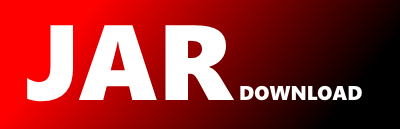
R.Job-methods.R Maven / Gradle / Ivy
# WARNING: AUTOGENERATED CODE
#
# This code was generated by a tool.
#
# Manual changes to this file may cause unexpected behavior in your application.
# Manual changes to this file will be overwritten if the code is regenerated.
# ##############################################################################
#' JobClient methods
#' @include AllClasses.R
#' @include AllGenerics.R
#' @include commons.R
#' @description This function implements the OpenCGA calls for managing Jobs.
#' The following table summarises the available *actions* for this client:
#'
#' | endpointName | Endpoint WS | parameters accepted |
#' | -- | :-- | --: |
#' | updateAcl | /{apiVersion}/jobs/acl/{members}/update | members[*], action[*], body[*] |
#' | create | /{apiVersion}/jobs/create | study, body[*] |
#' | distinct | /{apiVersion}/jobs/distinct | study, otherStudies, id, uuid, toolId, toolType, userId, priority, status, internalStatus, creationDate, modificationDate, visited, tags, input, output, acl, release, deleted, field[*] |
#' | retry | /{apiVersion}/jobs/retry | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, study, body[*] |
#' | search | /{apiVersion}/jobs/search | include, exclude, limit, skip, count, study, otherStudies, id, uuid, toolId, toolType, userId, priority, status, internalStatus, creationDate, modificationDate, visited, tags, input, output, acl, release, deleted |
#' | top | /{apiVersion}/jobs/top | limit, study, internalStatus, priority, userId, toolId |
#' | acl | /{apiVersion}/jobs/{jobs}/acl | jobs[*], member, silent |
#' | delete | /{apiVersion}/jobs/{jobs}/delete | study, jobs[*] |
#' | info | /{apiVersion}/jobs/{jobs}/info | include, exclude, jobs[*], study, deleted |
#' | update | /{apiVersion}/jobs/{jobs}/update | include, exclude, jobs[*], study, includeResult, body |
#' | kill | /{apiVersion}/jobs/{job}/kill | job[*], study |
#' | headLog | /{apiVersion}/jobs/{job}/log/head | job[*], study, offset, lines, type |
#' | tailLog | /{apiVersion}/jobs/{job}/log/tail | job[*], study, lines, type |
#'
#' @md
#' @seealso \url{http://docs.opencb.org/display/opencga/Using+OpenCGA} and the RESTful API documentation
#' \url{http://bioinfo.hpc.cam.ac.uk/opencga-prod/webservices/}
#' [*]: Required parameter
#' @export
setMethod("jobClient", "OpencgaR", function(OpencgaR, job, jobs, members, endpointName, params=NULL, ...) {
switch(endpointName,
#' @section Endpoint /{apiVersion}/jobs/acl/{members}/update:
#' Update the set of permissions granted for the member.
#' @param members Comma separated list of user or group ids.
#' @param action Action to be performed [ADD, SET, REMOVE or RESET]. Allowed values: ['SET ADD REMOVE RESET']
#' @param data JSON containing the parameters to add ACLs.
updateAcl=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=NULL, subcategory="acl",
subcategoryId=members, action="update", params=params, httpMethod="POST", as.queryParam=c("action"),
...),
#' @section Endpoint /{apiVersion}/jobs/create:
#' Register an executed job with POST method.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data job.
create=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="create", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/distinct:
#' Job distinct method.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param otherStudies Flag indicating the entries being queried can belong to any related study, not just the primary one.
#' @param id Comma separated list of job IDs up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param uuid Comma separated list of job UUIDs up to a maximum of 100.
#' @param toolId Tool ID executed by the job. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param toolType Tool type executed by the job [OPERATION, ANALYSIS].
#' @param userId User that created the job.
#' @param priority Priority of the job.
#' @param status Filter by status.
#' @param internalStatus Filter by internal status.
#' @param creationDate Creation date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param modificationDate Modification date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param visited Visited status of job.
#' @param tags Job tags.
#' @param input Comma separated list of file IDs used as input.
#' @param output Comma separated list of file IDs used as output.
#' @param acl Filter entries for which a user has the provided permissions. Format: acl={user}:{permissions}. Example: acl=john:WRITE,WRITE_ANNOTATIONS will return all entries for which user john has both WRITE and WRITE_ANNOTATIONS permissions. Only study owners or administrators can query by this field. .
#' @param release Release when it was created.
#' @param deleted Boolean to retrieve deleted entries.
#' @param field Comma separated list of fields for which to obtain the distinct values.
distinct=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="distinct", params=params, httpMethod="GET", as.queryParam=c("field"), ...),
#' @section Endpoint /{apiVersion}/jobs/retry:
#' Relaunch a failed job.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data job.
retry=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="retry", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/search:
#' Job search method.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param limit Number of results to be returned.
#' @param skip Number of results to skip.
#' @param count Get the total number of results matching the query. Deactivated by default.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param otherStudies Flag indicating the entries being queried can belong to any related study, not just the primary one.
#' @param id Comma separated list of job IDs up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param uuid Comma separated list of job UUIDs up to a maximum of 100.
#' @param toolId Tool ID executed by the job. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param toolType Tool type executed by the job [OPERATION, ANALYSIS].
#' @param userId User that created the job.
#' @param priority Priority of the job.
#' @param status Filter by status.
#' @param internalStatus Filter by internal status.
#' @param creationDate Creation date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param modificationDate Modification date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param visited Visited status of job.
#' @param tags Job tags.
#' @param input Comma separated list of file IDs used as input.
#' @param output Comma separated list of file IDs used as output.
#' @param acl Filter entries for which a user has the provided permissions. Format: acl={user}:{permissions}. Example: acl=john:WRITE,WRITE_ANNOTATIONS will return all entries for which user john has both WRITE and WRITE_ANNOTATIONS permissions. Only study owners or administrators can query by this field. .
#' @param release Release when it was created.
#' @param deleted Boolean to retrieve deleted entries.
search=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="search", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/top:
#' Provide a summary of the running jobs.
#' @param limit Maximum number of jobs to be returned.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param internalStatus Filter by internal status.
#' @param priority Priority of the job.
#' @param userId User that created the job.
#' @param toolId Tool ID executed by the job. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
top=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="top", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{jobs}/acl:
#' Return the acl of the job. If member is provided, it will only return the acl for the member.
#' @param jobs Comma separated list of job IDs or UUIDs up to a maximum of 100.
#' @param member User or group id.
#' @param silent Boolean to retrieve all possible entries that are queried for, false to raise an exception whenever one of the entries looked for cannot be shown for whichever reason.
acl=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=jobs, subcategory=NULL, subcategoryId=NULL,
action="acl", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{jobs}/delete:
#' Delete existing jobs.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param jobs Comma separated list of job ids.
delete=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=jobs, subcategory=NULL, subcategoryId=NULL,
action="delete", params=params, httpMethod="DELETE", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{jobs}/info:
#' Get job information.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param jobs Comma separated list of job IDs or UUIDs up to a maximum of 100.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param deleted Boolean to retrieve deleted jobs.
info=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=jobs, subcategory=NULL, subcategoryId=NULL,
action="info", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{jobs}/update:
#' Update some job attributes.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param jobs Comma separated list of job IDs or UUIDs up to a maximum of 100.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data body.
update=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=jobs, subcategory=NULL, subcategoryId=NULL,
action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{job}/kill:
#' Send a signal to kill a pending or running job.
#' @param job Job ID or UUID.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
kill=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=job, subcategory=NULL, subcategoryId=NULL,
action="kill", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{job}/log/head:
#' Show the first lines of a log file (up to a limit).
#' @param job Job ID or UUID.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param offset Starting byte from which the file will be read.
#' @param lines Maximum number of lines to be returned up to a maximum of 1000.
#' @param type Log file to be shown (stdout or stderr).
headLog=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=job, subcategory="log", subcategoryId=NULL,
action="head", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/jobs/{job}/log/tail:
#' Show the last lines of a log file (up to a limit).
#' @param job Job ID or UUID.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param lines Maximum number of lines to be returned up to a maximum of 1000.
#' @param type Log file to be shown (stdout or stderr).
tailLog=fetchOpenCGA(object=OpencgaR, category="jobs", categoryId=job, subcategory="log", subcategoryId=NULL,
action="tail", params=params, httpMethod="GET", as.queryParam=NULL, ...),
)
})
© 2015 - 2025 Weber Informatics LLC | Privacy Policy