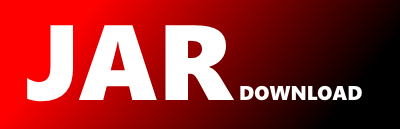
R.Operation-methods.R Maven / Gradle / Ivy
# WARNING: AUTOGENERATED CODE
#
# This code was generated by a tool.
#
# Manual changes to this file may cause unexpected behavior in your application.
# Manual changes to this file will be overwritten if the code is regenerated.
# ##############################################################################
#' OperationClient methods
#' @include AllClasses.R
#' @include AllGenerics.R
#' @include commons.R
#' @description This function implements the OpenCGA calls for managing Operations - Variant Storage.
#' The following table summarises the available *actions* for this client:
#'
#' | endpointName | Endpoint WS | parameters accepted |
#' | -- | :-- | --: |
#' | configureCellbase | /{apiVersion}/operation/cellbase/configure | project, annotationUpdate, annotationSaveId, body |
#' | aggregateVariant | /{apiVersion}/operation/variant/aggregate | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | deleteVariantAnnotation | /{apiVersion}/operation/variant/annotation/delete | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, project, annotationId |
#' | indexVariantAnnotation | /{apiVersion}/operation/variant/annotation/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, project, study, body |
#' | saveVariantAnnotation | /{apiVersion}/operation/variant/annotation/save | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, project, body |
#' | configureVariant | /{apiVersion}/operation/variant/configure | project, study, body |
#' | deleteVariant | /{apiVersion}/operation/variant/delete | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | aggregateVariantFamily | /{apiVersion}/operation/variant/family/aggregate | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | indexVariantFamily | /{apiVersion}/operation/variant/family/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | indexVariant | /{apiVersion}/operation/variant/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | launcherVariantIndex | /{apiVersion}/operation/variant/index/launcher | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | runVariantJulie | /{apiVersion}/operation/variant/julie/run | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, project, body[*] |
#' | repairVariantMetadata | /{apiVersion}/operation/variant/metadata/repair | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body |
#' | synchronizeVariantMetadata | /{apiVersion}/operation/variant/metadata/synchronize | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | pruneVariant | /{apiVersion}/operation/variant/prune | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body |
#' | deleteVariantSample | /{apiVersion}/operation/variant/sample/delete | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | indexVariantSample | /{apiVersion}/operation/variant/sample/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | variantSampleIndexConfigure | /{apiVersion}/operation/variant/sample/index/configure | study, skipRebuild, body |
#' | deleteVariantScore | /{apiVersion}/operation/variant/score/delete | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, name, resume, force |
#' | indexVariantScore | /{apiVersion}/operation/variant/score/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | variantSecondaryAnnotationIndex | /{apiVersion}/operation/variant/secondary/annotation/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, project, study, body |
#' | variantSecondarySampleIndex | /{apiVersion}/operation/variant/secondary/sample/index | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#' | configureVariantSecondarySampleIndex | /{apiVersion}/operation/variant/secondary/sample/index/configure | study, skipRebuild, body |
#' | secondaryIndexVariant | /{apiVersion}/operation/variant/secondaryIndex | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, project, study, body |
#' | deleteVariantSecondaryIndex | /{apiVersion}/operation/variant/secondaryIndex/delete | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, samples |
#' | setupVariant | /{apiVersion}/operation/variant/setup | study, body |
#' | deleteVariantStats | /{apiVersion}/operation/variant/stats/delete | study, jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | indexVariantStats | /{apiVersion}/operation/variant/stats/index | study, jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, body[*] |
#' | deleteVariantStudy | /{apiVersion}/operation/variant/study/delete | jobId, jobDescription, jobDependsOn, jobTags, jobScheduledStartTime, jobPriority, jobDryRun, study, body |
#'
#' @md
#' @seealso \url{http://docs.opencb.org/display/opencga/Using+OpenCGA} and the RESTful API documentation
#' \url{http://bioinfo.hpc.cam.ac.uk/opencga-prod/webservices/}
#' [*]: Required parameter
#' @export
setMethod("operationClient", "OpencgaR", function(OpencgaR, endpointName, params=NULL, ...) {
switch(endpointName,
#' @section Endpoint /{apiVersion}/operation/cellbase/configure:
#' Update Cellbase configuration.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param annotationUpdate Create and load variant annotations into the database.
#' @param annotationSaveId Save a copy of the current variant annotation at the database.
#' @param data New cellbase configuration.
configureCellbase=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="cellbase",
subcategoryId=NULL, action="configure", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/aggregate:
#' Find variants where not all the samples are present, and fill the empty values, excluding HOM-REF (0/0) values.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant aggregate params.
aggregateVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="variant",
subcategoryId=NULL, action="aggregate", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/annotation/delete:
#' Deletes a saved copy of variant annotation.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param annotationId Annotation identifier.
deleteVariantAnnotation=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/annotation", subcategoryId=NULL, action="delete", params=params,
httpMethod="DELETE", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/annotation/index:
#' Create and load variant annotations into the database.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant annotation index params.
indexVariantAnnotation=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/annotation", subcategoryId=NULL, action="index", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/annotation/save:
#' Save a copy of the current variant annotation at the database.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param data Variant annotation save params.
saveVariantAnnotation=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/annotation", subcategoryId=NULL, action="save", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/configure:
#' Update Variant Storage Engine configuration. Can be updated at Project or Study level.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Configuration params to update.
configureVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="variant",
subcategoryId=NULL, action="configure", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/delete:
#' Remove variant files from the variant storage.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant delete file params.
deleteVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="variant",
subcategoryId=NULL, action="delete", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/family/aggregate:
#' Find variants where not all the samples are present, and fill the empty values.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant aggregate family params.
aggregateVariantFamily=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/family", subcategoryId=NULL, action="aggregate", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/family/index:
#' DEPRECATED: integrated in index (DEPRECATED Build the family index).
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant family index params.
indexVariantFamily=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/family", subcategoryId=NULL, action="index", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/index:
#' Index variant files into the variant storage.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant index params.
indexVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="variant",
subcategoryId=NULL, action="index", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/index/launcher:
#' Detect non-indexed VCF files in the study, and submit a job for indexing them.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data .
launcherVariantIndex=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/index", subcategoryId=NULL, action="launcher", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/julie/run:
#' Transform VariantStats into PopulationFrequency values and updates the VariantAnnotation.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param project project.
#' @param data Julie tool params. Specify list of cohorts from multiple studies with {study}:{cohort}.
runVariantJulie=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/julie", subcategoryId=NULL, action="run", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/metadata/repair:
#' Execute some repairs on Variant Storage Metadata. Advanced users only.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Variant storage metadata repair params.
repairVariantMetadata=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/metadata", subcategoryId=NULL, action="repair", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/metadata/synchronize:
#' Synchronize catalog with variant storage metadata.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant storage metadata synchronize params.
synchronizeVariantMetadata=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/metadata", subcategoryId=NULL, action="synchronize", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/prune:
#' Prune orphan variants from studies in a project.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Variant prune params. Use dry-run to just generate a report with the orphan variants.
pruneVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="variant",
subcategoryId=NULL, action="prune", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/sample/delete:
#' Remove variant samples from the variant storage.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant delete sample params.
deleteVariantSample=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/sample", subcategoryId=NULL, action="delete", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/sample/index:
#' DEPRECATED You should use the new sample index method instead.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant sample index params.
indexVariantSample=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/sample", subcategoryId=NULL, action="index", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/sample/index/configure:
#' DEPRECATED You should use the new sample index configure method.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param skipRebuild Skip sample index re-build.
#' @param data New SampleIndexConfiguration.
variantSampleIndexConfigure=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/sample/index", subcategoryId=NULL, action="configure", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/score/delete:
#' Remove a variant score in the database.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param name Unique name of the score within the study.
