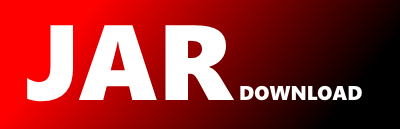
org.opencb.cellbase.lib.managers.TranscriptManager Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015-2020 OpenCB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.opencb.cellbase.lib.managers;
import org.opencb.biodata.models.core.Transcript;
import org.opencb.cellbase.core.api.TranscriptQuery;
import org.opencb.cellbase.core.api.query.ProjectionQueryOptions;
import org.opencb.cellbase.core.config.CellBaseConfiguration;
import org.opencb.cellbase.core.exception.CellBaseException;
import org.opencb.cellbase.core.result.CellBaseDataResult;
import org.opencb.cellbase.lib.impl.core.CellBaseCoreDBAdaptor;
import org.opencb.cellbase.lib.impl.core.TranscriptMongoDBAdaptor;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class TranscriptManager extends AbstractManager implements AggregationApi {
private TranscriptMongoDBAdaptor transcriptDBAdaptor;
public TranscriptManager(String species, CellBaseConfiguration configuration) throws CellBaseException {
this(species, null, configuration);
}
public TranscriptManager(String species, String assembly, CellBaseConfiguration configuration)
throws CellBaseException {
super(species, assembly, configuration);
this.init();
}
private void init() {
transcriptDBAdaptor = dbAdaptorFactory.getTranscriptDBAdaptor();
}
@Override
public CellBaseCoreDBAdaptor getDBAdaptor() {
return transcriptDBAdaptor;
}
public List> info(List ids, ProjectionQueryOptions query, String source, int dataRelease,
String apiKey) throws CellBaseException {
return transcriptDBAdaptor.info(ids, query, source, dataRelease, apiKey);
}
public CellBaseDataResult getCdna(String id, int dataRelease) throws CellBaseException {
TranscriptQuery query = new TranscriptQuery();
query.setDataRelease(dataRelease);
query.setTranscriptsXrefs(Collections.singletonList(id));
CellBaseDataResult transcriptCellBaseDataResult = transcriptDBAdaptor.query(query);
String cdnaSequence = null;
if (transcriptCellBaseDataResult != null && !transcriptCellBaseDataResult.getResults().isEmpty()) {
for (Transcript transcript: transcriptCellBaseDataResult.getResults()) {
// transcript.id will have version. id is from the user, so can include the version or not.
if (transcript.getId().startsWith(id)) {
cdnaSequence = transcript.getCdnaSequence();
break;
}
}
}
return new CellBaseDataResult<>(id, transcriptCellBaseDataResult.getTime(), transcriptCellBaseDataResult.getEvents(),
transcriptCellBaseDataResult.getNumResults(), Collections.singletonList(cdnaSequence), 1);
}
private List> getCdna(List idList, int dataRelease) throws CellBaseException {
List> cellBaseDataResults = new ArrayList<>();
for (String id : idList) {
cellBaseDataResults.add(getCdna(id, dataRelease));
}
return cellBaseDataResults;
}
public List> getSequence(String id, int dataRelease) throws CellBaseException {
List transcriptsList = Arrays.asList(id.split(","));
List> queryResult = getCdna(transcriptsList, dataRelease);
for (int i = 0; i < transcriptsList.size(); i++) {
queryResult.get(i).setId(transcriptsList.get(i));
}
return queryResult;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy