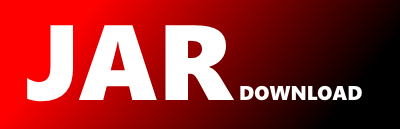
R.Alignment-methods.R Maven / Gradle / Ivy
# WARNING: AUTOGENERATED CODE
#
# This code was generated by a tool.
#
# Manual changes to this file may cause unexpected behavior in your application.
# Manual changes to this file will be overwritten if the code is regenerated.
# ##############################################################################
#' AlignmentClient methods
#' @include AllClasses.R
#' @include AllGenerics.R
#' @include commons.R
#' @description This function implements the OpenCGA calls for managing Analysis - Alignment.
#' The following table summarises the available *actions* for this client:
#'
#' | endpointName | Endpoint WS | parameters accepted |
#' | -- | :-- | --: |
#' | runBwa | /{apiVersion}/analysis/alignment/bwa/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | runCoverageIndex | /{apiVersion}/analysis/alignment/coverage/index/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | coverageQcGeneCoverageStatsRun | /{apiVersion}/analysis/alignment/coverage/qc/geneCoverageStats/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | queryCoverage | /{apiVersion}/analysis/alignment/coverage/query | file[*], study, region, gene, offset, onlyExons, range, windowSize, splitResults |
#' | ratioCoverage | /{apiVersion}/analysis/alignment/coverage/ratio | file1[*], file2[*], study, skipLog2, region, gene, offset, onlyExons, windowSize, splitResults |
#' | statsCoverage | /{apiVersion}/analysis/alignment/coverage/stats | file[*], gene[*], study, threshold |
#' | runDeeptools | /{apiVersion}/analysis/alignment/deeptools/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | runFastqc | /{apiVersion}/analysis/alignment/fastqc/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | runIndex | /{apiVersion}/analysis/alignment/index/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | runPicard | /{apiVersion}/analysis/alignment/picard/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | runQc | /{apiVersion}/analysis/alignment/qc/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | query | /{apiVersion}/analysis/alignment/query | limit, skip, count, file[*], study, region, gene, offset, onlyExons, minMappingQuality, maxNumMismatches, maxNumHits, properlyPaired, maxInsertSize, skipUnmapped, skipDuplicated, regionContained, forceMDField, binQualities, splitResults |
#' | runSamtools | /{apiVersion}/analysis/alignment/samtools/run | study, jobId, jobDependsOn, jobDescription, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#'
#' @md
#' @seealso \url{http://docs.opencb.org/display/opencga/Using+OpenCGA} and the RESTful API documentation
#' \url{http://bioinfo.hpc.cam.ac.uk/opencga-prod/webservices/}
#' [*]: Required parameter
#' @export
setMethod("alignmentClient", "OpencgaR", function(OpencgaR, endpointName, params=NULL, ...) {
switch(endpointName,
#' @section Endpoint /{apiVersion}/analysis/alignment/bwa/run:
#' BWA is a software package for mapping low-divergent sequences against a large reference genome.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data BWA parameters.
runBwa=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL, subcategory="alignment/bwa",
subcategoryId=NULL, action="run", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/coverage/index/run:
#' Compute coverage for a list of alignment files.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Coverage computation parameters.
runCoverageIndex=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/coverage/index", subcategoryId=NULL, action="run", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/coverage/qc/geneCoverageStats/run:
#' Compute gene coverage stats for a given alignment file and a list of genes.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Gene coverage stats parameters for a given BAM file and a list of genes.
coverageQcGeneCoverageStatsRun=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/coverage/qc/geneCoverageStats", subcategoryId=NULL, action="run", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/coverage/query:
#' Query the coverage of an alignment file for regions or genes.
#' @param file File ID.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param region Comma separated list of regions 'chr:start-end, e.g.: 2,3:63500-65000.
#' @param gene Comma separated list of genes, e.g.: BCRA2,TP53.
#' @param offset Offset to extend the region, gene or exon at up and downstream.
#' @param onlyExons Only exons are taking into account when genes are specified.
#' @param range Range of coverage values to be reported. Minimum and maximum values are separated by '-', e.g.: 20-40 (for coverage values greater or equal to 20 and less or equal to 40). A single value means to report coverage values less or equal to that value.
#' @param windowSize Window size for the region coverage (if a coverage range is provided, window size must be 1).
#' @param splitResults Split results into regions (or gene/exon regions).
queryCoverage=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/coverage", subcategoryId=NULL, action="query", params=params, httpMethod="GET",
as.queryParam=c("file"), ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/coverage/ratio:
#' Compute coverage ratio from file #1 vs file #2, (e.g. somatic vs germline).
#' @param file1 Input file #1 (e.g. somatic file).
#' @param file2 Input file #2 (e.g. germline file).
