VariantFileMetadata_MetadataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
org.opencb.biodata.models.variant.protobuf.VariantAnnotationProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy