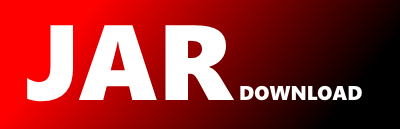
R.Cohort-methods.R Maven / Gradle / Ivy
# WARNING: AUTOGENERATED CODE
#
# This code was generated by a tool.
#
# Manual changes to this file may cause unexpected behavior in your application.
# Manual changes to this file will be overwritten if the code is regenerated.
# ##############################################################################
#' CohortClient methods
#' @include AllClasses.R
#' @include AllGenerics.R
#' @include commons.R
#' @description This function implements the OpenCGA calls for managing Cohorts.
#' The following table summarises the available *actions* for this client:
#'
#' | endpointName | Endpoint WS | parameters accepted |
#' | -- | :-- | --: |
#' | updateAcl | /{apiVersion}/cohorts/acl/{members}/update | study, members[*], action[*], body[*] |
#' | loadAnnotationSets | /{apiVersion}/cohorts/annotationSets/load | study, variableSetId[*], path[*], parents, annotationSetId, body |
#' | create | /{apiVersion}/cohorts/create | include, exclude, study, variableSet, variable, includeResult, body[*] |
#' | distinct | /{apiVersion}/cohorts/distinct | study, id, name, uuid, type, creationDate, modificationDate, deleted, status, internalStatus, annotation, acl, samples, numSamples, release, field[*] |
#' | generate | /{apiVersion}/cohorts/generate | include, exclude, study, id, somatic, individualId, fileIds, creationDate, modificationDate, internalStatus, status, phenotypes, annotation, acl, release, snapshot, includeResult, body[*] |
#' | search | /{apiVersion}/cohorts/search | include, exclude, limit, skip, count, flattenAnnotations, study, id, name, uuid, type, creationDate, modificationDate, deleted, status, internalStatus, annotation, acl, samples, numSamples, release |
#' | acl | /{apiVersion}/cohorts/{cohorts}/acl | cohorts[*], study, member, silent |
#' | delete | /{apiVersion}/cohorts/{cohorts}/delete | study, cohorts[*] |
#' | info | /{apiVersion}/cohorts/{cohorts}/info | include, exclude, flattenAnnotations, cohorts[*], study, deleted |
#' | update | /{apiVersion}/cohorts/{cohorts}/update | include, exclude, cohorts[*], study, samplesAction, annotationSetsAction, includeResult, body |
#' | updateAnnotationSetsAnnotations | /{apiVersion}/cohorts/{cohort}/annotationSets/{annotationSet}/annotations/update | cohort[*], study, annotationSet[*], action, body |
#'
#' @md
#' @seealso \url{http://docs.opencb.org/display/opencga/Using+OpenCGA} and the RESTful API documentation
#' \url{http://bioinfo.hpc.cam.ac.uk/opencga-prod/webservices/}
#' [*]: Required parameter
#' @export
setMethod("cohortClient", "OpencgaR", function(OpencgaR, annotationSet, cohort, cohorts, members, endpointName, params=NULL, ...) {
switch(endpointName,
#' @section Endpoint /{apiVersion}/cohorts/acl/{members}/update:
#' Update the set of permissions granted for the member.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param members Comma separated list of user or group ids.
#' @param action Action to be performed [ADD, SET, REMOVE or RESET]. Allowed values: ['SET ADD REMOVE RESET']
#' @param data JSON containing the parameters to add ACLs.
updateAcl=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=NULL, subcategory="acl",
subcategoryId=members, action="update", params=params, httpMethod="POST", as.queryParam=c("action"),
...),
#' @section Endpoint /{apiVersion}/cohorts/annotationSets/load:
#' Load annotation sets from a TSV file.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param variableSetId Variable set ID or name.
#' @param path Path where the TSV file is located in OpenCGA or where it should be located.
#' @param parents Flag indicating whether to create parent directories if they don't exist (only when TSV file was not previously associated).
#' @param annotationSetId Annotation set id. If not provided, variableSetId will be used.
#' @param data JSON containing the 'content' of the TSV file if this has not yet been registered into OpenCGA.
loadAnnotationSets=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=NULL,
subcategory="annotationSets", subcategoryId=NULL, action="load", params=params, httpMethod="POST",
as.queryParam=c("variableSetId","path"), ...),
#' @section Endpoint /{apiVersion}/cohorts/create:
#' Create a cohort.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param variableSet Deprecated: Use /generate web service and filter by annotation.
#' @param variable Deprecated: Use /generate web service and filter by annotation.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing cohort information.
create=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="create", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/distinct:
#' Cohort distinct method.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param id Comma separated list of cohort IDs up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param name Comma separated list of cohort names up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param uuid Comma separated list of cohort IDs up to a maximum of 100.
#' @param type Cohort type.
#' @param creationDate creationDate.
#' @param modificationDate modificationDate.
#' @param deleted deleted.
#' @param status status.
#' @param internalStatus internalStatus.
#' @param annotation Cohort annotation.
#' @param acl acl.
#' @param samples Cohort sample IDs.
#' @param numSamples Number of samples.
#' @param release release.
#' @param field Comma separated list of fields for which to obtain the distinct values.
distinct=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=NULL, subcategory=NULL,
subcategoryId=NULL, action="distinct", params=params, httpMethod="GET", as.queryParam=c("field"), ...),
#' @section Endpoint /{apiVersion}/cohorts/generate:
#' Create a cohort based on a sample query.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param id Comma separated list sample IDs or UUIDs up to a maximum of 100.
#' @param somatic Somatic sample.
#' @param individualId Individual ID or UUID.
#' @param fileIds Comma separated list of file IDs, paths or UUIDs.
#' @param creationDate Creation date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param modificationDate Modification date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param internalStatus Filter by internal status.
#' @param status Filter by status.
#' @param phenotypes Comma separated list of phenotype ids or names. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param annotation Annotation filters. Example: age>30;gender=FEMALE. For more information, please visit http://docs.opencb.org/display/opencga/AnnotationSets+1.4.0.
#' @param acl Filter entries for which a user has the provided permissions. Format: acl={user}:{permissions}. Example: acl=john:WRITE,WRITE_ANNOTATIONS will return all entries for which user john has both WRITE and WRITE_ANNOTATIONS permissions. Only study owners or administrators can query by this field. .
#' @param release Release when it was created.
#' @param snapshot Snapshot value (Latest version of the entry in the specified release).
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing cohort information.
generate=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=NULL, subcategory=NULL,
subcategoryId=NULL, action="generate", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/search:
#' Search cohorts.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param limit Number of results to be returned.
#' @param skip Number of results to skip.
#' @param count Get the total number of results matching the query. Deactivated by default.
#' @param flattenAnnotations Flatten the annotations?.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param id Comma separated list of cohort IDs up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param name Comma separated list of cohort names up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param uuid Comma separated list of cohort IDs up to a maximum of 100.
#' @param type Cohort type.
#' @param creationDate creationDate.
#' @param modificationDate modificationDate.
#' @param deleted deleted.
#' @param status status.
#' @param internalStatus internalStatus.
#' @param annotation Cohort annotation.
#' @param acl acl.
#' @param samples Cohort sample IDs.
#' @param numSamples Number of samples.
#' @param release release.
search=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="search", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/{cohorts}/acl:
#' Return the acl of the cohort. If member is provided, it will only return the acl for the member.
#' @param cohorts Comma separated list of cohort IDs or UUIDs up to a maximum of 100.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param member User or group id.
#' @param silent Boolean to retrieve all possible entries that are queried for, false to raise an exception whenever one of the entries looked for cannot be shown for whichever reason.
acl=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=cohorts, subcategory=NULL, subcategoryId=NULL,
action="acl", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/{cohorts}/delete:
#' Delete cohorts.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param cohorts Comma separated list of cohort ids.
delete=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=cohorts, subcategory=NULL,
subcategoryId=NULL, action="delete", params=params, httpMethod="DELETE", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/{cohorts}/info:
#' Get cohort information.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param flattenAnnotations Flatten the annotations?.
#' @param cohorts Comma separated list of cohort IDs or UUIDs up to a maximum of 100.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param deleted Boolean to retrieve deleted cohorts.
info=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=cohorts, subcategory=NULL,
subcategoryId=NULL, action="info", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/{cohorts}/update:
#' Update some cohort attributes.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param cohorts Comma separated list of cohort ids.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param samplesAction Action to be performed if the array of samples is being updated. Allowed values: ['ADD SET REMOVE']
#' @param annotationSetsAction Action to be performed if the array of annotationSets is being updated. Allowed values: ['ADD SET REMOVE']
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data body.
update=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=cohorts, subcategory=NULL,
subcategoryId=NULL, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/cohorts/{cohort}/annotationSets/{annotationSet}/annotations/update:
#' Update annotations from an annotationSet.
#' @param cohort Cohort ID.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param annotationSet AnnotationSet ID to be updated.
#' @param action Action to be performed: ADD to add new annotations; REPLACE to replace the value of an already existing annotation; SET to set the new list of annotations removing any possible old annotations; REMOVE to remove some annotations; RESET to set some annotations to the default value configured in the corresponding variables of the VariableSet if any. Allowed values: ['ADD SET REMOVE RESET REPLACE']
#' @param data Json containing the map of annotations when the action is ADD, SET or REPLACE, a json with only the key 'remove' containing the comma separated variables to be removed as a value when the action is REMOVE or a json with only the key 'reset' containing the comma separated variables that will be set to the default value when the action is RESET.
updateAnnotationSetsAnnotations=fetchOpenCGA(object=OpencgaR, category="cohorts", categoryId=cohort,
subcategory="annotationSets", subcategoryId=annotationSet, action="annotations/update", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
)
})
© 2015 - 2025 Weber Informatics LLC | Privacy Policy