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param skipLog2 Do not apply Log2 to normalise the coverage ratio.
#' @param region Comma separated list of regions 'chr:start-end, e.g.: 2,3:63500-65000.
#' @param gene Comma separated list of genes, e.g.: BCRA2,TP53.
#' @param offset Offset to extend the region, gene or exon at up and downstream.
#' @param onlyExons Only exons are taking into account when genes are specified.
#' @param windowSize Window size for the region coverage (if a coverage range is provided, window size must be 1).
#' @param splitResults Split results into regions (or gene/exon regions).
ratioCoverage=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/coverage", subcategoryId=NULL, action="ratio", params=params, httpMethod="GET",
as.queryParam=c("file1","file2"), ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/coverage/stats:
#' Compute coverage stats per transcript for a list of genes.
#' @param file File ID.
#' @param gene Comma separated list of genes, e.g.: BCRA2,TP53.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param threshold Only regions whose coverage depth is under this threshold will be reported.
statsCoverage=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/coverage", subcategoryId=NULL, action="stats", params=params, httpMethod="GET",
as.queryParam=c("file","gene"), ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/deeptools/run:
#' Deeptools is a suite of python tools particularly developed for the efficient analysis of high-throughput sequencing data, such as ChIP-seq, RNA-seq or MNase-seq.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Deeptools parameters. Supported Deeptools commands: bamCoverage, bamCompare.
runDeeptools=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/deeptools", subcategoryId=NULL, action="run", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/fastqc/run:
#' A high throughput sequence QC analysis tool.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data FastQC parameters.
runFastqc=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL, subcategory="alignment/fastqc",
subcategoryId=NULL, action="run", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/index/run:
#' Index alignment file.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Alignment index params.
runIndex=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL, subcategory="alignment/index",
subcategoryId=NULL, action="run", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/picard/run:
#' Picard is a set of command line tools (in Java) for manipulating high-throughput sequencing (HTS) data and formats such as SAM/BAM/CRAM and VCF. Supported Picard commands: CollectHsMetrics, CollectWgsMetrics, BedToIntervalList.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Picard parameters. Supported Picard commands: CollectHsMetrics, CollectWgsMetrics, BedToIntervalList.
runPicard=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL, subcategory="alignment/picard",
subcategoryId=NULL, action="run", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/qc/run:
#' Compute quality control (QC) metrics for a given alignment file: samtools stats, samtools flag stats and FastQC metrics.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Alignment quality control (QC) parameters. It computes: stats, flag stats and fastqc metrics. The BAM file ID is mandatory and in order to skip some metrics, use the following keywords (separated by commas): stats, flagstats, fastqc.
runQc=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL, subcategory="alignment/qc",
subcategoryId=NULL, action="run", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/query:
#' Search over indexed alignments.
#' @param limit Number of results to be returned.
#' @param skip Number of results to skip.
#' @param count Get the total number of results matching the query. Deactivated by default.
#' @param file File ID.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param region Comma separated list of regions 'chr:start-end, e.g.: 2,3:63500-65000.
#' @param gene Comma separated list of genes, e.g.: BCRA2,TP53.
#' @param offset Offset to extend the region, gene or exon at up and downstream.
#' @param onlyExons Only exons are taking into account when genes are specified.
#' @param minMappingQuality Minimum mapping quality.
#' @param maxNumMismatches Maximum number of mismatches.
#' @param maxNumHits Maximum number of hits.
#' @param properlyPaired Return only properly paired alignments.
#' @param maxInsertSize Maximum insert size.
#' @param skipUnmapped Skip unmapped alignments.
#' @param skipDuplicated Skip duplicated alignments.
#' @param regionContained Return alignments contained within boundaries of region.
#' @param forceMDField Force SAM MD optional field to be set with the alignments.
#' @param binQualities Compress the nucleotide qualities by using 8 quality levels.
#' @param splitResults Split results into regions (or gene/exon regions).
query=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL, subcategory="alignment",
subcategoryId=NULL, action="query", params=params, httpMethod="GET", as.queryParam=c("file"), ...),
#' @section Endpoint /{apiVersion}/analysis/alignment/samtools/run:
#' Samtools is a program for interacting with high-throughput sequencing data in SAM, BAM and CRAM formats. Supported Samtools commands: sort, index, view, stats, flagstat, dict, faidx, depth, plot-bamstats.
#' @param study study.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobDescription Job description.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Samtools parameters. Supported Samtools commands: sort, index, view, stats, flagstat, dict, faidx, depth, plot-bamstats.
runSamtools=fetchOpenCGA(object=OpencgaR, category="analysis", categoryId=NULL,
subcategory="alignment/samtools", subcategoryId=NULL, action="run", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
)
})
© 2015 - 2025 Weber Informatics LLC | Privacy Policy