#' @param resume Resume a previously failed remove.
#' @param force Force remove of partially indexed scores.
deleteVariantScore=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/score", subcategoryId=NULL, action="delete", params=params, httpMethod="DELETE",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/score/index:
#' Index a variant score in the database.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant score index params. scoreName: Unique name of the score within the study. cohort1: Cohort used to compute the score. Use the cohort 'ALL' if all samples from the study where used to compute the score. cohort2: Second cohort used to compute the score, typically to compare against the first cohort. If only one cohort was used to compute the score, leave empty. inputColumns: Indicate which columns to load from the input file. Provide the column position (starting in 0) for the column with the score with 'SCORE=n'. Optionally, the PValue column with 'PVALUE=n'. The, to indicate the variant associated with the score, provide either the columns ['CHROM', 'POS', 'REF', 'ALT'], or the column 'VAR' containing a variant representation with format 'chr:start:ref:alt'. e.g. 'CHROM=0,POS=1,REF=3,ALT=4,SCORE=5,PVALUE=6' or 'VAR=0,SCORE=1,PVALUE=2'. resume: Resume a previously failed indexation.
indexVariantScore=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/score", subcategoryId=NULL, action="index", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/secondary/annotation/index:
#' Creates a secondary index using a search engine. If samples are provided, sample data will be added to the secondary index. (New!).
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant secondary annotation index params.
variantSecondaryAnnotationIndex=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/secondary/annotation", subcategoryId=NULL, action="index", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/secondary/sample/index:
#' Build and annotate the sample index. (New!) .
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant sample index params.
variantSecondarySampleIndex=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/secondary/sample", subcategoryId=NULL, action="index", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/secondary/sample/index/configure:
#' Update SampleIndex configuration (New!).
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param skipRebuild Skip sample index re-build.
#' @param data New SampleIndexConfiguration.
configureVariantSecondarySampleIndex=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/secondary/sample/index", subcategoryId=NULL, action="configure", params=params,
httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/secondaryIndex:
#' DEPRECATED you should use the new annotation index method instead.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param project Project [organization@]project where project can be either the ID or the alias.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant secondary annotation index params.
secondaryIndexVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant", subcategoryId=NULL, action="secondaryIndex", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/secondaryIndex/delete:
#' Remove a secondary index from the search engine for a specific set of samples.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param samples Samples to remove. Needs to provide all the samples in the secondary index.
deleteVariantSecondaryIndex=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/secondaryIndex", subcategoryId=NULL, action="delete", params=params,
httpMethod="DELETE", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/setup:
#' Execute Variant Setup to allow using the variant engine. This setup is necessary before starting any variant operation.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant setup params.
setupVariant=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL, subcategory="variant",
subcategoryId=NULL, action="setup", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/stats/delete:
#' Deletes the VariantStats of a cohort/s from the database.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Variant stats delete params.
deleteVariantStats=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/stats", subcategoryId=NULL, action="delete", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/stats/index:
#' Compute variant stats for any cohort and any set of variants and index the result in the variant storage database.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param data Variant stats params.
indexVariantStats=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/stats", subcategoryId=NULL, action="index", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/operation/variant/study/delete:
#' Remove whole study from the variant storage.
#' @param jobId Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
#' @param jobDescription Job description.
#' @param jobDependsOn Comma separated list of existing job IDs the job will depend on.
#' @param jobTags Job tags.
#' @param jobScheduledStartTime Time when the job is scheduled to start.
#' @param jobPriority Priority of the job.
#' @param jobDryRun Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
#' @param study Study [[organization@]project:]study where study and project can be either the ID or UUID.
#' @param data Variant delete study params.
deleteVariantStudy=fetchOpenCGA(object=OpencgaR, category="operation", categoryId=NULL,
subcategory="variant/study", subcategoryId=NULL, action="delete", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
)
})
© 2015 - 2025 Weber Informatics LLC | Privacy Policy